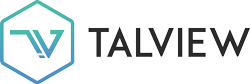
Introduction
The Talview API is organized around REST. Our API has predictable, resource-oriented URLs, and uses HTTP response codes to indicate API errors. We use built-in HTTP features, like HTTP codes and HTTP verbs, which are understood by off-the-shelf HTTP clients. We support cross-origin resource sharing (CORS), allowing you to interact securely with our API from a client-side web application (though you should never expose your secret credentials in any public website's client-side code). JSON is returned by all API responses, including errors.
There are some conventions followed throughout the API
- All object types unless virtual and explicity mentioned will have timestamp fields
created_at
andupdated_at
- All timestamp fields will contain timestamp in ISO 8601 format and will be UTC
- All list requests will be paginated
- All root level objects have a external_id attribute that can be used as reconicallation/ reference with primary keys of integrating system. This column is fully indexed and searchable,
Versioning
All Talview's API are versioned. The current API version is 4 . When we make backward-incompatible changes to API a new major version is released the new version is accessible at seperate endpoints. The previous version will continue to be accessible for existing customers and Talview will support your migration path to the new version
Pagination
All top-level API resources have support for bulk fetches via "list" API methods.
For instance you can list candidates
, list assessments
, and list questions
These list API methods share a common structure, taking at least these two parameters pageSize
and page
The following pagination information is included in all list requests using the HTTP headers
X-Pagination-Total-Count
: The total number of resources;X-Pagination-Page-Count
: The number of pages;X-Pagination-Current-Page
: The current page (1-based);X-Pagination-Per-Page
: The number of resources in each page;Link
: A set of navigational links allowing client to traverse the resources page by page.
Expanding Objects
Many objects contain the ID of a related object in their response properties.
For example, a Assessment
may have an associated Status
ID. Those objects can be expanded inline with the
expand request parameter. Objects that can be expanded are noted in this documentation.
This parameter is available on all API requests, and applies to the response of that request only.
You can nest expand requests with the dot property. For example, requesting candidate.status
on an invitation
will expand the candidate
property into a full Candidate
object, and will then expand the status
property
on that candidate
into a full status
object.
You can expand multiple objects at once by identifying multiple items in the expand array.
Authentication
Authenticate your account when using the API by including your secret API credentials in the request.
Your API credentials carry many privileges, so be sure to keep them secret! Do not share your secret API credentials
in publicly accessible areas such GitHub, client-side code, and so forth.
All API requests must be made over HTTPS. Calls made over plain HTTP will fail.
API requests without authentication will also fail.
All API requests are authenticated using OAuth2 Protocol. There are many supported grant types in the OAuth2 specification. Talview API v4 supports two grant_types
.
Resource Owner Password Credentials (i.e. password ) for all standard requests in all modules.
[Json Web Token (JWT) for Service Account requests used for request contexts where requests are made on behalf of another user in use cases such as Applicant Tracking System (ATS), Assessment Service Provider, Account Provisioning etc,
Resource Owner Password Credentials
A sample request
POST /oauth2/token HTTP/1.1
Host: apiv4.talview.com
Content-Type: application/json
{
"grant_type": "password",
"client_id": "YOUR CLIENT ID",
"client_secret": "YOUR CLIENT SECRET",
"username": "USERNAME",
"password": "PASSWORD"
}
Access Token sample output
{
"access_token": "64d496c5ccd6e912746b827711d5102e67524dde",
"expires_in": 86400,
"token_type": "Bearer",
"scope": null,
"refresh_token": "1c816754927a03e231bc20828dd00c3e9c6f21f9"
}
To acquire access token password grant type has to be used. Token can be refreshed using the refresh_token grant type by sending refresh token request. The request can have the parameters
Field | Type | Description |
---|---|---|
refresh_token (optional) | String | Refresh Token |
client_id | String | Client ID of the application |
client_secret | String | Client Secret of the application |
username | String | Username of the application |
password | String | Password of the application |
grant_type | String | Allowed values: refresh_token, password |
JSON Web Token (JWT)
A sample http request for JWT
POST /oauth2/token HTTP/1.1
Host: apiv4.talview.com
Content-Type: application/json
{
grant_type:"urn:ietf:params:oauth:grant-type:jwt-bearer",
assertion:"assertion"
}
If the request is successful you will recieve a response in this format
HTTP/1.1 200 OK
{
"access_token":"03807cb363bdf6c777d4df2455369c0d3b3c35",
"expires_in":3600,
"token_type": "bearer",
"scope": null
}
The service provider application makes a two step/ two legged OAuth (2LO) handshake call to the API on behalf of the Service Account and the users are not directly part of this process unlike the Three legged user authentication mechanisms used in other API calls.
Usually the service account is used to modify the service providers own data ( such as assessments etc.) rather than directly interact with user data. Once you Obtain the service provider client ID and private key from your Talview point of contact your application needs to execute this sequence of steps in order to make a request to Talview API
- Create a JSON Web Token (JWT) including a header, claim and a signature,
- Request for a Bearer Authorization token from Talview OAuth2 API authorization server
- Handle the response from Talview API server
- If the request is successful, then use the token to obtain access to resources on Talview API
- When the token expires, your application can make another JWT and requests another access token.
The rest of this section describes the specifics of creating a JWT, signing the JWT, forming the access token request, and handling the response.
Creating a JWT
A JWT is composed of three parts: a header, a payload, and a signature. The header and payload are JSON objects. These JSON objects are serialized to UTF-8 bytes, then encoded using the Base64url encoding. This provides resilience against encoding changes due to repeated encoding operations. The header, payload, and signature are concatenated together with a period (.) character.
A JWT is composed as:
{Base64url encoded header}.{Base64url encoded payload}.{Base64url encoded signature}
The base string for the signature is :
{Base64url encoded header}.{Base64url encoded payload}
Forming the JWT header
The header consists of two fields that indicate the signing algorithm and the format of the assertion. Both fields are mandatory, and each field has only one value. As additional algorithms and formats are introduced, this header will change accordingly. Service accounts rely on the RSA SHA-256 algorithm and the JWT token format. As a result, the JSON representation of the header is :
{"alg":"RS256","typ":"JWT"}
The Base64url representation of this is :
eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCJ9
Forming the JWT payload
The JWT payload contains information about the JWT, including the permissions being requested (scopes), the target of the token, the issuer, the time the token was issued, and the lifetime of the token. Most of the fields are mandatory. Like the JWT header, the JWT claim set is a JSON object and is used in the calculation of the signature.
Required claims : The required claims are as below, they may appear in any order in the claim set.
Name | Description |
---|---|
iss | The email address of the service account |
scope | A space-delimited list of the permissions that the application requests |
aud | A descriptor of the intended target of the assertion. When making an access token request this value is always https://apiv4.talview.com/oauth2/token |
exp | The expiration time of the assertion, specified as seconds since 00:00:00 UTC, January 1, 1970 (UNIX epoch time) . This value has a maximum of 24 hour after the issued time. |
iat | The time the assertion was issued, specified as seconds since 00:00:00 UTC, January 1, 1970 (UNIX epoch time) |
Like the JWT header, the JWT payload should be serialized to UTF-8 and Base64url-safe encoded.
A example of a JWT payload
{
"iss":"myserviceaccount@talview.com ",
"scope": "section evaluation invitation",
"aud":"https://apiv4.talview.com/oauth2/token ",
"exp": 1451937316,
"iat": 1451937316
}
Computing the signature
The JWT header and payload is signed using the JSON web signature JWS. The input for the signature is the byte array of the content:
{Base64url encoded header}.{Base64url encoded claim set}
The signing algorithm in the JWT header must be used when computing the signature. Talview currently supports the RSA method using SHA-256
hashing algorithm. This is expressed as RS256 in the alg field in the JWT header. Sign the UTF-8 representation of the input using SHA256withRSA
(also known as RSASSA-PKCS1-V1_5-SIGN
with the SHA-256
hash function) with the private key obtained from your account manager. The output will be a byte array. The signature must then be Base64url encoded. The header, payload, and signature are concatenated together with a period (.) character. The result is the JWT. It should be {Base64url encoded header}. {Base64url encoded claim set}. {Base64url encoded signature}
An example of a JWT before Base64url encoding:
{"alg":"RS256","typ":"JWT"}.
{
"iss":"myserviceaccount@talview.com ",
"scope": "section evaluation invitation",
"aud":"https://apiv4.talview.com/oauth2/ token ",
"exp": 1451937316,
"iat": 1451937316
}.
[signature bytes]
Making the access token request
After generating the signed JWT, your application can use it to request an access token. This access token request is an HTTPS POST request, and the body is URL encoded. The request needs to be made to the token url: https://apiv4.talview.com.com/oauth2/token
These parameters are required in the HTTPS POST request:
Name | Description |
---|---|
grant_type | Use the string (URL-encoded as necessary): urn:ietf:params:oauth:grant-type:jwt-bearer |
assertion | The JWT, including signature. |
Access tokens usually expire in 1 hour and can be reused as many times as required until the time they expire. On expiry a fresh JWT token needs to be generated and a fresh access token needs to be generated.
The 2 stepped authentication, is the standard the Oauth 2.0 JWT token generation and authorization mechanism, there are existing libraries in your language/framework of choice which has already implemented this authorization mechanism for you, refer to your language / framework documentation for configuring the same.
HTTP Response Code
A request with error
GET /some-end-point
HTTP/1.1
Host: apiv4.talview.com
Accept: application/json
Content-Type: application/json
Authorization: Bearer TOKEN
If the response has error, it will be in the following format with error suppression off
HTTP/1.1 400 Bad Request
Content-Type: application/json
{
"name": "Bad Request",
"message": "Error message",
"code": 0,
'status': 400
}
With error suppression the same request will be
GET /some-end-point?suppress_error_code=true
HTTP/1.1
Host: apiv4.talview.com
Accept: application/json
Content-Type: application/json
Authorization: Bearer TOKEN
the response will be
HTTP/1.1 200 OK
Content-Type: application/json
{
"success": false,
"data": {
"name": "Bad Request",
"message": "Error message",
"code": 0,
"status": 400
}
}
Talview uses conventional HTTP response codes to indicate the success or failure of an API request. In general, codes in the 2xx range indicate success, codes in the 4xx range indicate an error that failed given the information provided (e.g., a required parameter was omitted etc), and codes in the 5xx range indicate an error with our servers (these are rare). The following error codes are used in the application
HTTP Code | Description |
---|---|
200 | OK. Everything worked as expected. |
201 | A resource was successfully created in response to a POST request. The Location header contains the URL pointing to the newly created resource. |
204 | The request was handled successfully and the response contains no body content (like a DELETE request) |
304 | The resource was not modified. You can use the cached version. |
400 | Bad request. This could be caused by various actions by the user, such as providing invalid JSON data in the request body, providing invalid action parameters, etc. |
401 | Authentication failed. |
403 | The authenticated user is not allowed to access the specified API endpoint. |
404 | The requested resource does not exist. |
405 | Method not allowed. Please check the Allow header for the allowed HTTP methods. |
415 | Unsupported media type. The requested content type or version number is invalid. |
422 | Data validation failed (in response to a POST request, for example). Please check the response body for detailed error messages. |
429 | Too many requests. The request was rejected due to rate limiting. |
500 | Internal server error. This could be caused by internal program errors. |
Supressing Error Codes
HTTP Code Suppression
If the client does not support HTTP error codes the suppress_response_code
parameter can be used to
suppress error code in HTTP response.
Suppress error response codes using the suppress_error_code
parameter
Helpers
Collection of helpers API endpoints
Profile Image Placeholder
This end point generates image for a given text. Currently only FiraMono-Bold
monospace font is supported
Query Parameters
Parameter | Type | Mandatory | Comments |
---|---|---|---|
text | String | Yes | The text to be generated as image |
fillColor | String | No | Hex Code for foreground text color |
bgColor | String | No | Hex Code for background color |
x | Integer | No | Starting point of the text on x axis max: 1024 |
y | Integer | No | Starting point of the text on y axis max: 1024 |
height | Integer | No | Image height max: 1024 |
width | Integer | No | Image width max: 1024 |
fSize | Integer | No | Font Size of the text |
GET appv4.talview.com/api/image/placeholder?text=MK&fillColor=123456&bgColor=ccc
Will generate
List All Settings
GET stagingapi.talview.com/settings HTTP/1.1
Host: apiv4.talview.com
Authorization: Bearer token
Sample response
[
{
"id": 1,
"name": "Timezone",
"description": "Timezone",
"constrained": true,
"data_type": null,
"min_value": null,
"max_value": null
},
{
"id": 16,
"name": "template-261",
"description": null,
"constrained": true,
"data_type": null,
"min_value": null,
"max_value": null
}
]
End point for listing all types of allowed setting types such as timezone , user preference , colors, optional configurations. Some settings are constrained, i.e. can take be set to preset values, rest are unconstrained and can be set between min and max values
Get A Setting
GET stagingapi.talview.com/settings/1 HTTP/1.1
Host: apiv4.talview.com
Authorization: Bearer token
Sample response
{
"id": 1,
"name": "Timezone",
"description": "Timezone",
"constrained": true,
"data_type": null,
"min_value": null,
"max_value": null
}
Endpoint for getting a single setting
List All setting allowed values
GET stagingapi.talview.com/settings/1/allowed-value HTTP/1.1
Host: apiv4.talview.com
Authorization: Bearer token
Sample Response
[
{
"id": 143,
"settings_id": 1,
"item_value": "Pacific/Midway",
"caption": "(GMT-11:00) Midway Island, Samoa",
"created_at": "2015-05-12 18:24:32+00",
"updated_at": null
},
{
"id": 144,
"settings_id": 1,
"item_value": "America/Adak",
"caption": "(GMT-10:00) Hawaii-Aleutian",
"created_at": "2015-05-12 18:24:32+00",
"updated_at": null
}
]
End point for listing all allowed values for a setting. This end point is applicable only for settings of type constrained. If the settings is not constrained the response will be an empty array
Get a setting allowed value
GET stagingapi.talview.com/settings/1/allowed-value/143 HTTP/1.1
Host: apiv4.talview.com
Authorization: Bearer token
Sample Response
{
"id": 143,
"settings_id": 1,
"item_value": "Pacific/Midway",
"caption": "(GMT-11:00) Midway Island, Samoa",
"created_at": "2015-05-12 18:24:32+00",
"updated_at": null
}
Endpoint for getting a single setting allowed value for a given setting. Note the endpoint is nested within the settings end point
Me
Collection of actions for configuration and settings for the authenticated user i.e. self
The ME Object
{
"id": 41,
"external_id": "vikas",
"username": "user+tumgoucxkw2@talview.com",
"name": "vikas2",
"location": "India",
"is_common_login": true,
"email": "user+tumgoucxkw2@talview.com",
"roles": [
"Account Manager",
"Content Manager",
"Evaluator",
"Hiring Manager",
"Master Hiring Manager",
"Master Recruiter",
"Recruiter",
"Sourcer"
],
"organization_id": 2,
"phone": null,
"current_role": "Account Manager",
"status": 10,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2020-12-06 16:34:12+00",
"created_by": 1,
"updated_by": 1,
"logged_in_at": "2020-12-06 16:34:12+00",
"logged_in_from": "0.0.0.0",
"gravatarLink": "https://www.gravatar.com/avatar/30284b632a71f240d086e3d877b3e70d?s=100&d=mm&r=g",
"appState": null,
"profilePicUrl": null,
"defaultLocale": "en-US",
"countryCode": null,
"password_reset_token": "",
"isPrivacyAccepted": true,
"isTermsAccepted": true,
"_links": {
"self": {
"href": "/user/me"
},
"permissions": {
"href": "/user/me/permission?type=2"
},
"settings_data": {
"href": "/user/me/settings_data"
},
"index": {
"href": "/user"
},
"metrics": {
"href": "/user/metrics/41"
},
"categories": {
"href": "/models/user-category?user_id=41"
},
"busyCalendar": {
"href": "/user/me/calendar?type=3&user_id=41&startsAt=1607272473"
},
"liveCalendar": {
"href": "/user/me/calendar?type=1&user_id=41&startsAt=1607272473"
},
"availability": {
"href": "/user/me/calendar?type=2&user_id=41&startsAt=1607272473"
},
"userProfilePic": {
"href": "/user/display-picture"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
external_id | string | The external id of the user |
username | string | The name of the user |
name | string | The profile name of the user |
location | string | The location of the user |
is_common_login | boolen | if true, common login for the user is allowed |
string | The email of the user | |
roles | array | The roles of the user |
organization_id | integer | The organization_id of the user |
phone | numeric | The phone number of the user |
current_role | string | The current role of the user |
status | integer | The status of the user |
logged_in_at | datetime | The time when the user last logged in |
logged_in_from | string | The IP address from which the user logged in |
gravatarLink | string | The user profile gravatar link |
appState | json | The appState object set to the user |
profilePicUrl | string | The url of the user profile picture |
defaultLocale | string | The default locale of the user |
countryCode | string | The country code of the user |
password_reset_token | string | The user password reset token |
isPrivacyAccepted | boolean | Whether the user has accepted the talview privacy policy(true) or not(false) |
isTermsAccepted | boolean | Whether the user has accepted the talview terms and conditions(true) or not(false) |
List of user status
status | status_id |
---|---|
NEW | 10 |
ACTIVE | 20 |
INACTIVE | 30 |
SUSPENDED | 40 |
Self
This endpoint retrieves info about me
Get state of current user
GET /user/me HTTP/1.1
Host: apiv4.talview.com
Authorization: Bearer token
the response object will look like
{
"id": 41,
"external_id": "vikas",
"username": "user+tumgoucxkw2@talview.com",
"name": "vikas2",
"location": "India",
"is_common_login": true,
"email": "user+tumgoucxkw2@talview.com",
"roles": [
"Account Manager",
"Content Manager",
"Evaluator",
"Hiring Manager",
"Master Hiring Manager",
"Master Recruiter",
"Recruiter",
"Sourcer"
],
"organization_id": 2,
"phone": null,
"current_role": "Account Manager",
"status": 10,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2020-12-06 16:34:12+00",
"created_by": 1,
"updated_by": 1,
"logged_in_at": "2020-12-06 16:34:12+00",
"logged_in_from": "0.0.0.0",
"gravatarLink": "https://www.gravatar.com/avatar/30284b632a71f240d086e3d877b3e70d?s=100&d=mm&r=g",
"appState": null,
"profilePicUrl": null,
"defaultLocale": "en-US",
"countryCode": null,
"password_reset_token": "",
"isPrivacyAccepted": true,
"isTermsAccepted": true,
"_links": {
"self": {
"href": "/user/me"
},
"permissions": {
"href": "/user/me/permission?type=2"
},
"settings_data": {
"href": "/user/me/settings_data"
},
"index": {
"href": "/user"
},
"metrics": {
"href": "/user/metrics/41"
},
"categories": {
"href": "/models/user-category?user_id=41"
},
"busyCalendar": {
"href": "/user/me/calendar?type=3&user_id=41&startsAt=1607272473"
},
"liveCalendar": {
"href": "/user/me/calendar?type=1&user_id=41&startsAt=1607272473"
},
"availability": {
"href": "/user/me/calendar?type=2&user_id=41&startsAt=1607272473"
},
"userProfilePic": {
"href": "/user/display-picture"
}
}
}
HTTP Request
GET https://apiv4.talview.com/user/me
This request does not support query parameters
Update Me
This end point updates the authenticated user
PUT /user/me HTTP/1.1
Host: apiv4.talview.com
Authorization: Bearer token
Sample response
{
"id": 41,
"external_id": "vikas",
"username": "user+tumgoucxkw2@talview.com",
"name": "vikas2",
"location": "India",
"is_common_login": true,
"email": "user+tumgoucxkw2@talview.com",
"roles": [
"Account Manager",
"Content Manager",
"Evaluator",
"Hiring Manager",
"Master Hiring Manager",
"Master Recruiter",
"Recruiter",
"Sourcer"
],
"organization_id": 2,
"phone": null,
"current_role": "Account Manager",
"status": 10,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2020-12-06T12:45:46+00:00",
"created_by": 1,
"updated_by": 1,
"logged_in_at": "2020-12-06 12:45:31+00",
"logged_in_from": "0.0.0.0",
"gravatarLink": "https://www.gravatar.com/avatar/30284b632a71f240d086e3d877b3e70d?s=100&d=mm&r=g",
"appState": null,
"profilePicUrl": null,
"defaultLocale": "en-US",
"countryCode": null,
"isPrivacyAccepted": true,
"isTermsAccepted": true,
"_links": {
"self": {
"href": "/user/me"
},
"permissions": {
"href": "/user/me/permission?type=2"
},
"settings_data": {
"href": "/user/me/settings_data"
},
"index": {
"href": "/user"
},
"metrics": {
"href": "/user/metrics/41"
},
"categories": {
"href": "/models/user-category?user_id=41"
},
"busyCalendar": {
"href": "/user/me/calendar?type=3&user_id=41&startsAt=1607258746"
},
"liveCalendar": {
"href": "/user/me/calendar?type=1&user_id=41&startsAt=1607258746"
},
"availability": {
"href": "/user/me/calendar?type=2&user_id=41&startsAt=1607258746"
},
"userProfilePic": {
"href": "/user/display-picture"
}
}
}
The update me request can have these body parameters
Parameter | Type | Mandatory | Comments | Default Value |
---|---|---|---|---|
external_id | String | NO | the external id of the user | NO |
status | Integer | NO | the status of the user | 20 |
phone | String | NO | the phone number of the user | NO |
location | String | NO | the location of user | NO |
current_role | String | NO | the current role of the user | NO |
cf | JSON String | NO | custom field object for the user | NO |
name | String | NO | the user profile name | NO |
appState | JSON String | NO | the appState to set for the user | NO |
roles | String | NO | the roles of the user. The list of allowed roles defined below | NO |
is_common_login | Boolean | NO | enable/disable common login | true |
isSingleSignOn | Boolean | NO | enable/disable SSO sign on | false |
username | NO | the name of the user, must be unique | NO | |
No | the email of the user, must be unique | NO | ||
countryCode | String | NO | the country code of the user | NO |
isPrivacyAccepted | Boolean | NO | whether the user has accepted talview privacy policy | false |
isTermsAccepted | Boolean | NO | whether the user has accepted talview terms and conditions | false |
List of allowed roles
Roles |
---|
Master Recruiter |
Recruiter |
Evaluator |
Update Password
POST /user/me/update-password HTTP/1.1
Host: stagingapi.talview.com
Authorization: Bearer token
Sample response
{
"current_password":"current_password",
"new_password":"&%^$",
"new_password_repeat":"&%^$"
}
The update password request requires these body parameters. All parameters are mandatory
Parameter | Type | Mandatory | Comments |
---|---|---|---|
current_password | String | Yes | the current password of the user |
new_password | String | Yes | the updated password |
new_passowrd_repeat | String | Yes | the updated password repeated |
Validation Errors
Field | Type |
---|---|
current_password | Invalid current password |
new_password | This is a weak password, Please set stronger password for your account |
new_password | Your new password is same as the old password. Please use another password |
new_password | new_password_repeat does not match new_password |
Password Strength
POST /user/me/password-strength HTTP/1.1
Host: apiv4.talview.com
Authorization: Bearer token
Content-Type: application/json
{
"password": "eight wide field"
}
Sample response
{
"score": 4
}
The strength of potential new password is validated. The strength is calculates taking into account attributes such as name, email current password . The password strength request requires these body parameters. All parameters are mandatory
Parameter | Type | Mandatory | Comments |
---|---|---|---|
password | String | Yes | password for which strength needs to be validated |
There are no custom validation errors for this request
Get 2 Factor Token
GET /user/me/generate-otp-uri HTTP/1.1
Host: apiv4.talview.com
Authorization: Bearer token
Sample response
{
"qr-code": "https://chart.googleapis.com/chart?chs=200x200&chld=M%7C0&cht=qr&chl=otpauth%3A%2F%2Ftotp%2FTalview%253Adarth.vader%2540talview.com%3Fissuer%3DTalview%26secret%3DI5BECQZTLBFE2USXKVETCRBWJE",
"secret": "I5BECQZTLBFE2USXKVETCRBWJE"
}
The two factor registration token request for enabling two factor authentication for the user. The qr code image url generates a totp request which can be read by apps supporting TOTP protocol such as Google Authenticator. There are no parameters supported for this request
List Settings Data
GET /user/me/settings_data HTTP/1.1
Host: apiv4.talview.com
Authorization: Bearer token
Sample response
[
{
"id": 46,
"settings_id": 2,
"settings_allowed_value_id": 131,
"value": "39",
"unconstrained_value": null,
"created_at": "2015-04-27 12:54:48+05:30",
"updated_at": null
}
]
Endpoint for all settings for the user currently applied. Settings applied is cascaded. User settings inherit Organization which in turn inherit application settings. Settings can be expanded or interacted with directly at (Settings) [#]
Get a single setting datum
GET /user/me/settings_data/46 HTTP/1.1
Host: apiv4.talview.com
Authorization: Bearer token
Sample response
{
"id": 46,
"settings_id": 2,
"settings_allowed_value_id": 131,
"value": "39",
"unconstrained_value": null,
"created_at": "2015-04-27 12:54:48+05:30",
"updated_at": null
}
Endpoint for specific settings by id
Admin
Category
The category endpoint lists the categories that belongs to an organization.
The Category Object
{
"id": 12,
"external_id": "421",
"name": "anony",
"type": 3,
"created_at": "2019-12-02 12:29:59+00",
"updated_at": "2019-12-03 05:29:52+00",
"created_by": 41,
"updated_by": 41,
"is_deleted": true,
"organization_id": 2
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
name | text | The name of the Category |
type | integer | The type of the Category. Find the list of types below. |
is_deleted | boolean | if true the category is deleted |
organization_id | integer | the organization id to whom the category belongs |
external_id | text | the external id of the Category |
Key | Value |
---|---|
TYPE_BUSINESS | 1 |
TYPE_SECTION | 2 |
TYPE_SKILL | 3 |
TYPE_PARAMETER | 100 |
List all Category
GET admin/organization/2/category HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 12,
"external_id": "421",
"name": "anony",
"type": 3,
"created_at": "2019-12-02 12:29:59+00",
"updated_at": "2019-12-03 05:29:52+00",
"created_by": 41,
"updated_by": 41,
"is_deleted": true,
"organization_id": 2
},
{
"id": 12,
"external_id": "421",
"name": "anony",
"type": 3,
"created_at": "2019-12-02 12:29:59+00",
"updated_at": "2019-12-03 05:29:52+00",
"created_by": 41,
"updated_by": 41,
"is_deleted": true,
"organization_id": 2
}
...
]
This endpoint returns all the details of categories.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/category
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
name | text | false | Filter records based on Category name |
type | integer | false | Filter records based on type of the Category |
is_deleted | boolean | false | if passed true, returns the deleted categories |
oid | integer | true | the organization id for whom the category belongs |
s | text | false | filters by text in name |
Retrieve a Category
GET admin/organization/<oid>/category/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 12,
"external_id": "421",
"name": "anony",
"type": 3,
"created_at": "2019-12-02 12:29:59+00",
"updated_at": "2019-12-03 05:29:52+00",
"created_by": 41,
"updated_by": 41,
"is_deleted": true,
"organization_id": 2
}
This endpoint retrieves a category based on the id passed
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/category/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create a Category
POST admin/organization/<oid>/category HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"name": "category-test"
}
The above request returns JSON structured like this:
{
"id": 12,
"external_id": "421",
"name": "anony",
"type": 3,
"created_at": "2019-12-02 12:29:59+00",
"updated_at": "2019-12-03 05:29:52+00",
"created_by": 41,
"updated_by": 41,
"is_deleted": true,
"organization_id": 2
}
This endpoint creates a new category..
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/category
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
name | text | true | The name of the category |
type | integer | false | The type of the category |
Update an Category
PUT admin/organization/<oid>/category/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON PUT request
{
"name": "test-category",
"type": 1
}
The above request returns JSON structured like this:
{
"id": 12,
"external_id": "421",
"name": "anony",
"type": 3,
"created_at": "2019-12-02 12:29:59+00",
"updated_at": "2019-12-03 05:29:52+00",
"created_by": 41,
"updated_by": 41,
"is_deleted": true,
"organization_id": 2
}
Updates the category with the requested parameter.
HTTP Request
PUT https://apiv4.talview.com/admin/organization/<oid>/category/<id>
Url Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Delete a Category
DELETE /admin/organization/<oid>/category/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer toke
The above request returns the status code : 204 No Content
This endpoint allows you to delete an existing Category.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<oid>/category/<id>
Custom Field Master
An organization's custom fields.
The Custom Field Master Object
[
{
"id": 487,
"type": 2,
"sort_order": null,
"data_type": null,
"is_report": null,
"label": "today",
"key": "today test",
"updated_at": "2019-10-23 11:06:38+05:30",
"created_by": 30023,
"updated_by": 30023,
"created_at": "2019-10-23 11:04:18+05:30",
"is_hidden": false,
"is_deleted": false
}
]
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
type | smallint | Describes whether candidate or assessment type |
sort_order | smallint | The sort order value for custom field master |
is_report | boolean | Whether the report is available |
label | text | The label for the custom field |
key | varchar | Key for the upstream system |
is_hidden | boolean | type if true, the custom field master is hiddeni |
is_deleted | boolean | If true, the customer field master is deleted |
List all Custom Field Master
GET /admin/organization/<oid>/custom-field-master HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
[
{
"id": 487,
"type": 2,
"sort_order": null,
"data_type": null,
"is_report": null,
"label": "today",
"key": "today test",
"updated_at": "2019-10-23 11:06:38+05:30",
"created_by": 30023,
"updated_by": 30023,
"created_at": "2019-10-23 11:04:18+05:30",
"is_hidden": false,
"is_deleted": false
}
]
Returns all the details of custom field master.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/custom-field-master
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the custom field master |
id | integer | false | Filter based on id |
ids | array of id | false | filters by the ids provided |
type | smallint | false | Filter based on type |
label | varchar | false | Filter based on label |
key | varchar | false | Filter based on key |
is_deleted | boolean | false | if true, deleted custom field masters will be retrieved |
Retrieve a Custom Field Master
GET /models/custom-field-master/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
[
{
"id": 487,
"type": 2,
"sort_order": null,
"data_type": null,
"is_report": null,
"label": "today",
"key": "today test",
"updated_at": "2019-10-23 11:06:38+05:30",
"created_by": 30023,
"updated_by": 30023,
"created_at": "2019-10-23 11:04:18+05:30",
"is_hidden": false,
"is_deleted": false
}
]
Retrieve a custom field master.
HTTP Request
GET https://apiv4.talview.com/models/custom-field-master/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the custom filed master |
id | integer | true | Filter based on id |
Create a Custom Field Master
POST /admin/organization/<oid>/custom-field-master HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Body :
[
{
"id": 487,
"type": 2,
"sort_order": null,
"data_type": null,
"is_report": null,
"label": "today",
"key": "today test",
"updated_at": "2019-10-23 11:06:38+05:30",
"created_by": 30023,
"updated_by": 30023,
"created_at": "2019-10-23 11:04:18+05:30",
"is_hidden": false,
"is_deleted": false
}
]
The above command returns : 201 Created
Adds a custom field master.
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/custom-field-master
Body Parameters
Parameter | Type | isOptional | Description |
---|---|---|---|
type | integer | false | The type of the custom field master. The allowed types are mentioned below |
label | text | false | The label of the custom field |
key | text | false | Key for the upstream system |
sort_order | smallint | false | The sort order value for custom field master |
is_report | boolean | true | Whether the report is available |
Type
Key | Value |
---|---|
ASSESSMENT | 1 |
CANDIDATE | 2 |
Update a Custom Field Master
PUT /organization/<oid>/custom-field-master/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns :
[
{
"id": 487,
"type": 2,
"sort_order": null,
"data_type": null,
"is_report": null,
"label": "today",
"key": "today test",
"updated_at": "2019-10-23 11:06:38+05:30",
"created_by": 30023,
"updated_by": 30023,
"created_at": "2019-10-23 11:04:18+05:30",
"is_hidden": false,
"is_deleted": false
}
]
Updates a custom field master.
HTTP Request
PUT https://apiv4.talview.com/organization/<oid>/custom-field-master/<id>
URL Parameters
Parameter | Type | is Optional | Description |
---|---|---|---|
oid | integer | false | the organization ifd of the custom field master |
id | integer | false | The unique identifier of the object |
Body Parameters
Parameter | Type | isOptional | Description |
---|---|---|---|
type | integer | true | Describes whether candidate or assessment type |
label | text | true | The label of the custom field |
key | text | true | Key for the upstream system |
sort_order | smallint | true | The sort order value for custom field master |
is_hidden | boolean | true | if true, the custom field master is hiddeni |
is_deleted | boolean | true | If true, the customer field master is deleted |
Delete a Custom Field Master
DELETE /admin/organization/<oid>/custom-field-masterin/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns : 204 No Content
Delete a custom field master.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<oid>/custom-field-masterin/<id>
DevOps
The DevOps endpoint contains the following actions :
1. TbiGenerate - to generate Tbi report for assessment candidates
2. Process Async - to convert asynchronous videos manually
3. ProcessSpt - to generate speech to text for assessment candidates
The TbiGenerate action
POST /admin/dev-ops/tbi-generate HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"assessment_candidate_id":5,
"role":"test",
"text":true
}
The above request returns JSON structured like this:
{
"response": "TBI report is getting processed"
}
This endpoint generates the TBI report for a candidate who has completed the assessment.
HTTP Request
POST https://apiv4.talview.com/admin/dev-ops/tbi-generate
Body Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
assessment_candidate_id | integer | false | The id of the invite(assessment_candidate) |
role | text | true | Configuration name for Tbi report generation |
text | Boolean | true | If true, the report will be generated for the assessment candidates for whom the answer has text(converted by spt service) |
The ProcessSpt action
POST /admin/dev-ops/process-spt HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
This endpoint processes the answers of an assessment candidate and converts the speech in the answer to text.
HTTP Request
POST https://apiv4.talview.com/admin/dev-ops/process-spt
Body Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
assessmentCandidates | array | Integer | false |
force | boolean | true | The flag to run spt |
Process Async
The endpoint is used to convert asynchronous videos manually.
POST /admin/dev-ops/process-async HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"candidate_id": 5545420
}
The above request returns JSON structured like this:
{
"response": "Conversion in progress"
}
HTTP Request
POST https://apiv4.talview.com/admin/dev-ops/process-async
Body Parameter
Parameter | Type | Mandatory | Description |
---|---|---|---|
candidate_id | integer | true | candidate id for which the answer videos needs to be converted |
Form
The Form endpoint is used to perform CRUD on form.
The Form Object
{
"id": 6,
"name": "form3",
"structure": [
{
"id": 1,
"name": "Assessment form",
"fields": {
"1": {
"id": "cf_fn_name",
"name": "fname",
"type": "text",
"label": "First Name",
"autocomplete": "on"
},
"2": {
"id": "cf_ln_name",
"name": "lname",
"type": "text",
"label": "Last Name",
"autocomplete": "on"
}
},
"expandable": false
}
],
"url": "custom-url",
"type": 1,
"status": null,
"start_date": null,
"end_date": null,
"fieldKeys": [],
"is_public": null,
"article_id": null,
"custom_url": "custom-url",
"position_id": null,
"organization_id": "3",
"is_editable": null,
"created_at": "2019-12-16T12:15:50+00:00",
"updated_at": "2019-12-16T12:15:50+00:00",
"is_deleted": false
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
name | text | The name of the Form |
structure | jsonb | The structure of the Form in Json format |
url | text | The url of for the Form |
type | smallint | The type of Form. Example: Evaluation Form, Feedback From etc. The Form Type are listed below |
status | smallint | The status of the Form |
start_date | timestamptz | The date and time from when the Form is active |
end_date | timestamptz | The date and time from when the Form is deactivate |
fieldKeys | jsonb | It describes the required field in the form and is in Json format |
is_public | boolean | States whether the form is public or not |
custom_url | text | The url for the custom Forms |
organization_id | integer | The id of the Organization |
is_editable | boolean | States whether the Form is editable or |
article_id | integer | the article_id of thr form |
position_id | integer | the position_id of the form |
is_deleted | boolean | if true, the model is deleted |
List of Form Types:
Form Types | Key | Description |
---|---|---|
Web | 1 | Web Form |
Evaluation | 2 | Evaluation Form |
Assessment Candidate | 3 | Assessment Candidate Form |
Feedback | 4 | Feedback Form |
Requisition | 5 | Requisition Form |
Assessment Feedback | 6 | Assessment Feedback Form |
Fitment | 7 | Fitment Form |
Background Check Submission | 8 | Background Check Submission Form |
Candidate Verification | 9 | Candidate Verification Form |
On Boarding | 10 | On Boarding Form |
Offer Prepare | 11 | Offer Prepare Form |
Offer Candidate | 12 | Offer Candidate Form |
Offer Make | 13 | Offer Make Form |
Background Check Info Request | 14 | Background Check Info Request Form |
On Boarding User | 15 | On Boarding User Form |
On Boarding Candidate | 16 | On Boarding Candidate Form |
Candidate Data | 17 | Candidate Data Form |
Custom Assessment Section Candidate | 18 | Custom Assessment Section Candidate Form |
Event Registration | 19 | Form to register an event |
List all Forms
GET admin/organization/2/form HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 6,
"name": "form3",
"structure": [
{
"id": 1,
"name": "Assessment form",
"fields": {
"1": {
"id": "cf_fn_name",
"name": "fname",
"type": "text",
"label": "First Name",
"autocomplete": "on"
},
"2": {
"id": "cf_ln_name",
"name": "lname",
"type": "text",
"label": "Last Name",
"autocomplete": "on"
}
},
"expandable": false
}
],
"url": "custom-url",
"type": 1,
"status": null,
"start_date": null,
"end_date": null,
"fieldKeys": [],
"is_public": null,
"article_id": null,
"custom_url": "custom-url",
"position_id": null,
"organization_id": "3",
"is_editable": null,
"created_at": "2019-12-16T12:15:50+00:00",
"updated_at": "2019-12-16T12:15:50+00:00",
"is_deleted": false
}
]
This endpoint returns the details of all forms
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/form
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the form |
id | integer | false | filters record by the id passed |
ids | array of ids | false | filter records by multiple ids |
name | text | false | The name of the Form |
type | smallint | false | The type of Form. Example: Evaluation Form, Feedback From etc. The Form Type are listed below |
article_id | integer | false | The id of the CMS [Article] |
is_deleted | boolean | false | if passed true, deleted models will be retrieved |
Create a Form
POST admin/organization/2/form HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST request
{
"type": 1,
"structure": [
{
"id": 1,
"name": "Assessment form",
"fields": {
"1": {
"id": "cf_fn_name",
"name": "fname",
"type": "text",
"label": "First Name",
"autocomplete": "on"
},
"2": {
"id": "cf_ln_name",
"name": "lname",
"type": "text",
"label": "Last Name",
"autocomplete": "on"
}
},
"expandable": false
}
],
"name": "form3",
"fieldKeys": [
"First Name",
"Last Name",
"Phone"
],
"custom_url": "custom-url"
}
The above request returns JSON structured like this:
Please refer to sample response above
This endpoint creates a form.
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/form
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the form |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
name | text | true | The name of the Form |
type | smallint | true | The type of Form. Example: Evaluation Form, Feedback From etc. The Form Type are listed above |
structure | array ofobjects | false | structure of the form |
url | text | false | the url to the form |
status | integer | false | the status f the form |
is_public | boolean | false | describes if the form public |
custom_url | text | false | the custom url to the form |
is_editable | boolean | false | if the form editable |
Update a Form
PUT admin/organization/2/form/1 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST request
{
"name": "form4"
}
The above request returns JSON structured like this:
{
"id": 6,
"name": "form3",
"structure": [
{
"id": 1,
"name": "Assessment form",
"fields": {
"1": {
"id": "cf_fn_name",
"name": "fname",
"type": "text",
"label": "First Name",
"autocomplete": "on"
},
"2": {
"id": "cf_ln_name",
"name": "lname",
"type": "text",
"label": "Last Name",
"autocomplete": "on"
}
},
"expandable": false
}
]
...
...
...
}
This endpoint updates a specific form.
HTTP Request
PUT https://apiv4.talview.com/admin/organization/<oid>/form/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the form |
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the form |
name | text | true | The name of the Form |
type | smallint | true | The type of Form. Example: Evaluation Form, Feedback From etc. The Form Type are listed above |
structure | array of objects | false | structure of the form |
url | text | false | the url to the form |
status | integer | false | the status of the form |
is_public | boolean | false | describes if the form public |
custom_url | text | false | the custom url to the form |
is_editable | boolean | false | if the form editable |
Delete a Form
DELETE admin/organization/<oid>/form/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This endpoint deletes a specific form.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<oid>/form/<id>
Menu Card
The endpoint is used to do CRUD on Menu Card. Menu Cards are job carts where assessments can be added and shared publicly.
The Menu Card Object
{
"id": 1,
"is_private": null,
"name": "test-menu-card",
"imageUrl": null,
"description": "menu-card-description",
"starts_at": null,
"code": 64399250,
"ends_at": null,
"organization_id": "2",
"created_at": "2019-11-28T00:56:29+00:00",
"updated_at": "2019-11-28T00:56:29+00:00",
"created_by": 41,
"updated_by": 41,
"is_deleted": false,
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
is_private | boolean | Status whether Menu Card is private or not |
name | string | The name of the Menu Card |
imageUrl | string | The url for the Organization logo |
description | string | The description of the Menu Card |
starts_at | timestamptz | The date and time from when the Menu Card Assessment is active |
code | integer | Random Code generated from the backend |
ends_at | timestamptz | The date and time when the Menu Card expires |
organization_id | integer | The id of the Organization for whom the Menu Card belongs to |
created_by | integer | The id of User who created the Menu Card |
updated_by | integer | The id of the User who updated the Menu Card |
is_deleted | boolean | If true, menu card is deleted |
List Menu Card
GET admin/organization/2/menu-card HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1,
"is_private": null,
"name": "test-menu-card",
"imageUrl": null,
"description": "menu-card-description",
"starts_at": null,
"code": 64399250,
"ends_at": null,
"organization_id": "2",
"created_at": "2019-11-28T00:56:29+00:00",
"updated_at": "2019-11-28T00:56:29+00:00",
"created_by": 41,
"updated_by": 41,
"is_deleted": false,
}
This endpoint returns all the details of the menu cards.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/menu-card
Query Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
oid | integer | false | The organization of the Menu Card |
id | integer | true | Filter records based on the Id of the object |
ids | array of integer | true | Filter records based on the Ids of the objects |
code | intger | true | Filter records by given code |
is_deleted | boolean | true | if passed true, filters deleted records |
s | text | true | filters by text in name and code |
Retrieve a Menu Card
GET admin/organization/2/menu-card/1 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1,
"is_private": null,
"name": "test-menu-card",
"imageUrl": null,
"description": "menu-card-description",
"starts_at": null,
"code": 64399250,
"ends_at": null,
"organization_id": "2",
"created_at": "2019-11-28T00:56:29+00:00",
"updated_at": "2019-11-28T00:56:29+00:00",
"created_by": 41,
"updated_by": 41,
"is_deleted": false,
}
This endpoint retrieves a Menu Card based on the id passed
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/menu-card/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | The organization of the Menu Card |
id | integer | true | Filter records based on Id |
Create a Menu Card
POST admin/organization/2/menu-card HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"name": "test-menu-card",
"description": "menu-card-description"
}
The above request returns JSON structured like this:
{
"id": 1,
"is_private": null,
"name": "test-menu-card",
"imageUrl": null,
"description": "menu-card-description",
"starts_at": null,
"code": 64399250,
"ends_at": null,
"organization_id": "2",
"created_at": "2019-11-28T00:56:29+00:00",
"updated_at": "2019-11-28T00:56:29+00:00",
"created_by": 41,
"updated_by": 41,
"is_deleted": false,
}
This endpoint creates a new Menu Card..
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/menu-card
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
name | text | true | The name of the Menu Card |
is_private | boolean | false | Status whether Menu Card is private or not |
description | string | false | The description of the Menu Card |
starts_at | timestamptz | false | The date and time from when the Menu Card Assessment is active |
ends_at | timestamptz | false | The date and time when the Menu Card expires |
Update an Menu Card
PUT admin/organization/2/menu-card/1 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON PUT request
{
"is_private":true
}
The above request returns JSON structured like this:
{
"id": 1,
"is_private": true,
"name": "test-menu-card",
"imageUrl": null,
"description": "menu-card-description",
"starts_at": "2019-11-28 00:56:30+00",
"code": "64399250",
"ends_at": "2019-11-28 00:56:30+00",
"organization_id": 2,
"created_at": "2019-11-28 00:56:29+00",
"updated_at": "2019-11-28T01:17:41+00:00",
"created_by": 41,
"updated_by": 41,
"is_deleted": false,
}
Updates the Menu Card with the requested parameter.
HTTP Request
PUT https://apiv4.talview.com/admin/organization/<oid>/menu-card/<id>
Url Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Delete a Menu Card
DELETE /admin/organization/2/menu-card/1 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer toke
The above request returns the status code : 204 No Content
This endpoint allows you to delete an existing Menu Card.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<oid>/menu-card/<id>
Notification Log
The Notification Log endpoint gives the details of the notifications that have been sent to a candidate/recruiter/panel. By default the logged in user can view all the notifications sent for the past 1 hour irrespective of the organization. Organization specific notification logs can be viewed using query filters.
The Notification Log object
{
"id": 2889368,
"to_address": "sahana.b+161612@talview.com",
"cc_address": null,
"assessment_id": 727354,
"bcc_address": null,
"content": "<p>Dear sahana.b+161612@talview.com,\n</p>....................................",
"subject": "Activate your Talview account\n",
"created_at": "2019-10-18 16:25:24+05:30",
"created_by": 30023,
"sent_by": null,
"notification_template_type_id": 108,
"updated_at": "2019-10-18 16:25:24+05:30",
"status": "enqueued",
"scheduled_at": "2019-10-18 16:30:50+05:30",
"sent_at": "2019-10-18 16:30:50+05:30",
"read_at": null,
"from_address": "Cognizant <talentacquisition@cognizant.com>",
"type_id": 108,
"organization_id": 496,
"candidate_id": null,
"assessment_section_candidate_id": null,
"assessment_candidate_id": null,
"_links": {
"assessment": {
"href": "/models/assessment/view"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
to_address | the address to whom the email will be sent | |
cc_address | CC address | |
assessment_id | integer | the id of the assessment |
bcc_address | BCC address | |
content | text | The content of notification template |
subject | text | The subject of the notification template |
created_at | timestamptz | The time when the notification was created |
created_by | integer | The id of the user who created the notificarion |
sent_by | integer | The id of the user who has sent the notification |
notification_template_type_id | integer | The id of the notification template type |
updated_at | timestamptz | The time when the notification template type was updated |
status | integer | The status of the notification |
scheduled_at | timestamptz | The time when the assessment was scheduled |
sent_at | timestamptz | The time when the the notification was sent |
read_at | timestamptz | The time when the notification was read by the receiver |
from_address | The email address of the sender | |
type_id | integer | The type id |
organization_id | Integer | The identifier of the organization |
candidate_id | Integer | The identifier of the candidate |
assessment_section_candidate_id | Integer | The identifier of the assessment section candidate |
assessment_candidate_id | Integer | true |
assessment_id | integer | true |
List all Notification Log
GET /admin/notification-log HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 2889368,
"to_address": "sahana.b+161612@talview.com",
"cc_address": null,
"assessment_id": 727354,
"bcc_address": null,
"content": "<p>Dear sahana.b+161612@talview.com,\n</p>....................................",
"subject": "Activate your Talview account\n",
"created_at": "2019-10-18 16:25:24+05:30",
"created_by": 30023,
"sent_by": null,
"notification_template_type_id": 108,
"updated_at": "2019-10-18 16:25:24+05:30",
"status": "enqueued",
"scheduled_at": "2019-10-18 16:30:50+05:30",
"sent_at": "2019-10-18 16:30:50+05:30",
"read_at": null,
"from_address": "Cognizant <talentacquisition@cognizant.com>",
"type_id": 108,
"organization_id": 496,
"candidate_id": null,
"assessment_section_candidate_id": null,
"assessment_candidate_id": null,
"_links": {
"assessment": {
"href": "/models/assessment/view"
}
}
}
This endpoint returns all the details of notification logs for all the organizations.
HTTP Request
GET https://apiv4.talview.com/admin/notification-log
Query Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
type_id | integer | true | Filter records based on id |
status | Text | true | Filter records based on status of the notification |
organization_id | Integer | true | Filter records based on the organization_id |
from | date | true | Will display records greater than or equal to the given date |
to | date | true | Will display records lesser than or equal to the given date |
assessment_id | Integer | true | Filter records based on the assessment_id |
s | text | true | Filter records based on to_address, subject, content, from_address |
pageSize | Integer | true | Displays records according to the page size given |
candidate_id | Integer | true | Filter records based on the candidate id |
assessment_section_candidate_id | Integer | true | Filter records based on the assessment section candidate id |
assessment_candidate_id | Integer | true | Filter records based on the assessment candidate id |
label | text | true | Filter based on the label of notification template type |
View one Notification Log
GET /admin/notification-log/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 2889368,
"to_address": "sahana.b+161612@talview.com",
"cc_address": null,
"assessment_id": 727354,
"bcc_address": null,
"content": "<p>Dear sahana.b+161612@talview.com,\n</p>....................................",
"subject": "Activate your Talview account\n",
"created_at": "2019-10-18 16:25:24+05:30",
"created_by": 30023,
"sent_by": null,
"notification_template_type_id": 108,
"updated_at": "2019-10-18 16:25:24+05:30",
"status": "enqueued",
"scheduled_at": "2019-10-18 16:30:50+05:30",
"sent_at": "2019-10-18 16:30:50+05:30",
"read_at": null,
"from_address": "Cognizant <talentacquisition@cognizant.com>",
"type_id": 108,
"organization_id": 496,
"candidate_id": null,
"assessment_section_candidate_id": null,
"assessment_candidate_id": null,
"_links": {
"assessment": {
"href": "/models/assessment/view"
}
}
}
This endpoint returns the details of notification log for a particular id.
HTTP Request
GET https://apiv4.talview.com/admin/notification-log/<id>
Re-Send Notification
PATCH /admin/notification-log/<id>/resend HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
data : {
"organization_id":<oid>
}
The above request returns JSON structured like this:
{
"id": 4,
"to_address": "karthik.sg@talview.com",
"cc_address": null,
"assessment_id": 2,
"bcc_address": null,
"content": "<p>Dear xllri elece,\n</p><p>You have been invited to attend an interview for the position of All Sections. Last date to complete the assessment is Tuesday, October 29, 2019 at 12:00:00 AM India Standard Time.\n</p><p>\n</p><p>In case this is the first time you are attending an assessment from Talview you can refer to the <a href=\"https://info.talview.com/candidatehub\">Candidate Guide</a>. You can also attend a <strong>Practice Assessment</strong> by downloading the mobile app from <a href=\"https://play.google.com/store/apps/details?id=com.talview.candidate\">Play Store (Android)</a>/<a href=\"https://itunes.apple.com/us/app/talview-candidate-app/id1015320525?ls=1&mt=8\">Apple iTunes</a> and use the Demo Interview option. Please refer to the notes at the end of the mail for more details.\n</p><p>Once you are ready to take your official assessment please enter the verification code below in the app using Enter Code option in the home page.\n</p><p>Your verification code is <strong>318751043 </strong>\n</p><p>You can also scan <strong><img src=\"https://apiv4.talview.com/qrcode/318751043\" alt=\"\" /></strong>\n</p><p>\n</p><p>Alternatively if you want to attend the assessment from your laptop or desktop computer or if your assessment is not supported on mobile click 'Attend' link below and enter the code above. Please note that you can use this link only once.\n</p><p><a href=\"https://talview.page.link/UcvxVDLDZBP7CHC9A\">Attend</a>\n</p><p>Kindly call +91-80-6741-4716 or raise the support ticket using <a href=\"https://talview.freshdesk.com/support/tickets/new\">Raise Support Ticket</a> in case of any assistance with your assessment at the time of taking the assessment itself.\n</p><p><strong>Important Notes:</strong><br />\n</p><ol>\n\t<li>You must have an active Internet connection with a minimum speed of 256 Kbps and front camera to attend the assessment from smartphone or webcam, a microphone and speakers / headphones in order to attend the assessment from laptop or desktop.</li>\n\t<li>If you are attending the assessment from laptop or desktop computer, please use a good quality microphone ; inbuilt mic in laptop can be used but do not use mobile phone mic.</li>\n\t<li>Ensure that you complete it in advance to avoid last minutes technical snags.</li>\n\t<li>If you do not have a Android Smartphone to do the practice assessment you can click <a href=\"https://attend.talview.com/as/272263/landing\">here</a> to complete it from your laptop or desktop computer.</li>\n\t<li>The mobile applications only support Asynchronous or Automated Video Interviews and Video Proctored Objective Test. If you get a \"Not Supported\" message on entering the verification code in the mobile application or you have been specifically instructed by the prospective employer that the assessment is to write an essay or a code test, you need to use a laptop or desktop computer for the same.</li>\n\t<li>The list of supported browsers are Google Chrome (Latest Version) (49+), Mozilla Firefox (Latest Version) (21+), Internet Explorer (11+) and Microsoft Edge.</li>\n</ol><p>Best of Luck & Regards, company 1 -Public Ltd.\n</p><p>*************************************************************************************\n</p><p><small>This is a system generated message. Please do not reply to this email.</small>\n</p>",
"subject": "company 1 -Public Ltd. is inviting you to attend an assessment.",
"created_at": "2020-07-21 13:31:40+00",
"created_by": 41,
"sent_by": 41,
"notification_template_type_id": 210,
"updated_at": "2020-07-21 13:31:40+00",
"status": "enqueued",
"scheduled_at": "2020-07-21 13:31:39+00",
"sent_at": "2020-07-21 13:31:41+00",
"read_at": null,
"from_address": "\"company 1 -Public Ltd.\" <no-reply@talview.com>",
"type_id": 210,
"organization_id": 2,
"candidate_id": 139,
"assessment_section_candidate_id": 100099,
"assessment_candidate_id": 100,
"_links": {
"assessment": {
"href": "/models/assessment/2"
}
}
}
This endpoint re-send the email notification if the status is failed or enqueued and if the email has sent in the last 4 hours
HTTP Request
PATCH https://apiv4.talview.com/admin/notification-log/<id>/resend
URL Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
id | integer | false | notification log id |
Body Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
organization_id | integer | flase | organization_id of notification log |
Notification Template
The endpoint lists the Notification Templates that are used to notify consumers of the application.
The Notification Template Object
{
"id": 16,
"from_address": null,
"type_id": 802,
"content": "<!doctype html>\n<html>\n\t<head>\n\t\t<meta name=\"viewport\" content=\"width=device-width\">\n\t\t<meta http-equiv=\"Content-Type\" content=\"text/html; charset=UTF-8\">\n\t\t<title>Reset your Password</title>\n\t\t<style media=\"all\" type=\"text/css\">\n\t\t/* -------------------------------------\n\t\tCONFIGURE YOUR</body>\n",
"subject": "Reset your Password\n",
"category_id": null,
"organization_id": null,
"assessment_id": null,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"created_by": null,
"updated_by": null,
"is_deleted": false,
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
from_address | text | from the address the notification will get triggered |
type_id | integer | The type of the notification template type |
is_deleted | boolean | if true the notification template is deleted |
content | text | the content of the notification template |
subject | text | the subject of the notification template |
category_id | integer | the category of the notification |
organization_id | integer | the organization id to whom the notification template belongs |
assessment_id | integer | notification template for an specific assessment |
List all Notification Template
GET admin/organization/2/notification-template HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 16,
"from_address": null,
"type_id": 802,
"content": "<!doctype html>\n<html>\n\t<head>\n\t\t<meta name=\"viewport\" content=\"width=device-width\">\n\t\t<meta http-equiv=\"Content-Type\" content=\"text/html; charset=UTF-8\">\n\t\t<title>Reset your Password</title>\n\t\t<style media=\"all\" type=\"text/css\">\n\t\t/* -------------------------------------\n\t\tCONFIGURE YOUR</body>\n",
"subject": "Reset your Password\n",
"category_id": null,
"organization_id": null,
"assessment_id": null,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"created_by": null,
"updated_by": null,
"is_deleted": false,
}
...
]
This endpoint returns a lists of notification templates.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/notification-template
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
type_id | integer | false | filters records based on type of the notification template |
id | integer | false | filters records based on Id |
ids | Array of ids | false | filters records based on multiple ids |
is_deleted | boolean | false | if passed true, returns the deleted Notification Templates |
oid | integer | true | the organization id of the notification template |
assessment_id | integer | false | filters based on assessment id |
subject | text | false | filters by passed text in subject |
content | text | false | filters by passed text in content |
from_address | text | false | filters by the from_address passed as query param |
Retrieve a Notification Template
GET admin/organization/2/notification-template/16 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 16,
"from_address": null,
"type_id": 802,
"content": "<!doctype html>\n<html>\n\t<head>\n\t\t<meta name=\"viewport\" content=\"width=device-width\">\n\t\t<meta http-equiv=\"Content-Type\" content=\"text/html; charset=UTF-8\">\n\t\t<title>Reset your Password</title>\n\t\t<style media=\"all\" type=\"text/css\">\n\t\t/* -------------------------------------\n\t\tCONFIGURE YOUR</body>\n",
"subject": "Reset your Password\n",
"category_id": null,
"organization_id": null,
"assessment_id": null,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"created_by": null,
"updated_by": null,
"is_deleted": false,
}
This endpoint retrieves a notification template based on the id passed
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/notification-template/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
oid | integer | true | the organization id of the notification template |
Create a notification template
POST admin/organization/<oid>/notification-template HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"type_id":200,
"subject":"test-subject",
"content":"<!doctype html>\n<html>\n\t<head>\n\t\t<meta name=\"viewport\" content=\"width=device-width\">\n\t\t<meta http-equiv=\"Content-Type\" content=\"text/html; charset=UTF-8\">\n\t\t<title>Reset your Password</title>\n\t\t<style media=\"all\" type=\"text/css\">\n\t\t/* -------------------------------------\n\t\tCONFIGURE YOUR</body>\n"
}
The above request returns JSON structured like this:
{
"id": 16,
"from_address": null,
"type_id": 200,
"content": "<!doctype html>\n<html>\n\t<head>\n\t\t<meta name=\"viewport\" content=\"width=device-width\">\n\t\t<meta http-equiv=\"Content-Type\" content=\"text/html; charset=UTF-8\">\n\t\t<title>Reset your Password</title>\n\t\t<style media=\"all\" type=\"text/css\">\n\t\t/* -------------------------------------\n\t\tCONFIGURE YOUR</body>\n",
"subject": "test-subject",
"category_id": null,
"organization_id": null,
"assessment_id": null,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"created_by": null,
"updated_by": null,
"is_deleted": false,
}
This endpoint creates a new notification template..
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/notification-template
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
type_id | integer | true | The type of the notification template |
subject | text | true | The subject of the notification template |
content | text | true | The content of the notification template |
Update a notification template
PUT admin/organization/<oid>/notification-template/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON PUT request
{
"subject": "notification template subject"
}
The above request returns JSON structured like this:
{
"id": 16,
"from_address": null,
"type_id": 200,
"content": "<!doctype html>\n<html>\n\t<head>\n\t\t<meta name=\"viewport\" content=\"width=device-width\">\n\t\t<meta http-equiv=\"Content-Type\" content=\"text/html; charset=UTF-8\">\n\t\t<title>Reset your Password</title>\n\t\t<style media=\"all\" type=\"text/css\">\n\t\t/* -------------------------------------\n\t\tCONFIGURE YOUR</body>\n",
"subject": "notification template subject",
"category_id": null,
"organization_id": null,
"assessment_id": null,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"created_by": null,
"updated_by": null,
"is_deleted": false,
}
Updates the notification template with the requested parameter.
HTTP Request
PUT https://apiv4.talview.com/admin/organization/<oid>/notification-template/<id>
Url Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
oid | integer | true | the organization id of the |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
subject | text | false | The subject of the notification template |
content | text | false | The content of the notification template |
Delete a notification-template
DELETE /admin/organization/<oid>/notification-template/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This endpoint allows you to delete an existing notification template.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<oid>/notification-template/<id>
Notification Template Tag
The Notification Template Tag endpoint lists the notification template tags available which can be used in Notification Templates as variable.
The Notification Template Tag Object
{
"id": 827,
"tag": "{%assessment-name}",
"description": null,
"type_id": 220,
"created_at": "2019-11-28 09:59:56+00",
"updated_at": "2019-11-28T10:28:05+00:00",
"created_by": 41,
"updated_by": 41,
"is_deleted": false
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
tag | text | The tag. Example: {%assessment-name} |
description | text | The description of the Tag |
type_id | integer | The Id of the Notification Template Type |
is_deleted | boolean | If true, the notification tag is deleted |
List all Notification Template Tag
GET /admin/notification-template-tag HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 827,
"tag": "test-tag",
"description": null,
"type_id": 220,
"created_at": "2019-11-28 09:59:56+00",
"updated_at": "2019-11-28T10:28:05+00:00",
"created_by": 41,
"updated_by": 41,
"is_deleted": false
}
....
]
This endpoint returns all the details of the notification template tags.
HTTP Request
GET https://apiv4.talview.com/admin/notification-template-tag
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | filters records based on the Id of the object |
ids | array of integer | false | filters records based on the Ids of the objects |
tag | text | false | filters records based on Tag name. |
description | text | false | filters records based on the description of the Tag |
type_id | integer | false | filters records based on the Id of the Notification Template Type |
s | text | false | filters records by text in tag and description |
is_deleted | boolean | false | If passed true, only deleted tags will be retrieved |
Notification Template Type
The Notification Template Type endpoint gives the details of all the Notification Template available.
The Notification Template Type Object
{
"id": 315,
"label": "Remind",
"description": "Event Panel Attend Remind",
"type_id": 315,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"created_by": 41,
"updated_by": 41
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
label | text | The label of the notification template type |
description | text | description of the notification template type |
type_id | integer | The type id of the notification template type |
List all Notification Template Type
GET admin/notification-template-type HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 315,
"label": "Remind",
"description": "Event Panel Attend Remind",
"type_id": 315,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"created_by": 41,
"updated_by": 41
},
{
"id": 314,
"label": "Cancel",
"description": "Event Panel Register Cancel",
"type_id": 314,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"created_by": 41,
"updated_by": 41
}
...
]
This endpoint lists all notification template type.
HTTP Request
GET https://apiv4.talview.com/admin/notification-template-type
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
type_id | integer | false | Filters records based on type_id |
id | integer | false | Filter records based on id |
ids | array of integer | false | Filter records based on multiple ids |
description | text | false | filters by description text |
s | text | false | filters by text in description and label |
category | integer | false | fileters by category of the notification template type. Category 1 defines type "EMAIL" and 2 defines "SMS" |
Retrieve a Notification Template Type
GET admin/notification-template-type/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 315,
"label": "Remind",
"description": "Event Panel Attend Remind",
"type_id": 315,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"created_by": 41,
"updated_by": 41
}
This endpoint retrieves a notification template type based on the id passed
HTTP Request
GET https://apiv4.talview.com/admin/notification-template-type<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Organization
It stores the details of the organization.
The Organization Object
{
"id": 3,
"name": "company 2 -Pvt. Ltd.",
"external_id": null,
"domain": null,
"address1": null,
"address2": null,
"city": null,
"state": null,
"country": null,
"pincode": null,
"phone": null,
"isInterview": true,
"isLive": true,
"isObjective": true,
"isEssay": true,
"isCode": true,
"isEvaluation": false,
"promo": null,
"isTyping": false,
"isSimulation": false,
"logo": "https://assets.talview.com/images/talview_grey.png",
"advancedProctorV3Enabled": true,
"isUploadPreForm": null,
"defaultFont": "Roboto, sans-serif",
"isPearsonEnabled": false,
"created_by": 1,
"updated_by": null,
"senderEmailEnabled": "no",
"mailDateTimeFormat": "full",
"isPublicAttachment": true,
"updated_at": null,
"theme": "default",
"hideCandidateInfoPanel": false,
"termsLink": "https://pages.talview.com/terms/",
"hasAssessmentCreate": true,
"logoutUrl": "/login",
"timeZone": "Asia/Kolkata",
"isAdhocLive": true,
"isIconEvaluation": true,
"created_at": "2019-10-09 14:51:18+00",
"isMobileAssessment": true,
"isBpmEnabled": true,
"isBfiEnabled": true,
"live_bulk_schedule_enabled": false,
"bulk_schedule_url": null,
"chatbot_enabled": true,
"watermark_enabled": false,
"isPartnerAssessment": false,
"hasSmsNotify": true,
"isWhatsapp": false,
"cssSkin": null,
"isBubbleEvaluation": false,
"proviewToken": "U23A5311",
"formUrl": "https://forms.talview.com/candidate/default/index.html",
"meetDomain": "meet.talview.com",
"recruitDomain": "recruit.talview.com",
"postAssessmentUrl": null,
"description": null,
"brandingURL": null,
"customThankyouPage": null,
"is_deleted": false,
"isTbiEnabled": false,
"isAddFormEnabled": "1",
"isTbiMidwayCheckEnabled": null,
"fromAddress": "\"company 2 -Pvt. Ltd.\" <no-reply@talview.com>",
"proviewUrl": "https://cdn.proview.io/init.js",
"emailServiceType": "mailgun",
"calendarService": null,
"azureTenantID": null,
"customPublicPage": null,
"hasResumeMatch": false
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object. |
name | string | The name of the organization. |
external_id | string | The external id of an organization. |
domain | string | The domain name of an organization. |
address1 | string | The address1 of an organization. |
address2 | string | The address2 of an organization. |
city | string | The city of an organization. |
state | string | The state of an organization. |
country | string | The country of an organization. |
pincode | string | The pincode of an organization. |
phone | string | The phone of an organization. |
isInterview | boolean | Flag to enable/disable Interview for an organization. |
isLive | boolean | Flag to enable/disable Live Interview for an organization. |
isObjective | boolean | Flag to enable/disable Objective Test for an organization. |
isEssay | boolean | Flag to enable/disable Essay Test for an organization. |
isCode | boolean | Flag to enable/disable Codding test for an organization. |
isEvaluation | boolean | Flag to enable/disable Evaluation for an organization. |
promo | text | The promo of an organization. |
isTyping | boolean | Flag to enable/disable Typing Test for an organization. |
isSimulation | boolean | Flag to enable/disable simulation for an organization. |
advancedProctorV3Enabled | boolean | Flag to enable/disable advance v3 proctor for an organization. |
isUploadPreForm | boolean | Flag to enable/disable isUploadPreForm for an organization. |
defaultFont | string | The default font in an organization. |
isPearsonEnabled | boolean | pearson service enabled/disabled for an organization. |
created_by | integer | The id of the user who created an organization. |
updated_by | integer | The id of the user who updated an organization. |
senderEmailEnabled | boolean | Flag to enable/disable sender email for an organization. |
mailDateTimeFormat | text | The date-time format for email notifications. |
isPublicAttachment | boolean | Flag to set attachment public/private for an organization. |
updated_at | timestamptz | The time of updation of the an organization. |
theme | text | theme of an organization. |
hideCandidateInfoPanel | boolean | hideCandidateInfoPanel enabled for an organization. |
termsLink | text | Link of terms of an organization. |
hasAssessmentCreate | boolean | hasAssessmentCreate enabled for an organization. |
logoutUrl | text | The logoutUrl of an organization. |
timeZone | timestamptz | The timezone of an organization. |
isAdhocLive | boolean | Flag to enable/disable adhoc live for an organization. |
isIconEvaluation | boolean | isIconEvaluation enabled for an organization. |
created_at | timestamptz | The time of creation of the an organization. |
isMobileAssessment | boolean | Flag to enable/disable mobile assessment for an organization. |
isBpmEnabled | boolean | is behavioral profile match is enabled/disable for an organization. |
isBfiEnabled | boolean | is behavioral fitment index is enabled/disable for an organization. |
live_bulk_schedule_enabled | boolean | flag to enable or disable bulk schedule feature for an organization. |
bulk_schedule_url | string | The url to the bulk schedule of an organization. |
chatbot_enabled | boolean | Flag to enable/disable chatbot for an organization. |
watermark_enabled | boolean | Flag to enable or disable water mark feature for an organization. |
isPartnerAssessment | boolean | Flag to enable/disable partner assessment for an organization. |
hasSmsNotify | boolean | Flag to enable/disable sms service for an organization. |
isWhatsapp | boolean | Flag to enable/disable Whatsapp service for an organization. |
cssSkin | string | The url of CSS Skin Configuration of an organization. |
isBubbleEvaluation | boolean | Flag to enable/disable Bubbling up evaluation for an organization. |
proviewToken | string | The Proview Token Configuration of an organization. |
formUrl | text | The url of the form of an organization. |
meetDomain | text | The domain name of meet url of an organization. |
recruitDomain | text | The domain name for recruit application url of an organization. |
postAssessmentUrl | text | url of the post assessment page of an organization. |
description | text | The description of the an organization. |
brandingURL | text | The branding url of an organization. |
customThankyouPage | text | Thank you page of an organization. |
is_deleted | boolean | delete enabled/disabled for an organization. |
isTbiEnabled | boolean | isTbiEnabled enabled for an organization. |
isAddFormEnabled | boolean | add form configuration enabled/disabled for an organization. |
isTbiMidwayCheckEnabled | boolean | isTbiMidwayCheckEnabled enabled for an organization. |
fromAddress | text | The fromAddress of the an organization. |
proviewUrl | text | The proviewUrl of the an organization. |
emailServiceType | text | The type of email service configuration of the an organization |
calendarService | text | calendarService for an organization. |
azureTenantID | text | azureTenantID for an organization. |
customPublicPage | text | customPublicPage of an organization. |
hasResumeMatch | boolean | ResumeMatch feature enabled for an organization. |
Retrieve an Organization
GET admin/organization/3 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
{
"id": 3,
"name": "company 2 -Pvt. Ltd.",
"external_id": null,
"domain": null,
"address1": null,
"address2": null,
"city": null,
"state": null,
"country": null,
"pincode": null,
"phone": null,
"isInterview": true,
"isLive": true,
"isObjective": true,
"isEssay": true,
"isCode": true,
"isEvaluation": false,
"promo": null,
"isTyping": false,
"isSimulation": false,
"logo": "https://assets.talview.com/images/talview_grey.png",
"advancedProctorV3Enabled": true,
"isUploadPreForm": null,
"defaultFont": "Roboto, sans-serif",
"isPearsonEnabled": false,
"created_by": 1,
"updated_by": null,
"senderEmailEnabled": "no",
"mailDateTimeFormat": "full",
"isPublicAttachment": true,
"updated_at": null,
"theme": "default",
"hideCandidateInfoPanel": false,
"termsLink": "https://pages.talview.com/terms/",
"hasAssessmentCreate": true,
"logoutUrl": "/login",
"timeZone": "Asia/Kolkata",
"isAdhocLive": true,
"isIconEvaluation": true,
"created_at": "2019-10-09 14:51:18+00",
"isMobileAssessment": true,
"isBpmEnabled": true,
"isBfiEnabled": true,
"live_bulk_schedule_enabled": false,
"bulk_schedule_url": null,
"chatbot_enabled": true,
"watermark_enabled": false,
"isPartnerAssessment": false,
"formUrl": "https://forms.talview.com/candidate/default/index.html",
"meetDomain": "meet.talview.com",
"recruitDomain": "recruit.talview.com",
"postAssessmentUrl": null,
"description": null,
"brandingURL": null,
"customThankyouPage": null,
"is_deleted": false,
"isTbiEnabled": false,
"isAddFormEnabled": "1",
"isTbiMidwayCheckEnabled": null,
"fromAddress": "\"company 2 -Pvt. Ltd.\" <no-reply@talview.com>",
"proviewUrl": "https://cdn.proview.io/init.js",
"emailServiceType": "mailgun",
"calendarService": null,
"azureTenantID": null,
"customPublicPage": null,
"hasResumeMatch": false
}
Retrieves the details of an existing organization.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<organization_id>
URL Parameters
Parameter | Type | Description |
---|---|---|
id | integer | Filter records based on id |
Create an Organization
POST admin/organization HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"name": "company 2 -Pvt. Ltd.",
}
The above command returns JSON structured like this:
{
"id": 3,
"name": "company 2 -Pvt. Ltd.",
"external_id": null,
"domain": null,
"address1": null,
"address2": null,
"city": null,
"state": null,
"country": null,
"pincode": null,
"phone": null,
"isInterview": true,
"isLive": true,
"isObjective": true,
"isEssay": true,
"isCode": true,
"isEvaluation": false,
"promo": null,
"isTyping": false,
"isSimulation": false,
"logo": "https://assets.talview.com/images/talview_grey.png",
"advancedProctorV3Enabled": true,
"isUploadPreForm": null,
"defaultFont": "Roboto, sans-serif",
"isPearsonEnabled": false,
"created_by": 1,
"updated_by": null,
"senderEmailEnabled": "no",
"mailDateTimeFormat": "full",
"isPublicAttachment": true,
"updated_at": null,
"theme": "default",
"hideCandidateInfoPanel": false,
"termsLink": "https://pages.talview.com/terms/",
"hasAssessmentCreate": true,
"logoutUrl": "/login",
"timeZone": "Asia/Kolkata",
"isAdhocLive": true,
"isIconEvaluation": true,
"created_at": "2019-10-09 14:51:18+00",
"isMobileAssessment": true,
"isBpmEnabled": true,
"isBfiEnabled": true,
"live_bulk_schedule_enabled": false,
"bulk_schedule_url": null,
"chatbot_enabled": true,
"watermark_enabled": false,
"isPartnerAssessment": false,
"formUrl": "https://forms.talview.com/candidate/default/index.html",
"meetDomain": "meet.talview.com",
"recruitDomain": "recruit.talview.com",
"postAssessmentUrl": null,
"description": null,
"brandingURL": null,
"customThankyouPage": null,
"is_deleted": false,
"isTbiEnabled": false,
"isAddFormEnabled": "1",
"isTbiMidwayCheckEnabled": null,
"fromAddress": "\"company 2 -Pvt. Ltd.\" <no-reply@talview.com>",
"proviewUrl": "https://cdn.proview.io/init.js",
"emailServiceType": "mailgun",
"calendarService": null,
"azureTenantID": null,
"customPublicPage": null,
"hasResumeMatch": false
}
Creates a new organization.
HTTP Request
POST https://apiv4.talview.com/admin/organization
Body Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
name | string | false | The name of the organization. |
external_id | string | true | The external id of an organization. |
domain | string | true | The domain name of an organization. |
address1 | string | true | The address1 of an organization. |
address2 | string | true | The address2 of an organization. |
city | string | true | The city of an organization. |
state | string | true | The state of an organization. |
country | string | true | The country of an organization. |
pincode | string | true | The pincode of an organization. |
phone | string | true | The phone of an organization. |
isInterview | boolean | true | Flag to enable/disable Interview for an organization. |
isLive | boolean | true | Flag to enable/disable Live Interview for an organization. |
isObjective | boolean | true | Flag to enable/disable Objective Test for an organization. |
isEssay | boolean | true | Flag to enable/disable Essay Test for an organization. |
isCode | boolean | true | Flag to enable/disable Codding test for an organization. |
isEvaluation | boolean | true | Flag to enable/disable Evaluation for an organization. |
promo | text | true | The promo of an organization. |
isTyping | boolean | true | Flag to enable/disable Typing Test for an organization. |
isSimulation | boolean | true | Flag to enable/disable simulation for an organization. |
advancedProctorV3Enabled | boolean | true | Flag to enable/disable advance v3 proctor for an organization. |
isUploadPreForm | boolean | true | Flag to enable/disable isUploadPreForm for an organization. |
defaultFont | string | true | The default font in an organization. |
isPearsonEnabled | boolean | true | pearson service enabled/disabled for an organization. |
created_by | integer | true | The id of the user who created an organization. |
updated_by | integer | true | The id of the user who updated an organization. |
senderEmailEnabled | boolean | true | Flag to enable/disable sender email for an organization. |
mailDateTimeFormat | text | true | The date-time format for email notifications. |
isPublicAttachment | boolean | true | Flag to set attachment public/private for an organization. |
updated_at | timestamptz | true | The time of updation of the an organization. |
theme | text | true | theme of an organization. |
hideCandidateInfoPanel | boolean | true | hideCandidateInfoPanel enabled for an organization. |
termsLink | text | true | Link of terms of an organization. |
hasAssessmentCreate | boolean | true | hasAssessmentCreate enabled for an organization. |
logoutUrl | text | true | The logoutUrl of an organization. |
timeZone | timestamptz | true | The timezone of an organization. |
isAdhocLive | boolean | true | Flag to enable/disable adhoc live for an organization. |
isIconEvaluation | boolean | true | isIconEvaluation enabled for an organization. |
created_at | timestamptz | true | The time of creation of the an organization. |
isMobileAssessment | boolean | true | Flag to enable/disable mobile assessment for an organization. |
isBpmEnabled | boolean | true | is behavioral profile match is enabled/disable for an organization. |
isBfiEnabled | boolean | true | is behavioral fitment index is enabled/disable for an organization. |
live_bulk_schedule_enabled | boolean | true | flag to enable or disable bulk schedule feature for an organization. |
bulk_schedule_url | string | true | The url to the bulk schedule of an organization. |
chatbot_enabled | boolean | true | Flag to enable/disable chatbot for an organization . |
watermark_enabled | boolean | true | Flag to enable or disable water mark feature for an organization. |
isPartnerAssessment | boolean | true | Flag to enable/disable partner assessment for an organization. |
formUrl | text | true | The url of the form of an organization. |
meetDomain | text | true | The domain name of meet url of an organization. |
recruitDomain | text | true | The domain name for recruit application url of an organization. |
postAssessmentUrl | text | true | url of the post assessment page of an organization. |
description | text | true | The description of the an organization. |
brandingURL | text | true | The branding url of an organization. |
customThankyouPage | text | true | Thank you page of an organization. |
is_deleted | boolean | true | delete enabled/disabled for an organization. |
isTbiEnabled | boolean | true | isTbiEnabled enabled for an organization. |
isAddFormEnabled | boolean | true | add form configuration enabled/disabled for an organization. |
isTbiMidwayCheckEnabled | boolean | true | isTbiMidwayCheckEnabled enabled for an organization. |
fromAddress | text | true | The fromAddress of the an organization. |
proviewUrl | text | true | The proviewUrl of the an organization. |
emailServiceType | text | true | The type of email service configuration of the an organization. |
calendarService | text | true | calendarService for an organization. |
azureTenantID | text | true | azureTenantID for an organization. |
customPublicPage | text | true | customPublicPage of an organization. |
hasResumeMatch | boolean | true | ResumeMatch feature enabled for an organization. |
Update an Organization
This endpoint Update an organization.
PUT admin/organization/3 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"name": "company 3 -Pvt. Ltd.",
}
The above command returns JSON structured like this:
{
"id": 3,
"name": "company 3 -Pvt. Ltd.",
"external_id": null,
"domain": null,
"address1": null,
"address2": null,
"city": null,
"state": null,
"country": null,
"pincode": null,
"phone": null,
"isInterview": true,
"isLive": true,
"isObjective": true,
"isEssay": true,
"isCode": true,
"isEvaluation": false,
"promo": null,
"isTyping": false,
"isSimulation": false,
"logo": "https://assets.talview.com/images/talview_grey.png",
"advancedProctorV3Enabled": true,
"isUploadPreForm": null,
"defaultFont": "Roboto, sans-serif",
"isPearsonEnabled": false,
"created_by": 1,
"updated_by": null,
"senderEmailEnabled": "no",
"mailDateTimeFormat": "full",
"isPublicAttachment": true,
"updated_at": null,
"theme": "default",
"hideCandidateInfoPanel": false,
"termsLink": "https://pages.talview.com/terms/",
"hasAssessmentCreate": true,
"logoutUrl": "/login",
"timeZone": "Asia/Kolkata",
"isAdhocLive": true,
"isIconEvaluation": true,
"created_at": "2019-10-09 14:51:18+00",
"isMobileAssessment": true,
"isBpmEnabled": true,
"isBfiEnabled": true,
"live_bulk_schedule_enabled": false,
"bulk_schedule_url": null,
"chatbot_enabled": true,
"watermark_enabled": false,
"isPartnerAssessment": false,
"hasSmsNotify": true,
"isWhatsapp": false,
"cssSkin": null,
"isBubbleEvaluation": false,
"proviewToken": "U23A5311",
"formUrl": "https://forms.talview.com/candidate/default/index.html",
"meetDomain": "meet.talview.com",
"recruitDomain": "recruit.talview.com",
"postAssessmentUrl": null,
"description": null,
"brandingURL": null,
"customThankyouPage": null,
"is_deleted": false,
"isTbiEnabled": false,
"isAddFormEnabled": "1",
"isTbiMidwayCheckEnabled": null,
"fromAddress": "\"company 2 -Pvt. Ltd.\" <no-reply@talview.com>",
"proviewUrl": "https://cdn.proview.io/init.js",
"emailServiceType": "mailgun",
"calendarService": null,
"azureTenantID": null,
"customPublicPage": null,
"hasResumeMatch": false
}
HTTP Request
PUT https://apiv4.talview.com/admin/organization/<organization_id>
URl Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
id | integer | false | The unique identifier of the object |
Permission
The Permission endpoint gives the list of the master auth items.
List all permissions
GET /admin/permission HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The Response
[
"assessment.create",
"assessment.delete",
"assessment.duplicate",
"assessment.publish",
"assessment.share",
"assessment.update",
"assessment-owner.update",
"assessment-emailtemplate.update",
"assessment-jd.update",
"assessment-jd.create",
"candidate.create",
"candidate.bulk-upload",
"candidate-resume.create",
"candidate-resume.delete",
"candidate-resume.download",
"candidate.invite.create",
"candidate.invite.bulk-upload",
"candidate.invite.reminder",
"candidate.invite.delete",
"candidate.invite.extend-date",
"candidate.invite.status.update",
"live.schedule.create",
"live.schedule.cancel",
"live.reschedule",
"live.reminder",
"live.panel.assign",
"panel.assign",
"panel.availability",
"panel.reminder",
"panel.unassign",
"question.create",
"question.bulk-upload",
"question.delete",
"question.update",
"evaluate.create",
"assc.status.update",
"simulation.schedule",
"simulation.cancel",
"simulation.reschedule",
"owner.update",
"assessment.section.reinvite",
"add.education",
"delete.education",
"add.experience",
"delete.experience",
"event.share",
"event.create",
"event.update"
]
HTTP Request
GET https://apiv4.talview.com/admin/permission
Rbac
The Rbac endpoint gives the details of all the permissions available for a user/organization. The permission to be added should be present in auth_item table.
The RBAC Object
[
{
"id": 1516457,
"type": 2,
"user_id": 30876,
"organization_id": 488,
"item_name": "panel.assign",
"created_at": null
}
]
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the profile object |
type | integer | The type of the permission granted |
user_id | integer | The id of the user |
organization_id | integer | The id of the organization |
item_name | text | The label of the permission granted. (Based on the name described in auth_item) |
created_at | timestamp | The timestamp of when the permission was granted |
List of Item Types:
Type | Value |
---|---|
1 | TYPE_ROLE |
2 | TYPE_PERMISSION |
List all Permissions
GET /admin/organization/<oid>/rbac HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 1516457,
"type": 2,
"user_id": 30876,
"organization_id": 488,
"item_name": "panel.assign",
"created_at": null
}
]
Returns all the permissions granted for the user/organization.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/rbac
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
type | integer | false | Filter records based on the type of the permission |
item_name | text | false | Filter records based on the name of the permission |
user_id | integer | false | Filter records based on the id of the user. |
organization_id | integer | false | Filter records based on the id of the organization |
Create a Permission
POST /admin/organization/<oid>/rbac HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 1516457,
"type": 2,
"user_id": 30876,
"organization_id": 488,
"item_name": "panel.assign",
"created_at": null
}
]
This endpoint checks for the item_name in the auth_item, if present it is assigned either in user or organization level. If the item_name is not present in the auth_item it is first created and then assigned to the user/organization.
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/rbac
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
type | integer | true | The type of the permission |
item_name | text | true | The name of the permission |
user_id | integer | true | The id of the user |
organization_id | integer | true | The id of the organization |
Update an Permission
PUT /admin/organization/<oid>/rbac HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 1516457,
"type": 2,
"user_id": 30876,
"organization_id": 488,
"item_name": "panel.assign",
"created_at": null
}
]
Updates the permissions of user/organization.
HTTP Request
PUT https://apiv4.talview.com/admin/organization/<oid>/rbac
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
item_name | text | true | The name of the permission |
user_id | integer | false | The id of the user. |
organization_id | integer | false | The id of the organization |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
type | integer | false | The type of the permission |
item_name | text | true | The name of the permission |
user_id | integer | false | The id of the user. |
organization_id | integer | false | The id of the organization |
Delete a permission
DELETE /admin/organization/<oid>/rbac HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This endpoint allows you to delete an existing permission for a user/organization.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<oid>/rbac
Select Field Data
This endpoint is used to perform CRUD on select field data.
The Select Field Data Object
{
"id": 8,
"key": "1",
"value": "10",
"parent_value": 22,
"parent_id": null,
"select_field_type_id": 1,
"sort_order": 1,
"updated_by": 41,
"updated_at": "2019-12-16T08:49:23+00:00",
"created_at": "2019-12-16T08:49:23+00:00",
"created_by": 41,
"is_deleted": false
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
key | text | The Key value of the Select Field Data |
value | text | The value of the Select Field Data |
parent_value | text | The parent value of the Select Field Data. Child select field data is associated with parent select field data. If we select the parent value then the child Select Field Data will be triggered |
parent_id | integer | The Id of the parent Select Field Data |
select_field_type_id | integer | The id of the Select Field Type |
sort_order | integer | The sequence order of the Select Field Data |
is_deleted | boolean | if true, the model is deleted |
List all Select Field Data
GET admin/organization/2/select-field-data HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 8,
"key": "1",
"value": "10",
"parent_value": 22,
"parent_id": null,
"select_field_type_id": 1,
"sort_order": 1,
"updated_by": 41,
"updated_at": "2019-12-16T08:49:23+00:00",
"created_at": "2019-12-16T08:49:23+00:00",
"created_by": 41,
"is_deleted": false
}
...
]
This endpoint lists all the existing select field data.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/select-field-data
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the model |
id | integer | false | filters records by the id passed |
ids | array of ids | false | filters records by multiple ids |
key | text | false | filters records by the key passed |
value | text | false | filters records by the value passed(like search) |
parent_value | text | false | filters records by the parent_value passed |
parent_id | integer | false | filters records by the parent_id passed |
select_field_type_id | integer | false | filter records bySelect Field Type id |
is_deleted | boolean | false | if passed true, only deleted models will be retrieved |
value_exact | text | false | filters records by the value passede(exact search) |
Create Select Field Data
POST admin/organization/<oid>/select-field-data HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST request
{
"key": "1",
"value": "10",
"parent_value": 22,
"parent_id": null,
"select_field_type_id": 1,
"sort_order": 1
}
The above request returns JSON structured like this:
{
"id": 8,
"key": "1",
"value": "10",
"parent_value": 22,
"parent_id": null,
"select_field_type_id": 1,
"sort_order": 1,
"updated_by": 41,
"updated_at": "2019-12-16T08:49:23+00:00",
"created_at": "2019-12-16T08:49:23+00:00",
"created_by": 41,
"is_deleted": false
}
This endpoint creates a new select field data.
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/select-field-data
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
select_field_type_id | integer | true | The id of the Select Field Type |
key | text | true | The Key value of the Select Field Data |
value | text | true | The value of the Select Field Data |
parent_value | text | false | The parent value of the Select Field Data |
parent_id | integer | false | The Id of the parent Select Field Data |
data | integer | false | the data of select field data |
sort_order | integer | false | The sort_order is helpful in sorting. When multiples models are retrived, the models are sorted based on the sor order |
Update Select Field Data
PUT admin/organization/2/select-field-data/1 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON PUT request
{
"select_field_type_id":2
}
The above request returns JSON structured like this:
{
"id": 1,
"key": "1",
"value": "2",
"parent_value": null,
"parent_id": null,
"select_field_type_id": 2,
"sort_order": null,
"updated_by": 41,
"updated_at": "2019-12-16T11:07:44+00:00",
"created_at": "2019-12-16 08:34:40+00",
"created_by": 41,
"is_deleted": false
}
This endpoint updates an existing select field data.
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/select-field-data/<id>
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
select_field_type_id | integer | true | The id of the Select Field Type |
key | text | true | The Key value of the Select Field Data |
value | text | true | The value of the Select Field Data |
parent_value | text | false | The parent value of the Select Field Data |
parent_id | integer | false | The Id of the parent Select Field Data |
data | integer | false | the data of select field data |
is_deleted | boolean | false | if passed true then recovers a deleted model |
Delete Select Field Data
DELETE admin/organization/<oid>/select-field-data/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code: 204 No Content
This endpoint deletes an existing select field data.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<oid>/select-field-data/<id>
Settings Data
The endpoint is used to perform CRUD on Settings Data.
The Settings Data Object
{
"id": 68,
"settings_id": 15,
"settings_allowed_value_id": 190,
"value": "AssessmentCandidate",
"unconstrained_value": null,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"is_deleted": false
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
settings_id | integer | the settings id of the settins data |
settings_allowed_value_id | integer | the settings allowed value id of settings data |
value | text | the value of settings data |
unconstrained_value | integer | the unconstrained value of settings data |
is_deleted | boolean | if true, the model is deleted |
List all Settings Data
GET admin/organization/2/settings-data HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 68,
"settings_id": 15,
"settings_allowed_value_id": 190,
"value": "AssessmentCandidate",
"unconstrained_value": null,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"is_deleted": false
}
...
]
This endpoint returns all the existing settings data. By default, the endpoint returns all the non-deleted models.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/settings-data
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
id | integer | false | the id of the user category |
ids | array of ids | false | filters by multiplel ids |
is_deleted | boolean | false | filters by is_deleted, if passed true, deleted models will be returned |
settings_id | integer | false | filters by settings id |
Retrieve an Settings Data
GET admin/organization/2/settings-data/68 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 68,
"settings_id": 15,
"settings_allowed_value_id": 190,
"value": "AssessmentCandidate",
"unconstrained_value": null,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"is_deleted": false
}
This endpoint retrieves a single settings data based on the id passed in the URL
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/settings-data/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
id | integer | true | filters record based on the id passed |
Create an Settings Data
POST admin/organization/2/settings-data HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"settings_id":15,
"settings_allowed_value_id":190
}
The above request returns JSON structured like this:
{
"id": 68,
"settings_id": 15,
"settings_allowed_value_id": 190,
"value": "AssessmentCandidate",
"unconstrained_value": null,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"is_deleted": false
}
This endpoint creates a new settings data.
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/settings-data
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
Body Parameters
Parameter | Type | Mandatory |
---|---|---|
settings_id | integer | true |
settings_allowed_value_id | text | true |
unconstrained_value | json | false |
user_id | integer | false |
Update an Settings Data
PUT admin/organization/2/settings-data/68 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON PUT request
{
"settings_allowed_value_id": 150
}
The above request returns JSON structured like this:
{
"id": 68,
"settings_id": 15,
"settings_allowed_value_id": 150,
"value": "AssessmentCandidate",
"unconstrained_value": null,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"is_deleted": false
}
The endpoint updates the settings data for the id which is passed in the URL.
HTTP Request
PUT https://apiv4.talview.com/admin/organization/<oid>/settings-data/<id>
Body Parameters
Parameter | Type | Mandatory |
---|---|---|
settings_id | integer | false |
settings_allowed_value_id | text | false |
is_deleted | boolean | false |
Url Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
id | integer | true | filters record based on the id passed |
Delete an Settings Data
DELETE admin/organization/2/settings-data/68 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer toke
The above request returns the status code : 204 No Content
This endpoint allows you to delete an existing settings data.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<oid>/settings-data/<id>
User Category
The endpoint is used to perform CRUD on User Category.
The User Category Object
{
"id": 1,
"user_id": "41",
"category_id": 1,
"created_at": "2019-12-16T05:57:07+00:00",
"updated_at": "2019-12-16T05:57:07+00:00",
"is_deleted": false
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
user_id | integer | The user id to whom a category is added |
category_id | integer | The is of the Category. Category id can be found in Category |
is_deleted | boolean | if true, the user category is deleted |
List all User Category
GET admin/organization/2/user/41/category HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 1,
"user_id": "41",
"category_id": 1,
"created_at": "2019-12-16T05:57:07+00:00",
"updated_at": "2019-12-16T05:57:07+00:00",
"is_deleted": false
},
{
"id": 2,
"user_id": "41",
"category_id": 2,
"created_at": "2019-12-16T05:57:07+00:00",
"updated_at": "2019-12-16T05:57:07+00:00",
"is_deleted": false
}
...
]
This endpoint returns all the existing non-deleted user categories.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/user/<user_id>/category
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
id | integer | false | the id of the user category |
ids | array of ids | false | filters by multiplel ids |
user_id | integer | true | filters user category by an user_id |
category_id | integer | false | filters user category by a category id |
s | text | false | filters by text in Category name |
Retrieve an User Category
GET admin/organization/2/user/41/category/1 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1,
"user_id": "41",
"category_id": 1,
"created_at": "2019-12-16T05:57:07+00:00",
"updated_at": "2019-12-16T05:57:07+00:00",
"is_deleted": false
}
This endpoint retrieves a single user category based on the id passed in the URL
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/user/<user_id>/category/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
user_id | integer | true | the user id of the user category |
id | integer | true | filters record based on the id passed |
Create an User Category
POST admin/organization/2/user/41/category HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"category_id":1
}
The above request returns JSON structured like this:
{
"id": 1,
"user_id": "41",
"category_id": 1,
"created_at": "2019-12-16T05:57:07+00:00",
"updated_at": "2019-12-16T05:57:07+00:00",
"is_deleted": false
}
This endpoint creates a new user category..
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/user/<user_id>/category
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
user_id | integer | true | the user id of the user category |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
category_id | integer | false | the category id of the user category |
Update an User Category
PUT admin/organization/2/user/41/category/1 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON PUT request
{
"category_id" : 2
}
The above request returns JSON structured like this:
{
"id": 1,
"user_id": "41",
"category_id": 2,
"created_at": "2019-12-16T05:57:07+00:00",
"updated_at": "2019-12-16T05:57:07+00:00",
"is_deleted": false
}
The endpoint updates the user category for the id which is passed in the URL.
HTTP Request
PUT https://apiv4.talview.com/admin/organization/<oid>/user/<user_id>/category/<id>
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
category_id | integer | false | the category id of the user category |
is_deleted | boolean | false | if passd true then recovers a deleted user category |
Url Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
user_id | integer | true | the user id of the user category |
id | integer | true | filters record based on the id passed |
Delete an User Category
DELETE /admin/organization/2/user/41/category/1 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer toke
The above request returns the status code : 204 No Content
This endpoint allows you to delete an existing user category.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<oid>/user/<user_id>/category/<id>
Ui Filter
The endpoint is used to perform CRUD on Ui Filter.
The Ui Filter Object
{
"id": 154,
"object_type": "a",
"filter": {
"type": "text"
},
"created_at": "2019-12-07T07:47:09+00:00",
"updated_at": "2019-12-07T07:47:09+00:00",
"is_deleted": false,
"organization_id": "2",
"version": "1.1"
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
object_type | integer | the object type of the object type. Allowed objet_types are mentioned below |
organization_id | integer | the organization of the ui_filter |
is_deleted | boolean | if true, the model is deleted |
version | text | the version of the ui filter |
filter | json | the filter object of ui filter |
List of object type:
object type | referes to object |
---|---|
a | assessment |
ac | assessment candidate |
c | candidate |
pc | panel candidate |
r-ls | recruit live sesssion |
p-ls | panel live session |
List all Ui Filters
GET admin/organization/2/filter HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 154,
"object_type": "a",
"filter": {
"type": "text"
},
"created_at": "2019-12-07T07:47:09+00:00",
"updated_at": "2019-12-07T07:47:09+00:00",
"is_deleted": false,
"organization_id": "2",
"version": "1.1"
}
...
]
This endpoint returns all the existing global filters as well as the filter that belongs to the organization passed in the request. By default, the endpoint returns all the non-deleted models.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/filter
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
id | integer | false | the id of the user category |
ids | array of ids | false | filters by multiplel ids |
is_deleted | boolean | false | filters by is_deleted, if passed true, deleted models will be returned |
object_type | integer | false | filters by object type |
version | text | false | filters by the version of the model |
Retrieve an Ui Filter
GET admin/organization/2/filter/154 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 154,
"object_type": "a",
"filter": {
"type": "text"
},
"created_at": "2019-12-07T07:47:09+00:00",
"updated_at": "2019-12-07T07:47:09+00:00",
"is_deleted": false,
"organization_id": "2",
"version": "1.1"
}
This endpoint retrieves a single ui column based on the id passed in the URL
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/filter/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
id | integer | true | filters record based on the id passed |
Create an Ui Filter
POST admin/organization/2/filter HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"object_type": "a",
"filter": {
"type": "text"
},
"version": 1.1
}
The above request returns JSON structured like this:
{
"id": 154,
"object_type": "a",
"filter": {
"type": "text"
},
"created_at": "2019-12-07T07:47:09+00:00",
"updated_at": "2019-12-07T07:47:09+00:00",
"is_deleted": false,
"organization_id": "2",
"version": "1.1"
}
This endpoint creates a new Ui Filter.
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/filter
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
object_type | integer | true | filters user object type |
version | text | false | filters by the version of the model |
filter | json | true | the filter object of ui filter |
Update an Ui Filter
PUT admin/organization/2/filter/154 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON PUT request
{
"version" : "1.2"
}
The above request returns JSON structured like this:
{
"id": 154,
"object_type": "a",
"filter": {
"type": "text"
},
"created_at": "2019-12-07T07:47:09+00:00",
"updated_at": "2019-12-07T07:47:09+00:00",
"is_deleted": false,
"organization_id": "2",
"version": "1.2"
}
The endpoint updates the Ui Filter for the id which is passed in the URL.
HTTP Request
PUT https://apiv4.talview.com/admin/organization/<oid>/filter/<id>
Body Parameters
Parameter | Type | Mandatory |
---|---|---|
object_type | integer | false |
version | text | false |
filter | json | false |
is_deleted | boolean | false |
Url Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
id | integer | true | filters record based on the id passed |
Delete an Ui Filter
DELETE admin/organization/2/filter/154 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer toke
The above request returns the status code : 204 No Content
This endpoint allows you to delete an existing ui filter.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<oid>/filter/<id>
User Permission
The Permission endpoint gives the details of the permissions/access given to a user.
The User Permission Object
{
"id": 136,
"type": 2,
"user_id": 41,
"organization_id": 4,
"item_name": "event.create",
"created_at": 1556236219
},
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
item_name | text | The name of the auth_item |
organization_id | boolean | The id of the organization |
type | integer | The type of permission given |
user_id | integer | The id of the user |
created_at | timestamp | The timestamp when the permission was created |
updated_at | timestamp | The timestamp when the permission was updated |
Id | Type |
---|---|
1 | TYPE_ROLE |
2 | TYPE_PERMISSION |
List all User Permission
GET admin/organization/2/user/41/permission HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The Response
[
{
"created_at": 1556236219,
"id": 435367,
"item_name": "event.create",
"organization_id": 488,
"type": 2,
"updated_at": 1556236219,
"user_id": null
},
...
]
This endpoint returns all the permissions given to an user.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/user/<uid>/permission
Query Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
type | integer | true | Filter records based on the type of the object |
item_name | text | true | Filter records based on the item name |
uid | integer | false | Filter records by an user id |
oid | integer | false | Filters records by organization id |
View an User Permission
GET /admin/organization/2/user/41/permission/435367 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The Response
{
"created_at": 1556236219,
"id": 435367,
"item_name": "event.create",
"organization_id": 2,
"type": 2,
"updated_at": 1556236219,
"user_id": 41
}
This endpoint return an user permission.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<oid>/user/<uid>/permssion/<id>
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The Id of the auth assignment model |
uid | integer | false | The id of the user object |
oid | integer | false | The id of the organization object |
Create an User Permission
POST admin/organization/2/user/41/permission HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"item_name": "event.create",
}
The above request returns JSON structured like this:
{
"created_at": 1556236219,
"id": 435367,
"item_name": "event.create",
"organization_id": 2,
"type": 2,
"updated_at": 1556236219,
"user_id": 41
}
This endpoint creates a new User Permission. Please find the item_name from permission master list.
HTTP Request
POST https://apiv4.talview.com/admin/organization/<oid>/user/<uid>/permission
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
oid | integer | true | the organization id of the user category |
uid | integer | true | the user id of the user category |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
item_name | text | true | the name of the auth_item |
Delete an User Permission
DELETE admin/organization/2/user/41/permission/72 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer toke
The above request returns the status code : 204 No Content
This endpoint allows you to delete an existing user permission.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<oid>/user/<uid>/permission/<id>
Models
Account Manager Organization(AMO)
Account Manager Organizations(AMO) are the special users who will have access to their designated organizations in the admin application. AMO is an user who maintains an organization. when an organization is created the user who created the organization will be considered as the Account Manager of the organization. Later the Account Manager can add the other users as account managers. An Account Manager can create, delete and update other Account Managers, but not allowed to delete himself/herself.
The AMO Object
{
"id": 712,
"is_deleted": false,
"role": "Account Manager",
"user_id": 307856,
"organization_id": 733,
"category_id": null,
"added_by": 1678569,
"added_at": "2019-10-02 17:03:11+05:30",
"deleted_by": null,
"deleted_at": null,
"created_at": "2019-10-02 17:03:10+05:30",
"updated_at": "2019-10-02 17:03:10+05:30",
"updated_by": 1678569
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
is_deleted | boolean | true for deleted AMO and false for the AMOs which are not deleted |
role | text | The role of the AMO. Example: Admin, Superadmin, Account Manager |
user_id | integer | The user id of the AMO |
organization_id | integer | The organization id of the AMO |
category_id | integer | The category id of the AMO |
added_by | integer | The user id who added this AMO |
added_at | timestamptz | The time when the AMO is added |
deleted_by | integer | The user id who deleted the AMO |
deleted_at | timestamptz | The time when the AMO was deleted |
created_at | timestamptz | The time when the AMO was created |
updated_at | timestamptz | The time when the AMO was updated |
updated_by | integer | The user id who update the AMO recently |
List all AMOs
GET /admin/organization/<organization_id>/amo HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 712,
"is_deleted": false,
"role": "Account Manager",
"user_id": 307856,
"organization_id": 733,
"category_id": null,
"added_by": 1678569,
"added_at": "2019-10-02 17:03:11+05:30",
"deleted_by": null,
"deleted_at": null,
"created_at": "2019-10-02 17:03:10+05:30",
"updated_at": "2019-10-02 17:03:10+05:30",
"updated_by": 1678569
},
{
"id": 711,
"is_deleted": true,
"role": "Account Manager",
"user_id": 167859,
"organization_id": 733,
"category_id": null,
"added_by": 16XXX69,
"added_at": null,
"deleted_by": null,
"deleted_at": null,
"created_at": "2019-10-02 17:01:40+05:30",
"updated_at": "2019-10-04 19:45:55+05:30",
"updated_by": 1678569
}
]
This end point returns all the details of AMOs
HTTP Request
GET https://apiv4.talview.com/admin/organization/<organization_id>/amo
Retrieve an AMO
GET /admin/organization/<organization_id>/amo/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 711,
"is_deleted": true,
"role": "Account Manager",
"user_id": 167859,
"organization_id": 733,
"category_id": null,
"added_by": 1678569,
"added_at": null,
"deleted_by": null,
"deleted_at": null,
"created_at": "2019-10-02 17:01:40+05:30",
"updated_at": "2019-10-04 19:45:55+05:30",
"updated_by": 1678569
}
Retrieves the details of an existing AMO.
HTTP Request
GET https://apiv4.talview.com/admin/organization/<organization_id>/amo/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create an AMO
POST /admin/organization/<organization_id>/amo HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"user_id":477859,
"category_id":1
}
The above request returns JSON structured like this:
{
"id": 711,
"is_deleted": true,
"role": "Account Manager",
"user_id": 477859,
"organization_id": 488,
"category_id": 1,
"added_by": 167869,
"added_at": null,
"deleted_by": null,
"deleted_at": null,
"created_at": "2019-10-02 17:01:40+05:30",
"updated_at": "2019-10-04 19:45:55+05:30",
"updated_by": 167869
}
This end point Creates a new AMO.
HTTP Request
POST https://apiv4.talview.com/admin/organization/<organization_id>/amo
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
user_id | text | true | The user id who will be added as an AMO to an organization |
category_id | text | false | The category id of the AMO |
Update an AMO
This endpoint Updates an AMO.
PUT /admin/organization/<organization_id>/amo/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"category_id":1
}
The above request returns JSON structured like this:
{
"id": 711,
"is_deleted": true,
"role": "Account Manager",
"user_id": 477859,
"organization_id": 488,
"category_id": 1,
"added_by": 167869,
"added_at": null,
"deleted_by": null,
"deleted_at": null,
"created_at": "2019-10-02 17:01:40+05:30",
"updated_at": "2019-10-04 19:45:55+05:30",
"updated_by": 167869
}
HTTP Request
PUT https://apiv4.talview.com/admin/organization/<organization_id>/amo/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Delete an AMO
DELETE /admin/organization/<organization_id>/amo/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This end point Deletes an AMO.
HTTP Request
DELETE https://apiv4.talview.com/admin/organization/<organization_id>/amo/<id>
Spreadsheet
The Spreadsheet endpoint is used for uploading a csv to get the rows as objects.
The Spreadsheet Object
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
file | file | The file to be uploaded |
file_type_id | integer | THe type of the file |
Bulk import
POST /spreadsheet HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
HTTP Request
POST https://apiv4.talview.com/spreadsheet
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
file | file | true | The file |
file_type_id | integer | false | The type of the file |
Answer
The Answer endpoint provides all the answers submitted by each candidate for the section of the assessment(Assessment Section) that the candidate was invited to.
The Answer Object
{
"id": 8,
"status": 6,
"question_id": 141,
"answered_at": "2012-06-06 16:14:46+05:30",
"choice": null,
"text": null,
"typingSpeed": 0,
"plainTxtContent": "(not set)",
"score": null,
"typingAccuracy": "",
"typingCompletion": "",
"assessment_section_id": 6574,
"assessment_candidate_id": 4,
"started_at": "2015-05-05 15:25:21+05:30",
"created_at": "2016-04-19 11:39:01+05:30",
"updated_at": "2017-08-29 07:52:39+05:30",
"assessment_section_candidate_id": 12605,
"_links": {
"question": {
"href": "/models/question?id=141"
},
"thumbnails": {
"href": "/models/file?answer_id=8&file_type_id=15"
},
"videos": {
"href": "/models/file?answer_id=8&file_type_id%5B0%5D=4&file_type_id%5B1%5D=5"
},
"attachments": {
"href": "/models/file?answer_id=8&file_type_id%5B0%5D=25"
},
"vmRecordings": {
"href": "/models/file?answer_id=8&file_type_id%5B0%5D=37"
},
"vmAttachment": {
"href": "/models/file?answer_id=8&file_type_id%5B0%5D=38"
},
"answerCodeSubmissions": {
"href": "/models/answer-code-submission?answer_id=8&type=2"
}
}
},
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
status | smallint | The status of the Answer. Example: Created, Completed. The answer status are described in the enumerator list below. |
question_id | integer | The id of the Question |
answered_at | timestamptz | The time when the question was answered |
choice | integer | Selected option for MCQ and MCA in the form of integer. Example: 3(indicate choice 3). In case of MCA if more then one choices are checked then the choices are concatenated and sent. Example: If 1 and 3 are selected, it is taken as 13. |
typingSpeed | integer | The number of words typed per minute |
plainTxtContent | text | The Answer in the plain text format |
score | integer | The score obtained by the candidate in MCQ and MCA type question |
typingAccuracy | integer | The rate of typing accuracy |
typingCompletion | integer | The typing speed rate |
assessment_section_id | integer | The id of the Assessment Section |
assessment_candidate_id | integer | The id of Assessment Candidate |
assessment_section_candidate_id | integer | The id of Assessment Section Candidate |
List of Answer Status:
Status | Key | Description |
---|---|---|
Created | 1000 | The answer is created for assessment |
Completed | 3000 | The candidate had selected an answer |
List all Answer
Returns all answers selected by the candidates for each section of the assessment.
GET /models/answer HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 10,
"status": 6,
"question_id": 143,
"answered_at": "2012-06-06 16:16:01+05:30",
"choice": null,
"text": null,
"typingSpeed": 0,
"plainTxtContent": "(not set)",
"score": null,
"typingAccuracy": "",
"typingCompletion": "",
"assessment_section_id": 6574,
"assessment_candidate_id": 4,
"started_at": "2015-05-05 15:25:21+05:30",
"created_at": "2016-04-19 11:39:01+05:30",
"updated_at": "2017-08-29 07:52:40+05:30",
"assessment_section_candidate_id": 12605,
"_links": {
"question": {
"href": "/models/question?id=143"
},
"thumbnails": {
"href": "/models/file?answer_id=10&file_type_id=15"
},
"videos": {
"href": "/models/file?answer_id=10&file_type_id%5B0%5D=4&file_type_id%5B1%5D=5"
},
"attachments": {
"href": "/models/file?answer_id=10&file_type_id%5B0%5D=25"
},
"vmRecordings": {
"href": "/models/file?answer_id=10&file_type_id%5B0%5D=37"
},
"vmAttachment": {
"href": "/models/file?answer_id=10&file_type_id%5B0%5D=38"
},
"answerCodeSubmissions": {
"href": "/models/answer-code-submission?answer_id=10&type=2"
}
}
},
...
]
HTTP Request
GET https://apiv4.talview.com/models/answer
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
question_id | integer | false | Filter record based on the Id of the Question |
assessment_candidate_id | integer | false | Filter record based on the Id of Assessment Candidate |
assessment_section_candidate_id | integer | false | Filter record based on the Id of Assessment Section Candidate |
assessment_section_id | integer | false | Filter record based on the Id of Assessment Section |
Retrieve an Answer
Retrieve an answer based on the id mentioned in the URL.
GET /models/answer/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 10,
"status": 6,
"question_id": 143,
"answered_at": "2012-06-06 16:16:01+05:30",
"choice": null,
"text": null,
"typingSpeed": 0,
"plainTxtContent": "(not set)",
"score": null,
"typingAccuracy": "",
"typingCompletion": "",
"assessment_section_id": 6574,
"assessment_candidate_id": 4,
"started_at": "2015-05-05 15:25:21+05:30",
"created_at": "2016-04-19 11:39:01+05:30",
"updated_at": "2017-08-29 07:52:40+05:30",
"assessment_section_candidate_id": 12605,
"_links": {
"question": {
"href": "/models/question?id=143"
},
"thumbnails": {
"href": "/models/file?answer_id=10&file_type_id=15"
},
"videos": {
"href": "/models/file?answer_id=10&file_type_id%5B0%5D=4&file_type_id%5B1%5D=5"
},
"attachments": {
"href": "/models/file?answer_id=10&file_type_id%5B0%5D=25"
},
"vmRecordings": {
"href": "/models/file?answer_id=10&file_type_id%5B0%5D=37"
},
"vmAttachment": {
"href": "/models/file?answer_id=10&file_type_id%5B0%5D=38"
},
"answerCodeSubmissions": {
"href": "/models/answer-code-submission?answer_id=10&type=2"
}
}
}
HTTP Request
GET https://apiv4.talview.com/models/answer/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Answer Code Submission
The Answer Code Submission endpoint gives the details of all the Answer Code submitted by the Candidate against Coding type Assessment.
The Answer Code Submission Object
{
"id": 897,
"answer_id": 4441883,
"source": "/*\n#include <iostream>\nusing namespace std;\nint main()\n{\n cout << \"Hello World!\" << endl;\n return 0;\n}\n*/\n//Write your code here",
"score": null,
"status": 0,
"input": null,
"memory": 0,
"language": "1",
"language_id": "1",
"compiler": null,
"cmpinfo": "/usr/lib/gcc/x86_64-linux-gnu/6/../../../x86_64-linux-gnu/Scrt1.o: In function `_start':\n(.text+0x20): undefined reference to `main'\ncollect2: error: ld returned 1 exit status\n",
"stderr": null,
"time": 0,
"output": null,
"result": "/usr/lib/gcc/x86_64-linux-gnu/6/../../../x86_64-linux-gnu/Scrt1.o: In function `_start':\n(.text+0x20): undefined reference to `main'\ncollect2: error: ld returned 1 exit status\n"
},
{
"id": 699,
"answer_id": 2608879,
"source": "/* IMPORTANT: Multiple classes and nested static classes are supported */\n\n/*\n* uncomment this if you want to read input.\nimport java.io.BufferedReader;\nimport java.io.InputStreamReader;\n*/\nclass TestClass {\n public static void main(String args[] ) throws Exception {\n /*\n * Read input from stdin and provide input before running\n BufferedReader br = new BufferedReader(new InputStreamReader(System.in));\n String line = br.readLine();\n int N = Integer.parseInt(line);\n for (int i = 0; i < N; i++) {\n System.out.println(\"hello world\");\n }\n */\n System.out.println(\"Hello World!\");\n }\n}\n//Write your code here",
"score": null,
"status": 0,
"input": null,
"memory": 2841600,
"language": "10",
"language_id": "10",
"compiler": null,
"cmpinfo": null,
"stderr": null,
"time": 0,
"output": "Hello World!\n",
"result": null
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
answer_id | integer | The id of the Answer |
source | text | The score obtained in the Answer Code Submission |
score | integer | The score code submitted by the Candidate |
status | integer | The status of the answer code submitted for compilation. Example: Waiting, execution etc. The status are listed in the table below |
input | text | The test cases of code assessment |
memory | integer | The average memory consumed by the code |
language | text | The language used for coding |
compiler | text | The compiler used for compiling |
cmpinfo | text | The information of the compiler |
stderr | text | The standard error of the submitted code |
time | timestamptz | The time required for the compilation of the code |
output | text | The output form the code |
result | text | The result of the code assessment |
Status of Compilation:
Status | Key | Description |
---|---|---|
waiting | 0 | Submission is waiting in the queue |
compilation | 1,2 | Program is being compiled (for compiled languages) |
execution | 3 | Program is being executed |
compilation error | 11 | The program could not be executed due to a compilation error |
runtime error | 12 | An error occurred while the program was running (e.g. division by zero) |
time limit exceeded | 13 | The program exceeded the time limit |
success | 15 | The program was executed correctly |
memory limit exceeded | 17 | The program exceeded the memory limit |
illegal system call | 19 | The program tried to call illegal system function |
internal error | 20 | An unexpected error occurred on the Sphere Engine side try making the submission again and if this occurs again, please contact us |
List all Answer Code Submission
Returns all the answer code submission submitted by the candidates for the code section in an assessment.
GET /models/answer-codesubmission HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 897,
"answer_id": 4441883,
"source": "/*\n#include <iostream>\nusing namespace std;\nint main()\n{\n cout << \"Hello World!\" << endl;\n return 0;\n}\n*/\n//Write your code here",
"score": null,
"status": 0,
"input": null,
"memory": 0,
"language": "1",
"language_id": "1",
"compiler": null,
"cmpinfo": "/usr/lib/gcc/x86_64-linux-gnu/6/../../../x86_64-linux-gnu/Scrt1.o: In function `_start':\n(.text+0x20): undefined reference to `main'\ncollect2: error: ld returned 1 exit status\n",
"stderr": null,
"time": 0,
"output": null,
"result": "/usr/lib/gcc/x86_64-linux-gnu/6/../../../x86_64-linux-gnu/Scrt1.o: In function `_start':\n(.text+0x20): undefined reference to `main'\ncollect2: error: ld returned 1 exit status\n"
}
]
HTTP Request
GET https://apiv4.talview.com/models/answer-code-submission
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
answer_id | integer | false | Filter record based on the Id of the Answer |
source | text | false | Filter record based on the source code of the coding assessment |
input | text | false | Filter record based on the test cases of code assessment |
language | text | false | Filter record based on the language used for coding |
output | text | false | Filter record based on the output form the code |
Retrieve an Answer Code Submission
Retrieve an answer code submitted by the candidates for the code section in an assessment based on the id mentioned in the URL.
GET /models/answer-codesubmission/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 897,
"answer_id": 4441883,
"source": "/*\n#include <iostream>\nusing namespace std;\nint main()\n{\n cout << \"Hello World!\" << endl;\n return 0;\n}\n*/\n//Write your code here",
"score": null,
"status": 0,
"input": null,
"memory": 0,
"language": "1",
"language_id": "1",
"compiler": null,
"cmpinfo": "/usr/lib/gcc/x86_64-linux-gnu/6/../../../x86_64-linux-gnu/Scrt1.o: In function `_start':\n(.text+0x20): undefined reference to `main'\ncollect2: error: ld returned 1 exit status\n",
"stderr": null,
"time": 0,
"output": null,
"result": "/usr/lib/gcc/x86_64-linux-gnu/6/../../../x86_64-linux-gnu/Scrt1.o: In function `_start':\n(.text+0x20): undefined reference to `main'\ncollect2: error: ld returned 1 exit status\n"
}
HTTP Request
GET https://apiv4.talview.com/models/answer-code-submission/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filters records based on Id |
Assessment
The Assessment endpoint is used to view, update and delete an assessment created through Create Assessment action. Each Assessment must have at least one Sections associated with it and each Assessment can have one or more candidates invited to it.
The Assessment Object
{
"id": 861338,
"external_id": null,
"title": "qwertyui",
"status_id": null,
"description": "<span class=\"not-set\">(not set)</span>",
"organization_id": 488,
"position_id": null,
"cf": [
null
],
"first_assessment_section_id": null,
"formUrl": "https://abc/pqr/xys/index.html",
"reportUrl": "https://abc/pqr/xys/861338.xls",
"hasLive": false,
"created_at": "2019-03-13 14:54:35+00",
"updated_at": "2019-03-13 14:54:35+00",
"customFields": [],
"start_date": null,
"end_date": null,
"owned_by": 30876,
"_links": {
"metrics": {
"href": "/reports/assessment-time/861338"
},
"notificationLog": {
"href": "/models/notification-log?assessment_id=861338"
},
"templates": {
"href": "/models/notification-template?assessment_id=861338"
},
"menuCardAssessments": {
"href": "/models/menu-card-assessment?assessment_id=861338"
},
"all": {
"href": "/models/assessment-candidate?assessment_id=861338"
},
"categories": {
"href": "/models/assessment-category?assessment_id=861338"
},
"assessmentSummary": {
"href": "/assessment/861338"
},
"publicSection": {
"href": "/models/assessment-section?assessment_id=861338§ion_type_id%5B0%5D=21§ion_type_id%5B1%5D=19§ion_type_id%5B2%5D=20§ion_type_id%5B3%5D=4§ion_type_id%5B4%5D=5§ion_type_id%5B5%5D=6§ion_type_id%5B6%5D=10§ion_type_id%5B7%5D=17"
},
"assessmentSections": {
"href": "/models/assessment-section?assessment_id=861338§ion_type_id%5B0%5D=21§ion_type_id%5B1%5D=19§ion_type_id%5B2%5D=20§ion_type_id%5B3%5D=4§ion_type_id%5B4%5D=5§ion_type_id%5B5%5D=6§ion_type_id%5B6%5D=15§ion_type_id%5B7%5D=7§ion_type_id%5B8%5D=16§ion_type_id%5B9%5D=10§ion_type_id%5B10%5D=13§ion_type_id%5B11%5D=14§ion_type_id%5B12%5D=18§ion_type_id%5B13%5D=9§ion_type_id%5B14%5D=17§ion_type_id%5B15%5D=22"
},
"evaluateSections": {
"href": "/models/assessment-section?assessment_id=861338§ion_type_id%5B0%5D=4§ion_type_id%5B1%5D=5§ion_type_id%5B2%5D=10§ion_type_id%5B3%5D=13§ion_type_id%5B4%5D=15§ion_type_id%5B5%5D=14§ion_type_id%5B6%5D=18§ion_type_id%5B7%5D=20§ion_type_id%5B8%5D=19§ion_type_id%5B9%5D=17§ion_type_id%5B10%5D=21§ion_type_id%5B11%5D=22§ion_type_id%5B12%5D=7"
},
"allSections": {
"href": "/models/assessment-section?assessment_id=861338"
},
"added": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=10"
},
"invited": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=20"
},
"attended": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=50"
},
"rejected": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=90"
},
"evaluated": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=60"
},
"selected": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=70"
},
"hold": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=80"
},
"inProgress": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=30"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of an object |
external_id | string | The reference Id for an object |
title | string | The title of an Assessment |
status_id | integer | The Status Id of the Assessment. Example: Draft, Close. The available Status for Assessment are mentioned in the enumerator list below |
description | string | The description of the Assessment |
organization_id | integer | The Id of the organization to which an Assessment belongs to |
position_id | integer | The Id of the position for which an Assessment is created |
cf | Object | The Custom Field object |
first_assessment_section_id | integer | The Id of the first Assessment Section |
addedCount | integer | The count of the Candidate added for an Assessment |
invitedCount | integer | The count of Candidates invited for an Assessment |
hasLargeForm | boolean | If true, The Assessment has a form for a large screen |
inProgressCount | integer | The count of Candidate whose Assessment is in progress |
attendedCount | integer | The count of Candidates who have attended and completed an assessment |
assessedCount | integer | The count of Candidates whose Assessment has been evaluated |
selectedCount | integer | The count of Candidates who have been selected for the position for which an Assessment was created |
hasLive | boolean | Flag to check whether an Assessment has a live session |
holdCount | integer | The count of candidates who are neither rejected nor selected in an assessment and are kept in hold |
rejectedCount | integer | The count of candidates who have been rejected after an Assessment |
customFields | Array of Object | The Details of all the available Custom Fields |
start_date | integer | The date and time from when the Assessment is active and can be attended |
end_date | integer | The last date and time to attend an Assessment |
owned_by | integer | The Id of the user who own the assessment. The user here is the Recruiter who created the Assessment |
List of Assessment Status:
Status | Key | Description |
---|---|---|
Draft | 1 | When no candidate is assigned to an assessment |
Closed | 2 | The assessment is closed |
Open | 3 | The assessment is open for candidate |
Filled | 4 | The requirement of positions is filled |
List all Assessment
This endpoint returns all existing Assessments.
GET /models/assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 861338,
"external_id": null,
"title": "qwertyui",
"status_id": null,
"description": "<span class=\"not-set\">(not set)</span>",
"organization_id": 488,
"position_id": null,
"cf": [
null
],
"first_assessment_section_id": null,
"formUrl": "https://forms.talview.com/candidate/default/index.html",
"reportUrl": "https://apiv4.talview.com/reports/excel/assessment/861338.xls",
"hasLive": false,
"created_at": "2019-03-13 14:54:35+00",
"updated_at": "2019-03-13 14:54:35+00",
"customFields": [],
"start_date": null,
"end_date": null,
"owned_by": 30876,
"_links": {
"metrics": {
"href": "/reports/assessment-time/861338"
},
"notificationLog": {
"href": "/models/notification-log?assessment_id=861338"
},
"templates": {
"href": "/models/notification-template?assessment_id=861338"
},
"menuCardAssessments": {
"href": "/models/menu-card-assessment?assessment_id=861338"
},
"all": {
"href": "/models/assessment-candidate?assessment_id=861338"
},
"categories": {
"href": "/models/assessment-category?assessment_id=861338"
},
"assessmentSummary": {
"href": "/assessment/861338"
},
"publicSection": {
"href": "/models/assessment-section?assessment_id=861338§ion_type_id%5B0%5D=21§ion_type_id%5B1%5D=19§ion_type_id%5B2%5D=20§ion_type_id%5B3%5D=4§ion_type_id%5B4%5D=5§ion_type_id%5B5%5D=6§ion_type_id%5B6%5D=10§ion_type_id%5B7%5D=17"
},
"assessmentSections": {
"href": "/models/assessment-section?assessment_id=861338§ion_type_id%5B0%5D=21§ion_type_id%5B1%5D=19§ion_type_id%5B2%5D=20§ion_type_id%5B3%5D=4§ion_type_id%5B4%5D=5§ion_type_id%5B5%5D=6§ion_type_id%5B6%5D=15§ion_type_id%5B7%5D=7§ion_type_id%5B8%5D=16§ion_type_id%5B9%5D=10§ion_type_id%5B10%5D=13§ion_type_id%5B11%5D=14§ion_type_id%5B12%5D=18§ion_type_id%5B13%5D=9§ion_type_id%5B14%5D=17§ion_type_id%5B15%5D=22"
},
"evaluateSections": {
"href": "/models/assessment-section?assessment_id=861338§ion_type_id%5B0%5D=4§ion_type_id%5B1%5D=5§ion_type_id%5B2%5D=10§ion_type_id%5B3%5D=13§ion_type_id%5B4%5D=15§ion_type_id%5B5%5D=14§ion_type_id%5B6%5D=18§ion_type_id%5B7%5D=20§ion_type_id%5B8%5D=19§ion_type_id%5B9%5D=17§ion_type_id%5B10%5D=21§ion_type_id%5B11%5D=22§ion_type_id%5B12%5D=7"
},
"allSections": {
"href": "/models/assessment-section?assessment_id=861338"
},
"added": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=10"
},
"invited": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=20"
},
"attended": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=50"
},
"rejected": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=90"
},
"evaluated": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=60"
},
"selected": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=70"
},
"hold": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=80"
},
"inProgress": {
"href": "/models/assessment-candidate?assessment_id=861338&status_sort_key=30"
}
}
},
...
]
HTTP Request
GET https://apiv4.talview.com/models/assessment
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
external_id | string | false | Filter records based on External Id |
title | string | true | Filter records based on title of an Assessment |
start_date | integer | false | Filter records based on start date of an Assessment |
end_date | integer | false | Filter records based on end date of an Assessment |
cf | Object | false | The Custom Field for an Assessment |
status | Object | false | The status of the form |
owned_by | integer | false | The Id of the owner who created an Assessment |
Retrieve an Assessment
Retrieve an assessment based on the assessment id mentioned in the URL.
GET /models/assessment/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 860765,
"external_id": "1322019",
"title": "MCQ + Interview + Essay ",
"status_id": null,
"description": "<span class=\"not-set\">(not set)</span>",
"organization_id": 488,
"position_id": null,
"cf": [
null
],
"first_assessment_section_id": 6922566,
"formUrl": "https://forms.talview.com/candidate/default/index.html",
"reportUrl": "https://apiv4.talview.com/reports/excel/assessment/860765.xls",
"hasLive": false,
"created_at": "2019-03-13 04:40:05+00",
"updated_at": "2019-03-13 11:07:19+00",
"customFields": [],
"start_date": "2019-03-13 11:08:15+00",
"end_date": "2019-03-23 11:07:15+00",
"owned_by": 30876,
"_links": {
"metrics": {
"href": "/reports/assessment-time/860765"
},
"notificationLog": {
"href": "/models/notification-log?assessment_id=860765"
},
"templates": {
"href": "/models/notification-template?assessment_id=860765"
},
"menuCardAssessments": {
"href": "/models/menu-card-assessment?assessment_id=860765"
"all" {
"href": "/models/assessment-candidate?assessment_id=860765"
},
"categories": {
"href": "/models/assessment-category?assessment_id=860765"
},
"assessmentSummary": {
"href": "/assessment/860765"
},
"publicSection": {
"href": "/models/assessment-section?assessment_id=860765§ion_type_id%5B0%5D=21§ion_type_id%5B1%5D=19§ion_type_id%5B2%5D=20§ion_type_id%5B3%5D=4§ion_type_id%5B4%5D=5§ion_type_id%5B5%5D=6§ion_type_id%5B6%5D=10§ion_type_id%5B7%5D=17"
},
"assessmentSections": {
"href": "/models/assessment-section?assessment_id=860765§ion_type_id%5B0%5D=21§ion_type_id%5B1%5D=19§ion_type_id%5B2%5D=20§ion_type_id%5B3%5D=4§ion_type_id%5B4%5D=5§ion_type_id%5B5%5D=6§ion_type_id%5B6%5D=15§ion_type_id%5B7%5D=7§ion_type_id%5B8%5D=16§ion_type_id%5B9%5D=10§ion_type_id%5B10%5D=13§ion_type_id%5B11%5D=14§ion_type_id%5B12%5D=18§ion_type_id%5B13%5D=9§ion_type_id%5B14%5D=17§ion_type_id%5B15%5D=22"
},
"evaluateSections": {
"href": "/models/assessment-section?assessment_id=860765§ion_type_id%5B0%5D=4§ion_type_id%5B1%5D=5§ion_type_id%5B2%5D=10§ion_type_id%5B3%5D=13§ion_type_id%5B4%5D=15§ion_type_id%5B5%5D=14§ion_type_id%5B6%5D=18§ion_type_id%5B7%5D=20§ion_type_id%5B8%5D=19§ion_type_id%5B9%5D=17§ion_type_id%5B10%5D=21§ion_type_id%5B11%5D=22§ion_type_id%5B12%5D=7"
},
"allSections": {
"href": "/models/assessment-section?assessment_id=860765"
},
"added": {
"href": "/models/assessment-candidate?assessment_id=860765&status_sort_key=10"
},
"invited": {
"href": "/models/assessment-candidate?assessment_id=860765&status_sort_key=20"
},
"attended": {
"href": "/models/assessment-candidate?assessment_id=860765&status_sort_key=50"
},
"rejected": {
"href": "/models/assessment-candidate?assessment_id=860765&status_sort_key=90"
},
"evaluated": {
"href": "/models/assessment-candidate?assessment_id=860765&status_sort_key=60"
},
"selected": {
"href": "/models/assessment-candidate?assessment_id=860765&status_sort_key=70"
},
"hold": {
"href": "/models/assessment-candidate?assessment_id=860765&status_sort_key=80"
},
"inProgress": {
"href": "/models/assessment-candidate?assessment_id=860765&status_sort_key=30"
}
}
}
HTTP Request
GET https://apiv4.talview.com/models/assessment/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create an Assessment
This end point is used to create a new Assessment.
POST /models/assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"title": "Pearson Testing",
"status_id": null,
"description": "<p>sdfgsdfg</p>",
"start_date": "2019-04-12 06:53:00+00",
"end_date": "2019-04-15 06:53:00+00",
"owned_by": 30876
}
The above request returns JSON structured like this:
{
"id": 887754,
"external_id": null,
"title": "Pearson Testing",
"status_id": null,
"description": "<p>sdfgsdfg</p>",
"organization_id": 488,
"position_id": null,
"cf": [
null
],
"publicLinkUrl": null,
"first_assessment_section_id": null,
"formUrl": "https://forms.talview.com/candidate/default/index.html",
"reportUrl": "https://apiv4.talview.com/reports/excel/assessment/887754.xls",
"hasLive": false,
"created_at": "2019-04-11T09:04:25+00:00",
"updated_at": "2019-04-11T09:04:25+00:00",
"customFields": [],
"start_date": "2019-04-12 06:53:00+00",
"end_date": "2019-04-15 06:53:00+00",
"owned_by": 30876,
"_links": {
"metrics": {
"href": "/reports/assessment-time/887754"
},
"notificationLog": {
"href": "/models/notification-log?assessment_id=887754"
},
"templates": {
"href": "/models/notification-template?assessment_id=887754"
},
"menuCardAssessments": {
"href": "/models/menu-card-assessment?assessment_id=887754"
},
"all": {
"href": "/models/assessment-candidate?assessment_id=887754"
},
"categories": {
"href": "/models/assessment-category?assessment_id=887754"
},
"assessmentSummary": {
"href": "/assessment/887754"
},
"publicSection": {
"href": "/models/assessment-section?assessment_id=887754§ion_type_id%5B0%5D=21§ion_type_id%5B1%5D=19§ion_type_id%5B2%5D=15§ion_type_id%5B3%5D=20§ion_type_id%5B4%5D=4§ion_type_id%5B5%5D=5§ion_type_id%5B6%5D=6§ion_type_id%5B7%5D=10§ion_type_id%5B8%5D=17"
},
"assessmentSections": {
"href": "/models/assessment-section?assessment_id=887754§ion_type_id%5B0%5D=21§ion_type_id%5B1%5D=19§ion_type_id%5B2%5D=20§ion_type_id%5B3%5D=4§ion_type_id%5B4%5D=5§ion_type_id%5B5%5D=6§ion_type_id%5B6%5D=15§ion_type_id%5B7%5D=7§ion_type_id%5B8%5D=16§ion_type_id%5B9%5D=10§ion_type_id%5B10%5D=13§ion_type_id%5B11%5D=14§ion_type_id%5B12%5D=18§ion_type_id%5B13%5D=9§ion_type_id%5B14%5D=17§ion_type_id%5B15%5D=22"
},
"evaluateSections": {
"href": "/models/assessment-section?assessment_id=887754§ion_type_id%5B0%5D=4§ion_type_id%5B1%5D=5§ion_type_id%5B2%5D=10§ion_type_id%5B3%5D=13§ion_type_id%5B4%5D=15§ion_type_id%5B5%5D=14§ion_type_id%5B6%5D=18§ion_type_id%5B7%5D=20§ion_type_id%5B8%5D=19§ion_type_id%5B9%5D=17§ion_type_id%5B10%5D=21§ion_type_id%5B11%5D=22§ion_type_id%5B12%5D=7"
},
"allSections": {
"href": "/models/assessment-section?assessment_id=887754"
},
"added": {
"href": "/models/assessment-candidate?assessment_id=887754&status_sort_key=10"
},
"invited": {
"href": "/models/assessment-candidate?assessment_id=887754&status_sort_key=20"
},
"attended": {
"href": "/models/assessment-candidate?assessment_id=887754&status_sort_key=50"
},
"rejected": {
"href": "/models/assessment-candidate?assessment_id=887754&status_sort_key=90"
},
"evaluated": {
"href": "/models/assessment-candidate?assessment_id=887754&status_sort_key=60"
},
"selected": {
"href": "/models/assessment-candidate?assessment_id=887754&status_sort_key=70"
},
"hold": {
"href": "/models/assessment-candidate?assessment_id=887754&status_sort_key=80"
},
"inProgress": {
"href": "/models/assessment-candidate?assessment_id=887754&status_sort_key=30"
}
}
}
HTTP Request
POST https://apiv4.talview.com/models/assessment
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
title | string | true | The title of the Assessment |
description | string | true | The description of the Assessment |
start_date | integer | false | The date from when the Assessment is active and can be attended |
end_date | integer | false | The last date and time to attend the Assessment |
owned_by | integer | false | Filter records based on the Id of the owner who created an Assessment |
status | text | false | The status of the Assessment |
Update an Assessment
Updates the details of an assessment based on the assessment id mentioned in the URL.
PUT /models/assessment/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON PUT Request:
{
"title": "Pearson Testing",
"status_id": null,
"description": "<p>sdfgsdfg</p>",
"start_date": "2019-04-12 06:53:00+00",
"end_date": "2019-04-16 06:53:00+00",
"owned_by": 30876
}
The above request returns JSON structured like this:
{
"id": 734419,
"external_id": "56",
"title": "Duplicate question testing ",
"status_id": null,
"description": "<span class=\"not-set\">(not set)</span>",
"organization_id": 2,
"position_id": null,
"cf": [
null
],
"first_assessment_section_id": null,
"addedCount": null,
"invitedCount": 0,
"hasLargeForm": false,
"inProgressCount": 0,
"attendedCount": 0,
"assessedCount": 0,
"selectedCount": 0,
"formUrl": "https://forms.talview.com/candidate/default/index.html",
"reportUrl": "/reports/excel/assessment/734419.xls",
"hasLive": false,
"holdCount": 0,
"rejectedCount": 0,
"created_at": "2019-02-13 13:58:24+05:30",
"updated_at": "2019-02-19T09:39:09+00:00",
"customFields": [],
"start_date": null,
"end_date": null,
"owned_by": 270471,
"_links": {
"metrics": {
"href": "/reports/assessment-time/734419"
},
"notificationLog": {
"href": "/models/notification-log?assessment_id=734419"
},
"templates": {
"href": "/models/notification-template?assessment_id=734419"
},
"menuCardAssessments": {
"href": "/models/menu-card-assessment?assessment_id=734419"
},
"all": {
"href": "/models/assessment-candidate?assessment_id=734419"
},
"assessmentSummary": {
"href": "/assessment/734419"
},
"publicSection": {
"href": "/models/assessment-section?assessment_id=734419§ion_type_id%5B0%5D=21§ion_type_id%5B1%5D=19§ion_type_id%5B2%5D=20§ion_type_id%5B3%5D=4§ion_type_id%5B4%5D=5§ion_type_id%5B5%5D=6§ion_type_id%5B6%5D=10§ion_type_id%5B7%5D=17"
},
"assessmentSections": {
"href": "/models/assessment-section?assessment_id=734419§ion_type_id%5B0%5D=21§ion_type_id%5B1%5D=19§ion_type_id%5B2%5D=20§ion_type_id%5B3%5D=4§ion_type_id%5B4%5D=5§ion_type_id%5B5%5D=6§ion_type_id%5B6%5D=15§ion_type_id%5B7%5D=7§ion_type_id%5B8%5D=16§ion_type_id%5B9%5D=10§ion_type_id%5B10%5D=13§ion_type_id%5B11%5D=14§ion_type_id%5B12%5D=18§ion_type_id%5B13%5D=9§ion_type_id%5B14%5D=17§ion_type_id%5B15%5D=22"
},
"evaluateSections": {
"href": "/models/assessment-section?assessment_id=734419§ion_type_id%5B0%5D=4§ion_type_id%5B1%5D=5§ion_type_id%5B2%5D=10§ion_type_id%5B3%5D=13§ion_type_id%5B4%5D=15§ion_type_id%5B5%5D=14§ion_type_id%5B6%5D=18§ion_type_id%5B7%5D=20§ion_type_id%5B8%5D=19§ion_type_id%5B9%5D=17§ion_type_id%5B10%5D=21§ion_type_id%5B11%5D=22§ion_type_id%5B12%5D=7"
},
"allSections": {
"href": "/models/assessment-section?assessment_id=734419"
},
"added": {
"href": "/models/assessment-candidate?assessment_id=734419&status_sort_key=10"
},
"invited": {
"href": "/models/assessment-candidate?assessment_id=734419&status_sort_key=20"
},
"attended": {
"href": "/models/assessment-candidate?assessment_id=734419&status_sort_key=50"
},
"rejected": {
"href": "/models/assessment-candidate?assessment_id=734419&status_sort_key=90"
},
"evaluated": {
"href": "/models/assessment-candidate?assessment_id=734419&status_sort_key=60"
},
"selected": {
"href": "/models/assessment-candidate?assessment_id=734419&status_sort_key=70"
},
"hold": {
"href": "/models/assessment-candidate?assessment_id=734419&status_sort_key=80"
},
"inProgress": {
"href": "/models/assessment-candidate?assessment_id=734419&status_sort_key=30"
}
}
}
HTTP Request
PUT https://apiv4.talview.com/models/assessment/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
title | string | true | The title of the Assessment |
description | string | true | The description of the Assessment |
description | text | false | The description of the assessment |
start_date | integer | false | The date from when the Assessment is active and can be attended |
end_date | integer | false | The last date and time to attend the assessment |
owned_by | integer | false | Filter records based on the Id of the owner who created an Assessment |
Delete an Assessment
DELETE /models/assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns a status code : 204 No Content
Deletes the details of an assessment based on the assessment id mentioned in the URL.
HTTP Request
DELETE https://apiv4.talview.com/models/assessment/<id>
Assessment Candidate
The Assessment Candidate endpoint provides all the Assessment status details of a Candidate who have been invited to any of the Assessment. A candidate can be invited to one or more Assessments.
The Assessment Candidate Object
{
"id": 1556661,
"assessment_id": 682126,
"candidate_id": 5720911,
"status_id": 2,
"invited_by": 30876,
"proviewRating": null,
"invited_at": "2019-01-11 09:48:59+00",
"attended_at": null,
"evaluated_at": null,
"created_at": "2019-01-11 09:48:59+00",
"updated_at": "2019-01-11 09:48:59+00",
"_links": {
"assessmentSectionCandidates": {
"href": "/models/assessment-section-candidate?assessment_candidate_id=1556661"
},
"evaluations": {
"href": "/models/evaluation?assessment_candidate_id=1556661"
},
"assessment": {
"href": "/models/assessment/682126"
},
"candidate": {
"href": "/models/candidate/5720911"
},
"files": {
"href": "/models/file?assessment_candidate_id=1556661"
},
"tbiReport": {
"href": "/models/file?assessment_candidate_id=1556661&file_type_id=40"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
assessment_id | integer | The id of an Assessment |
candidate_id | integer | The id of the Candidate who is invited for an Assessment |
status_id | smallint | The status of an Assessment Candidate. Example: Invited, Hold. The available Status for Assessment Candidate are mentioned in the enumerator list below |
invited_by | integer | The Id of the User who invited the Assessment |
proviewRating | integer | Indicate whether the candidate has cheated during the Assessment by switching to other Tab,going offline etc. High means No cheating, Low means cheating |
invited_at | timestamptz | The date and time when the candidate was invited to an Assessment |
attended_at | timestamptz | The date and time when the candidate attended an Assessment |
evaluated_at | timestamptz | The date and time when the Assessment was evaluated by the Evaluator |
List of Assessment Candidate Status:
Status | Key | Description |
---|---|---|
Invited | 10 | The candidate has been invited for the Assessment |
Mailed | 20 | The Candidate has been sent invitation/reminder email for the Assessment |
Attending | 30 | The Candidate is attending the Assessment |
Completed | 50 | The Candidate has completed the Assessment |
Assessed | 60 | The Assessment of the Candidate has been Evaluated |
Selected | 70 | The Candidate has been Selected |
Hold | 80 | The Candidate is kept in hold |
Rejected | 90 | The Candidate has been Rejected |
Suspended | 100 | The Candidate has been Suspended |
List all Assessment Candidate
Returns all the candidates who have been assigned to an Assessment.
GET /models/assessment-candidate HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 346328,
"assessment_id": 89313,
"candidate_id": 1841093,
"status_id": 2,
"invited_by": 65996,
"invited_at": "2018-04-16 12:47:42+00",
"attended_at": null,
"evaluated_at": null,
"created_at": "2018-04-16 12:47:41+00",
"updated_at": "2018-04-16 12:47:41+00",
"_links": {
"assessmentSectionCandidates": {
"href": "/models/assessment-section-candidate?assessment_candidate_id=346328"
},
"assessment": {
"href": "/models/assessment/89313"
},
"candidate": {
"href": "/models/candidate/1841093"
},
"files": {
"href": "/models/file?assessment_candidate_id=346328"
},
"tbiReport": {
"href": "/models/file?assessment_candidate_id=346328&file_type_id=40"
}
}
},
{
"id": 346327,
"assessment_id": 14427,
"candidate_id": 5755874,
"status_id": 3,
"invited_by": 23617,
"proviewRating": null,
"invited_at": "2019-01-19 14:49:51+05:30",
"attended_at": null,
"evaluated_at": null,
"created_at": "2019-01-19 14:49:51+05:30",
"updated_at": "2019-01-19 14:52:45+05:30",
"_links": {
"assessmentSectionCandidates": {
"href": "/models/assessment-section-candidate?assessment_candidate_id=1590541"
},
"evaluations": {
"href": "/models/evaluation?assessment_candidate_id=1590541"
},
"assessment": {
"href": "/models/assessment/14427"
},
"candidate": {
"href": "/models/candidate/5755874"
},
"files": {
"href": "/models/file?assessment_candidate_id=1590541"
},
"tbiReport": {
"href": "/models/file?assessment_candidate_id=1590541&file_type_id=40"
}
}
}
]
HTTP Request
GET https://apiv4.talview.com/models/assessment-candidate
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
assessment_id | integer | false | Filters records based on Assessment Id |
candidate_id | integer | false | Filter records based on Candidate Id |
s | text | false | Filter records by any of the following field of Candidate - first_name, last_name, email, external_id |
status_id | integer | false | Filter records based on Status Id |
panel_id | integer | false | Filter records based on Panel Id. Panels are assigned for the Assessment Section |
isPendingInvite | integer | false | Filter records based on pending invites |
isUnassigned | integer | false | Filter records based on Assessment for which Panel is not assigned |
isPendingEval | integer | false | Filter records based on Assessment whose evaluation is pending |
isRecentEval | integer | false | Filter records based on recent evaluated Assessment |
Retrieve an Assessment Candidate
Retrieves the details of all the candidates who are being invited for an Assessment based on the Id provided in the URl.
GET /models/assessment-candidate/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1556661,
"assessment_id": 682126,
"candidate_id": 5720911,
"status_id": 2,
"invited_by": 30876,
"proviewRating": null,
"invited_at": "2019-01-11 09:48:59+00",
"attended_at": null,
"evaluated_at": null,
"created_at": "2019-01-11 09:48:59+00",
"updated_at": "2019-01-11 09:48:59+00",
"_links": {
"assessmentSectionCandidates": {
"href": "/models/assessment-section-candidate?assessment_candidate_id=1556661"
},
"evaluations": {
"href": "/models/evaluation?assessment_candidate_id=1556661"
},
"assessment": {
"href": "/models/assessment/682126"
},
"candidate": {
"href": "/models/candidate/5720911"
},
"files": {
"href": "/models/file?assessment_candidate_id=1556661"
},
"tbiReport": {
"href": "/models/file?assessment_candidate_id=1556661&file_type_id=40"
}
}
}
HTTP Request
GET https://apiv4.talview.com/models/assessment-candidate/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Update an Assessment Candidate
Update an Assessment Candidate based on the id provided.
PUT /models/assessment-candidate/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1556661,
"assessment_id": 682126,
"candidate_id": 5720911,
"status_id": 2,
"invited_by": 30876,
"proviewRating": null,
"invited_at": "2019-01-11 09:48:59+00",
"attended_at": null,
"evaluated_at": null,
"created_at": "2019-01-11 09:48:59+00",
"updated_at": "2019-01-11 09:48:59+00",
"_links": {
"assessmentSectionCandidates": {
"href": "/models/assessment-section-candidate?assessment_candidate_id=1556661"
},
"evaluations": {
"href": "/models/evaluation?assessment_candidate_id=1556661"
},
"assessment": {
"href": "/models/assessment/682126"
},
"candidate": {
"href": "/models/candidate/5720911"
},
"files": {
"href": "/models/file?assessment_candidate_id=1556661"
},
"tbiReport": {
"href": "/models/file?assessment_candidate_id=1556661&file_type_id=40"
}
}
}
HTTP Request
PUT https://apiv4.talview.com/models/assessment-candidate/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessment_id | integer | false | The id of an Assessment |
candidate_id | integer | false | The id of the Candidate who is invited for an Assessment |
status_id | integer | false | The id of the Assessment Status. The Assessment status are mentioned in the Assessment Model |
proviewRating | integer | false | Indicate whether the candidate has cheated during the Assessment by switching to other Tab,going offline etc. High means No cheating, Low means cheating |
attended_at | timestamptz | false | The date and time when the candidate attended an Assessment |
evaluated_at | timestamptz | false | The date and time when the Assessment was evaluated by the Evaluator |
Delete an Assessment Candidate
Deletes an Assessment Candidate based on the id provided.
DELETE /models/assessment-candidate/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns status code : 204 No Content
HTTP Request
DELETE https://apiv4.talview.com/models/assessment-candidate/<id>
Assessment Candidate File
The Assessment Candidate File endpoint gives the details of uploaded file related to a Candidate invited for the Assessment(Assessment Candidate).
Example: Resume.
The Assessment Candidate File Object
{
"id": 2984863,
"assessment_candidate_id": 142789,
"assessment_section_candidate_id": 131162,
"file_id": 4805609
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
assessment_candidate_id | integer | The Id of the Assessment Candidate |
assessment_section_candidate_id | integer | The Id of the Assessment Section Candidate |
file_id | integer | The Id of the File attached |
List all Assessment Candidate File
This end point returns all the files of the candidates assigned to an assessment.
GET /models/assessment-candidate-file HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 2984863,
"assessment_candidate_id": 142789,
"assessment_section_candidate_id": 131162,
"file_id": 4805609
},
{
"id": 2984864,
"assessment_candidate_id": 142789,
"assessment_section_candidate_id": 131162,
"file_id": 4805610
},
{
"id": 2984865,
"assessment_candidate_id": 142789,
"assessment_section_candidate_id": 131162,
"file_id": 4805611
}
]
HTTP Request
GET https://apiv4.talview.com/models/assessment-candidate-file
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
assessment_candidate_id | integer | false | Filter based on assessment_candidate_id |
assessment_section_candidate_id | integer | false | Filter based on assessment_section_candidate_id |
file_id | integer | false | Filter records based on File Id |
Retrieve an Assessment Candidate File
Retrieve a file based on the assessment id mentioned in the URL.
GET /models/assessment-candidate-file/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 2984863,
"assessment_candidate_id": 142789,
"assessment_section_candidate_id": 131162,
"file_id": 4805609
}
HTTP Request
GET https://apiv4.talview.com/models/assessment-candidate-file/id
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter based on Id |
Create a new Assessment Candidate File
Creates a file for the candidate assigned with an assessment.
POST /models/assessment-candidate-file HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 4065678,
"assessment_candidate_id": 142789,
"assessment_section_candidate_id": 131162,
"file_id": 4805609
}
HTTP Request
POST https://apiv4.talview.com/models/assessment-candidate-file
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
file_id | integer | true | The Id of the File attached |
assessment_candidate_id | integer | true | The Id of the Assessment Candidate |
assessment_section_candidate_id | integer | true | The Id of the Assessment Section Candidate |
Update an Assessment Candidate File
PUT /models/assessment-candidate-file/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 4065678,
"assessment_candidate_id": 142789,
"assessment_section_candidate_id": 131162,
"file_id": 4805609
}
This endpoint updates an existing Assessment Candidate file.
HTTP Request
PUT https://apiv4.talview.com/models/assessment-candidate-file/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Deletes an Assessment Candidate File
Deletes the details of candidate file based on the id mentioned in the URL.
DELETE /models/assessment-candidate-file/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns status code: 204 No Content
HTTP Request
DELETE https://apiv4.talview.com/models/assessment-candidate-file/<id>
Assessment Category
The Assessment Category endpoint gives all the details of the Categorised Assesment.
The Assessment Category Object
{
"id": 1,
"assessment_id": 3127,
"category_id": 9,
"created_by": null,
"created_at": "2016-04-19 11:39:01+05:30",
"updated_by": null,
"updated_at": null
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
assessment_id | integer | The Id of the Assesment |
category_id | integer | The Id of the Category |
List all Assessment Category
Returns all the details of the Assessment Category.
GET /models/assessment-category HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 1,
"assessment_id": 3127,
"category_id": 9,
"created_by": null,
"created_at": "2016-04-19 11:39:01+05:30",
"updated_by": null,
"updated_at": null
},
{
"id": 2,
"assessment_id": 3129,
"category_id": 9,
"created_by": null,
"created_at": "2016-04-19 11:39:01+05:30",
"updated_by": null,
"updated_at": null
},
...
]
HTTP Request
GET https://apiv4.talview.com/models/assessment-category
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the object |
assessment_id | integer | false | Filter records based on the Id of the Assessment |
category_id | integer | false | Filter records based on the Id of the Category |
Retrieve an Assessment Category
Retrieve an assessment category based on the id mentioned in the URL.
GET /models/assessment-category/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1,
"assessment_id": 3127,
"category_id": 9,
"created_by": null,
"created_at": "2016-04-19 11:39:01+05:30",
"updated_by": null,
"updated_at": null
}
HTTP Request
GET https://apiv4.talview.com/models/assessment-category/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create an Assessment Category
POST /models/assessment-category/ HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1,
"assessment_id": 3127,
"category_id": 9,
"created_by": null,
"created_at": "2016-04-19 11:39:01+05:30",
"updated_by": null,
"updated_at": null
}
This end point Creates a new Assessment Category.
HTTP Request
POST https://apiv4.talview.com/models/assessment-category
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessment_id | integer | true | The Id of the Assessment |
category_id | integer | true | The Id of the Category |
Updates an Assessment Category
This endpoint Updates an Assessment Category.
PUT /models/assessment-category/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1,
"assessment_id": 3127,
"category_id": 9,
"created_by": null,
"created_at": "2016-04-19 11:39:01+05:30",
"updated_by": null,
"updated_at": null
}
HTTP Request
PUT https://apiv4.talview.com/models/assessment-category/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
arameter | Type | Mandatory | Description |
---|---|---|---|
assessment_id | integer | false | The Id of the Assessment |
category_id | integer | false | The Id of the Category |
Delete an Assessment Category
DELETE /models/assessment-category/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This end point Deletes an Assessment Category
HTTP Request
DELETE https://apiv4.talview.com/models/assessment-category/<id>
Assessment Collaborator
The Assessment Collaborator end point gives the details of all the User who are associated with some Assessment. Collaborators have limited access to the Application and work collaboratively with the core User.
The Assessment Collaborator Object
{
"id": 1,
"role": 1,
"assessment_id": 21807,
"user_id": 30848,
"created_by": 30848,
"created_at": "2017-11-21 02:08:28+00",
"updated_by": 30848,
"updated_at": "2017-11-21 02:08:28+00"
}
Parameter | Type | Description |
---|---|---|
id | bigint | The unique identifier of the object |
role | smallint | The role of the User as collaborator |
assessment_id | integer | The id of the Assessment |
user_id | integer | The id of the User |
List all Assessment Collaborator
GET /models/assessment-collaborator HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 10951601,
"role": 1,
"assessment_id": 708720,
"user_id": 169297,
"created_by": 31117,
"created_at": "2019-02-08 04:32:00+00",
"updated_by": 31117,
"updated_at": "2019-02-08 04:32:00+00"
},
{
"id": 10951611,
"role": 1,
"assessment_id": 708720,
"user_id": 169320,
"created_by": 31117,
"created_at": "2019-02-08 04:49:55+00",
"updated_by": 31117,
"updated_at": "2019-02-08 04:49:55+00"
}
...
]
Returns all the assessment collaborators.
HTTP Request
GET https://apiv4.talview.com/models/assessment-collaborator
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
role | integer | true | Filter records based on role |
assessment_id | integer | true | Filter records based on the Id of the Assessment |
user_id | integer | true | Filter records based on the Id of the User |
Retrieve an Assessment Collaborator
GET /models/assessment-collaborator/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 10951601,
"role": 1,
"assessment_id": 708720,
"user_id": 169297,
"created_by": 31117,
"created_at": "2019-02-08 04:32:00+00",
"updated_by": 31117,
"updated_at": "2019-02-08 04:32:00+00"
}
Retrieve an assessment collaborators.
HTTP Request
GET https://apiv4.talview.com/models/assessment-collaborator/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create an Assessment Collaborator
POST /models/assessment-collaborator HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1,
"role": 1,
"assessment_id": 21807,
"user_id": 30848,
"created_by": 30848,
"created_at": "2017-11-21 02:08:28+00",
"updated_by": 30848,
"updated_at": "2017-11-21 02:08:28+00"
}
This end point adds an assessment collaborator.
HTTP Request
POST https://apiv4.talview.com/models/assessment-collaborator
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
role | smallint | true | The role of the User as collaborator |
assessment_id | integer | true | The id of the Assessment |
user_id | integer | true | The id of the User |
Updates an Assessment Collaborator
PUT /models/assessment-collaborator/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1,
"role": 1,
"assessment_id": 21807,
"user_id": 30848,
"created_by": 30848,
"created_at": "2017-11-21 02:08:28+00",
"updated_by": 30848,
"updated_at": "2017-11-21 02:08:28+00"
}
Updates an assessment collaborator.
HTTP Request
PUT https://apiv4.talview.com/models/assessment-collaborator
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
role | smallint | false | The role of the User as collaborator |
Delete an Assessment Collaborator
DELETE /models/assessment-collaborator HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns status code : 204 No Content
Deletes an assessment collaborator.
HTTP Request
DELETE https://apiv4.talview.com/models/assessment-collaborator
Assessment Section
The Assessment Section endpoint provides all the details of the Sections attached to an Assessment. An Assessment can have more then one section attached to it.
The Assessment Section Object
{
"id": 128203,
"is_public": false,
"is_proctor": true,
"is_review": false,
"isSkipAllowed": true,
"is_visible": true,
"is_retake": false,
"isRetake": false,
"start_date": null,
"end_date": null,
"isPlaylist": true,
"section_id": 1,
"assessment_id": 21318,
"publicLinkUrl": null,
"max_section_time": null,
"max_question_time": null,
"questionDisplayTime": 11,
"intro": null,
"isObjectiveScoreBreakup": true,
"conclusion": null,
"reportUrl": null,
"cut_off": null,
"positive_mark": "4.00",
"negative_mark": "1.00",
"evaluation_start_date": null,
"evaluation_end_date": null,
"proview_type" : "ai_proctor",
"created_at": "2017-11-03 15:46:17+00",
"updated_at": "2019-03-12 09:04:06+00",
"_links": {
"unassigned": {
"href": "/models/assessment-section-candidate?assessment_section_id=128203&unassigned=1"
},
"parameters": {
"href": "/models/parameter?section_id=1"
},
"assessment": {
"href": "/models/assessment/21318"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
is_public | boolean | If true, the Assessment is public. Public Assessment is open and can be attempted by any candidate by registering themself, and in case of private Assessment only invited Candidate can attempt the Assessment |
is_proctor | boolean | Whether the proctor is available for a particular Assessment Section |
is_review | boolean | If true, the review of all the question of that section will be displayed at last |
isSkipAllowed | boolean | If true,skipping of question is allowed |
is_retake | boolean | Whether the question in the section can be retaken |
isRetake | boolean | Whether the question in the section can be retaken |
start_date | timestamptz | The date and time from when the Assessment Section is active and can be attended |
end_date | timestamptz | The last date and time to attend an Assessment |
isPlaylist | boolean | If true, list of all questions will be displayed all the time during assessment |
section_id | integer | The Id of the Section |
assessment_id | integer | The id of the Assessment |
publicLinkUrl | string | Link for the candidate to register themselves for an Assessment Section |
max_section_time | interval second | Maximum time to attempt the Assessment Section |
max_question_time | interval second | Maximum time to attempt the questions of the Section. |
questionDisplayTime | interval second | Time duration for displaying the question |
intro | text | Introduction of the Assessment Section |
isObjectiveScoreBreakup | boolean | Whether the objective score can be broke up |
conclusion | text | Conclusion message of the Assessment Section |
reportUrl | string | The url for getting section level report of the Assessment Section |
cut_off | integer | Cut off mark for the assessment section |
positive_mark | integer | Positive mark for each question of Assessment Section |
negative_mark | integer | Negative mark for each question of Assessment Section |
evaluation_start_date | timestamptz | The date and time when the evaluation of the Assessment Section is started |
evaluation_end_date | timestamptz | The date and time when the evaluation of the Assessment Section is ended |
proview_type | string | The type of proview session. The allowrd session types are listed below |
Type of allowed proview sesstion types
Session Type | Description |
---|---|
ai_proctor | AI Proctoring Session. In this, the proctored session is evaluated automatically |
live_proctor | Live Proctoring Session. In this, the proctored session is evaluated live by panel/proctor |
candidate_auth | Candidate Authentication. This is proctoring solution for Live and Asyc interview types |
record_and_review | Record and Review. In this, the proview recordings are reviewed by the proctor. |
List all Assessment Section
GET /models/assessment-section HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
[
{
"id": 128203,
"is_public": false,
"is_proctor": true,
"is_review": false,
"isSkipAllowed": true,
"is_visible": true,
"is_retake": false,
"isRetake": false,
"start_date": null,
"end_date": null,
"isPlaylist": true,
"section_id": 1,
"assessment_id": 21318,
"publicLinkUrl": null,
"max_section_time": null,
"max_question_time": null,
"questionDisplayTime": 11,
"intro": null,
"isObjectiveScoreBreakup": true,
"conclusion": null,
"reportUrl": null,
"cut_off": null,
"positive_mark": "4.00",
"negative_mark": "1.00",
"evaluation_start_date": null,
"evaluation_end_date": null,
"proview_type" : "ai_proctor",
"created_at": "2017-11-03 15:46:17+00",
"updated_at": "2019-03-12 09:04:06+00",
"_links": {
"unassigned": {
"href": "/models/assessment-section-candidate?assessment_section_id=128203&unassigned=1"
},
"parameters": {
"href": "/models/parameter?section_id=1"
},
"assessment": {
"href": "/models/assessment/21318"
}
}
}
...
]
Returns all the Assessment Section
HTTP Request
GET https://apiv4.talview.com/models/assessment-section
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
assessment_id | integer | false | Filter records based on the Id of Assessment |
section_type_id | integer | false | Filter records based on Section Type |
Retrieve an Assessment Section
GET /models/assessment-section/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 128203,
"is_public": false,
"is_proctor": true,
"is_review": false,
"isSkipAllowed": true,
"is_visible": true,
"is_retake": false,
"isRetake": false,
"start_date": null,
"end_date": null,
"isPlaylist": true,
"section_id": 1,
"assessment_id": 21318,
"publicLinkUrl": null,
"max_section_time": null,
"max_question_time": null,
"questionDisplayTime": 11,
"intro": null,
"isObjectiveScoreBreakup": true,
"conclusion": null,
"reportUrl": null,
"cut_off": null,
"positive_mark": "4.00",
"negative_mark": "1.00",
"evaluation_start_date": null,
"evaluation_end_date": null,
"created_at": "2017-11-03 15:46:17+00",
"updated_at": "2019-03-12 09:04:06+00",
"proview_type" : "ai_proctor",
"_links": {
"unassigned": {
"href": "/models/assessment-section-candidate?assessment_section_id=128203&unassigned=1"
},
"parameters": {
"href": "/models/parameter?section_id=1"
},
"assessment": {
"href": "/models/assessment/21318"
}
}
}
Retrieve an Assessment Section.
HTTP Request
GET https://apiv4.talview.com/models/assessment-section/<id>
URL Parameters
Parameter | type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create an Assessment Section
POST /models/assessment-section HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 842829,
"is_public": false,
"is_proctor": true,
"is_retake": false,
"start_date": null,
"end_date": null,
"section_id": 1,
"assessment_id": 103545,
"max_section_time": null,
"max_question_time": null,
"questionDisplayTime": 3,
"intro": null,
"isObjectiveScoreBreakup": true,
"conclusion": null,
"cut_off": null,
"positive_mark": "4.00",
"negative_mark": "1.00",
"evaluation_start_date": null,
"evaluation_end_date": null,
"proview_type" : "ai_proctor",
"created_at": "2018-04-07 08:52:05+00",
"updated_at": "2018-04-07 08:52:05+00",
"_links": {
"parameters": {
"href": "/models/parameter?section_id=1"
},
"assessment": {
"href": "/models/assessment/103545"
}
}
}
This endpoint creates an Assessment Section.
HTTP Request
POST https://apiv4.talview.com/models/assessment-section
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
is_review | boolean | true | If true, the review of all the question of that section will be displayed at last |
isSkipAllowed | boolean | true | If true,skipping of question is allowed |
isRetake | boolean | true | Whether the question in the section can be retaken |
isPlaylist | boolean | true | If true, list of all questions will be displayed all the time during assessment |
section_id | integer | true | The Id of the Section |
assessment_id | integer | true | The id of the Assessment |
section_type_id | integer | true | The Id of the Section type |
max_section_time | interval second | true, when Section type is Objective, Essay, Simulation, Code | Maximum time to attempt the Assessment Section |
max_question_time | interval second | true, When Section type is interview | Maximum time to attempt the questions of the Section |
questionDisplayTime | interval second | true | Time duration for displaying the question |
intro | text | true | Introduction of the Assessment Section |
conclusion | text | true | Conclusion message of the Assessment Section |
cut_off | integer | true, when Section type id Objective | Cut off mark for the assessment section |
positive_mark | integer | true, when Section type id Objective | Positive mark for each question of Assessment Section |
negative_mark | integer | true, when Section type id Objective | Negative mark for each question of Assessment Section |
evaluation_end_date | timestamptz | true | The date and time when the evaluation of the Assessment Section is ended |
proview_type | string | false | The type of proview session |
Update an Assessment Section
PUT /models/assessment-section/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
{
"id": 128203,
"is_public": false,
"is_proctor": true,
"is_review": false,
"isSkipAllowed": true,
"is_visible": true,
"is_retake": false,
"isRetake": false,
"start_date": null,
"end_date": null,
"isPlaylist": true,
"section_id": 1,
"assessment_id": 21318,
"publicLinkUrl": null,
"max_section_time": null,
"max_question_time": null,
"questionDisplayTime": 11,
"intro": null,
"isObjectiveScoreBreakup": true,
"conclusion": null,
"reportUrl": null,
"cut_off": null,
"positive_mark": "4.00",
"negative_mark": "1.00",
"evaluation_start_date": null,
"evaluation_end_date": null,
"proview_type" : "ai_proctor",
"created_at": "2017-11-03 15:46:17+00",
"updated_at": "2019-03-12 09:04:06+00",
"_links": {
"unassigned": {
"href": "/models/assessment-section-candidate?assessment_section_id=128203&unassigned=1"
},
"parameters": {
"href": "/models/parameter?section_id=1"
},
"assessment": {
"href": "/models/assessment/21318"
}
}
}
This endpoint updates an Assessment Section.
HTTP Request
PUT https://apiv4.talview.com/models/assessment-section/id
URL Parameters
Parameter | type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
is_review | boolean | false | If true, the review of all the question of that section will be displayed at last |
isSkipAllowed | boolean | false | If true,skipping of question is allowed |
isRetake | boolean | false | Whether the question in the section can be retaken |
isPlaylist | boolean | false | If true, list of all questions will be displayed all the time during assessment |
section_id | integer | false | The Id of the Section |
max_section_time | interval second | false | Maximum time to attempt the Assessment Section |
max_question_time | interval second | false | Maximum time to attempt the questions of the Section |
questionDisplayTime | interval second | false | Time duration for displaying the question |
intro | text | false | Introduction of the Assessment Section |
conclusion | text | false | Conclusion message of the Assessment Section |
cut_off | integer | false | Cut off mark for the assessment section |
positive_mark | integer | true | Positive mark for each question of Assessment Section |
negative_mark | integer | true | Negative mark for each question of Assessment Section |
evaluation_end_date | timestamptz | true | The date and time when the evaluation of the Assessment Section is ended |
proview_type | string | false | The type of proview session |
Delete an Assessment Section
DELETE /models/assessment-section/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns a status code: 204 No Content
This endpoint deletes an assessment section.
HTTP Request
DELETE https://apiv4.talview.com/models/assessment-section/id
Assessment Section Candidate
The Assessment Section Candidate endpoint provides the details of the Assessment Section mapped to a Candidate.
The Assessment Section Candidate Object
{
"id": 2091612,
"external_id": null,
"assessment_candidate_id": 1936000,
"timeElapsed": 30,
"isComplete": true,
"section_id": 3513028,
"assessment_id": 859988,
"assessment_section_id": 6915639,
"c": "UosxGRZ9zVWcksoxG7VtbCFuTEx1dgm5kHWxshvGLogxNTUyNDU3ODk5MjA5MTYxMg__",
"preTemplate": "",
"verification_code": "328633399",
"start_date": null,
"externalComplete": false,
"end_date": "2019-03-22 11:41:55+00",
"isRegistered": true,
"intro": null,
"conclusion": null,
"hasBooked": false,
"duration": "2 - 3 minutes",
"started_at": null,
"postAssessmentUrl": null,
"reportUrl": null,
"status_id": 14,
"completed_at": "2019-03-12 12:00:44+00",
"status_reason_id": null,
"status": {
"id": 14,
"sort_key": 60,
"label": "Evaluated",
"type": 20,
"created_at": "2016-04-19 06:09:01+00",
"updated_at": null
},
"created_at": "2019-03-12 11:42:04+00",
"updated_at": "2019-03-13 06:17:26+00",
"evaluated_at": null,
"score": "0.00",
"invited_at": "2019-03-12 11:42:05+00",
"created_by": 234078,
"proctorLog": null,
"updated_by": 234078,
"positiveScore": 0,
"negativeScore": 0,
"_links": {
"self": {
"href": "/models/assessment-section-candidate?id=2091612"
},
"answers": {
"href": "/models/answer?assessment_section_candidate_id=2091612"
},
"formInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612"
},
"preFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612&type%5B0%5D=3&type%5B1%5D=17&type%5B2%5D=18"
},
"evaluationFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612&type=2"
},
"evaluations": {
"href": "/models/evaluation?assessment_section_candidate_id=2091612"
},
"selfEvaluations": {
"href": "/models/evaluation?assessment_section_candidate_id=2091612&self=1"
},
"panelCandidates": {
"href": "/models/panel-candidate?assessment_section_candidate_id=2091612"
},
"status": {
"href": "/status?id=14"
},
"index": {
"href": "/models/assessment-section-candidate"
},
"reports": {
"href": "/models/file?assessment_section_candidate_id=2091612&file_type_id%5B0%5D=31&file_type_id%5B1%5D=40"
},
"liveSessions": {
"href": "/models/live-session?assessment_section_candidate_id=2091612"
},
"liveSessionsWithRecording": {
"href": "/models/live-session?assessment_section_candidate_id=2091612&expand=recordings"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
external_id | string | The reference Id for the object |
assessment_candidate_id | integer | The Id of the Candidate Associated with an Assessment(Assessment Candidate) |
timeElapsed | timestamptz | The remainig time of the Assessment Section |
isComplete | boolean | If true, the Assessment Section is attempted by the Candidate and is complete |
section_id | integer | The Id of the Section |
assessment_id | integer | The Id of the Assessment |
assessment_section_id | integer | The Id of the Assessment section |
c | string | The hash value for the Id |
preTemplate | string | Template for customising verification page |
verification_code | integer | The Verification Code for the Assessment Section that is sent to the candidate to verify the Assessment. The candidate must enter the verification code to attempt the Assessment Section. |
start_date | timestamptz | The date and time from when the Assessment Section is active for a particular Candidate |
externalComplete | boolean | Whether the external evaluation is complete |
end_date | timestamptz | The date and time until when the Assessment Section is active for a particular Candidate |
isRegistered | boolean | If true, the Candidate has registered for the Assessment Section |
intro | text | The introduction or the instruction to the candidate before attending the Assessment Section |
conclusion | text | The Conclusion message to the Candidate after completing the Assessment Section |
duration | string | Duration of the Assessment Section |
started_at | timestamptz | The date and time when the candidate started attempting the Assessment Section |
postAssessmentUrl | string | The Url that is provided after the completion of the Assessment Section. This is Optional |
reportUrl | string | The Url for the report of the Assessment Section Candidate |
status_id | integer | The status of the Assessment Candidate. Example: Invited, Selected. The available Status for Assessment Section Candidate are mentioned in the enumerator list below |
completed_at | timestamptz | The date and time when the candidate completed attempting the Assessment Section |
status_reason_id | integer | The Id of the Status Reason |
status | object | The object of the Status |
evaluated_at | timestamptz | The date and time when the Assessment Section Candidate was evaluated |
score | integer | The score obtained by a particular candidate for the Assessment Candidate |
invited_at | timestamptz | The date and time when the Candidate was invited for the Assessment Section |
created_by | integer | The Id of the User who created the Assessment Section. User may be Master Recruiter or the Recruiter. |
proctorLog | integer | The proctoLog of the Assessment Section Candidate |
updated_by | integer | The Id of the User who updated the Assessment Section. User may be Master Recruiter or the Recruiter. |
positiveScore | integer | The positive score for the Assessment Section |
negativeScore | integer | The negative score for the Assessment Section |
List of Assessment Section Candidate Status:
Status | Key | Description |
---|---|---|
Invited | 10 | The candidate has been invited for the Assessment |
Mailed | 20 | The Candidate has been sent invitation/reminder email for the Assessment |
Attending | 30 | The Candidate is attending the Assessment |
Completed | 50 | The Candidate has completed the Assessment |
Assessed | 60 | The Assessment of the Candidate has been Evaluated |
Selected | 70 | The Candidate has been Selected |
Hold | 80 | The Candidate is kept in hold |
Rejected | 90 | The Candidate has been Rejected |
Suspended | 100 | The Candidate has been Suspended |
List all Assessment Section Candidate
GET /models/assessment-section-candidate HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 2091612,
"external_id": null,
"assessment_candidate_id": 1936000,
"timeElapsed": 30,
"isComplete": true,
"section_id": 3513028,
"assessment_id": 859988,
"assessment_section_id": 6915639,
"c": "UosxGRZ9zVWcksoxG7VtbCFuTEx1dgm5kHWxshvGLogxNTUyNDU3ODk5MjA5MTYxMg__",
"preTemplate": "",
"verification_code": "328633399",
"start_date": null,
"externalComplete": false,
"end_date": "2019-03-22 11:41:55+00",
"isRegistered": true,
"intro": null,
"conclusion": null,
"hasBooked": false,
"duration": "2 - 3 minutes",
"started_at": null,
"postAssessmentUrl": null,
"reportUrl": null,
"status_id": 14,
"completed_at": "2019-03-12 12:00:44+00",
"status_reason_id": null,
"status": {
"id": 14,
"sort_key": 60,
"label": "Evaluated",
"type": 20,
"created_at": "2016-04-19 06:09:01+00",
"updated_at": null
},
"created_at": "2019-03-12 11:42:04+00",
"updated_at": "2019-03-13 06:17:26+00",
"evaluated_at": null,
"score": "0.00",
"invited_at": "2019-03-12 11:42:05+00",
"created_by": 234078,
"proctorLog": null,
"updated_by": 234078,
"positiveScore": 0,
"negativeScore": 0,
"_links": {
"self": {
"href": "/models/assessment-section-candidate?id=2091612"
},
"answers": {
"href": "/models/answer?assessment_section_candidate_id=2091612"
},
"formInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612"
},
"preFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612&type%5B0%5D=3&type%5B1%5D=17&type%5B2%5D=18"
},
"evaluationFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612&type=2"
},
"evaluations": {
"href": "/models/evaluation?assessment_section_candidate_id=2091612"
},
"selfEvaluations": {
"href": "/models/evaluation?assessment_section_candidate_id=2091612&self=1"
},
"panelCandidates": {
"href": "/models/panel-candidate?assessment_section_candidate_id=2091612"
},
"status": {
"href": "/status?id=14"
},
"index": {
"href": "/models/assessment-section-candidate"
},
"reports": {
"href": "/models/file?assessment_section_candidate_id=2091612&file_type_id%5B0%5D=31&file_type_id%5B1%5D=40"
},
"liveSessions": {
"href": "/models/live-session?assessment_section_candidate_id=2091612"
},
"liveSessionsWithRecording": {
"href": "/models/live-session?assessment_section_candidate_id=2091612&expand=recordings"
}
}
}
...
]
This end point returns all the Assessment Section Candidate based on the Query Parameter passed.
HTTP Request
GET https://apiv4.talview.com/models/assessment-section-candidate
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
assessment_section_id | integer | false | Filter records based on Assessment Section Id |
status_id | integer | false | Filter records based on Status Id |
assessment_candidate_id | integer | false | Filter records based on the Id of Assessment(Assessment Candidate) |
section_id | integer | false | Filter records based on the Id of the Section |
assessment_id | integer | false | Filter records based on the Id of the Assessment |
assessment_section_id | integer | false | Filter records based on the Id of the Assessment section |
started_at | timestamptz | false | Filter records based on the time when the candidate started attempting the Assessment Section |
status_id | integer | false | Filter records based on the Id of the Assessment Status |
completed_at | timestamptz | false | Filter records based on the date and time of Completion of the Assessment Section |
evaluated_at | timestamptz | false | Filter records based on the date and time of Evaluation of the Assessment Section |
Retrieve a Assessment Section Candidate
GET /models/assessment-section-candidate/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 2091612,
"external_id": null,
"assessment_candidate_id": 1936000,
"timeElapsed": 30,
"isComplete": true,
"section_id": 3513028,
"assessment_id": 859988,
"assessment_section_id": 6915639,
"c": "UosxGRZ9zVWcksoxG7VtbCFuTEx1dgm5kHWxshvGLogxNTUyNDU3ODk5MjA5MTYxMg__",
"preTemplate": "",
"verification_code": "328633399",
"start_date": null,
"externalComplete": false,
"end_date": "2019-03-22 11:41:55+00",
"isRegistered": true,
"intro": null,
"conclusion": null,
"hasBooked": false,
"duration": "2 - 3 minutes",
"started_at": null,
"postAssessmentUrl": null,
"reportUrl": null,
"status_id": 14,
"completed_at": "2019-03-12 12:00:44+00",
"status_reason_id": null,
"status": {
"id": 14,
"sort_key": 60,
"label": "Evaluated",
"type": 20,
"created_at": "2016-04-19 06:09:01+00",
"updated_at": null
},
"created_at": "2019-03-12 11:42:04+00",
"updated_at": "2019-03-13 06:17:26+00",
"evaluated_at": null,
"score": "0.00",
"invited_at": "2019-03-12 11:42:05+00",
"created_by": 234078,
"proctorLog": null,
"updated_by": 234078,
"positiveScore": 0,
"negativeScore": 0,
"_links": {
"self": {
"href": "/models/assessment-section-candidate?id=2091612"
},
"answers": {
"href": "/models/answer?assessment_section_candidate_id=2091612"
},
"formInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612"
},
"preFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612&type%5B0%5D=3&type%5B1%5D=17&type%5B2%5D=18"
},
"evaluationFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612&type=2"
},
"evaluations": {
"href": "/models/evaluation?assessment_section_candidate_id=2091612"
},
"selfEvaluations": {
"href": "/models/evaluation?assessment_section_candidate_id=2091612&self=1"
},
"panelCandidates": {
"href": "/models/panel-candidate?assessment_section_candidate_id=2091612"
},
"status": {
"href": "/status?id=14"
},
"index": {
"href": "/models/assessment-section-candidate"
},
"reports": {
"href": "/models/file?assessment_section_candidate_id=2091612&file_type_id%5B0%5D=31&file_type_id%5B1%5D=40"
},
"liveSessions": {
"href": "/models/live-session?assessment_section_candidate_id=2091612"
},
"liveSessionsWithRecording": {
"href": "/models/live-session?assessment_section_candidate_id=2091612&expand=recordings"
}
}
}
This end point Retrieve the details of an existing assessment section candidate based on the Id passed.
HTTP Request
GET https://apiv4.talview.com/models/assessment-section-candidate/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Update a Assessment Section Candidate
PUT /models/assessment-section-candidate/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 2091612,
"external_id": null,
"assessment_candidate_id": 1936000,
"timeElapsed": 30,
"isComplete": true,
"section_id": 3513028,
"assessment_id": 859988,
"assessment_section_id": 6915639,
"c": "UosxGRZ9zVWcksoxG7VtbCFuTEx1dgm5kHWxshvGLogxNTUyNDU3ODk5MjA5MTYxMg__",
"preTemplate": "",
"verification_code": "328633399",
"start_date": null,
"externalComplete": false,
"end_date": "2019-03-22 11:41:55+00",
"isRegistered": true,
"intro": null,
"conclusion": null,
"hasBooked": false,
"duration": "2 - 3 minutes",
"started_at": null,
"postAssessmentUrl": null,
"reportUrl": null,
"status_id": 14,
"completed_at": "2019-03-12 12:00:44+00",
"status_reason_id": null,
"status": {
"id": 14,
"sort_key": 60,
"label": "Evaluated",
"type": 20,
"created_at": "2016-04-19 06:09:01+00",
"updated_at": null
},
"created_at": "2019-03-12 11:42:04+00",
"updated_at": "2019-03-13 06:17:26+00",
"evaluated_at": null,
"score": "0.00",
"invited_at": "2019-03-12 11:42:05+00",
"created_by": 234078,
"proctorLog": null,
"updated_by": 234078,
"positiveScore": 0,
"negativeScore": 0,
"_links": {
"self": {
"href": "/models/assessment-section-candidate?id=2091612"
},
"answers": {
"href": "/models/answer?assessment_section_candidate_id=2091612"
},
"formInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612"
},
"preFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612&type%5B0%5D=3&type%5B1%5D=17&type%5B2%5D=18"
},
"evaluationFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=2091612&type=2"
},
"evaluations": {
"href": "/models/evaluation?assessment_section_candidate_id=2091612"
},
"selfEvaluations": {
"href": "/models/evaluation?assessment_section_candidate_id=2091612&self=1"
},
"panelCandidates": {
"href": "/models/panel-candidate?assessment_section_candidate_id=2091612"
},
"status": {
"href": "/status?id=14"
},
"index": {
"href": "/models/assessment-section-candidate"
},
"reports": {
"href": "/models/file?assessment_section_candidate_id=2091612&file_type_id%5B0%5D=31&file_type_id%5B1%5D=40"
},
"liveSessions": {
"href": "/models/live-session?assessment_section_candidate_id=2091612"
},
"liveSessionsWithRecording": {
"href": "/models/live-session?assessment_section_candidate_id=2091612&expand=recordings"
}
}
}
This end point Updates the details of an existing Assessment Section for a particular Candidate.
HTTP Request
PUT https://apiv4.talview.com/models/assessment-section-candidate/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
start_date | timestamptz | false | The date and time from when the Assessment Section is active for a particular Candidate |
externalComplete | boolean | false | Whether the external evaluation is complete |
end_date | timestamptz | false | The date and time until when the Assessment Section is active for a particular Candidate |
status_id | integer | false | The status of the Assessment. Click here for the Status |
completed_at | timestamptz | false | The date and time when the candidate completed attempting the Assessment Section |
status_reason_id | integer | false | The Id of the Status Reason |
evaluated_at | timestamptz | false | The date and time when the Assessment Section Candidate was evaluated |
score | integer | false | The score obtained by a particular candidate for the Assessment Candidate |
Calendar
The Calendar endpoint stores the Calendar details of the logged in user. The User Calendar details can be set using the User Calendar endpoint.
The Calendar Object
{
"id": 3042793,
"from": "2019-03-13 05:10:00+00",
"to": "2019-03-13 07:10:00+00",
"eventType": "live-schedule",
"isAllDay": false,
"created_at": "2019-03-13 04:59:20+00",
"updated_at": "2019-03-13 04:59:20+00",
"event": "Live Session with Bidu Monu for Essay Parameters Checking ",
"type": 3,
"user_id": 45331,
"_links": {
"user": {
"href": "/models/user?id=45331"
},
"self": {
"href": "/models/user-calendar?id=3042793"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
from | timestamptz | The starting date and time of an event |
to | timestamptz | The ending date and time of an event |
eventType | text | The type of the event. Example: Available, Busy. The event types are described in the enumerator list below |
isAllDay | boolean | States whether is all day event |
event | string | The description of event |
type | integer | The key to an event type. The keys to the event types are mentioned in the enumerator list below |
user_id | integer | The id of the user associated to an user calendar object |
List of Event Types:
Event Types | Key | Description |
---|---|---|
Live-Schedule | 1 | A live session event has been scheduled |
Available | 2 | The user is available for an event |
Busy | 3 | The user is busy in an event |
Tentaive | 4 | The schedule is not certain |
List all Calendar Details
GET /user/me/calendar HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
Array of calendar Deatils for the current logged in user
[
{
"id": 3042793,
"from": "2019-03-13 05:10:00+00",
"to": "2019-03-13 07:10:00+00",
"eventType": "live-schedule",
"isAllDay": false,
"created_at": "2019-03-13 04:59:20+00",
"updated_at": "2019-03-13 04:59:20+00",
"event": "Live Session with Bidu Monu for Essay Parameters Checking ",
"type": 3,
"user_id": 45331,
"_links": {
"user": {
"href": "/models/user?id=45331"
},
"self": {
"href": "/models/user-calendar?id=3042793"
}
}
},
{
"id": 3042791,
"from": "2019-03-13 05:10:00+00",
"to": "2019-03-13 07:10:00+00",
"eventType": "live-schedule",
"isAllDay": false,
"created_at": "2019-03-13 04:59:19+00",
"updated_at": "2019-03-13 04:59:19+00",
"event": "Live Session with Bidu Monu for Essay Parameters Checking ",
"type": 3,
"user_id": 30876,
"_links": {
"user": {
"href": "/models/user?id=30876"
},
"self": {
"href": "/models/user-calendar?id=3042791"
}
}
}
...
]
This end point returns an array of calendar details
HTTP Request
GET https://apiv4.talview.com/user/me/calendar
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
from | timestamptz | false | Filter records based on the starting date and time of an event |
to | timestamptz | false | Filter records based on the end date and time of an event |
type | integer | false | Filter records based on an event type. The keys are mentioned in the enumerator list above |
user_id | integer | false | Filter records based on the User Id |
Add Calendar Details
POST /user/me/calendar HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
Request Body Sample
{
"from": "2018-03-21 19:30:00+00",
"to": "2018-03-21 20:30:00+00",
"event": "Available",
"type": 1
}
Response for new calendar event for logged-in user
{
"id": 3042791,
"from": "2019-03-13 05:10:00+00",
"to": "2019-03-13 07:10:00+00",
"eventType": "live-schedule",
"isAllDay": false,
"created_at": "2019-03-13 04:59:19+00",
"updated_at": "2019-03-13 04:59:19+00",
"event": "Live Session with Bidu Monu for Essay Parameters Checking ",
"type": 3,
"user_id": 30876,
"_links": {
"user": {
"href": "/models/user?id=30876"
},
"self": {
"href": "/models/user-calendar?id=3042791"
}
}
},
This end point adds a new Calendar details
HTTP Request
POST https://apiv4.talview.com/user/me/calendar
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
from | timestamptz | true | The time from when an event is scheduled |
to | timestamptz | true | The time when an event ends |
event | text | true | The name of the event |
type | integer | true | The key to an event type. The keys to the event types are mentioned in the enumerator list |
Update Calendar Details
PUT /user/me/calendar HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
Response for new calendar event for logged-in user
{
"id": 3042791,
"from": "2019-03-13 05:10:00+00",
"to": "2019-03-13 07:10:00+00",
"eventType": "live-schedule",
"isAllDay": false,
"created_at": "2019-03-13 04:59:19+00",
"updated_at": "2019-03-13 04:59:19+00",
"event": "Live Session with Bidu Monu for Essay Parameters Checking ",
"type": 3,
"user_id": 30876,
"_links": {
"user": {
"href": "/models/user?id=30876"
},
"self": {
"href": "/models/user-calendar?id=3042791"
}
}
}
It updates the calendar details based on id
HTTP Request
PUT https://apiv4.talview.com/user/me/calendar/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
from | timestamptz | false | The time from when an event is scheduled |
to | timestamptz | false | The time when an event ends |
type | integer | false | The key to an event type. The keys are mentioned in the enumerator list above |
event | text | false | The name of the event |
Delete Calendar Details
DELETE /user/me/calendar HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns status code : 204 No Content
This end point deletes the calendar details based on id
HTTP Request
DELETE https://apiv4.talview.com/user/me/calendar/<id>
Candidate
The Candidate endpoint gives the details of the Candidate.
Following are the ways to Create Candidate.
1) The Candidate can be created by Master Recruiter or the Recruiter. The Candidate can also be created in bulk.
2) If the Assessment is public, the link for the Assessment is shared publicly and a Candidate must Register himself before attempting the Assessment. Once the candidate register himself, the Candidate is created.
The Candidate Object
{
"id": 6507787,
"email": "amith.patel+12320191218@talview.com",
"first_name": "amith",
"middle_name": null,
"last_name": "patel",
"phone": "7410852963",
"external_id": null,
"assigned_to": null,
"status_id": 25,
"profile_pic": null,
"bio": null,
"cf": [],
"organization_id": 488,
"customFields": [],
"industry": null,
"isRegistered": false,
"icard": null,
"created_at": "2019-03-12 06:48:16+00",
"updated_at": "2019-03-12 11:59:19+00",
"_links": {
"image": {
"href": "/models/candidate-image/6507787"
},
"resume": {
"href": "/models/file?candidate_id=6507787&file_type_id=11"
},
"calendar": {
"href": "/models/candidate-calendar?candidate_id=6507787"
},
"assessmentCandidates": {
"href": "/models/assessment-candidate?candidate_id=6507787"
},
"candidateFileAttachments": {
"href": "/models/candidate-file-attachment?candidate_id=6507787"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
text | The email of the Candidate | |
first_name | text | The first name of the Candidate |
middle_name | text | The middle name of the Candidate |
last_name | text | The last name of the Candidate |
phone | text | The phone number of the Candidate |
assigned_to | integer | The Id of the Panel who has been assigned for the Candidate |
external_id | string | The reference Id for the object |
organization_id | integer | The Id of the organization to which the Candidate is associated. |
isRegistered | false | Whether the candidate has registered for the Assessment. This is for public Assessment |
cf | jsonb | Custom field details |
profile_pic | string | Profile picture of the Candidate |
status_id | integer | The status of the candidate .. example: Invited , attending. |
List all Candidate
GET /models/candidate HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 6507787,
"email": "amith.patel+12320191218@talview.com",
"first_name": "amith",
"middle_name": null,
"last_name": "patel",
"phone": "7410852963",
"external_id": null,
"assigned_to": null,
"status_id": 25,
"profile_pic": null,
"bio": null,
"cf": [],
"organization_id": 488,
"customFields": [],
"industry": null,
"isRegistered": false,
"icard": null,
"created_at": "2019-03-12 06:48:16+00",
"updated_at": "2019-03-12 11:59:19+00",
"_links": {
"image": {
"href": "/models/candidate-image/6507787"
},
"resume": {
"href": "/models/file?candidate_id=6507787&file_type_id=11"
},
"calendar": {
"href": "/models/candidate-calendar?candidate_id=6507787"
},
"assessmentCandidates": {
"href": "/models/assessment-candidate?candidate_id=6507787"
},
"candidateFileAttachments": {
"href": "/models/candidate-file-attachment?candidate_id=6507787"
}
}
},
{
"id": 6506985,
"email": "trideep.k+30@talview.com",
"first_name": "Trdp",
"middle_name": null,
"last_name": null,
"phone": "454545454545",
"external_id": null,
"assigned_to": null,
"status_id": 25,
"profile_pic": null,
"bio": null,
"cf": [],
"organization_id": 488,
"customFields": [],
"industry": null,
"isRegistered": false,
"icard": null,
"created_at": "2019-03-12 05:31:21+00",
"updated_at": "2019-03-12 11:35:31+00",
"_links": {
"image": {
"href": "/models/candidate-image/6506985"
},
"resume": {
"href": "/models/file?candidate_id=6506985&file_type_id=11"
},
"calendar": {
"href": "/models/candidate-calendar?candidate_id=6506985"
},
"assessmentCandidates": {
"href": "/models/assessment-candidate?candidate_id=6506985"
},
"candidateFileAttachments": {
"href": "/models/candidate-file-attachment?candidate_id=6506985"
}
}
}
...
]
This end point returns all the details of candidates
HTTP Request
GET https://apiv4.talview.com/models/candidate
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
false | Filter records based on email | ||
status_id | integer | false | Filter records based on status Id |
organization_id | integer | false | Filter based on organization Id |
Retrieve a Candidate
GET /models/candidate/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 6506985,
"email": "trideep.k+30@talview.com",
"first_name": "Trdp",
"middle_name": null,
"last_name": null,
"phone": "454545454545",
"external_id": null,
"assigned_to": null,
"status_id": 25,
"profile_pic": null,
"bio": null,
"cf": [],
"organization_id": 488,
"customFields": [],
"industry": null,
"isRegistered": false,
"icard": null,
"created_at": "2019-03-12 05:31:21+00",
"updated_at": "2019-03-12 11:35:31+00",
"_links": {
"image": {
"href": "/models/candidate-image/6506985"
},
"resume": {
"href": "/models/file?candidate_id=6506985&file_type_id=11"
},
"calendar": {
"href": "/models/candidate-calendar?candidate_id=6506985"
},
"assessmentCandidates": {
"href": "/models/assessment-candidate?candidate_id=6506985"
},
"candidateFileAttachments": {
"href": "/models/candidate-file-attachment?candidate_id=6506985"
}
}
}
Retrieves the details of an existing candidate.
HTTP Request
GET https://apiv4.talview.com/models/candidate/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create a Candidate
POST /models/candidate/ HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 6506985,
"email": "trideep.k+30@talview.com",
"first_name": "Trdp",
"middle_name": null,
"last_name": null,
"phone": "454545454545",
"external_id": null,
"assigned_to": null,
"status_id": 25,
"profile_pic": null,
"bio": null,
"cf": [],
"organization_id": 488,
"customFields": [],
"industry": null,
"isRegistered": false,
"icard": null,
"created_at": "2019-03-12 05:31:21+00",
"updated_at": "2019-03-12 11:35:31+00",
"_links": {
"image": {
"href": "/models/candidate-image/6506985"
},
"resume": {
"href": "/models/file?candidate_id=6506985&file_type_id=11"
},
"calendar": {
"href": "/models/candidate-calendar?candidate_id=6506985"
},
"assessmentCandidates": {
"href": "/models/assessment-candidate?candidate_id=6506985"
},
"candidateFileAttachments": {
"href": "/models/candidate-file-attachment?candidate_id=6506985"
}
}
}
This end point Creates a new candidate.
HTTP Request
POST https://apiv4.talview.com/models/candidate
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
text | true | The email of the candidate | |
first_name | text | false | The first name of the Candidate |
middle_name | text | false | The middle name of the Candidate |
last_name | text | false | The last name of the Candidate |
phone | text | false | The phone number of the Candidate |
Updates a Candidate
This endpoint Updates a Candidate.
PUT /models/candidate/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 6506985,
"email": "trideep.k+30@talview.com",
"first_name": "Trdp",
"middle_name": null,
"last_name": null,
"phone": "454545454545",
"external_id": null,
"assigned_to": null,
"status_id": 25,
"profile_pic": null,
"bio": null,
"cf": [],
"organization_id": 488,
"customFields": [],
"industry": null,
"isRegistered": false,
"icard": null,
"created_at": "2019-03-12 05:31:21+00",
"updated_at": "2019-03-12 11:35:31+00",
"_links": {
"image": {
"href": "/models/candidate-image/6506985"
},
"resume": {
"href": "/models/file?candidate_id=6506985&file_type_id=11"
},
"calendar": {
"href": "/models/candidate-calendar?candidate_id=6506985"
},
"assessmentCandidates": {
"href": "/models/assessment-candidate?candidate_id=6506985"
},
"candidateFileAttachments": {
"href": "/models/candidate-file-attachment?candidate_id=6506985"
}
}
}
HTTP Request
PUT https://apiv4.talview.com/models/candidate/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
text | false | The email of the candidate | |
first_name | text | false | The first name of the Candidate |
middle_name | text | false | The middle name of the Candidate |
last_name | text | false | The last name of the Candidate |
phone | text | false | The phone number of the Candidate |
profile_pic | string | false | Profile picture of the Candidate |
Delete a Candidate
DELETE /models/candidate/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This end point Deletes a Candidate.
HTTP Request
DELETE https://apiv4.talview.com/models/candidate/<id>
Candidate Calendar
The Candidate Calender endpoint gives the calendar details associated with a Candidate.
The Candidate Calendar Object
{
"id": 19403,
"from": "2016-09-29 17:00:00+05:30",
"candidate_id": 279942,
"to": "2016-09-29 18:00:00+05:30",
"event": "Live interview Interview with Talview",
"type": 1,
"created_at": "2019-02-06 14:57:30+05:30",
"created_by": 270471,
"updated_at": "2019-02-06 14:57:30+05:30",
"updated_by": 270471
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
from | timestamptz | The date and time from when the event starts |
candidate_id | integer | The Id of the Candidate |
to | timestamptz | The date and time till when the event is |
event | text | The details of the event |
type | smallint | The type of the event |
Retrieve a list of candidate calendar
GET /models/candidate-calendar HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 19403,
"from": "2016-09-29 17:00:00+05:30",
"candidate_id": 279942,
"to": "2016-09-29 18:00:00+05:30",
"event": "Live interview Interview with Talview",
"type": 1,
"created_at": "2019-02-06 14:57:30+05:30",
"created_by": 270471,
"updated_at": "2019-02-06 14:57:30+05:30",
"updated_by": 270471
},
{
"id": 19402,
"from": "2016-09-29 17:00:00+05:30",
"candidate_id": 279942,
"to": "2016-09-29 18:00:00+05:30",
"event": "Live interview Interview with Talview",
"type": 1,
"created_at": "2019-02-06 14:57:24+05:30",
"created_by": 270471,
"updated_at": "2019-02-06 14:57:24+05:30",
"updated_by": 270471
}
]
Retrieves the details of all the existing candidate calendar.
HTTP Request
GET https://apiv4.talview.com/models/candidate-calendar
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
type | integer | false | Filter records based on the type of event |
Retrieve a candidate calendar
GET /models/candidate-calendar/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 19403,
"from": "2016-09-29 17:00:00+05:30",
"candidate_id": 279942,
"to": "2016-09-29 18:00:00+05:30",
"event": "Live interview Interview with Talview",
"type": 1,
"created_at": "2019-02-06 14:57:30+05:30",
"created_by": 270471,
"updated_at": "2019-02-06 14:57:30+05:30",
"updated_by": 270471
}
Retrieves the details of an existing candidate calendar.
HTTP Request
GET https://apiv4.talview.com/models/candidate-calendar/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on the Id of the object |
Create a candidate calendar
POST /models/candidate-calendar HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 19403,
"from": "2016-09-29 17:00:00+05:30",
"candidate_id": 279942,
"to": "2016-09-29 18:00:00+05:30",
"event": "Live interview Interview with Talview",
"type": 1,
"created_at": "2019-02-06 14:57:30+05:30",
"created_by": 270471,
"updated_at": "2019-02-06 14:57:30+05:30",
"updated_by": 270471
}
Creates a candidate calendar.
HTTP Request
POST https://apiv4.talview.com/models/candidate-calendar
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
from | timestamptz | true | The date and time from when the event starts |
candidate_id | integer | true | The Id of the Candidate |
to | timestamptz | true | The date and time till when the event is |
type | smallint | true | The type of the event |
Update a candidate calendar
PUT /models/candidate-calendar/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
{
"id": 19403,
"from": "2016-09-29 17:00:00+05:30",
"candidate_id": 279942,
"to": "2016-09-29 18:00:00+05:30",
"event": "Live interview Interview with Talview",
"type": 1,
"created_at": "2019-02-06 14:57:30+05:30",
"created_by": 270471,
"updated_at": "2019-02-06 14:57:30+05:30",
"updated_by": 270471
}
Updates the details of an existing candidate calendar.
HTTP Request
PUT https://apiv4.talview.com/models/candidate-calendar/<id>
Query Parameters
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
from | timestamptz | false | The date and time from when the event starts |
candidate_id | integer | false | The Id of the Candidate |
to | timestamptz | false | The date and time till when the event is |
type | smallint | false | The type of the event |
Candidate Education
The Candidate Education end point gives the details of the Candidate Education.
The Candidate Education Object
[
{
"id": 31,
"candidate_id": 644252,
"title": null,
"type": null,
"field_of_study": null,
"grade": null,
"degree": null,
"school": "test",
"description": null,
"activities": null,
"started_at": null,_
"completed_at": null,
"created_at": "2018-12-26 15:59:04+05:30",
"created_by": 67566,
"updated_at": "2018-12-26 15:59:04+05:30",
"updated_by": 67566
}
]
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
candidate_id | integer | The Id of the Candidate |
title | text | The title of the Education. Example: Master In Computer Applications |
type | text | The type of Education. Example: Regular, Distance. |
field_of_study | text | The field of study. Example: Specialised in Cloud Technology |
grade | text | The grade Obtained by the Candidate on the particular degree |
degree | text | The degree of the candidate. Example: BTech, MCA |
school | text | The Name of the School |
description | text | The description of the Education |
activities | text | The extra curricular activities during education period |
started_at | timestamptz | The date when the Candidate started the mentioned degree |
completed_at | timestamptz | The date when the Candidate completed the mentioned degree |
created_by | integer | The id of who created candidate education |
updated_by | integer | The id of who updated candidate education |
List all Candidate Education
GET /models/candidate-education HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 31,
"candidate_id": 644252,
"title": null,
"type": null,
"field_of_study": null,
"grade": null,
"degree": null,
"school": "test",
"description": null,
"activities": null,
"started_at": null,
"completed_at": null,
"created_at": "2018-12-26 15:59:04+05:30",
"created_by": 67566,
"updated_at": "2018-12-26 15:59:04+05:30",
"updated_by": 67566
}
...
]
Returns all the candidate education details.
HTTP Request
GET https://apiv4.talview.com/models/candidate-education
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
candidate_id | integer | false | Filter records based on the Id of the Candidate |
title | text | false | Filter records based on the title of the Candidate Education |
type | text | false | Filter records based on the type of the Candidate Education |
field_of_study | text | false | Filter records based on the Field of Study of the Candidate Education |
grade | text | false | Filter records based on the Grade of the Candidate Education |
degree | text | flase | Filter records based on the degree of the candidate. Example: BTech, MCA |
school | text | false | Filter records based on the name of the School |
Retrieves a Candidate Education
GET /models/candidate-education/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
{
"id": 31,
"candidate_id": 644252,
"title": null,
"type": null,
"field_of_study": null,
"grade": null,
"degree": null,
"school": "test",
"description": null,
"activities": null,
"started_at": null,
"completed_at": null,
"created_at": "2018-12-26 15:59:04+05:30",
"created_by": 67566,
"updated_at": "2018-12-26 15:59:04+05:30",
"updated_by": 67566
}
This end point retrieves a candidate's education details.
HTTP Request
GET https://apiv4.talview.com/models/candidate-education/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create a Candidate Education
POST /models/candidate-education HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 31,
"candidate_id": 644252,
"title": null,
"type": null,
"field_of_study": null,
"grade": null,
"degree": null,
"school": "test",
"description": null,
"activities": null,
"started_at": null,
"completed_at": null,
"created_at": "2018-12-26 15:59:04+05:30",
"created_by": 67566,
"updated_at": "2018-12-26 15:59:04+05:30",
"updated_by": 67566
}
]
This end point creates a candidate's education details.
HTTP Request
POST https://apiv4.talview.com/models/candidate-education
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
candidate_id | integer | true | The Id of the Candidate |
title | text | false | The title of the degree. Example: Integrated Master in Computer Application |
type | text | false | The type of education. Example: Regular, Distance etc |
field_of_study | text | false | Any specialization in education. Example: Specialized in Cloud Technology |
degree | text | false | The degree of the candidate. Example: BTech, MCA |
school | text | true | The Name of the School |
description | text | false | The description of the Education |
activities | text | false | The extra curricular activities during education period |
completed_at | timestamptz | false | The date when the Candidate completed the mentioned degree |
started_at | timestamptz | false | The date when the Candidate started the mentioned degree |
Update a Candidate Education
PUT /models/candidate-education/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 31,
"candidate_id": 644252,
"title": null,
"type": null,
"field_of_study": null,
"grade": null,
"degree": null,
"school": "test",
"description": null,
"activities": null,
"started_at": null,
"completed_at": null,
"created_at": "2018-12-26 15:59:04+05:30",
"created_by": 67566,
"updated_at": "2018-12-26 15:59:04+05:30",
"updated_by": 67566
}
]
This end point updates a candidate's education details.
HTTP Request
PUT https://apiv4.talview.com/models/candidate-education/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
title | text | false | The title of the Education. Example: Master In Computer Applications |
type | text | false | The type of Education. Example: Regular, Distance. |
field_of_study | text | false | The field of study. Example: Specialised in Cloud Technology |
degree | text | false | The degree of the candidate. Example: BTech, MCA |
school | text | false | The Name of the School |
description | text | false | The description of the Education |
activities | text | false | The extra curricular activities during education period |
completed_at | timestamptz | false | The date when the Candidate completed the mentioned degree |
started_at | timestamptz | false | The date when the Candidate started the mentioned degree |
Candidate Experience
The Candidate Experience endpoint provides the details related to the experience of the Candidates present in the Candidate model.
The Candidate Experience Object
{
"id": 19,
"is_current": true,
"candidate_id": 644252,
"organization": "test",
"designation": null,
"title": "C",
"location": null,
"started_at": "2018-12-18",
"completed_at": null,
"description": null,
"created_at": "2018-12-26 16:12:40+05:30",
"created_by": 67566,
"updated_at": "2018-12-26 16:12:40+05:30",
"updated_by": 67566
},
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
is_current | boolean | If true, The Candidate is currently working on some organization |
candidate_id | integer | The id of the Candidate |
organization | text | The name of the organization where the Candidate is/was working |
designation | text | The current designation of the Candidate |
title | text | The title of the Experience |
location | text | The location of the organization where the Candidate is/was working |
started_at | timestamptz | The date when the Candidate started working |
completed_at | timestamptz | The date when the Candidate left the organization |
description | text | The description of the Experience |
List all Candidate Experience
GET /models/candidate-experience HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 53,
"is_current": false,
"candidate_id": 1914994,
"organization": "TalView",
"designation": null,
"title": "UI Designer",
"location": "Banglore",
"started_at": "2017-01-13",
"completed_at": null,
"description": "Test descriptio",
"created_at": "2019-01-11 14:15:51+05:30",
"created_by": 67566,
"updated_at": "2019-01-11 14:21:51+05:30",
"updated_by": 67566
}
...
]
This end point Returns all the details of candidate-experience.
HTTP Request
GET https://apiv4.talview.com/models/candidate-experience
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
candidate_id | integer | false | Filter records based on the Id of the Candidate |
organization | text | false | Filter records based on organization |
designation | text | false | Filter records based on designation |
title | text | false | Filter records based on title |
location | text | false | Filter records based on location |
started_at | timestamptz | false | Filter records based on the time when the Candidate started working |
completed_at | timestamptz | false | Filter records based on the time when the Candidate left working |
Retrieve a Candidate Experience
GET /models/candidate-experience/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 53,
"is_current": false,
"candidate_id": 1914994,
"organization": "TalView",
"designation": null,
"title": "UI Designer",
"location": "Banglore",
"started_at": "2017-01-13",
"completed_at": null,
"description": "Test descriptio",
"created_at": "2019-01-11 14:15:51+05:30",
"created_by": 67566,
"updated_at": "2019-01-11 14:21:51+05:30",
"updated_by": 67566
}
This end point Retrieves a candidate experience.
HTTP Request
GET https://apiv4.talview.com/models/candidate-experience/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on id |
Create a Candidate Experience
POST /models/candidate-experience HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 53,
"is_current": false,
"candidate_id": 1914994,
"organization": "TalView",
"designation": null,
"title": "UI Designer",
"location": "Banglore",
"started_at": "2017-01-13",
"completed_at": null,
"description": "Test descriptio",
"created_at": "2019-01-11 14:15:51+05:30",
"created_by": 67566,
"updated_at": "2019-01-11 14:21:51+05:30",
"updated_by": 67566
}
This end point Creates a candidate experience.
HTTP Request
POST https://apiv4.talview.com/models/candidate-experience
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
candidate_id | integer | true | The id of the Candidate |
organization | text | true | The name of the organization where the Candidate is/was working |
designation | text | false | The current designation of the Candidate |
title | text | true | The title of the Experience |
is_current | boolean | false | If true, The Candidate is currently working on some organization |
location | text | false | The location of the organization where he is/was working |
started_at | timestamptz | true | The date when the Candidate started working |
completed_at | timestamptz | false | The date when the Candidate left the organization |
description | text | false | The description of the Experience |
Update a Candidate Experience
PUT /models/candidate-experience/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 53,
"is_current": false,
"candidate_id": 1914994,
"organization": "TalView",
"designation": null,
"title": "UI Designer",
"location": "Banglore",
"started_at": "2017-01-13",
"completed_at": null,
"description": "Test descriptio",
"created_at": "2019-01-11 14:15:51+05:30",
"created_by": 67566,
"updated_at": "2019-01-11 14:21:51+05:30",
"updated_by": 67566
}
This end point Updates a candidate experience.
HTTP Request
PUT https://apiv4.talview.com/models/candidate-experience/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
BODY Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
organization | text | false | The name of the organization where the Candidate is/was working |
designation | text | false | The current designation of the Candidate |
title | text | false | The title of the Experience |
is_current | boolean | false | If true, The Candidate is currently working on some organization |
location | text | false | The location of the organization where he is/was working |
started_at | timestamptz | false | The date when the Candidate started working |
completed_at | timestamptz | false | The date when the Candidate left the organization |
description | text | false | The description of the Experience |
Delete a Candidate experience
DELETE /models/candidate-experience/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns a status: 204 No Content
This end point Deletes a Candidate experience.
HTTP Request
DELETE https://apiv4.talview.com/models/candidate-experience/<id>
Candidate File Attachment
The Candidate File Attachment endpoint is used to handle the Files (Example: Resume, Photo) associated with the Candidates present in the Candidate model.
The Candidate File Attachment Object
{
"id": 2393441,
"candidate_id": 6507897,
"file_id": 13962889,
"created_at": "2019-03-18 13:43:19+00",
"updated_at": "2019-03-18 13:43:19+00",
"_links": {
"candidate": {
"href": "/models/candidate/6507897"
},
"file": {
"href": "/models/file/13962889"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
candidate_id | integer | The id of the Candidate |
file_id | integer | The id of the file attached |
List all Candidate File Attachment
GET /models/candidate-file-attachment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 2393441,
"candidate_id": 6507897,
"file_id": 13962889,
"created_at": "2019-03-18 13:43:19+00",
"updated_at": "2019-03-18 13:43:19+00",
"_links": {
"candidate": {
"href": "/models/candidate/6507897"
},
"file": {
"href": "/models/file/13962889"
}
}
},
{
"id": 2393688,
"candidate_id": 6534799,
"file_id": 13967144,
"created_at": "2019-03-19 10:35:47+00",
"updated_at": "2019-03-19 10:35:47+00",
"_links": {
"candidate": {
"href": "/models/candidate/6534799"
},
"file": {
"href": "/models/file/13967144"
}
}
}
]
This end point returns all the files related to a Candidate.
HTTP Request
GET https://apiv4.talview.com/models/candidate-file-attachment
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
candidate_id | integer | false | Filter records based on Candidate Id |
file_id | integer | false | Filter records based on File Id |
Retrieve a Candidate File Attachment
GET /models/candidate-file-attachment/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 2393441,
"candidate_id": 6507897,
"file_id": 13962889,
"created_at": "2019-03-18 13:43:19+00",
"updated_at": "2019-03-18 13:43:19+00",
"_links": {
"candidate": {
"href": "/models/candidate/6507897"
},
"file": {
"href": "/models/file/13962889"
}
}
}
This end point Retrieves all the files related to a Candidate.
HTTP Request
GET https://apiv4.talview.com/models/candidate-file-attachment/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Candidate Id |
Create a Candidate File Attachment
POST /models/candidate-file-attachment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 2393441,
"candidate_id": 6507897,
"file_id": 13962889,
"created_at": "2019-03-18 13:43:19+00",
"updated_at": "2019-03-18 13:43:19+00",
"_links": {
"candidate": {
"href": "/models/candidate/6507897"
},
"file": {
"href": "/models/file/13962889"
}
}
}
This end point Create a file attachment for a candidate.
HTTP Request
POST https://apiv4.talview.com/models/candidate-file-attachment
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
candidate_id | integer | true | The Id of the candidate |
file_id | integer | true | The Id of the file |
Candidate Image
This Candidate image endpoint gives the Image details of the Candidate
The Candidate Image object
{
"id": 6666685,
"profile_pic": null,
"created_at": "2019-03-20 08:40:34+00",
"updated_at": "2019-03-20 08:46:20+00",
"_links": {
"image": {
"href": "/models/candidate-image/6666685"
},
"resume": {
"href": "/models/file?candidate_id=6666685&file_type_id=11"
},
"calendar": {
"href": "/models/candidate-calendar?candidate_id=6666685"
},
"assessmentCandidates": {
"href": "/models/assessment-candidate?candidate_id=6666685"
},
"candidateFileAttachments": {
"href": "/models/candidate-file-attachment?candidate_id=6666685"
}
}
}
Parameter | type | Description |
---|---|---|
id | integer | The unique identifier of the object |
profile_pic | text | The url of the image |
List all Candidate Image
GET /models/candidate-image HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 6666962,
"profile_pic": null,
"created_at": "2019-03-20 09:09:53+00",
"updated_at": "2019-03-20 09:11:44+00",
"_links": {
"image": {
"href": "/models/candidate-image/6666962"
},
"resume": {
"href": "/models/file?candidate_id=6666962&file_type_id=11"
},
"calendar": {
"href": "/models/candidate-calendar?candidate_id=6666962"
},
"assessmentCandidates": {
"href": "/models/assessment-candidate?candidate_id=6666962"
},
"candidateFileAttachments": {
"href": "/models/candidate-file-attachment?candidate_id=6666962"
}
}
},
{
"id": 6666685,
"profile_pic": null,
"created_at": "2019-03-20 08:40:34+00",
"updated_at": "2019-03-20 08:46:20+00",
"_links": {
"image": {
"href": "/models/candidate-image/6666685"
},
"resume": {
"href": "/models/file?candidate_id=6666685&file_type_id=11"
},
"calendar": {
"href": "/models/candidate-calendar?candidate_id=6666685"
},
"assessmentCandidates": {
"href": "/models/assessment-candidate?candidate_id=6666685"
},
"candidateFileAttachments": {
"href": "/models/candidate-file-attachment?candidate_id=6666685"
}
}
}
]
This end point returns all the candidate images.
HTTP Request
GET https://apiv4.talview.com/models/candidate-image
Query parameters
parameter | type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
Retrieve a Candidate Image
GET /models/candidate-image/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
{
"id": 6666685,
"profile_pic": null,
"created_at": "2019-03-20 08:40:34+00",
"updated_at": "2019-03-20 08:46:20+00",
"_links": {
"image": {
"href": "/models/candidate-image/6666685"
},
"resume": {
"href": "/models/file?candidate_id=6666685&file_type_id=11"
},
"calendar": {
"href": "/models/candidate-calendar?candidate_id=6666685"
},
"assessmentCandidates": {
"href": "/models/assessment-candidate?candidate_id=6666685"
},
"candidateFileAttachments": {
"href": "/models/candidate-file-attachment?candidate_id=6666685"
}
}
}
Retrieve a candidate image.
HTTP Request
GET https://apiv4.talview.com/models/candidate-image/<id>
URL parameters
parameter | type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on Id |
candidate_id | integer | false | Filter records based on Candidate ID |
Category
The category endpoint gives the details of the categorized Assessment.
The Category Object
{
"id": 513,
"name": "Domain",
"type": 100,
"created_at": "2017-11-08 02:51:00+00",
"updated_at": null,
"created_by": 1,
"updated_by": 1,
"_links": {
"self": {
"href": "https://apiv4.talview.com/models/category/513?oid=484"
},
"index": {
"href": "https://apiv4.talview.com/models/category?oid=484"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
name | text | The name of the Category |
type | integer | The type of the Category. Example: Business, Section. The type of Categories are described in the enumerator list below |
created_by | integer | The id of the User who created the Category |
updated_by | integer | The id of the User who updated the category |
List of Category types:
Event Types | Key | Description |
---|---|---|
Bussiness | 1 | Business type Category |
Section | 2 | Section type Category |
Skill | 3 | Skill type Category |
Help | 10 | Help type Category |
Parameter | 100 | Parameter type Category |
List all Category
GET /models/category HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 513,
"name": "Domain",
"type": 100,
"created_at": "2017-11-08 02:51:00+00",
"updated_at": null,
"created_by": 1,
"updated_by": 1,
"_links": {
"self": {
"href": "https://apiv4.talview.com/models/category/513?oid=484"
},
"index": {
"href": "https://apiv4.talview.com/models/category?oid=484"
}
}
},
{
"id": 512,
"name": "Behavioural",
"type": 100,
"created_at": "2017-11-08 02:51:00+00",
"updated_at": null,
"created_by": 1,
"updated_by": 1,
"_links": {
"self": {
"href": "https://apiv4.talview.com/models/category/512?oid=484"
},
"index": {
"href": "https://apiv4.talview.com/models/category?oid=484"
}
}
}
...
]
This endpoint returns all the details of categories.
HTTP Request
GET https://apiv4.talview.com/models/category
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
name | text | false | Filter records based on Category name |
type | integer | false | Filter records based on type of the Category |
Retrieve a Category
GET /models/category/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 897,
"answer_id": 4441883,
"source": "/*\n#include <iostream>\nusing namespace std;\nint main()\n{\n cout << \"Hello World!\" << endl;\n return 0;\n}\n*/\n//Write your code here",
"score": null,
"status": 0,
"input": null,
"memory": 0,
"language": "1",
"language_id": "1",
"compiler": null,
"cmpinfo": "/usr/lib/gcc/x86_64-linux-gnu/6/../../../x86_64-linux-gnu/Scrt1.o: In function `_start':\n(.text+0x20): undefined reference to `main'\ncollect2: error: ld returned 1 exit status\n",
"stderr": null,
"time": 0,
"output": null,
"result": "/usr/lib/gcc/x86_64-linux-gnu/6/../../../x86_64-linux-gnu/Scrt1.o: In function `_start':\n(.text+0x20): undefined reference to `main'\ncollect2: error: ld returned 1 exit status\n"
}
This endpoint retrieves a category based on the id passed
HTTP Request
GET https://apiv4.talview.com/models/category/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Code Language
The Code Language endpoint gives the details of available Coding Languages along with the Id.
{
"7": "Ada (gnat 5.1.1)",
"45": "Assembler (gcc 4.9.3)",
"13": "Assembler (NASM 2.11.05)",
"42": "Assembler 64bit (nasm 2.12.01)",
"105": "AWK (mawk 1.3.3)",
"104": "AWK (gawk) (fawk 4.1.1)",
"28": "Bash (bash 4.3.33)",
"110": "bc (bc 1.06.95)",
"12": "Brainf**k (1.0.6)",
"11": "C (gcc 5.1.1)",
"27": "C# (Mono 4.0.2)",
"1": "C++ (5.1.1)",
"41": "C++ 4.3.2 (gcc-4.3.2)",
"44": "C++14 (gcc-5 5.1.1)",
"34": "C99 strict (gcc-5 5.1.1)",
"14": "CLIPS (clips 6.24)",
"111": "Clojure (clojure 1.7.0)",
"118": "COBOL (1.1.0)",
"106": "COBOL 85 (tinycobol-0.65.9)",
"31": "Common Lisp (sbcl 1.3.13)",
"32": "Common Lisp (clisp) (clisk 2.49)",
"102": "D (dmd 2.072.2)",
"20": "D (gdc-5 5.1.1)",
"36": "Erlang (erl 18)",
"124": "F# (1.3)",
"107": "Forth (gforth 0.7.2)",
"5": "Fortran (5.1.1)",
"114": "Go (1.4)",
"98": "Gosu (gosu 1.6.1)",
"121": "Groovy (2.4)",
"21": "Haskell (ghc 7.8)",
"16": "Icon (icon 9.4.3)",
"9": "Intercal (c-intercal 28.0-r1)",
"10": "Java (jdk 8u51)",
"55": "Java7 (sun-jdk-1.7.0_10)",
"35": "JavaScript (rhino) (rhino 1.7.7)",
"112": "JavaScript (spidermonkey) (24.2.0)",
"47": "Kotlin (kotlin 1.0.6)",
"26": "Lua (lua 7.2)",
"30": "Nemerle (ncc 1.2.0)",
"25": "Nice (0.9.13)",
"56": "Node.js (node 7.4.0)",
"43": "Objective-C (gcc-5 5.1.1)",
"8": "Ocaml (4.01.0)",
"127": "Octave (4.0.0)",
"22": "Pascal (fpc) (fpc 2.6.4+dfsg-6)",
"2": "Pascal (gpc) (gpc 20070904)",
"3": "Perl (perl 5.20.1)",
"54": "Perl 6 (perl6 2014.07)",
"29": "PHP (PHP 5.6.11-1)",
"19": "Pike (pike v7.8)",
"108": "Prolog (gnu prolog 1.4.5)",
"15": "Prolog (swi) (swi 7.2)",
"4": "Python (2.7.13)",
"99": "Python (Pypy) (PyPy 2.6.0)",
"116": "Python 3 (3.5.3)",
"117": "R (3.2.2)",
"17": "Ruby (ruby 2.1.5)",
"93": "Rust (1.14.0)",
"39": "Scala (2.11.7)",
"18": "Scheme (stalin 0.3)",
"97": "Scheme (chicken 4.11.0)",
"33": "Scheme (guile) (guile 2.0.11)",
"46": "Sed (sed 4.2.2)",
"23": "Smalltalk (gst 3.2.4)",
"40": "SQL (sqlite3-3.8.7)",
"85": "Swift (swift 3.0.2)",
"38": "Tcl (tclsh 8.6)",
"50": "VB.NET (mono 4.0.2)",
"6": "Whitespace (wspace 0.3)"
}
List all Code Language
GET /models/code-language HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"7": "Ada (gnat 5.1.1)",
"45": "Assembler (gcc 4.9.3)",
"13": "Assembler (NASM 2.11.05)",
"42": "Assembler 64bit (nasm 2.12.01)",
"105": "AWK (mawk 1.3.3)",
"104": "AWK (gawk) (fawk 4.1.1)",
"28": "Bash (bash 4.3.33)",
"110": "bc (bc 1.06.95)",
"12": "Brainf**k (1.0.6)",
"11": "C (gcc 5.1.1)",
"27": "C# (Mono 4.0.2)",
"1": "C++ (5.1.1)",
"41": "C++ 4.3.2 (gcc-4.3.2)",
"44": "C++14 (gcc-5 5.1.1)",
"34": "C99 strict (gcc-5 5.1.1)",
"14": "CLIPS (clips 6.24)",
"111": "Clojure (clojure 1.7.0)",
"118": "COBOL (1.1.0)",
"106": "COBOL 85 (tinycobol-0.65.9)",
"31": "Common Lisp (sbcl 1.3.13)",
"32": "Common Lisp (clisp) (clisk 2.49)",
"102": "D (dmd 2.072.2)",
"20": "D (gdc-5 5.1.1)",
"36": "Erlang (erl 18)",
"124": "F# (1.3)",
"107": "Forth (gforth 0.7.2)",
"5": "Fortran (5.1.1)",
"114": "Go (1.4)",
"98": "Gosu (gosu 1.6.1)",
"121": "Groovy (2.4)",
"21": "Haskell (ghc 7.8)",
"16": "Icon (icon 9.4.3)",
"9": "Intercal (c-intercal 28.0-r1)",
"10": "Java (jdk 8u51)",
"55": "Java7 (sun-jdk-1.7.0_10)",
"35": "JavaScript (rhino) (rhino 1.7.7)",
"112": "JavaScript (spidermonkey) (24.2.0)",
"47": "Kotlin (kotlin 1.0.6)",
"26": "Lua (lua 7.2)",
"30": "Nemerle (ncc 1.2.0)",
"25": "Nice (0.9.13)",
"56": "Node.js (node 7.4.0)",
"43": "Objective-C (gcc-5 5.1.1)",
"8": "Ocaml (4.01.0)",
"127": "Octave (4.0.0)",
"22": "Pascal (fpc) (fpc 2.6.4+dfsg-6)",
"2": "Pascal (gpc) (gpc 20070904)",
"3": "Perl (perl 5.20.1)",
"54": "Perl 6 (perl6 2014.07)",
"29": "PHP (PHP 5.6.11-1)",
"19": "Pike (pike v7.8)",
"108": "Prolog (gnu prolog 1.4.5)",
"15": "Prolog (swi) (swi 7.2)",
"4": "Python (2.7.13)",
"99": "Python (Pypy) (PyPy 2.6.0)",
"116": "Python 3 (3.5.3)",
"117": "R (3.2.2)",
"17": "Ruby (ruby 2.1.5)",
"93": "Rust (1.14.0)",
"39": "Scala (2.11.7)",
"18": "Scheme (stalin 0.3)",
"97": "Scheme (chicken 4.11.0)",
"33": "Scheme (guile) (guile 2.0.11)",
"46": "Sed (sed 4.2.2)",
"23": "Smalltalk (gst 3.2.4)",
"40": "SQL (sqlite3-3.8.7)",
"85": "Swift (swift 3.0.2)",
"38": "Tcl (tclsh 8.6)",
"50": "VB.NET (mono 4.0.2)",
"6": "Whitespace (wspace 0.3)"
}
This end point returns list of all code languages.
HTTP Request
GET https://apiv4.talview.com/models/code-language
Retrieve a Code Language
GET /models/code-language/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": "2",
"name": "Pascal (gpc) (gpc 20070904)"
}
Retrieve a code language.
HTTP Request
GET https://apiv4.talview.com/models/code-language/<id>
Query parameters
parameter | type | Mandatory | Description |
---|---|---|---|
id | integer | true | The Id of the Code Language |
Custom Field Master
An organization's custom fields.
The Custom Field Master Object
[
{
"id": 2,
"type": 1,
"sort_order": 2,
"is_report": false,
"label": "Requisition ID",
"key": "RequisitionID",
"updated_at": "2017-11-10 07:52:37+00",
"created_at": "2017-11-10 07:52:37+00"
},
{
"id": 9,
"type": 1,
"sort_order": 9,
"is_report": false,
"label": "Recruiter Assistant",
"key": "RecruiterAssistant",
"updated_at": "2017-11-10 07:52:37+00",
"created_at": "2017-11-10 07:52:37+00"
}
]
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
type | smallint | Describes whether candidate or assessment type |
sort_order | smallint | The sort order value for custom field master |
is_report | boolean | Whether the report is available |
label | string | The label for the custom field |
key | varchar | Key for the upstream system |
updated_at | timestamptz | The time when custom field master was updated |
created_at | timestamptz | The time when custom field master was created |
List all Custom Field Master
GET /models/custom-field-master HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
[
{
"id": 9,
"type": 1,
"sort_order": 9,
"is_report": false,
"label": "Recruiter Assistant",
"key": "RecruiterAssistant",
"updated_at": "2017-11-10 07:52:37+00",
"created_at": "2017-11-10 07:52:37+00"
}
]
Returns all the details of custom field master.
HTTP Request
GET https://apiv4.talview.com/models/custom-field-master
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter based on id |
type | smallint | false | Filter based on type |
label | varchar | false | Filter based on label |
key | varchar | false | Filter based on key |
Retrieve a Custom Field Master
GET /models/custom-field-master/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
[
{
"id": 9,
"type": 1,
"sort_order": 9,
"is_report": false,
"label": "Recruiter Assistant",
"key": "RecruiterAssistant",
"updated_at": "2017-11-10 07:52:37+00",
"created_at": "2017-11-10 07:52:37+00"
}
]
Retrieve a custom field master.
HTTP Request
GET https://apiv4.talview.com/models/custom-field-master/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter based on id |
Create a Custom Field Master
POST /models/custom-field-master HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Body :
[
{
"id": 9,
"type": 1,
"sort_order": 9,
"is_report": false,
"label": "Recruiter Assistant",
"key": "RecruiterAssistant",
"updated_at": "2017-11-10 07:52:37+00",
"created_at": "2017-11-10 07:52:37+00"
}
]
The above command returns : 201 Created
Adds a custom field master.
HTTP Request
POST https://apiv4.talview.com/models/custom-field-master
Query Parameters
Parameter | Type | isOptional | Description |
---|---|---|---|
type | integer | false | Describes whether candidate or assessment type |
label | text | false | The label of the custom field |
key | text | false | Key for the upstream system |
Update a Custom Field Master
PUT /models/custom-field-master/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns :
[
{
"id": 9,
"type": 1,
"sort_order": 9,
"is_report": false,
"label": "Recruiter Assistant",
"key": "RecruiterAssistant",
"updated_at": "2017-11-10 07:52:37+00",
"created_at": "2017-11-10 07:52:37+00"
}
]
Updates a custom field master.
HTTP Request
PUT https://apiv4.talview.com/models/custom-field-master/id
URL Parameters
Parameter | Type | is Optional | Description |
---|---|---|---|
id | integer | false | The unique identifier of the object |
Delete a Custom Field Master
DELETE /models/custom-field-master/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns : 204 No Content
Delete a custom field master.
HTTP Request
DELETE https://apiv4.talview.com/models/custom-field-master/<id>
Display Picture
The Display picture endpoint is used to view, create and delete the profile picture of a User.
The Display Picture Object
{
"location": "",
"bio": "",
"website": "",
"picture": {
"id": 2265,
"name": "ch_oo_pp",
"external_id": null,
"description": null,
"pdfUrl": null,
"file_type_id": 44,
"size": null,
"created_at": "2019-12-21 07:00:14+00",
"updated_at": "2019-12-21 07:00:14+00",
"resourceUrl": "https://assets.talview.com/test/mani/user/profile/ch_oo_pp.png",
"extension": "png",
"originalName": "ch oo pp .png",
"_links": {
"fileType": {
"href": "/models/file-type/44"
}
}
},
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2019-12-21T07:00:14+00:00"
}
Parameter | Type | Description |
---|---|---|
location | string | The location of the user |
bio | string | The bio of the user |
website | string | The website of the user |
picture | object | The file object of the user |
created_at | timestamp | The timestamp when the profile was created |
updated_at | timestamp | The timestamp when the profile was updated |
Allowed Extensions
['image/png','image/jpeg','image/bmp']
Retrieve a profile
GET api/user/display-picture HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"location": "",
"bio": "",
"website": "",
"picture": {
"id": 2265,
"name": "ch_oo_pp",
"external_id": null,
"description": null,
"pdfUrl": null,
"file_type_id": 44,
"size": null,
"created_at": "2019-12-21 07:00:14+00",
"updated_at": "2019-12-21 07:00:14+00",
"resourceUrl": "https://assets.talview.com/test/mani/user/profile/ch_oo_pp.png",
"extension": "png",
"originalName": "ch oo pp .png",
"_links": {
"fileType": {
"href": "/models/file-type/44"
}
}
},
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2019-12-21T07:00:14+00:00"
}
This endpoint returns all users of an organization.
HTTP Request
GET https://apiv4.talview.com/api/user/display-picture
Create a profile
POST api/user/display-picture HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"location": "",
"bio": "",
"website": "",
"picture": {
"id": 2265,
"name": "ch_oo_pp",
"external_id": null,
"description": null,
"pdfUrl": null,
"file_type_id": 44,
"size": null,
"created_at": "2019-12-21 07:00:14+00",
"updated_at": "2019-12-21 07:00:14+00",
"resourceUrl": "https://assets.talview.com/test/mani/user/profile/ch_oo_pp.png",
"extension": "png",
"originalName": "ch oo pp .png",
"_links": {
"fileType": {
"href": "/models/file-type/44"
}
}
},
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2019-12-21T07:00:14+00:00"
}
HTTP Request
POST https://apiv4.talview.com/api/user/display-picture
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
file | multipart | true | The file to be set as profile picture for the user |
Delete a profile
DELETE api/user/display-picture HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns 204 status code
HTTP Request
DELETE https://apiv4.talview.com/api/user/display-picture
Evaluation
The Evaluation endpoint gives evaluation result of a Candidate evaluated by the Panel for an Assessment Section.
The Evaluation Object
{
"id": 87214,
"panel_id": 22244,
"assessment_candidate_id": 346721,
"assessment_section_id": 988862,
"form_instance_id": null,
"assessment_section_candidate_id": 396163,
"recommendation": "hold",
"evaluated_at": "2018-04-18 11:00:34+00",
"evaluated_by": 66084,
"created_at": "2019-01-18 17:26:04+05:30",
"updated_at": "2019-01-18 17:26:04+05:30",
"_links": {
"evaluationComments": {
"href": "/models/evaluation-comment?evaluation_id=87214"
},
"evaluationRatings": {
"href": "/models/evaluation-rating?evaluation_id=87214"
},
"formInstance": {
"href": "/models/form-instance?evaluation_id=87214"
},
"panelCandidate": {
"href": "/models/panel-candidate?id=494897"
}
}
}
Parameter | Type | Description |
---|---|---|
panel_id | Integer | The Id of the Panel who is/are evaluating the Assessment Section |
assessment_candidate_id | Integer | The id of Assessment Candidate |
assessment_section_id | Integer | The id of Assessment Section |
form_instance_id | integer | The id of the Form[#forms] instance |
assessment_section_candidate_id | Integer | The id of Assessment Section Candidate |
recommendation | text | The recommendation given by the panel. The recommendation are Select, Reject and Hold. |
evaluated_at | timestamptz | The date and time of Evaluation |
evaluated_by | integer | The id of the Panel who evaluated the Assessment Section Candidate |
List all Evaluation
GET /models/evaluation HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 87214,
"panel_id": 22244,
"assessment_candidate_id": 346721,
"assessment_section_id": 988862,
"assessment_section_candidate_id": 396163,
"recommendation": "hold",
"evaluated_at": "2018-04-18 11:00:34+00",
"evaluated_by": 66084,
"_links": {
"evaluationComments": {
"href": "/models/evaluation-comment?evaluation_id=87214"
},
"evaluationRatings": {
"href": "/models/evaluation-rating?evaluation_id=87214"
},
"formInstance": {
"href": "/models/form-instance?evaluation_id=87214"
},
"panelCandidate": {
"href": "/models/panel-candidate?id=494897"
}
}
}
...
]
This end point returns all the details of evaluations
HTTP Request
GET https://apiv4.talview.com/models/evaluation
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
panel_id | Integer | false | Filter records based on Panel Id |
assessment_candidate_id | Integer | false | Filter records based on the Id of Assessment Candidate |
assessment_section_id | Integer | false | Filter records based on the Id of Assessment Section |
assessment_section_candidate_id | Integer | false | Filter records based on the Id of Assessment Section Candidate |
recommendation | text | false | Filter records based on recommendation |
evaluated_by | integer | false | Filter records based on the Panel who evaluated the Assessment Section Candidate |
Retrieve an Evaluation
GET /models/evaluation/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 87214,
"panel_id": 22244,
"assessment_candidate_id": 346721,
"assessment_section_id": 988862,
"assessment_section_candidate_id": 396163,
"recommendation": "hold",
"evaluated_at": "2018-04-18 11:00:34+00",
"evaluated_by": 66084,
"_links": {
"evaluationComments": {
"href": "/models/evaluation-comment?evaluation_id=87214"
},
"evaluationRatings": {
"href": "/models/evaluation-rating?evaluation_id=87214"
},
"formInstance": {
"href": "/models/form-instance?evaluation_id=87214"
},
"panelCandidate": {
"href": "/models/panel-candidate?id=494897"
}
}
}
This end point retrieves an evaluation based on id
HTTP Request
GET https://apiv4.talview.com/models/evaluation/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | Integer | true | Filter records based on Id |
Create a Evaluation
POST /models/evaluation HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 87214,
"panel_id": 22244,
"assessment_candidate_id": 346721,
"assessment_section_id": 988862,
"assessment_section_candidate_id": 396163,
"recommendation": "hold",
"evaluated_at": "2018-04-18 11:00:34+00",
"evaluated_by": 66084,
"_links": {
"evaluationComments": {
"href": "/models/evaluation-comment?evaluation_id=87214"
},
"evaluationRatings": {
"href": "/models/evaluation-rating?evaluation_id=87214"
},
"formInstance": {
"href": "/models/form-instance?evaluation_id=87214"
},
"panelCandidate": {
"href": "/models/panel-candidate?id=494897"
}
}
}
This end point creates a new evaluation
HTTP Request
POST https://apiv4.talview.com/models/evaluation
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessment_candidate_id | integer | true | The id of the assessment candidate |
panel_id | Integer | false | The Id of the Panel who is/are evaluating the Assessment Section |
assessment_section_id | integer | true | The id of the assessment section |
recommendation | text | true | The recommendation of the evaluation |
assessment_section_candidate_id | Integer | false | The id of Assessment Section Candidate |
Update a Evaluation
PUT /models/evaluation<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 87214,
"panel_id": 22244,
"assessment_candidate_id": 346721,
"assessment_section_id": 988862,
"assessment_section_candidate_id": 396163,
"recommendation": "hold",
"evaluated_at": "2018-04-18 11:00:34+00",
"evaluated_by": 66084,
"_links": {
"evaluationComments": {
"href": "/models/evaluation-comment?evaluation_id=87214"
},
"evaluationRatings": {
"href": "/models/evaluation-rating?evaluation_id=87214"
},
"formInstance": {
"href": "/models/form-instance?evaluation_id=87214"
},
"panelCandidate": {
"href": "/models/panel-candidate?id=494897"
}
}
}
this end point updates the specified evaluation by setting the values of the parameters passed.
HTTP Request
PUT https://apiv4.talview.com/models/evaluation/<id>
URL Parameters
Parameter | Type | is Optional | Description |
---|---|---|---|
id | integer | false | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessment_candidate_id | integer | false | The id of the assessment candidate |
panel_id | Integer | false | The Id of the Panel who is/are evaluating the Assessment Section |
assessment_section_id | integer | false | The id of the assessment section |
recommendation | text | false | The recommendation of the evaluation |
assessment_section_candidate_id | Integer | false | The id of Assessment Section Candidate |
Delete a Evaluation
DELETE /models/evaluation<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This end point deletes the specified evaluation.
HTTP Request
DELETE https://apiv4.talview.com/models/evaluation/<id>
Evaluation Comment
The Evaluation Comment endpoint provides the comments given by the Panel after evaluating an Assessment Section Candidate.
The Evaluation Comment object
{
"id": 24793,
"answer_id": null,
"content": "Well rounded. Good communication skills. Clear thought process. ",
"evaluation_id": 87143,
"created_at": "2018-04-17 18:23:27+00",
"updated_at": "2018-04-17 18:23:27+00"
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
answer_id | integer | The Id of the Answer |
content | string | The comment given by the Panel after evaluating an Assessment Section Candidate. |
evaluation_id | integer | The Id of the Evaluation |
List all Evaluation Comment
GET /models/evaluation-comment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 24793,
"answer_id": null,
"content": "Well rounded. Good communication skills. Clear thought process. ",
"evaluation_id": 87143,
"created_at": "2018-04-17 18:23:27+00"
},
{
"id": 24792,
"answer_id": null,
"content": "Very verbose responses. Unsure that he'd be a culture fit with the team.",
"evaluation_id": 87141,
"created_at": "2018-04-17 18:16:47+00"
},
{
"id": 24791,
"answer_id": null,
"content": "Does not seem to be very clear in terms of what he wants to do at this stage.",
"evaluation_id": 87140,
"created_at": "2018-04-17 18:09:34+00"
}
...
]
This endpoint returns all the details of evaluation comments given by the Panel after evaluating an Assessment Section Candidate.
HTTP Request
GET https://apiv4.talview.com/models/evaluation-comment
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
evaluation_id | integer | false | Filter records based on the Id of Evaluation |
answer_id | integer | false | Filter records based on the Id of the Answer |
content | string | false | Filter records based on the comment |
Retrieve an Evaluation Comment
GET /models/evaluation-comment/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 24793,
"answer_id": null,
"content": "Well rounded. Good communication skills. Clear thought process. ",
"evaluation_id": 87143,
"created_at": "2018-04-17 18:23:27+00"
}
This end point retrieve the details of an existing evaluation comments given by the Panel after evaluating an Assessment Section Candidate.
HTTP Request
GET https://apiv4.talview.com/models/evaluation-comment/<id>
URL Parameter
Parameter | type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create an Evaluation Comment
POST /models/evaluation-comment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 25673,
"answer_id": null,
"content": "test 1212",
"evaluation_id": 92627,
"created_at": "2018-05-31T10:41:03+00:00"
}
This end point creates a new evaluation comment for an Assessment Section given by the Panel
HTTP Request
POST https://apiv4.talview.com/models/evaluation-comment
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
evaluation_id | integer | true | The id of the evaluation where comment is associated |
answer_id | integer | false | The Id of the Answer |
content | string | true | The comment given by the Panel after evaluating an Assessment Section Candidate. |
Updates an Evaluation Comment
PUT /models/evaluation-comment/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 24793,
"answer_id": null,
"content": "Well rounded. Good communication skills. Clear thought process. ",
"evaluation_id": 87143,
"created_at": "2018-04-17 18:23:27+00"
}
This end point updates a evaluation comment given by the Panel after evaluating an Assessment Section Candidate.
HTTP Request
PUT https://apiv4.talview.com/models/evaluation-comment/<id>
URL Parameter
Parameter | type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
evaluation_id | integer | false | The id of the evaluation where comment is associated |
answer_id | integer | false | The Id of the Answer |
content | string | false | The comment given by the Panel after evaluating an Assessment Section Candidate. |
Deletes a Evaluation Comment
DELETE /models/evaluation-comment/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code: 204 No Content
This end point deletes an existing evaluation comment
HTTP Request
DELETE https://apiv4.talview.com/models/evaluation-comment/<id>
Evaluation Rating
The Evaluation Rating endpoint gives the rating given by the Panel after evaluating an Assessment Section Candidate.
The Evaluation Rating Object
{
"id": 63511200,
"evaluation_id": 12765172,
"rating": "0.00",
"name": "Testing",
"parameter_id": 116091,
"created_at": "2019-01-18 17:26:04+05:30",
"updated_at": "2019-01-18 17:26:04+05:30",
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
evaluation_id | integer | The Id of the Evaluation |
rating | numeric | The rating given by the Panel for an Assessment Section Candidate. |
name | text | The name of the Parameter |
parameter_id | integer | The Id of the Parameter |
List all Evaluation Rating
GET /models/evaluation-rating HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 63511200,
"evaluation_id": 12765172,
"rating": "0.00",
"name": "Testing",
"parameter_id": 116091,
"created_at": "2019-01-18 17:26:04+05:30",
"updated_at": "2019-01-18 17:26:04+05:30",
}
{
"id": 63569787,
"evaluation_id": 12799272,
"rating": "5.00",
"name": "Test19",
"text": null,
"parameter_id": 116916,
"created_at": "2019-02-18 13:15:59+00",
"updated_at": "2019-02-18 13:15:59+00"
}
...
]
This end point returns all the details of evaluation ratings.
HTTP Request
GET https://apiv4.talview.com/models/evaluation-rating
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
evaluation_id | integer | false | Filter records based on Evaluation Id. |
rating | Float | false | Filter records based on Evaluation Rating |
parameter_id | integer | false | Filter records based on parameter_id |
Retrieve a Evaluation Rating
GET /models/evaluation-rating/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 63569787,
"evaluation_id": 12799272,
"rating": "5.00",
"name": "Test19",
"parameter_id": 116916,
"created_at": "2019-02-18 13:15:59+00",
"updated_at": "2019-02-18 13:15:59+00"
}
This end point retrieves the details of an existing evaluation rating.
HTTP Request
GET https://apiv4.talview.com/models/evaluation-rating/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Update a Evaluation Rating
PUT /models/evaluation-rating/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 63569787,
"evaluation_id": 12799272,
"rating": "5.00",
"name": "Test19",
"parameter_id": 116916,
"created_at": "2019-02-18 13:15:59+00",
"updated_at": "2019-02-18 13:15:59+00"
}
This end point updates the details of an existing evaluation rating.
HTTP Request
PUT https://apiv4.talview.com/models/evaluation-rating/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
evaluation_id | integer | false | The Id of the Evaluation |
rating | Float | false | The rating given by the Panel for an Assessment Section Candidate. |
parameter_id | integer | false | The id of the Parameter |
Delete a Evaluation Rating
This end point deletes the details of an existing evaluation rating.
DELETE /models/evaluation-rating/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns status code : 204 No Content
HTTP Request
DELETE https://apiv4.talview.com/models/evaluation-rating/<id>
Event
Event is a workflow to create & automate a bulk hiring. The event end-point provides the facility to do CRUD operations on event.
The Event Object
{
"id": 196,
"is_public": true,
"external_id": "2345",
"organization_id": 488,
"locationImage": "<img src=\"https://maps.googleapis.com/maps/api/staticmap?zoom=15&markers=colour%3Ared%7C12.9715987%2C77.5945627&size=587x181&key=AIzaSyBAx581mlChMnFeTWYQFsy_6dfMkZDTGX4\" alt=\"\">",
"registration_start_date": "2019-05-22 14:25:00+05:30",
"registration_end_date": "2019-05-28 13:05:00+05:30",
"canSelectAssessment": true,
"registration_form_id": 488401,
"publicUrl": "https://talview.page.link/d3zX",
"status": 3,
"title": "event098",
"code": "1508369126",
"city": null,
"location": {
"url": "https://maps.google.com/?q=Bengaluru,+Karnataka,+India&ftid=0x3bae1670c9b44e6d:0xf8dfc3e8517e4fe0",
"place_id": "ChIJbU60yXAWrjsR4E9-UejD3_g",
"coordinate": {
"lat": 12.9715987,
"lng": 77.59456269999998
},
"formattedAddress": "Bengaluru, Karnataka, India"
},
"formUrl": null,
"start_date": "2019-05-21 13:35:00+05:30",
"end_date": "2019-05-29 06:20:00+05:30",
"owned_by": 433429,
"special_instructions": "defswefsew",
"point_of_contact": null,
"_links": {
"self": {
"href": "/models/event/196"
},
"users": {
"href": "/models/event-user?event_id=196"
},
"panel": {
"href": "/models/event-user?event_id=196&role=Evaluator"
},
"recruiter": {
"href": "/models/event-user?event_id=196&role=Recruiter"
},
"candidates": {
"href": "/models/event-candidate?event_id=196"
},
"eventAssessments": {
"href": "/models/event-assessment?event_id=196"
},
"assessments": {
"href": "/models/assessment?event_id=196"
},
"eventAssessmentsCount": {
"href": "/models/event-assessment?event_id=196&expand=addedCount%2CdeclinedCount%2CinvitedCount%2CinProgressCount%2CattendedCount%2CassessedCount%2CselectedCount%2CholdCount%2CrejectedCount%2CassessmentExpanded"
},
"assessmentsCount": {
"href": "/models/assessment?event_id=196&expand=addedCount%2CinvitedCount%2CinProgressCount%2CattendedCount%2CassessedCount%2CselectedCount%2CholdCount%2CrejectedCount"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
is_public | boolean | Whether the event is public or not |
external_id | text | The external id of the object |
title | text | The title of the Event |
organization_id | integer | The id of the organization to whom the event belongs |
locationImage | text | The url to the location where an event is takin place |
registration_start_date | timestamptz | The time when the registration window is open |
registration_end_date | timestamptz | The time when the registration window expires |
canSelectAssessment | boolean | Does an event has the provision of selecting assessment by candidates |
registration_form_id | integer | The id of the registration form |
publicUrl | text | The URL to register publically(Menu Card disabled) |
status | integer | The status of an event(The status are mentioned below with descriptions) |
code | text | An unique identification code of an event |
city | text | The city where the event is taking place |
location | object | Location details are provided |
formUrl | text | The url to the form used for an event |
start_date | timestamptz | The day from when an event is active |
end_date | timestamptz | The day when an event expires |
owned_by | integer | The id of the user who created the event(who owns the event) |
special_instructions | integer | special instruction is a detailed information of an event. Example: Hall no. 4 |
point_of_contact | integer | The person who is the point of contact |
List of Event Status:
Type | Key | Description |
---|---|---|
DRAFT | 1 | Initial state for an event. In this event status the event is private and the Event owner can edit all the Event details fields. |
OPEN | 2 | Event is public, candidates are created, and is open for candidates to register for the event. (when we allow candidates to choose the assessment) |
CANCELED | 3 | When an event has been cancelled |
LIVE | 4 | The event will be in this state on the Event date/day when the event is gone Live |
PANEL_REVIEW | 5 | When the event is complete and panelists are yet to complete evaluating candidates. |
COMPLETE | 6 | The an event is finished |
List all Events
GET /models/event HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 196,
"is_public": true,
"external_id": "2345",
"organization_id": 488,
"locationImage": "<img src=\"https://maps.googleapis.com/maps/api/staticmap?zoom=15&markers=colour%3Ared%7C12.9715987%2C77.5945627&size=587x181&key=AIzaSyBAx581mlChMnFeTWYQFsy_6dfMkZDTGX4\" alt=\"\">",
"registration_start_date": "2019-05-22 14:25:00+05:30",
"registration_end_date": "2019-05-28 13:05:00+05:30",
"canSelectAssessment": true,
"registration_form_id": 488401,
"publicUrl": "https://talview.page.link/d3zX",
"status": 3,
"title": "event098",
"code": "1508369126",
"city": null,
"location": {
"url": "https://maps.google.com/?q=Bengaluru,+Karnataka,+India&ftid=0x3bae1670c9b44e6d:0xf8dfc3e8517e4fe0",
"place_id": "ChIJbU60yXAWrjsR4E9-UejD3_g",
"coordinate": {
"lat": 12.9715987,
"lng": 77.59456269999998
},
"formattedAddress": "Bengaluru, Karnataka, India"
},
"formUrl": null,
"start_date": "2019-05-21 13:35:00+05:30",
"end_date": "2019-05-29 06:20:00+05:30",
"owned_by": 433429,
"special_instructions": "defswefsew",
"point_of_contact": null,
"_links": {
"self": {
"href": "/models/event/196"
},
"users": {
"href": "/models/event-user?event_id=196"
},
"panel": {
"href": "/models/event-user?event_id=196&role=Evaluator"
},
"recruiter": {
"href": "/models/event-user?event_id=196&role=Recruiter"
},
"candidates": {
"href": "/models/event-candidate?event_id=196"
},
"eventAssessments": {
"href": "/models/event-assessment?event_id=196"
},
"assessments": {
"href": "/models/assessment?event_id=196"
},
"eventAssessmentsCount": {
"href": "/models/event-assessment?event_id=196&expand=addedCount%2CdeclinedCount%2CinvitedCount%2CinProgressCount%2CattendedCount%2CassessedCount%2CselectedCount%2CholdCount%2CrejectedCount%2CassessmentExpanded"
},
"assessmentsCount": {
"href": "/models/assessment?event_id=196&expand=addedCount%2CinvitedCount%2CinProgressCount%2CattendedCount%2CassessedCount%2CselectedCount%2CholdCount%2CrejectedCount"
}
}
}
]
This endpoint returns all the existing events for an organization.
HTTP Request
GET https://apiv4.talview.com/models/event
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | filters records based on the Id of the object |
ids | array of integer | false | filter records based on the Ids of the objects |
title | text | false | filters records based on the title of an event |
external_id | boolean | false | filters records based on the external id of an event |
point_of_contact | boolean | false | filters records based on the point of contact of an event |
owned_by | integer | false | filters records based on the id of the user who owns an event |
city | integer | false | filters events by city |
s | text | false | filters by proivded search text |
assessment_id | id | false | filters events by assessment id |
Retrieve an Event
GET /models/event/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
This endpoint retrieves an event by id.
HTTP Request
GET https://apiv4.talview.com/models/event/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create an Event
This end point is used to create a new Event.
POST /models/event HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"external_id": null,
"registration_form_id": null,
"status": 1,
"is_public": true,
"title": "Event Test",
"city": null,
"formUrl": null,
"canSelectAssessment": true,
"special_instructions": null,
"point_of_contact": null,
"addedCount": null,
"invitedCount": null,
"inProgressCount": null,
"attendedCount": null,
"assessedCount": null,
"selectedCount": null,
"holdCount": null,
"rejectedCount": null,
"publicUrl": null,
"owned_by": "30876"
}
The above request returns JSON structured like this:
{
"id": 664,
"is_public": true,
"external_id": null,
"organization_id": 488,
"locationImage": null,
"registration_start_date": null,
"registration_end_date": null,
"canSelectAssessment": true,
"registration_form_id": null,
"publicUrl": "https://talview.page.link/Ns57",
"status": 1,
"title": "Event Test",
"code": 3816464517,
"city": null,
"location": [],
"formUrl": null,
"start_date": null,
"end_date": null,
"owned_by": "30876",
"special_instructions": null,
"point_of_contact": null,
"_links": {
"self": {
"href": "/models/event/664"
},
"users": {
"href": "/models/event-user?event_id=664"
},
"panel": {
"href": "/models/event-user?event_id=664&role=Evaluator"
},
"recruiter": {
"href": "/models/event-user?event_id=664&role=Recruiter"
},
"candidates": {
"href": "/models/event-candidate?event_id=664"
},
"eventAssessments": {
"href": "/models/event-assessment?event_id=664"
},
"assessments": {
"href": "/models/assessment?event_id=664"
},
"eventAssessmentsCount": {
"href": "/models/event-assessment?event_id=664&expand=addedCount%2CdeclinedCount%2CinvitedCount%2CinProgressCount%2CattendedCount%2CassessedCount%2CselectedCount%2CholdCount%2CrejectedCount%2CassessmentExpanded"
},
"assessmentsCount": {
"href": "/models/assessment?event_id=664&expand=addedCount%2CinvitedCount%2CinProgressCount%2CattendedCount%2CassessedCount%2CselectedCount%2CholdCount%2CrejectedCount"
}
}
}
HTTP Request
POST https://apiv4.talview.com/models/event
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
external_id | text | false | The external id of the object |
status | integer | false | The status of an event(The status are mentioned below with descriptions) |
is_public | boolean | false | Whether the event is public or not |
title | text | true | The title of the Assessment |
city | text | false | The city where the event is taking place |
canSelectAssessment | boolean | false | Does an event has the provision of selecting assessment by candidates |
special_instructions | text | false | Special instruction is a detailed information of an event. Example: Hall no. 4 |
point_of_contact | integer | false | The person who is the point of contact |
start_date | timestamptz | false | The day from when an event is active |
end_date | timestamptz | false | The day when an event expires |
location | object | false | Location details are provided |
registration_end_date | timestamptz | false | The time when the registration window expires |
registration_start_date | timestamptz | false | The time when the registration window is open |
owned_by | integer | false | The id of the user who created the event(who owns the event) |
Update an Event
Updates the details of an event for the event id provided in the URL.
PUT /models/event/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
HTTP Request
PUT https://apiv4.talview.com/models/event/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
external_id | text | false | The external id of the object |
status | integer | false | The status of an event(The status are mentioned below with descriptions) |
is_public | boolean | false | Whether the event is public or not |
title | text | false | The title of the Assessment |
city | text | false | The city where the event is taking place |
canSelectAssessment | boolean | false | Does an event has the provision of selecting assessment by candidates |
special_instructions | text | false | Special instruction is a detailed information of an event. Example: Hall no. 4 |
point_of_contact | integer | false | The person who is the point of contact |
start_date | timestamptz | false | The day from when an event is active |
end_date | timestamptz | false | The day when an event expires |
location | object | false | Location details are provided |
registration_end_date | timestamptz | false | The time when the registration window expires |
registration_start_date | timestamptz | false | The time when the registration window is open |
owned_by | integer | false | The id of the user who created the event(who owns the event) |
Delete an Event
DELETE /models/event/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns a status code : 204 No Content
The end-point deletes an event for an id provided as an URL parameter.
HTTP Request
DELETE https://apiv4.talview.com/models/event/<id>
Event Assessment
Event Assessment end point gives the details of the assessments added to the event by a recruiter.
The Event Assessment
{
"id": 471,
"event_id": 189,
"assessment_id": 67656,
"created_at": "2019-05-13 07:50:40+00",
"created_by": 30876,
"updated_at": "2019-05-13 07:50:40+00",
"updated_by": 30876
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
event_id | integer | The unique identifier of an Event |
assessment_id | integer | The unique identifier of an Assessment |
created_at | timestamp(0) with time zone | The timestamp of when the assessment was added to the event |
created_by | integer | The id of the User who added the assessment to the event |
updated_at | timestamp(0) with time zone | The timestamp of when the assessment details were updated |
updated_by | integer | The id of the user who updated the details of the event assessment |
List all Event Assessment
GET /models/event-assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 471,
"event_id": 189,
"assessment_id": 67656,
"created_at": "2019-05-13 07:50:40+00",
"created_by": 30876,
"updated_at": "2019-05-13 07:50:40+00",
"updated_by": 30876
}
This endpoint list all event assessment.
HTTP Request
GET https://apiv4.talview.com/models/event-assessment
Retrieve an Event Assessment
GET /models/event-assessment/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 471,
"event_id": 189,
"assessment_id": 67656,
"created_at": "2019-05-13 07:50:40+00",
"created_by": 30876,
"updated_at": "2019-05-13 07:50:40+00",
"updated_by": 30876
}
This endpoint retrieves a specific event assessment.
HTTP Request
GET https://apiv4.talview.com/models/event-assessment/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on the id |
Create an Event assessment
Creates a event assessment.
POST /models/event-assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
{
"id": 471,
"event_id": 189,
"assessment_id": 67656,
"created_at": "2019-05-13 07:50:40+00",
"created_by": 30876,
"updated_at": "2019-05-13 07:50:40+00",
"updated_by": 30876
}
HTTP Request
POST https://apiv4.talview.com/models/event-user
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
event_id | integer | true | The unique identifier of an Event |
assessment_id | integer | true | The unique identifier of a Assessment |
Update a Event Assessment
PUT /models/event-assessment/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 471,
"event_id": 189,
"assessment_id": 67656,
"created_at": "2019-05-13 07:50:40+00",
"created_by": 30876,
"updated_at": "2019-05-13 07:50:40+00",
"updated_by": 30876
}
This endpoint updates a specific event assessment.
HTTP Request
PUT https://apiv4.talview.com/models/event-assessment/<id>
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Delete a Event Assessment
DELETE /models/event-assessment/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This endpoint deletes a specific event assessment.
HTTP Request
DELETE https://apiv4.talview.com/models/event-assessment/<id>
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Event Candidate
Event Candidate is created when a candidate is invited to an event. The Event Candidate end point gives the details of the invite.
The Event Candidate Object
{
"id": 404,
"event_id": 660,
"candidate_id": 7422021,
"form_instance_id": null,
"assessment_candidate_id": null,
"created_by": 234078,
"created_at": "2019-06-25 07:19:04+00",
"updated_at": "2019-06-25 07:19:04+00",
"updated_by": 234078
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
event_id | integer | The unique identifier of the Event |
candidate_id | integer | The unique identifier of the Candidate |
form_instance_id | integer | The unique identifier of the Form Instance |
assessment_candidate_id | integer | The unique identifier of the Assessment Candidate |
created_by | integer | The id of the User who creates an Event Candidate |
created_at | timestamptz | The time when an Event Candidate is being created |
updated_at | timestamptz | The time when an Event Candidate is being updated |
updated_by | integer | The id of the User who updates an Event Candidate |
List all Event Candidate
GET /models/event-candidate HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 404,
"event_id": 660,
"candidate_id": 7422021,
"form_instance_id": null,
"assessment_candidate_id": null,
"created_by": 234078,
"created_at": "2019-06-25 07:19:04+00",
"updated_at": "2019-06-25 07:19:04+00",
"updated_by": 234078
},
{
"id": 403,
"event_id": 660,
"candidate_id": 7422020,
"form_instance_id": null,
"assessment_candidate_id": null,
"created_by": 234078,
"created_at": "2019-06-25 07:19:03+00",
"updated_at": "2019-06-25 07:19:03+00",
"updated_by": 234078
}
]
This endpoint returns all the existing Event Candidates of an Organization.
HTTP Request
GET https://apiv4.talview.com/models/event-candidate
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | filter records based by the event candidate id provided |
ids | array of integer | false | filter records by the event candidate ids(array of ids) |
assessment_id | integer | false | filter invites by the assessment id provided |
s | text | false | filter invites by the provided search text |
status_sort_key | integer | false | filter invites by the provided status sort key |
candidate_id | integer | false | filter invites by candidate id |
event_id | integer | false | filter invites by the id of an Event |
Retrieve an Event Candidate
GET /models/event-candidate/402 HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 402,
"event_id": 660,
"candidate_id": 7422019,
"form_instance_id": null,
"assessment_candidate_id": null,
"created_by": 234078,
"created_at": "2019-06-25 07:19:02+00",
"updated_at": "2019-06-25 07:19:02+00",
"updated_by": 234078
}
This endpoint retrieves an Event Cadidate by id provided as an URL parameter.
HTTP Request
GET https://apiv4.talview.com/models/event-candidate/<id>
URL Parameters|
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records by id |
Create an Event Candidate
This end point creates an Event Candidate.
POST /models/event-candidate HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"event_id":402,
"assessment_candidate_id": null,
"candidate_id":7422020
}
The above request returns JSON structured like this:
{
"id": 406,
"event_id": 402,
"candidate_id": 7422020,
"form_instance_id": null,
"assessment_candidate_id": null,
"created_by": 30876,
"created_at": "2019-06-25T09:38:56+00:00",
"updated_at": "2019-06-25T09:38:56+00:00",
"updated_by": 30876
}
HTTP Request
POST https://apiv4.talview.com/models/event-candidate
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
event_id | integer | true | The unique id of an Event |
assessment_candidate_id | integer | false | The unique id of an Assessment Candidate |
candidate_id | integer | true | The unique id of an Candidate |
Delete an Event Candidate
DELETE /models/event-candidate/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns a status code : 204 No Content
The end-point deletes Event Candidate for a specific id. The id needs to be passed as an URL parameter.
HTTP Request
DELETE https://apiv4.talview.com/models/event-candidate/<id>
Event User
The Event User end point gives the details of the user added to event.
The Event User Object
{
"id": 471,
"event_id": 189,
"user_id": 67656,
"status": 2,
"role": "Recruiter",
"panel_id": null,
"created_at": "2019-05-13 07:50:40+00",
"created_by": 30876,
"updated_at": "2019-05-13 07:50:40+00",
"updated_by": 30876
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
event_id | integer | The unique identifier of an Event |
user_id | integer | The unique identifier of a User |
status | integer | The status of the event user |
role | string | The role of the user in the event |
panel_id | integer | The id of the event panel(If the user has role set as evaluator) |
created_at | timestamp(0) with time zone | The timestamp of when the user was added to the event |
created_by | integer | The id of the user who added the event user in the event |
updated_at | timestamp(0) with time zone | The timestamp of when the user details were updated |
updated_by | integer | The id of the user who updated the details of the event user |
List of role
Role | Description |
---|---|
Recruiter | The recruiter is an event user who adds an assessment to the event |
Evaluator | The evaluator is an event user who is added as a panel to the event |
List of status
Status | Key | Description |
---|---|---|
PENDING | 1 | The event users who have been invited but not yet accepted the invitation for the event |
ACCEPTED | 2 | The event users have accepted the event invitation |
DECLINED | 3 | The event users who have rejected / declined the event invite |
List all Event User
GET /models/event-user HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 471,
"event_id": 189,
"user_id": 67656,
"status": 2,
"role": "Recruiter",
"panel_id": null,
"created_at": "2019-05-13 07:50:40+00",
"created_by": 30876,
"updated_at": "2019-05-13 07:50:40+00",
"updated_by": 30876
}
This endpoint list all event users.
HTTP Request
GET https://apiv4.talview.com/models/event-user
Retrieve an Event User
GET /models/event-user/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 471,
"event_id": 189,
"user_id": 67656,
"status": 2,
"role": "Recruiter",
"panel_id": null,
"created_at": "2019-05-13 07:50:40+00",
"created_by": 30876,
"updated_at": "2019-05-13 07:50:40+00",
"updated_by": 30876
}
This endpoint retrieves a specific event user.
HTTP Request
GET https://apiv4.talview.com/models/event-user/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on the id |
Create an Event User
Creates a event user.
POST /models/event-user HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
{
"id": 471,
"event_id": 189,
"user_id": 67656,
"status": 2,
"role": "Recruiter",
"panel_id": null,
"created_at": "2019-05-13 07:50:40+00",
"created_by": 30876,
"updated_at": "2019-05-13 07:50:40+00",
"updated_by": 30876
}
HTTP Request
POST https://apiv4.talview.com/models/event-user
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
event_id | integer | true | The unique identifier of an Event |
user_id | integer | true | The unique identifier of a User |
role | integer | true | The role of the user in the event |
Update an Event User
PUT /models/event-user/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 471,
"event_id": 189,
"user_id": 67656,
"status": 2,
"role": "Recruiter",
"panel_id": null,
"created_at": "2019-05-13 07:50:40+00",
"created_by": 30876,
"updated_at": "2019-05-13 07:50:40+00",
"updated_by": 30876
}
This endpoint updates a specific event user.
HTTP Request
PUT https://apiv4.talview.com/models/event-user/<id>
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Delete an Event User
DELETE /models/event-user/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This endpoint deletes a specific event user.
HTTP Request
DELETE https://apiv4.talview.com/models/event-user/<id>
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
External Assessment
The External Assessment endpoint gives the details of all the External Assessment
The Evaluation rating object
{
"id": 107,
"reference_id": null,
"title": "UI Test",
"start_date": "2017-11-28 00:00:00+05:30",
"end_date": "2017-12-04 18:30:00",
"description": null,
"duration": "01:00:00",
"status": 100,
"created_at": "2017-11-28 17:37:55+05:30",
"updated_at": "2017-11-28 17:37:55+05:30",
"addedCount": 0,
"invitedCount": 0,
"attendingCount": 1,
"completedCount": 0,
"evaluatedCount": 0,
"selectedCount": 0,
"rejectedCount": 0,
"_links": {
"self": {
"href": "/integration/service-provider/assessment/107"
},
"external-invitations": {
"href": "/integration/service-provider/assessment/107/invitation-code"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
reference_id | text | The external reference Id of the Object |
title | text | The title of the External Assessment |
start_date | timestamptz | The date and time from when the External Assessment is active and can be attended |
end_date | timestamptz | The last date and time to attend the External Assessment |
description | text | The description of the External Assessment |
duration | timestamptz | The duration to attain the External Assessment |
status | integer | The status of the External Assessment |
addedCount | integer | The number of Candidate who has been added for the External Assessment |
invitedCount | integer | The number of Candidate who has been invited for the External Assessment |
attendingCount | integer | The number of Candidate who has been further added for the External Assessment |
completedCount | integer | The number of Candidate who had completed the External Assessment |
evaluatedCount | integer | The number of Candidate whos External Assessment has been evalauted |
selectedCount | integer | The number of Candidate who has been selected in the External Assessment |
rejectedCount | integer | The number of Candidate who has been rejected in the External Assessment |
List of Status:
Status | Key | Description |
---|---|---|
INACTIVE | 10 | The external assessment is inactive |
ARCHIVED | 20 | The external assessment is archived |
ACTIVE | 100 | The external assessment is active |
Lists all external assessments
GET /models/external-assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 110,
"reference_id": null,
"title": "Testing assessment",
"start_date": "2017-11-29 00:00:00+05:30",
"end_date": "2017-12-05 18:30:00",
"description": null,
"duration": "01:00:00",
"status": 100,
"created_at": "2017-11-29 16:50:50+05:30",
"updated_at": "2017-11-29 16:50:50+05:30",
"addedCount": 0,
"invitedCount": 0,
"attendingCount": 0,
"completedCount": 0,
"evaluatedCount": 0,
"selectedCount": 0,
"rejectedCount": 0,
"_links": {
"self": {
"href": "/integration/service-provider/assessment/110"
},
"external-invitations": {
"href": "/integration/service-provider/assessment/110/invitation-code"
}
}
}
]
Returns a list of external assessment.
HTTP Request
GET https://apiv4.talview.com/models/external-assessment
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the unique identifier of the object |
reference_id | text | false | Filter records based on the external reference Id of the Object |
title | text | false | Filter records based on the title of the External Assessment |
start_date | timestamptz | false | Filter records based on the date and time from when the External Assessment is active and can be attended |
end_date | timestamptz | false | Filter records based on the last date and time to attend the External Assessment |
description | text | false | Filter records based on the description of the External Assessment |
duration | timestamptz | false | Filter records based on the duration to attain the External Assessment |
status | integer | false | Filter records based on the status of the External Assessment |
Retrieves an external assessments
GET /models/external-assessment/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 110,
"reference_id": null,
"title": "Testing assessment",
"start_date": "2017-11-29 00:00:00+05:30",
"end_date": "2017-12-05 18:30:00",
"description": null,
"duration": "01:00:00",
"status": 100,
"created_at": "2017-11-29 16:50:50+05:30",
"updated_at": "2017-11-29 16:50:50+05:30",
"addedCount": 0,
"invitedCount": 0,
"attendingCount": 0,
"completedCount": 0,
"evaluatedCount": 0,
"selectedCount": 0,
"rejectedCount": 0,
"_links": {
"self": {
"href": "/integration/service-provider/assessment/110"
},
"external-invitations": {
"href": "/integration/service-provider/assessment/110/invitation-code"
}
}
}
Returns an external assessment.
HTTP Request
GET https://apiv4.talview.com/models/external-assessment/id
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the unique identifier of the object |
Creates an external assessments
POST /models/external-assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 110,
"reference_id": null,
"title": "Testing assessment",
"start_date": "2017-11-29 00:00:00+05:30",
"end_date": "2017-12-05 18:30:00",
"description": null,
"duration": "01:00:00",
"status": 100,
"created_at": "2017-11-29 16:50:50+05:30",
"updated_at": "2017-11-29 16:50:50+05:30",
"addedCount": 0,
"invitedCount": 0,
"attendingCount": 0,
"completedCount": 0,
"evaluatedCount": 0,
"selectedCount": 0,
"rejectedCount": 0,
"_links": {
"self": {
"href": "/integration/service-provider/assessment/110"
},
"external-invitations": {
"href": "/integration/service-provider/assessment/110/invitation-code"
}
}
}
Creates an external assessment.
HTTP Request
POST https://apiv4.talview.com/models/external-assessment
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
reference_id | text | false | The external reference Id of the Object |
title | text | false | The title of the External Assessment |
start_date | timestamptz | false | The date and time from when the External Assessment is active and can be attended |
end_date | timestamptz | false | The last date and time to attend the External Assessment |
description | text | false | The description of the External Assessment |
duration | timestamptz | false | The duration to attain the External Assessment |
status | integer | false | The status of the External Assessment |
Updates an external assessments
PUT /models/external-assessment/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 110,
"reference_id": null,
"title": "Testing assessment",
"start_date": "2017-11-29 00:00:00+05:30",
"end_date": "2017-12-05 18:30:00",
"description": null,
"duration": "01:00:00",
"status": 100,
"created_at": "2017-11-29 16:50:50+05:30",
"updated_at": "2017-11-29 16:50:50+05:30",
"addedCount": 0,
"invitedCount": 0,
"attendingCount": 0,
"completedCount": 0,
"evaluatedCount": 0,
"selectedCount": 0,
"rejectedCount": 0,
"_links": {
"self": {
"href": "/integration/service-provider/assessment/110"
},
"external-invitations": {
"href": "/integration/service-provider/assessment/110/invitation-code"
}
}
}
Updates an external assessment.
HTTP Request
PUT https://apiv4.talview.com/models/external-assessment/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
reference_id | text | false | The external reference Id of the Object |
title | text | false | The title of the External Assessment |
start_date | timestamptz | false | The date and time from when the External Assessment is active and can be attended |
end_date | timestamptz | false | The last date and time to attend the External Assessment |
description | text | false | The description of the External Assessment |
duration | timestamptz | false | The duration to attain the External Assessment |
status | integer | false | The status of the External Assessment |
Deletes an external assessments
DELETE /models/external-assessment/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
Deletes an external assessment.
HTTP Request
DELETE https://apiv4.talview.com/models/external-assessment/id
Evaluation Invitation
The Evaluation Invitation endpoint gives the mapping details of the External Assessment and the Assessment Section Candidate
The Evaluation invitation object
{
"id": 340,
"external_assessment_id": null,
"assessment_section_candidate_id": 118816,
"candidate": {
"email": "ksajnani94@gmail.com",
"first_name": "Karan",
"last_name": "Sajnani"
},
"username": "ksajnani94@gmail.com",
"code": "98335615",
"status": 15,
"score": null,
"evaluation": null,
"report_url": "https://www.versanttest.com/ppass/ppuser/greport.jsp?pin=98335615",
"attend_url": "https://ptpprod.pearsontestservices.com/take_test",
"created_at": "2016-05-17 18:21:56+05:30",
"updated_at": "2018-03-17 18:26:08+05:30",
"_links": {
"self": {
"href": "/integration/service-provider/invitation-code/view?id=340"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
external_id | text | The external Id for the object |
is_deleted | boolean | If true, the External Assessment can be deleted |
username | text | The name of the User who created the External Assessment |
code | text | The code for the External Assessment |
external_assessment_id | integer | The Id of the External Assessment |
status | integer | The status of the External Assessment |
reprot_url | text | The URL for the report of External Assessment |
attend_url | text | The URL for attending the External Assessment |
assessment_section_candidate_is | integer | The Id of the Assessment Section Candidate |
Lists all external assessments
GET /models/external-assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 340,
"external_assessment_id": null,
"assessment_section_candidate_id": 118816,
"candidate": {
"email": "ksajnani94@gmail.com",
"first_name": "Karan",
"last_name": "Sajnani"
},
"username": "ksajnani94@gmail.com",
"code": "98335615",
"status": 15,
"score": null,
"evaluation": null,
"report_url": "https://www.versanttest.com/ppass/ppuser/greport.jsp?pin=98335615",
"attend_url": "https://ptpprod.pearsontestservices.com/take_test",
"created_at": "2016-05-17 18:21:56+05:30",
"updated_at": "2018-03-17 18:26:08+05:30",
"_links": {
"self": {
"href": "/integration/service-provider/invitation-code/view?id=340"
}
}
},
{
"id": 326,
"external_assessment_id": null,
"assessment_section_candidate_id": 118802,
"candidate": {
"email": "hrsj26@gmail.com",
"first_name": "Harshita",
"last_name": "Jaiswal"
},
"username": "hrsj26@gmail.com",
"code": "61760927",
"status": 15,
"score": null,
"evaluation": null,
"report_url": "https://www.versanttest.com/ppass/ppuser/greport.jsp?pin=61760927",
"attend_url": "https://ptpprod.pearsontestservices.com/take_test",
"created_at": "2016-05-17 18:21:43+05:30",
"updated_at": "2018-03-17 18:26:08+05:30",
"_links": {
"self": {
"href": "/integration/service-provider/invitation-code/view?id=326"
}
}
},
]
Returns a list of external assessment.
HTTP Request
GET https://apiv4.talview.com/models/external-assessment
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the unique identifier of the object |
username | text | false | Filter records based on the name of the User who created the External Assessment |
code | text | false | Filter records based on the code for the External Assessment |
external_assessment_id | integer | false | Filter records based on the Id of the External Assessment |
status | integer | false | Filter records based on the status of the External Assessment |
reprot_url | text | false | Filter records based on the URL for the report of External Assessment |
attend_url | text | false | Filter records based on the URL for attending the External Assessment |
assessment_section_candidate_is | integer | false | Filter records based on the Id of the Assessment Section Candidate |
Retrieves an external assessments
GET /models/external-assessment/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 340,
"external_assessment_id": null,
"assessment_section_candidate_id": 118816,
"candidate": {
"email": "ksajnani94@gmail.com",
"first_name": "Karan",
"last_name": "Sajnani"
},
"username": "ksajnani94@gmail.com",
"code": "98335615",
"status": 15,
"score": null,
"evaluation": null,
"report_url": "https://www.versanttest.com/ppass/ppuser/greport.jsp?pin=98335615",
"attend_url": "https://ptpprod.pearsontestservices.com/take_test",
"created_at": "2016-05-17 18:21:56+05:30",
"updated_at": "2018-03-17 18:26:08+05:30",
"_links": {
"self": {
"href": "/integration/service-provider/invitation-code/view?id=340"
}
}
}
Returns an external assessment.
HTTP Request
GET https://apiv4.talview.com/models/external-assessment/id
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on the unique identifier of the object |
Creates an external assessments
POST /models/external-assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 340,
"external_assessment_id": null,
"assessment_section_candidate_id": 118816,
"candidate": {
"email": "ksajnani94@gmail.com",
"first_name": "Karan",
"last_name": "Sajnani"
},
"username": "ksajnani94@gmail.com",
"code": "98335615",
"status": 15,
"score": null,
"evaluation": null,
"report_url": "https://www.versanttest.com/ppass/ppuser/greport.jsp?pin=98335615",
"attend_url": "https://ptpprod.pearsontestservices.com/take_test",
"created_at": "2016-05-17 18:21:56+05:30",
"updated_at": "2018-03-17 18:26:08+05:30",
"_links": {
"self": {
"href": "/integration/service-provider/invitation-code/view?id=340"
}
}
}
Creates an external assessment.
HTTP Request
POST https://apiv4.talview.com/models/external-assessment
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
attend_url | text | true | The URL for attending the External Assessment |
external_assessment_id | integer | true | The Id of the External Assessment |
username | text | false | The name of the User who created the External Assessment |
code | text | false | The code for the External Assessment |
status | integer | false | The status of the External Assessment |
reprot_url | text | false | The URL for the report of External Assessment |
Updates an external assessments
PUT /models/external-assessment/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 340,
"external_assessment_id": null,
"assessment_section_candidate_id": 118816,
"candidate": {
"email": "ksajnani94@gmail.com",
"first_name": "Karan",
"last_name": "Sajnani"
},
"username": "ksajnani94@gmail.com",
"code": "98335615",
"status": 15,
"score": null,
"evaluation": null,
"report_url": "https://www.versanttest.com/ppass/ppuser/greport.jsp?pin=98335615",
"attend_url": "https://ptpprod.pearsontestservices.com/take_test",
"created_at": "2016-05-17 18:21:56+05:30",
"updated_at": "2018-03-17 18:26:08+05:30",
"_links": {
"self": {
"href": "/integration/service-provider/invitation-code/view?id=340"
}
}
}
Updates an external assessment.
HTTP Request
PUT https://apiv4.talview.com/models/external-assessment/id
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
attend_url | text | false | The URL for attending the External Assessment |
external_assessment_id | integer | false | The Id of the External Assessment |
username | text | false | The name of the User who created the External Assessment |
code | text | false | The code for the External Assessment |
status | integer | false | The status of the External Assessment |
reprot_url | text | false | The URL for the report of External Assessment |
Deletes an external assessments
DELETE /models/external-assessment/id HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
Deletes an external assessment.
HTTP Request
DELETE https://apiv4.talview.com/models/external-assessment/id
File
The File endpoint gives details of all the uploaded file.
The File Object
{
"id": 13960368,
"name": "image_00001",
"external_id": null,
"description": null,
"pdfUrl": null,
"file_type_id": 15,
"size": null,
"created_at": "2019-03-18 08:30:03+00",
"updated_at": "2019-03-18 08:30:03+00",
"resourceUrl": "https://talview.s3.ap-southeast-1.amazonaws.com/converted-async/488/2009328/2166672/image_00001.png?X-Amz-Content-Sha256=UNSIGNED-PAYLOAD&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAJ5GZJUFNA7J2GFZQ%2F20190318%2Fap-southeast-1%2Fs3%2Faws4_request&X-Amz-Date=20190318T084008Z&X-Amz-SignedHeaders=host&X-Amz-Expires=518400&X-Amz-Signature=34e7f2d9cd6a3813dd48079e3fd3f46db4a7845de9c3993f7b30c4136e4b47c9",
"extension": "png",
"originalName": "image_00001.png",
"_links": {
"fileType": {
"href": "/models/file-type/15"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
name | text | The name of the File |
external_id | string | The reference Id of the object |
description | text | The description of File |
pdfUrl | string | The url of the files that are converted in to pdf internally |
file_type_id | integer | The id of File Type |
size | integer | The size of the File |
resourceUrl | string | The url of the resource |
extension | text | The extension of the File |
originalName | text | The original name of file with extension |
List all Files
GET /models/file HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 13960368,
"name": "image_00001",
"external_id": null,
"description": null,
"pdfUrl": null,
"file_type_id": 15,
"size": null,
"created_at": "2019-03-18 08:30:03+00",
"updated_at": "2019-03-18 08:30:03+00",
"resourceUrl": "https://talview.s3.ap-southeast-1.amazonaws.com/converted-async/488/2009328/2166672/image_00001.png?X-Amz-Content-Sha256=UNSIGNED-PAYLOAD&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAJ5GZJUFNA7J2GFZQ%2F20190318%2Fap-southeast-1%2Fs3%2Faws4_request&X-Amz-Date=20190318T084008Z&X-Amz-SignedHeaders=host&X-Amz-Expires=518400&X-Amz-Signature=34e7f2d9cd6a3813dd48079e3fd3f46db4a7845de9c3993f7b30c4136e4b47c9",
"extension": "png",
"originalName": "image_00001.png",
"_links": {
"fileType": {
"href": "/models/file-type/15"
}
}
},
{
"id": 13960367,
"name": "5091772",
"external_id": null,
"description": null,
"pdfUrl": null,
"file_type_id": 4,
"size": null,
"created_at": "2019-03-18 08:30:02+00",
"updated_at": "2019-03-18 08:30:02+00",
"resourceUrl": "https://talview.s3.ap-southeast-1.amazonaws.com/converted-async/488/2009328/2166672/5091772.mp4?X-Amz-Content-Sha256=UNSIGNED-PAYLOAD&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAJ5GZJUFNA7J2GFZQ%2F20190318%2Fap-southeast-1%2Fs3%2Faws4_request&X-Amz-Date=20190318T085603Z&X-Amz-SignedHeaders=host&X-Amz-Expires=518400&X-Amz-Signature=2e46240b32038647d277db6209bc29ee6942d58328e6e7a10c73dcee8f61d7fb",
"extension": "mp4",
"originalName": "5091772.mp4",
"_links": {
"fileType": {
"href": "/models/file-type/4"
}
}
}
]
This endpoint returns the details of all the stored Files.
HTTP Request
GET https://apiv4.talview.com/models/file
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
file_type_id | integer | false | Filter records based on the Id of File Type |
name | text | false | The name of the File |
Retrieve a File
GET /models/file/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 13960367,
"name": "5091772",
"external_id": null,
"description": null,
"pdfUrl": null,
"file_type_id": 4,
"size": null,
"created_at": "2019-03-18 08:30:02+00",
"updated_at": "2019-03-18 08:30:02+00",
"resourceUrl": "https://talview.s3.ap-southeast-1.amazonaws.com/converted-async/488/2009328/2166672/5091772.mp4?X-Amz-Content-Sha256=UNSIGNED-PAYLOAD&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAJ5GZJUFNA7J2GFZQ%2F20190318%2Fap-southeast-1%2Fs3%2Faws4_request&X-Amz-Date=20190318T085603Z&X-Amz-SignedHeaders=host&X-Amz-Expires=518400&X-Amz-Signature=2e46240b32038647d277db6209bc29ee6942d58328e6e7a10c73dcee8f61d7fb",
"extension": "mp4",
"originalName": "5091772.mp4",
"_links": {
"fileType": {
"href": "/models/file-type/4"
}
}
}
This endpoint is used for retrieving a particular file.
HTTP Request
GET https://apiv4.talview.com/models/file/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create a File
POST /models/file HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 13960367,
"name": "5091772",
"external_id": null,
"description": null,
"pdfUrl": null,
"file_type_id": 4,
"size": null,
"created_at": "2019-03-18 08:30:02+00",
"updated_at": "2019-03-18 08:30:02+00",
"resourceUrl": "https://talview.s3.ap-southeast-1.amazonaws.com/converted-async/488/2009328/2166672/5091772.mp4?X-Amz-Content-Sha256=UNSIGNED-PAYLOAD&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAJ5GZJUFNA7J2GFZQ%2F20190318%2Fap-southeast-1%2Fs3%2Faws4_request&X-Amz-Date=20190318T085603Z&X-Amz-SignedHeaders=host&X-Amz-Expires=518400&X-Amz-Signature=2e46240b32038647d277db6209bc29ee6942d58328e6e7a10c73dcee8f61d7fb",
"extension": "mp4",
"originalName": "5091772.mp4",
"_links": {
"fileType": {
"href": "/models/file-type/4"
}
}
}
This endpoint is used for creating a file.
HTTP Request
POST https://apiv4.talview.com/models/file
Body Parameters(Mutlipart body)
Parameter | Type | Mandatory | Description |
---|---|---|---|
file_type_id | integer | true | The id of File Type |
file | file | true | The File to be uploaded |
name | text | false | The name of the File |
is_public | boolean | false | Flag |
Update a File
PUT /models/file/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 13960367,
"name": "5091772",
"external_id": null,
"description": null,
"pdfUrl": null,
"file_type_id": 4,
"size": null,
"created_at": "2019-03-18 08:30:02+00",
"updated_at": "2019-03-18 08:30:02+00",
"resourceUrl": "https://talview.s3.ap-southeast-1.amazonaws.com/converted-async/488/2009328/2166672/5091772.mp4?X-Amz-Content-Sha256=UNSIGNED-PAYLOAD&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAJ5GZJUFNA7J2GFZQ%2F20190318%2Fap-southeast-1%2Fs3%2Faws4_request&X-Amz-Date=20190318T085603Z&X-Amz-SignedHeaders=host&X-Amz-Expires=518400&X-Amz-Signature=2e46240b32038647d277db6209bc29ee6942d58328e6e7a10c73dcee8f61d7fb",
"extension": "mp4",
"originalName": "5091772.mp4",
"_links": {
"fileType": {
"href": "/models/file-type/4"
}
}
}
This endpoint is used for updating a file.
HTTP Request
PUT https://apiv4.talview.com/models/file/<Id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
file_type_id | integer | false | The id of File Type |
description | text | false | The description of File |
name | text | false | The name of the File |
File Type
The File Type endpoint gives the details of the type of file that are available in File endpoint.
The File Type Object
{
"id": 1,
"name": "flash flv",
"description": "raw flv video file from flash server",
"location": null,
"created_at": "2019-01-11 09:34:44+00",
"updated_at": "2019-01-11 09:34:44+00",
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
name | text | The name of the File Type. Example: flash flv, flash mp4 etc |
description | text | The description of File Type |
List all File Type
GET /models/file-type HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 1,
"name": "flash flv",
"description": "raw flv video file from flash server",
"location": null,
"created_at": "2019-01-11 09:34:44+00",
"updated_at": "2019-01-11 09:34:44+00",
}
{
"id": 2,
"name": "flash mp4",
"description": "raw mp4 video file from flash server",
"location": null,
"created_at": "2019-01-11 09:34:44+00",
"updated_at": "2019-01-11 09:34:44+00",
}
]
This endpoint lists all file type.
HTTP Request
GET https://apiv4.talview.com/models/file-type
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
name | text | false | Filter records based on name |
description | text | false | Filter records based on description |
Retrieve a File Type
GET /models/file-type/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1,
"name": "flash flv",
"description": "raw flv video file from flash server",
"location": null,
"created_at": "2016-04-19 06:09:01+00",
"updated_at": null
}
This endpoint retrieves a specific file type.
HTTP Request
GET https://apiv4.talview.com/models/file-type/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
File Upload
The File Upload endpoint is used for uploading the file in the server.
The File Upload Object
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
file_type_id | integer | The Id of the File Type |
is_public | boolean | If true, the file can be retrieved |
organization_id | integer | The id of the Organization |
status | integer | The status of the file upload.Example: Uploaded, Queued. The Status of the File Upload are described in the list below |
server_id | integer | The Id of the server where the file will be uploaded |
name | text | The name of the File |
extension | text | The extension of the File |
path | string | The path where the File will be uploaded |
modified_by | integer | The id of the User who uploaded the file |
List of File Upload Status:
Status | Key | Description |
---|---|---|
Available | 100 | The file is available in the server |
UnAvailable | 999 | The file is not available in the server |
Queued | 101 | The file is queued for the process |
Processing | 102 | The file is under process |
Complete | 103 | The file upload is complete |
Error | 104 | Error while uploading the file |
Upload a File
POST /models/file-upload HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
This end point is used for uploading a file in the server.
HTTP Request
POST https://apiv4.talview.com/models/file-upload
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
data | text | true | The File (base64 string) |
name | text | false | The name of the File |
- data attribute is the base64 of the file uploaded.
Form
The Form endpoint gives the details of all the available form.
The Form Object
{
"id": 8363,
"name": "608327",
"structure": [
{
"id": 1,
"name": "Assessment form",
"fields": {
"1": {
"id": "cf_fn_name",
"name": "fname",
"type": "text",
"label": "First Name",
"autocomplete": "on"
},
"2": {
"id": "cf_ln_name",
"name": "lname",
"type": "text",
"label": "Last Name",
"autocomplete": "on"
},
"3": {
"id": "cf_email",
"name": "email",
"type": "text",
"label": "Email",
"autocomplete": "on"
},
"4": {
"id": "cf_phone",
"name": "phone",
"type": "text",
"label": "Phone",
"autocomplete": "on"
}
},
"expandable": false
}
],
"url": null,
"type": 3,
"status": 0,
"start_date": null,
"end_date": null,
"fieldKeys": [
"First Name",
"Last Name",
"Phone"
],
"is_public": false,
"custom_url": null,
"position_id": null,
"organization_id": 2,
"is_editable": false,
"created_at": "2018-11-29 02:07:30+05:30",
"updated_at": "2018-11-29 02:07:30+05:30"
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
name | text | The name of the Form |
structure | jsonb | The structure of the Form in Json format |
url | text | The url of for the Form |
type | smallint | The type of Form. Example: Evaluation Form, Feedback From etc. The Form Type are listed below |
status | smallint | The status of the Form |
start_date | timestamptz | The date and time from when the Form is active |
end_date | timestamptz | The date and time from when the Form is deactivate |
fieldKeys | jsonb | It describes the required field in the form and is in Json format |
is_public | boolean | States whether the form is public or not |
custom_url | text | The url for the custom Forms |
organization_id | integer | The id of the Organization |
is_editable | boolean | States whether the Form is editable or not |
List of Form Types:
Form Types | Key | Description |
---|---|---|
Web | 1 | Web Form |
Evaluation | 2 | Evaluation Form |
Assessment Candidate | 3 | Assessment Candidate Form |
Feedback | 4 | Feedback Form |
Requisition | 5 | Requisition Form |
Assessment Feedback | 6 | Assessment Feedback Form |
Fitment | 7 | Fitment Form |
Background Check Submission | 8 | Background Check Submission Form |
Candidate Verification | 9 | Candidate Verification Form |
On Boarding | 10 | On Boarding Form |
Offer Prepare | 11 | Offer Prepare Form |
Offer Candidate | 12 | Offer Candidate Form |
Offer Make | 13 | Offer Make Form |
Background Check Info Request | 14 | Background Check Info Request Form |
On Boarding User | 15 | On Boarding User Form |
On Boarding Candidate | 16 | On Boarding Candidate Form |
Candidate Data | 17 | Candidate Data Form |
Custom Assessment Section Candidate | 18 | Custom Assessment Section Candidate Form |
List all Forms
GET /models/form HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 6179,
"name": "21321",
"structure": [],
"url": null,
"type": 3,
"status": 0,
"start_date": null,
"end_date": null,
"is_public": false,
"article_id": null,
"custom_url": null,
"position_id": null,
"organization_id": 488,
"is_editable": false
}
]
This endpoint returns the details of all forms
HTTP Request
GET https://apiv4.talview.com/models/form
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
name | text | false | The name of the Form |
url | text | false | The url of for the Form |
type | smallint | false | The type of Form. Example: Evaluation Form, Feedback From etc. The Form Type are listed below |
is_public | boolean | false | States whether the form is public or not |
article_id | integer | false | The id of the CMS [Article] |
custom_url | text | false | The url for the custom Forms |
is_editable | boolean | false | States whether the Form is editable or not |
Retrieve a Form
GET /models/form/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 6179,
"name": "21321",
"structure": [],
"url": null,
"type": 3,
"status": 0,
"start_date": null,
"end_date": null,
"is_public": false,
"article_id": null,
"custom_url": null,
"position_id": null,
"organization_id": 488,
"is_editable": false
}
This endpoint retrieves a specific form.
HTTP Request
GET https://apiv4.talview.com/models/form/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on id |
Create a Form
POST /models/form HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 8369,
"name": "609565",
"structure": [
{
"id": 1,
"name": "Assessment form",
"fields": {
"1": {
"id": "cf_fn_name",
"name": "fname",
"type": "text",
"label": "First Name",
"autocomplete": "on"
},
"2": {
"id": "cf_ln_name",
"name": "lname",
"type": "text",
"label": "Last Name",
"autocomplete": "on"
},
"3": {
"id": "cf_email",
"name": "email",
"type": "text",
"label": "Email",
"autocomplete": "on"
},
"4": {
"id": "cf_phone",
"name": "phone",
"type": "text",
"label": "Phone",
"autocomplete": "on"
}
},
"expandable": false
}
],
"url": null,
"type": 3,
"status": 0,
"start_date": null,
"end_date": null,
"fieldKeys": [
"First Name",
"Last Name",
"Phone"
],
"is_public": false,
"custom_url": null,
"position_id": null,
"organization_id": 2,
"is_editable": false,
"created_at": "2018-11-29 10:47:18+05:30",
"updated_at": "2018-11-29 10:47:18+05:30"
}
This endpoint creates a form.
HTTP Request
POST https://apiv4.talview.com/models/form
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
type | integer | true | The type of the Form |
name | text | true | The name of the Form |
Update a Form
PUT /models/form/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 8369,
"name": "609565",
"structure": [
{
"id": 1,
"name": "Assessment form",
"fields": {
"1": {
"id": "cf_fn_name",
"name": "fname",
"type": "text",
"label": "First Name",
"autocomplete": "on"
},
"2": {
"id": "cf_ln_name",
"name": "lname",
"type": "text",
"label": "Last Name",
"autocomplete": "on"
},
"3": {
"id": "cf_email",
"name": "email",
"type": "text",
"label": "Email",
"autocomplete": "on"
},
"4": {
"id": "cf_phone",
"name": "phone",
"type": "text",
"label": "Phone",
"autocomplete": "on"
}
},
"expandable": false
}
],
"url": null,
"type": 3,
"status": 0,
"start_date": null,
"end_date": null,
"fieldKeys": [
"First Name",
"Last Name",
"Phone"
],
"is_public": false,
"custom_url": null,
"position_id": null,
"organization_id": 2,
"is_editable": false,
"created_at": "2018-11-29 10:47:18+05:30",
"updated_at": "2018-11-29 10:47:18+05:30"
}
This endpoint updates a specific form.
HTTP Request
PUT https://apiv4.talview.com/models/form/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
type | integer | false | The type of the Form |
name | text | false | The name of the Form |
Delete a Form
DELETE /models/form/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This endpoint deletes a specific form.
HTTP Request
DELETE https://apiv4.talview.com/models/form/<id>
Form Instance
The endpoint Form Instance gives the details of the instances of Form.
The Form Instance Object
{
"id": 447513,
"status": 500,
"evaluation_id": null,
"form_id": 8502,
"data": [
{
"key": "trdp_live_test",
"name": "FirstName",
"label": "First Name",
"value": "trdp_live_test"
},
{
"key": "sderfgy",
"name": "LastName",
"label": "Last Name",
"value": "sderfgy"
},
{
"key": "1598746325",
"name": "Phone",
"label": "Phone",
"value": "1598746325"
}
],
"assessment_section_id": 2652891,
"assessment_candidate_id": 1556430,
"assessment_section_candidate_id": 1696124,
"created_at": "2019-01-11 09:29:01+00",
"updated_at": "2019-01-11 09:29:01+00",
"_links": {
"file": {
"href": "/models/file?form_instance_id=447513"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
status | smallint | The status of the Form instance. Example: Draft, Complete. The status of the Form are listed below |
evaluation_id | integer | The Id of the Evaluation |
form_id | integer | The unique Id of the Form |
data | jsonb | The data in the form instance |
assessment_section_id | integer | The Id of the Assessment Section |
assessment_candidate_id | integer | The Id of the Assessment Candidate |
assessment_section_candidate_id | integer | The Id of the Assessment Section Candidate |
List of Form Status:
Status | Key | Description |
---|---|---|
Draft | 100 | Form is Drafted |
Complete | 500 | Form is Complete |
Late Complete | 600 | The form has been completed after the scheduled time |
Incomplete | 1000 | Form is incomplete |
List all Form Instances
GET /models/form-instance HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 447513,
"status": 500,
"evaluation_id": null,
"form_id": 8502,
"data": [
{
"key": "trdp_live_test",
"name": "FirstName",
"label": "First Name",
"value": "trdp_live_test"
},
{
"key": "sderfgy",
"name": "LastName",
"label": "Last Name",
"value": "sderfgy"
},
{
"key": "1598746325",
"name": "Phone",
"label": "Phone",
"value": "1598746325"
}
],
"assessment_section_id": 2652891,
"assessment_candidate_id": 1556430,
"assessment_section_candidate_id": 1696124,
"created_at": "2019-01-11 09:29:01+00",
"updated_at": "2019-01-11 09:29:01+00",
"_links": {
"file": {
"href": "/models/file?form_instance_id=447513"
}
}
}
...
]
Returns the details of all forms instances.
HTTP Request
GET https://apiv4.talview.com/models/form-instance
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
form_id | integer | false | Filter records based on Form Id |
assessment_section_id | integer | false | Filter records based on the Id of the Assessment Section |
assessment_candidate_id | integer | false | Filter records based on the Id id of the Assessment Candidate |
assessment_section_candidate_id | integer | false | Filter records based on the Id id of the Assessment Section Candidate |
status | smallint | false | Filter records based on Status Id |
Retrieve a Form Instance
GET /models/form-instance/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 447513,
"status": 500,
"evaluation_id": null,
"form_id": 8502,
"data": [
{
"key": "trdp_live_test",
"name": "FirstName",
"label": "First Name",
"value": "trdp_live_test"
},
{
"key": "sderfgy",
"name": "LastName",
"label": "Last Name",
"value": "sderfgy"
},
{
"key": "1598746325",
"name": "Phone",
"label": "Phone",
"value": "1598746325"
}
],
"assessment_section_id": 2652891,
"assessment_candidate_id": 1556430,
"assessment_section_candidate_id": 1696124,
"created_at": "2019-01-11 09:29:01+00",
"updated_at": "2019-01-11 09:29:01+00",
"_links": {
"file": {
"href": "/models/file?form_instance_id=447513"
}
}
}
This endpoint retrieves a specific form instance.
HTTP Request
GET https://apiv4.talview.com/models/form-instance/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Form Instance File
This endpoint gives the details of the Form Instance File.
The Form Instance File Object
{
"id": 34,
"key": "candidate-resume1",
"form_instance_id": 239849,
"file_id": 8050752,
"created_at": "2018-03-01 01:48:39+05:30",
"created_by": 1,
"updated_at": "2018-03-01 01:48:39+05:30",
"updated_by": 1
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
key | text | The key value to map the file attachment with the Form |
form_instance_id | Integer | The id of the Form Instance |
file_id | Integer | The id of the File |
created_by | Integer | The id of the User who created the Form Instance File |
updated_by | Integer | The id of the User who updated the Form Instance File |
List all Form Instance File
GET /models/form-instance-file HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 34,
"key": "candidate-resume1",
"form_instance_id": 239849,
"file_id": 8050752,
"created_at": "2018-03-01 01:48:39+05:30",
"created_by": 1,
"updated_at": "2018-03-01 01:48:39+05:30",
"updated_by": 1
}
{
"id": 33,
"key": "candidate-resume",
"form_instance_id": 239849,
"file_id": 8050751,
"created_at": "2018-03-01 01:48:39+05:30",
"created_by": 1,
"updated_at": "2018-03-01 01:48:39+05:30",
"updated_by": 1
}
...
]
This endpoint returns all the details of form instance file.
HTTP Request
GET https://apiv4.talview.com/models/form-instance-file
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
form_instance_id | Integer | false | Filter records based on the Id of Form Instance |
file_id | Integer | false | Filter records based on the Id of File |
Retrieve a Form Instance File
GET /models/form-instance-file/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 2951,
"key": "photo_file",
"form_instance_id": 268678,
"file_id": 9186916,
"created_at": "2018-07-07 15:41:54+05:30",
"created_by": 1,
"updated_at": "2018-07-07 15:41:54+05:30",
"updated_by": 1
}
This endpoint retrieves a specific form instance file.
HTTP Request
GET https://apiv4.talview.com/models/form-instance-file/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records bases on Id |
Update a Form Instance File
PUT /models/form-instance-file/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 3664,
"key": "Photo",
"form_instance_id": 290959,
"file_id": 9573897,
"created_at": "2018-08-07 08:45:30+00",
"created_by": 1,
"updated_at": "2018-08-07 08:45:30+00",
"updated_by": 1
}
This endpoint updates the specified form instance file.
HTTP Request
PUT https://apiv4.talview.com/models/form-instance-file/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
key | text | false | The key value of the Form Instance File |
form_instance_id | Integer | false | The id of the Form Instance |
file_id | Integer | false | The id of the File |
Delete a Form Instance File
DELETE /models/form-instance-file<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns the status code : 204 No Content
This endpoint deletes the specific form instance file.
HTTP Request
DELETE https://apiv4.talview.com/models/form-instance-file/<id>
Live Attendee
The Live Attendee endpoint gives the details of all the Live Attendee.( Live Attendee's are Candidate, Panel Members and the Organizer).
The Live Attendee Object
{
"id": 7233,
"live_session_id": 2324,
"email": "kumar.k+1@talview.com",
"phone": null,
"status": null,
"role": 1,
"candidate_id": 1825664,
"organization": {
"id": 2,
"name": "Talview",
"logo": "https://assets.talview.com/images/talview_grey.png",
"termsLink": "https://pages.talview.com/terms/"
},
"c": "7~fSFVG9iNEMzj5KRtf4WjE1NjlmZjk1YTEyYTU1ZjY3YjNjMGFlNDAzNzQ1ZmNkY2ZlYzQ5MmVkYmMxZWUxN2Y5YWUxNTYwZDRmMTNkNGUe62azfk2tzGAiJx3zIJGN3vIXAPBiMBE-oFXceNuM6A__",
"fullName": "kumar.k+1@talview.com",
"joined_at": "2018-04-12 13:45:32+00",
"user_id": null,
"left_at": "2018-04-12 13:45:32+00",
"passcode": "764873",
"joinUrl": "https://attend.talview.com/live-session/invite?c=D5rA3FvGxd3WeYW6zxakHWQ0M2M4NjRkMmFiN2NjNzJmYTk4ZWEzYWU1MjRmYjg1NmYwZjc0MjhkMWExMzI3MTE1YmVkYTU5ZjAxZTQ3YWThZuLfM-HiryuDxRAUQNG62hYnnm1XTakHtiaMaiFwlQ__",
"created_by": null,
"updated_by": null,
"created_at": "2018-04-12 13:45:31+00",
"theme": "default",
"skin": null,
"updated_at": "2018-04-12 13:45:31+00",
"_links": {
"liveAttendeeFlash": {
"href": "/models/live-attendee-flash/7233"
},
"liveSession": {
"href": "/models/live-session/2324"
},
"candidate": {
"href": "/models/candidate/1825664"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
live_session_id | integer | The Id for the Live Session |
text | The email of the Live Attendee | |
phone | text | The phone number of the Live Attendee |
status | integer | The status of the Live Session. Example: Scheduled, In Progress. The available Status are listed below |
role | integer | The role of the Live Attendee |
candidate_id | integer | The id of the Candidate |
organization | object | The details of the Organization |
postAssessmentUrl | string | The url for redirection after Live Session |
fullName | string | The full name of the Attendee |
joined_at | timestamptz | The time of joining the Live Session |
user_id | integer | The Id of the User who scheduled the Live Session |
left_at | timestamptz | The time when the Candidate left the Live Session |
joinUrl | string | The url for joining the Live Session |
created_by | integer | The id of the Organizer who created the live session |
updated_by | integer | The id of the Organizer who updated the live session |
created_at | timestamptz | The time when the live assessment was created |
theme | string | The theme for the Application |
updated_at | timestamptz | The time when the Live Session was updated |
List of Roles:
Roles | Key | Description |
---|---|---|
Participant | 1 | The participant of the Live Session |
Moderator | 2 | The moderator of the Live Session |
Organizer | 3 | The organizer of the Live Session |
Status of Live Session:
Staus | Key | Description |
---|---|---|
Scheduled | 1 | The Live Session is scheduled |
In Progress | 2 | The Live Session is in progress |
Completed | 3 | The Live Session is Completed |
Cancelled | 10 | The Live Session is Cancelled |
No Show | 11 | The Live Session is Scheduled but the Live Attendee has not joined the session |
List all Live Attendee
GET /models/live-attendee HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 7233,
"live_session_id": 2324,
"email": "kumar.k+1@talview.com",
"phone": null,
"status": null,
"role": 1,
"candidate_id": 1825664,
"organization": {
"id": 2,
"name": "Talview",
"logo": "https://assets.talview.com/images/talview_grey.png",
"termsLink": "https://pages.talview.com/terms/"
},
"c": "7~fSFVG9iNEMzj5KRtf4WjE1NjlmZjk1YTEyYTU1ZjY3YjNjMGFlNDAzNzQ1ZmNkY2ZlYzQ5MmVkYmMxZWUxN2Y5YWUxNTYwZDRmMTNkNGUe62azfk2tzGAiJx3zIJGN3vIXAPBiMBE-oFXceNuM6A__",
"fullName": "kumar.k+1@talview.com",
"joined_at": "2018-04-12 13:45:32+00",
"user_id": null,
"left_at": "2018-04-12 13:45:32+00",
"passcode": "764873",
"joinUrl": "https://attend.talview.com/live-session/invite?c=D5rA3FvGxd3WeYW6zxakHWQ0M2M4NjRkMmFiN2NjNzJmYTk4ZWEzYWU1MjRmYjg1NmYwZjc0MjhkMWExMzI3MTE1YmVkYTU5ZjAxZTQ3YWThZuLfM-HiryuDxRAUQNG62hYnnm1XTakHtiaMaiFwlQ__",
"created_by": null,
"updated_by": null,
"created_at": "2018-04-12 13:45:31+00",
"theme": "default",
"skin": null,
"updated_at": "2018-04-12 13:45:31+00",
"_links": {
"liveAttendeeFlash": {
"href": "/models/live-attendee-flash/7233"
},
"liveSession": {
"href": "/models/live-session/2324"
},
"candidate": {
"href": "/models/candidate/1825664"
}
}
}
]
This endpoint returns the details of all live attendee paginated by page.
HTTP Request
GET https://apiv4.talview.com/models/live-attendee
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
live_session_id | Integer | false | Filter records based on the Id of the Live Session |
text | false | Filter records based on email | |
phone | text | false | Filter records based on Phone number |
role | integer | false | Filter records based on Role. The Roles are listed below. |
Retrieve a Live Attendee
GET /models/live-attendee/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 204,
"live_session_id": 66,
"email": "s*******.talview@talview.com",
"phone": null,
"status": null,
"role": 2,
"candidate_id": null,
"organization": {
"id": 183,
"name": "Talview Internal Hiring",
"logo": "https://assets.talview.com/images/talview_grey.png",
"termsLink": "https://pages.talview.com/terms/"
},
"c": "3~5qwBfYHqMIM1OGo8IMZWQ1ZDlkNDVhNjY5N2QzYzE1NWZmNDQ0NWUwOGQ1NzlhOWJhYWUwM2RlNzcyOWQ1NDVmMWVjYzE4ZDA5M2MyZmYZbj9JJj3~vtpZs4ouuoi~iGanacH4lNjW9kejfDptaA__",
"fullName": "Jhon",
"joined_at": "2017-11-29 04:47:33+00",
"user_id": 26304,
"left_at": "2017-11-29 04:47:33+00",
"passcode": "137283",
"joinUrl": "https://attend.talview.com/live-session/invite?c=PKlYUIf3ihCAVavALlzE7mZhNWVjMjRhYTQ0MTVlYjllOWVhOTEwYzQ3N2EyYjg3MzlhYmZhOGE5ZDM5NjIzODAxYmQwZjY4NjBmYzVlZDkzeQPL72GqRkNsL9XpQ1UrbX6ZCcZElLJnRMqmEAVrIw__",
"created_by": 26304,
"updated_by": 26304,
"created_at": "2017-11-29 04:47:32+00",
"theme": "default",
"skin": null,
"updated_at": "2017-11-29 04:47:32+00",
"_links": {
"liveAttendeeFlash": {
"href": "/models/live-attendee-flash/204"
},
"liveSession": {
"href": "/models/live-session/66"
},
"candidate": {
"href": "/models/candidate/386619"
}
}
}
This end point retrieves a details of an existing live attendee.
HTTP Request
GET https://apiv4.talview.com/models/live-attendee/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Update a Live Attendee
PUT /models/live-attendee/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 181507,
"live_session_id": 58564,
"email": "testing@talview.com",
"phone": null,
"status": 2,
"role": 2,
"candidate_id": null,
"organization": {
"id": 488,
"name": "testing",
"logo": "https://assets.talview.com/clients/488/1513147530_logo.png",
"termsLink": "https://pages.talview.com/terms/"
},
"postAssessmentUrl": null,
"c": "46Q~EO5niHxQ40Qnkbr8~DAwY2ZkMTlmYjQ4OTE3MTExNzA0Y2FlYmEwM2NlZTRjMzI0NzJkMjViODVmOTNmZDdkM2ZkOWE1N2RkOGEwMTAIxlG3UhQqvaGH8CKTRimnn8D4zonhD~anICequ9ukFw__",
"fullName": "Dev Testing",
"joined_at": "2019-01-15 11:13:12+00",
"user_id": 30876,
"left_at": "2019-01-15 11:13:12+00",
"passcode": "673991821",
"joinUrl": "https://attend.talview.com/live-session/invite?c=Z2ZUfn50yLrBm2EuKAwhNGI3MWY1ZWM1NGNkNWJmN2Q5MzE2NmE0NTFiZmE5MGE2OTViNmRmYTI3NTQ2NTBhMDAwMDI1YTJlMWFhODZjMGMiVlQCugIcYdZWqUoMQuA301pXQZ3xApA-wIxn0L3IaA__",
"created_by": 30876,
"updated_by": 30876,
"created_at": "2019-01-15 11:13:12+00",
"theme": "default",
"skin": null,
"updated_at": "2019-01-15T13:18:23+00:00",
"_links": {
"liveAttendeeFlash": {
"href": "/models/live-attendee-flash/181507"
},
"liveSession": {
"href": "/models/live-session/58564"
},
"candidate": {
"href": "/models/candidate/5735265"
}
}
}
Modify the existing details of a live attendee.
HTTP Request
PUT https://apiv4.talview.com/models/live-attendee/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
Id | integer | True | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
live_session_id | false | integer | The Id for the Live Session |
text | false | The email of the Live Attendee | |
status | integer | false | The status of the Live Session |
Live Attendee Flash
The Live Attendee Flash endpoint gives the url for Joining the Live Session through Flash. This is for the browser which does not support Webrtc.
The Live Attendee Flash Object
{
"id": 275129,
"flashJoinUrl": null,
"created_at": "2019-03-12 13:24:42+00",
"updated_at": "2019-03-12 13:48:55+00",
"_links": {
"liveAttendeeFlash": {
"href": "/models/live-attendee-flash/275129"
},
"liveSession": {
"href": "/models/live-session/88570"
},
"candidate": {
"href": "/models/candidate/6507897"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
flashJoinUrl | string | The url for taking the Live Session through Flash |
List All Live Attendee Flash
GET /models/live-attendee-flash HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 160555,
"flashJoinUrl": "https://live101.talview.com/bigbluebutton/api/join?meetingID=493888504&fullName=amith+patel&password=M740272270&checksum=0b47d2915c568eae0b4d3915071d675f456d5846",
"_links": {
"liveAttendeeFlash": {
"href": "/models/live-attendee-flash/160555"
},
"liveSession": {
"href": "/models/live-session/51756"
},
"candidate": {
"href": "/models/candidate/5613827"
}
}
}
]
This endpoint returns all the details of the live attendee flash
HTTP Request
GET https://apiv4.talview.com/models/live-attendee-flash
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
live_session_id | false | integer | Filter records based on the Id of Live Session |
text | false | Filter records based on Email of Live Attendee |
Retrieve a Live Attendee Flash
GET /models/live-attendee-flash/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 181367,
"flashJoinUrl": "https://live101.talview.com/bigbluebutton/api/join?meetingID=858471012&fullName=bindu+Kanni&password=M110215397&checksum=7d84209e3285315af5d14abe5cb2d74056f5f520",
"_links": {
"liveAttendeeFlash": {
"href": "/models/live-attendee-flash/181367"
},
"liveSession": {
"href": "/models/live-session/58518"
},
"candidate": {
"href": "/models/candidate/5541725"
}
}
}
This endpoint retrieves the details of an existing live attendee flash.
HTTP Request
GET https://apiv4.talview.com/models/live-attendee-flash/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Update a Live Attendee Flash
PUT /models/live-attendee-flash/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 181367,
"flashJoinUrl": "https://live101.talview.com/bigbluebutton/api/join?meetingID=858471012&fullName=bindu+Kanni&password=M110215397&checksum=7d84209e3285315af5d14abe5cb2d74056f5f520",
"_links": {
"liveAttendeeFlash": {
"href": "/models/live-attendee-flash/181367"
},
"liveSession": {
"href": "/models/live-session/58518"
},
"candidate": {
"href": "/models/candidate/5541725"
}
}
}
This endpoint Updates the details of an existing live attendee flash.
HTTP Request
PUT https://apiv4.talview.com/models/live-attendee-flash/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
live_session_id | integer | false | The Id for the Live Session |
text | false | The email of the Live Attendee |
Live Session
The Live Session model gives the details of the Live Session created by the Organizer for the Candidate. Live Attendee are associated with Live Session.
The Live Session Object
{
"id": 1665,
"name": "612791",
"is_record": false,
"description": null,
"status": 1,
"starts_at": "2018-03-21 20:30:00+00",
"ends_at": "2018-03-21 21:30:00+00",
"duration": "01:00:00",
"timezone": "Asia/Kolkata",
"assessment_section_candidate_id": null,
"recording_status": null,
"hosted_by": 36209,
"version": 2,
"domain": "meet.talview.com",
"created_at": "2018-03-21 14:38:28+00",
"updated_at": "2018-03-21 14:38:28+00",
"created_by": 36209,
"updated_by": 36209,
"_links": {
"assessmentSectionCandidate": {
"href": "/models/assessment-section-candidate"
},
"liveRecordingFlash": {
"href": "/models/live-session-flash-recording?live_session_id=1665"
},
"webRTCRecordings": {
"href": "/models/file?live_session_id=1665&file_type_id=41"
},
"liveAttendees": {
"href": "/models/live-attendee?live_session_id=1665"
}
}
},
{
...
},
{
...
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
name | string | The name of the Live Session |
is_record | boolean | Flag to check whether live session recording start/stop feature is enabled for a live-session |
description | string | The description of the Live Session |
status | integer | The status of Live Session. Example: Scheduled, In Progress. The status of Live Session are listed in the table below |
location | string | The location where the live interview is scheduled. Used in offline Live Interview |
ends_at | timestamptz | The time when the Live Session ends |
duration | timestamptz | The duration of the Live Session |
timezone | timestamptz | The timezone of Live Attendee |
assessment_section_candidate_id | integer | The id of the Assessment Section Candidate |
recording_status | integer | The status of the Live Session Recording. Example: Disabled, Processing. The status of the recording status are listed in the table below. |
hosted_by | integer | The id of the Organizer who hosted the Live Session |
domain | string | The URl generated for Live Attendee to redirect to Live Session window |
created_by | integer | The id of the User who created the Live Session |
updated_by | integer | The id of the User who updated the Live Session |
List of Live Session Status :
Status | Key | Description |
---|---|---|
Scheduled | 1 | A Live Session event has been scheduled |
In Progress | 2 | Live Session event is in progress |
Complete | 3 | Live Session event is complete |
Cancelled | 10 | Live Session event has been cancelled |
Panel No Show | 11 | Panel is not online |
Candidate No Show | 12 | Candidate is not online |
Both No Show | 13 | Both Panel and Candidate are not Online |
List of Recording Status :
Status | Key | Description |
---|---|---|
Disabled | 1 | Recording id disabled |
Processing | 2 | Recording is processing |
Available | 3 | Recording is available |
Error | 4 | Error in recording |
List all Live Session
GET /models/live-session HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 1665,
"name": "612791",
"is_record": false,
"description": null,
"status": 1,
"starts_at": "2018-03-21 20:30:00+00",
"ends_at": "2018-03-21 21:30:00+00",
"duration": "01:00:00",
"timezone": "Asia/Kolkata",
"assessment_section_candidate_id": null,
"recording_status": null,
"hosted_by": 36209,
"domain": "meet.talview.com",
"created_at": "2018-03-21 14:38:28+00",
"updated_at": "2018-03-21 14:38:28+00",
"created_by": 36209,
"updated_by": 36209,
"_links": {
"assessmentSectionCandidate": {
"href": "/models/assessment-section-candidate"
},
"liveRecordingFlash": {
"href": "/models/live-session-flash-recording?live_session_id=1665"
},
"webRTCRecordings": {
"href": "/models/file?live_session_id=1665&file_type_id=41"
},
"liveAttendees": {
"href": "/models/live-attendee?live_session_id=1665"
}
}
},
{
...
},
{
...
}
]
This endpoint returns all the details of live sessions
HTTP Request
GET https://apiv4.talview.com/models/live-session
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
assessment_section_candidate_id | integer | false | Filter records based on Id of the Assessment Section Candidate |
name | string | false | Filter records based on the name of Live Session |
is_record | boolean | false | Filter records based on whether the Live Session is recorded or not |
description | string | false | Filter records based on the description of Live Session |
ends_at | timestamptz | false | Filter records based on the time when the Live Session ends |
recording_status | integer | false | Filter records based on the status of the Live Session |
hosted_by | integer | false | Filter records based on the Id of the Organizer who hosted the Live Session |
Retrieve a Live Session
GET /models/live-session/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1665,
"name": "612791",
"is_record": false,
"description": null,
"status": 1,
"starts_at": "2018-03-21 20:30:00+00",
"ends_at": "2018-03-21 21:30:00+00",
"duration": "01:00:00",
"timezone": "Asia/Kolkata",
"assessment_section_candidate_id": null,
"recording_status": null,
"hosted_by": 36209,
"domain": "meet.talview.com",
"created_at": "2018-03-21 14:38:28+00",
"updated_at": "2018-03-21 14:38:28+00",
"created_by": 36209,
"updated_by": 36209,
"_links": {
"assessmentSectionCandidate": {
"href": "/models/assessment-section-candidate"
},
"liveRecordingFlash": {
"href": "/models/live-session-flash-recording?live_session_id=1665"
},
"webRTCRecordings": {
"href": "/models/file?live_session_id=1665&file_type_id=41"
},
"liveAttendees": {
"href": "/models/live-attendee?live_session_id=1665"
}
}
}
This endpoint retrieves the details of an live session.
HTTP Request
GET https://apiv4.talview.com/models/live-session/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | array | true | Filter records based on Id |
Menu Card
The Menu Card endpoint gives all the details of Menu Card. Menu Card is the collection of Assessments within a Category
The Menu Card Object
{
"id": 83,
"is_private": false,
"name": "Co******te",
"imageUrl": "https://assets.talview.com/images/***********************.jpg",
"description": null,
"starts_at": "2018-10-27 17:21:13+05:30",
"code": "3990756",
"ends_at": "2018-10-27 17:21:13+05:30",
"organization_id": 567,
"created_by": 1,
"updated_by": 1,
"created_at": "2018-10-27 17:21:13+05:30",
"updated_at": "2018-10-27 17:21:13+05:30",
"_links": {
"candidates": {
"href": "/models/candidate?menu_card_id=83"
},
"assessments": {
"href": "/models/assessment?menu_card_id=83"
},
"sources": {
"href": "/models/source?menu_card_id=83"
},
"menuCardCandidates": {
"href": "/models/menu-card-candidate?menu_card_id=83"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
is_private | boolean | Status whether Menu Card is private or not |
name | string | The name of the Menu Card |
imageUrl | string | The url for the Organization logo |
description | string | The description of the Menu Card Assessment |
starts_at | timestamptz | The date and time from when the Menu Card Assessment is active |
code | integer | |
ends_at | timestamptz | The date and time when the Menu Card expires |
organization_id | integer | The id of the Organization for whom the Menu Card belongs to |
created_by | integer | The id of User who created the Menu Card |
updated_by | integer | The id of the User who updated the Menu Card |
List Menu Card
GET /models/menu-card HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 83,
"is_private": false,
"name": "Co******te",
"imageUrl": "https://assets.talview.com/images/***********************.jpg",
"description": null,
"starts_at": "2018-10-27 17:21:13+05:30",
"code": "3990756",
"ends_at": "2018-10-27 17:21:13+05:30",
"organization_id": 567,
"created_by": 1,
"updated_by": 1,
"created_at": "2018-10-27 17:21:13+05:30",
"updated_at": "2018-10-27 17:21:13+05:30",
"_links": {
"candidates": {
"href": "/models/candidate?menu_card_id=83"
},
"assessments": {
"href": "/models/assessment?menu_card_id=83"
},
"sources": {
"href": "/models/source?menu_card_id=83"
},
"menuCardCandidates": {
"href": "/models/menu-card-candidate?menu_card_id=83"
}
}
}
This endpoint returns all the details of the menu cards.
HTTP Request
GET https://apiv4.talview.com/models/menu-card
Query Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
is_private | boolean | false | Filter records based on the status whether Menu Card is private or not |
name | string | false | Filter records based on the name of the Menu Card |
description | string | false | Filter records based on the description of the Menu Card Assessment |
Menu Card Assessment
The Menu Card Assessment is the mapping of Menu Card and the Assessment.
The Menu Card Assessment Object
{
"id": 435,
"created_by": 246812,
"assessment_id": 549037,
"created_at": "2018-12-27 02:18:22+05:30",
"updated_at": "2018-12-27 02:18:22+05:30",
"updated_by": 246812,
"menu_card_id": 81
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
assessment_id | integer | The Id of the Assessment |
created_at | timestamptz | The date and time of when the Menu Card Assessment was created |
updated_at | timestamptz | The date and time of when the Menu Card Assessment was updated |
menu_card_id | integer | The id of the menu card |
List all Menu Card Assessment
GET /models/menu-card-assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 435,
"created_by": 246812,
"assessment_id": 549037,
"created_at": "2018-12-27 02:18:22+05:30",
"updated_at": "2018-12-27 02:18:22+05:30",
"updated_by": 246812,
"menu_card_id": 81
},
{
"id": 430,
"created_by": 1,
"assessment_id": 626074,
"created_at": "2018-12-07 13:01:00+05:30",
"updated_at": "2018-12-07 13:01:00+05:30",
"updated_by": 1,
"menu_card_id": 80
},
....
]
This endpoint returns all the details of menu card assessment.
HTTP Request
GET https://apiv4.talview.com/models/menu-card-assessment
Query Parameters
Parameter | Type | mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
assessment_id | integer | false | Filter records based on the Id of the Assessment |
menu_card_id | integer | false | Filter records based on the Id of the Menu Card |
Create a new Menu Card Assessment
POST /models/menu-card-assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 782,
"created_by": 246812,
"assessment_id": 224885,
"created_at": "2019-02-19T11:58:54+00:00",
"updated_at": "2019-02-19T11:58:54+00:00",
"updated_by": 246812,
"menu_card_id": 195
}
This endpoint allows you to create a new menu card assessment.
HTTP Request
POST https://apiv4.talview.com/models/menu-card-assessment
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessment_id | integer | true | Id of an existing Assessment |
menu_card_id | integer | true | Id of an existing Menu Card |
Delete an existing Menu Card Assessment
DELETE /models/menu-card-assessment/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This endpoint allows you to delete an existing menu card assessment.
HTTP Request
DELETE https://apiv4.talview.com/models/menu-card-assessment/<id>
Notification Log
The Notification Log endpoint gives all the log details of the notification sent to the Candidate as well as the Panel.
The Notification Log object
{
"id": 2889368,
"to_address": "sahana.b+161612@talview.com",
"cc_address": null,
"assessment_id": 727354,
"bcc_address": null,
"content": "<p>Dear sahana.b+161612@talview.com,\n</p>....................................",
"subject": "Talview Dev Team Testing is inviting you to attend an assessment.",
"created_at": "2019-01-17 08:41:41+00",
"created_by": 1,
"sent_by": 1,
"notification_template_type_id": 210,
"updated_at": "2019-01-17 08:41:42+00",
"status": "sent",
"scheduled_at": "2019-01-17 08:41:39+00",
"sent_at": "2019-01-17 08:41:41+00",
"read_at": null
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
to_address | The email address of the User to whom the notification is to be sent | |
cc_address | CC address for the Notification | |
assessment_id | integer | The id of the Assessment |
bcc_address | BCC address for the Notification | |
content | text | The content of Notification |
subject | text | The subject of the Notification |
created_by | integer | The id of the user who Created the Notification |
sent_by | integer | The id of the User who sent the Notification |
notification_template_type_id | integer | The id of the Notification Template type |
status | integer | The status of the Notification Log. Example: Pending, Sent. The status are listed below. |
scheduled_at | timestamptz | The time when the Notification mail is triggered |
sent_at | timestamptz | The time when the the Notification was sent |
read_at | timestamptz | The time when the Notification mail was read by the receiver |
List of Event Types:
Status | Value | Description |
---|---|---|
Pending | enqueued | The notification mail is pending |
Sent | sent | The notification mail is sent |
Delivered | delivered | The notification mail is delivered |
Failed | failed | The notification mail is not sent |
Bounced | bounced | The notification mail is bounced |
Read | read | The notification mail has been read |
Archived | archived | The notification mail is archived |
Scheduled | scheduled | The notification mail is scheduled |
Deleted | deleted | The notification mail is deleted |
List all Notification Log
GET /models/notification-log HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 2889368,
"to_address": "sahana.b+161612@talview.com",
"cc_address": null,
"assessment_id": 727354,
"bcc_address": null,
"content": "<p>Dear sahana.b+161612@talview.com,\n</p>....................................",
"subject": "Talview Dev Team Testing is inviting you to attend an assessment.",
"created_at": "2019-01-17 08:41:41+00",
"created_by": 1,
"sent_by": 1,
"notification_template_type_id": 210,
"updated_at": "2019-01-17 08:41:42+00",
"status": "sent",
"scheduled_at": "2019-01-17 08:41:39+00",
"sent_at": "2019-01-17 08:41:41+00",
"read_at": null
},
{
}
....
]
This endpoint returns all the details of notification logs.
HTTP Request
GET https://apiv4.talview.com/models/notification-log
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on Id |
to_address | false | Filter records based on the email address of the User to whom the notification is to be sent | |
cc_address | false | Filter records based on CC address for the Notification | |
assessment_id | integer | false | Filter records based on the id of the Assessment |
bcc_address | false | Filter records based on BCC address for the Notification | |
subject | text | false | Filter records based on the subject of the Notification |
status | integer | false | Filter records based on the status of the Notification |
sent_at | timestamptz | false | Filter records based on the time when the the Notification was sent |
read_at | timestamptz | false | Filter records based on the time when the Notification mail was read by the receiver |
Notification Template
The Notification Template end point gives the details of the Notification Template. Notification Template is the template for sending notification mail to the Candidate and the Panel.
The Notification Template Object
{
"id": 26244,
"from_address": null,
"type_id": 219,
"content": "<p>Dear {%full-name},</p><p>{%panel-name} Evaluated the candidate {%candidate-name} ..............",
"subject": "{%panel-name} Evaluated the candidate {%candidate-name} for {%assessment-title}\n",
"category_id": null,
"organization_id": 488,
"assessment_id": 701909,
"created_at": "2019-01-07 06:16:53+00",
"updated_at": "2019-01-07 06:16:53+00",
"created_by": 67656,
"updated_by": 67656,
"_links": {
"tags": {
"href": "/models/notification-template-tag?type_id=219"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
from_address | The email address from where the notification will be triggered | |
type_id | integer | The Id of the Notification Template type |
content | text | The content of notification |
subject | text | The subject of the notification |
category_id | integer | The category Id of the notification |
organization_id | integer | The Id of the Organization |
assessment_id | integer | The Id of the Assessment |
created_by | integer | The Id of the User who created the Notification Template |
updated_by | integer | The Id of the user who updated the Notification Template |
List all Notification Template
GET /models/notification-template HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 26244,
"from_address": null,
"type_id": 219,
"content": "<p>Dear {%full-name},</p><p>{%panel-name} Evaluated the candidate {%candidate-name} ..............",
"subject": "{%panel-name} Evaluated the candidate {%candidate-name} for {%assessment-title}\n",
"category_id": null,
"organization_id": 488,
"assessment_id": 701909,
"created_at": "2019-01-07 06:16:53+00",
"updated_at": "2019-01-07 06:16:53+00",
"created_by": 67656,
"updated_by": 67656,
"_links": {
"tags": {
"href": "/models/notification-template-tag?type_id=219"
}
}
},
{
}
....
]
This endpoint returns all the details of the notification templates.
HTTP Request
GET https://apiv4.talview.com/models/notification-template
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on Id |
from_address | false | Filter records based on the email address from where the notification will be triggered | |
type_id | integer | false | Filter records based on the Id of the Notification Template type |
subject | text | false | Filter records based on the subject of the notification |
assessment_id | integer | false | Filter records based on the Id of the Assessment |
Retrieve a Notification Template
GET /models/notification-template/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 26244,
"from_address": null,
"type_id": 219,
"content": "<p>Dear {%full-name},</p><p>{%panel-name} Evaluated the candidate {%candidate-name} ..............",
"subject": "{%panel-name} Evaluated the candidate {%candidate-name} for {%assessment-title}\n",
"category_id": null,
"organization_id": 488,
"assessment_id": 701909,
"created_at": "2019-01-07 06:16:53+00",
"updated_at": "2019-01-07 06:16:53+00",
"created_by": 67656,
"updated_by": 67656,
"_links": {
"tags": {
"href": "/models/notification-template-tag?type_id=219"
}
}
}
This endpoint lists a specific notification template.
HTTP Request
GET https://apiv4.talview.com/models/notification-template/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create Notification Template
POST /models/notification-template HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 25815,
"from_address": null,
"type_id": 219,
"content": "456123",
"subject": "123456",
"category_id": null,
"organization_id": 488,
"assessment_id": 678278,
"created_at": "2019-01-17T11:27:40+00:00",
"updated_at": "2019-01-17T11:27:40+00:00",
"created_by": 30876,
"updated_by": 30876,
"_links": {
"tags": {
"href": "/models/notification-template-tag?type_id=219"
}
}
}
This endpoint create a notification template.
HTTP Request
POST https://apiv4.talview.com/models/notification-template
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
type_id | integer | true | Type Id of the Notification Template |
content | text | true | Content of Notification |
subject | text | true | Subject of Notification |
Update Notification Template
PUT /models/notification-template/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 25816,
"from_address": null,
"type_id": 219,
"content": "456131",
"subject": "123457",
"category_id": null,
"organization_id": 488,
"assessment_id": 678278,
"created_at": "2019-01-17 17:06:37+05:30",
"updated_at": "2019-01-17 17:10:29+05:30",
"created_by": 30876,
"updated_by": 30876,
"_links": {
"tags": {
"href": "/models/notification-template-tag?type_id=219"
}
}
}
This endpoint update a specific notification template.
HTTP Request
PUT https://apiv4.talview.com/models/notification-template/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
content | text | false | Content of Notification |
subject | text | false | Subject of Notification |
Delete Notification Template
Deletes the specific notification template.
DELETE /models/notification-template/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
HTTP Request
DELETE https://apiv4.talview.com/models/notification-template/<id>
Notification Template Tag
The Notification Template Tag endpoint gives the detail of all the Tags that can be implemented in the Notification Template.
The Notification Template Tag Object
{
"id": 1221,
"tag": "{%assessment-name}",
"description": "Asessment Title",
"type_id": 264,
"created_at": "2018-10-16 14:45:22+05:30",
"updated_at": "2018-10-16 14:45:22+05:30",
"created_by": null,
"updated_by": null
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
tag | text | The tag. Example: {%assessment-name} |
description | text | The description of the Tag |
type_id | integer | The Id of the Notification Template Type |
List all Notification Template Tag
GET /models/notification-template-tag HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 1221,
"tag": "{%assessment-name}",
"description": "Asessment Title",
"type_id": 264,
"created_at": "2018-10-16 14:45:22+05:30",
"updated_at": "2018-10-16 14:45:22+05:30",
"created_by": null,
"updated_by": null
},
{
"id": 1220,
"tag": "{%live-session-link}",
"description": " Live Session Link",
"type_id": 210,
"created_at": "2018-08-06 17:14:39+05:30",
"updated_at": "2018-10-16 14:45:22+05:30",
"created_by": null,
"updated_by": null
},
{
}
....
]
This endpoint returns all the details of the notification template tags.
HTTP Request
GET https://apiv4.talview.com/models/notification-template-tag
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
tag | text | false | Filter records based on Tag name. |
description | text | false | Filter records based on the description of the Tag |
type_id | integer | false | Filter records based on the Id of the Notification Template Type |
Notification Template Type
The Notification Template Type endpoint gives the details of all the Notification Template available.
The Notification Template Type Object
{
"id": 286,
"label": "Simulation Booking",
"description": null,
"type_id": 286,
"created_at": "2018-08-06 16:20:51+05:30",
"updated_at": null,
"created_by": null,
"updated_by": null
},
{
"id": 628,
"label": "booking",
"description": "live session moderator booking",
"type_id": 628,
"created_at": "2017-11-20 17:19:33+05:30",
"updated_at": null,
"created_by": 1,
"updated_by": 1
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
label | text | The label of Notification Template |
description | text | The description of Notification Template |
type_id | integer | The Id Notification Template Type |
List all Notification Template Type
GET /models/notification-template-type HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 286,
"label": "Simulation Booking",
"description": null,
"type_id": 286,
"created_at": "2018-08-06 16:20:51+05:30",
"updated_at": null,
"created_by": null,
"updated_by": null
},
{
"id": 628,
"label": "booking",
"description": "live session moderator booking",
"type_id": 628,
"created_at": "2017-11-20 17:19:33+05:30",
"updated_at": null,
"created_by": 1,
"updated_by": 1
},
{
}
....
]
This endpoint returns all the details of notification template type.
HTTP Request
GET https://apiv4.talview.com/models/notification-template-type
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
description | text | false | Filter records based on the description of Notification Template |
type_id | integer | false | Filter records based on the Id of Notification Template Type |
Panel
The Panel endpoint gives the details of the Panel associated with Assessment Section Candidate. Apart form MCQ and MCA type Assessment Section Panel are assigned to all other Assessment Section.
The Panel Object
The panel object
{
"id": 19394,
"selectedCount": 1,
"rejectedCount": 0,
"holdCount": 0,
"evaluatedCount": 1,
"assignedCount": 7,
"assessment_section_id": 128233,
"user_id": 30876,
"created_at": "2017-11-03 19:54:20+00",
"updated_at": "2017-11-03 19:54:20+00",
"created_by": 30876,
"updated_by": 30876,
"_links": {
"self": {
"href": "/models/panel/19394"
},
"pending": {
"href": "/models/panel-candidate?evaluated=false&panel_id=19394"
},
"evaluated": {
"href": "/models/panel-candidate?evaluated=true&panel_id=19394"
},
"selected": {
"href": "/models/panel-candidate?recommendation=select&panel_id=19394"
},
"rejected": {
"href": "/models/panel-candidate?recommendation=reject&panel_id=19394"
},
"hold": {
"href": "/models/panel-candidate?recommendation=hold&panel_id=19394"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
selectedCount | integer | The number of Candidate selected by the Panel |
rejectedCount | integer | The number of Candidate rejected by the Panel |
holdCount | integer | The number of candidate Candidate that are kept in hold by the Panel |
evaluatedCount | integer | The number of Candidate evaluated by the Panel |
assignedCount | integer | The number of Candidate Assigned to the Panel |
assessment_section_id | integer | The Id of Assessment Section |
user_id | integer | The id of the User who assigned the Panel |
List all Panel
GET /models/panel HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 19394,
"selectedCount": 1,
"rejectedCount": 0,
"holdCount": 0,
"evaluatedCount": 1,
"assignedCount": 7,
"assessment_section_id": 128233,
"user_id": 30876,
"created_at": "2017-11-03 19:54:20+00",
"updated_at": "2017-11-03 19:54:20+00",
"created_by": 30876,
"updated_by": 30876,
"_links": {
"self": {
"href": "/models/panel/19394"
},
"pending": {
"href": "/models/panel-candidate?evaluated=false&panel_id=19394"
},
"evaluated": {
"href": "/models/panel-candidate?evaluated=true&panel_id=19394"
},
"selected": {
"href": "/models/panel-candidate?recommendation=select&panel_id=19394"
},
"rejected": {
"href": "/models/panel-candidate?recommendation=reject&panel_id=19394"
},
"hold": {
"href": "/models/panel-candidate?recommendation=hold&panel_id=19394"
}
},
}
]
Returns details of all the panel
HTTP Request
GET https://apiv4.talview.com/models/panel
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
assessment_section_id | integer | false | Filter records based on the Id of the Assessment Section |
assessment_id | integer | false | Filter records based on the Id of the Assessment |
user_id | integer | false | Filter records based on the Id of the user who created the panel |
Retrieve a Panel
GET /models/panel/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 19394,
"selectedCount": null,
"rejectedCount": null,
"holdCount": null,
"evaluatedCount": null,
"assignedCount": null,
"assessment_section_id": 128233,
"user_id": 30876,
"created_at": "2017-11-03 19:54:20+00",
"updated_at": "2017-11-03 19:54:20+00",
"created_by": 30876,
"updated_by": 30876,
"_links": {
"self": {
"href": "/models/panel/19394"
},
"pending": {
"href": "/models/panel-candidate?evaluated=false&panel_id=19394"
},
"evaluated": {
"href": "/models/panel-candidate?evaluated=true&panel_id=19394"
},
"selected": {
"href": "/models/panel-candidate?recommendation=select&panel_id=19394"
},
"rejected": {
"href": "/models/panel-candidate?recommendation=reject&panel_id=19394"
},
"hold": {
"href": "/models/panel-candidate?recommendation=hold&panel_id=19394"
}
}
}
Retrieves the details of an existing panel.
HTTP Request
GET https://apiv4.talview.com/models/panel/<id>
URL Parameters
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
Create new Panel
POST /models/panel HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Request:
{
"assessment_section_id": 128233,
"user_id": 30876
}
The above command returns JSON structured like this:
{
"id": 375582,
"selectedCount": null,
"rejectedCount": null,
"holdCount": null,
"evaluatedCount": null,
"assignedCount": null,
"assessment_section_id": 128233,
"user_id": 30876,
"created_at": "2019-01-12T13:18:26+00:00",
"updated_at": "2019-01-12T13:18:26+00:00",
"created_by": 30876,
"updated_by": 30876,
"_links": {
"self": {
"href": "/models/panel/375582"
},
"pending": {
"href": "/models/panel-candidate?evaluated=false&panel_id=375582"
},
"evaluated": {
"href": "/models/panel-candidate?evaluated=true&panel_id=375582"
},
"selected": {
"href": "/models/panel-candidate?recommendation=select&panel_id=375582"
},
"rejected": {
"href": "/models/panel-candidate?recommendation=reject&panel_id=375582"
},
"hold": {
"href": "/models/panel-candidate?recommendation=hold&panel_id=375582"
}
}
}
This endpoint creates a new panel.
HTTP Request
POST https://apiv4.talview.com/models/panel
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessment_section_id | integer | true | The Id of Assessment Section |
user_id | integer | true | The id of the User who assigned the Panel |
Panel Candidate
The panel candidate gives the details of the Panel associated with the Assessment Candidate.
The Panel Candidate Object
{
"id": 111146,
"panel_id": 22287,
"assessment_candidate_id": 347099,
"created_at": "2018-04-19 11:55:21+00",
"isEvaluated": false,
"updated_at": "2018-04-19 11:55:21+00",
"added_by": 275,
"assessment_section_candidate_id": 396658,
"_links": {
"evaluations": {
"href": "/evaluation?assessment_section_candidate_id=396658&panel_id=22287"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
panel_id | integer | The Id of the Panel. |
assessment_candidate_id | integer | The id of the Assessment Candidate |
isEvaluated | boolean | States whether the Assessment Section Candidate is evaluated or not |
added_by | integer | The id of the User who added the Panel |
assessment_section_candidate_id | integer | The id of the Assessment Section Candidate |
List all Panel Candidate
GET /models/panel-candidate HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 111146,
"panel_id": 22287,
"assessment_candidate_id": 347099,
"created_at": "2018-04-19 11:55:21+00",
"isEvaluated": false,
"updated_at": "2018-04-19 11:55:21+00",
"added_by": 275,
"assessment_section_candidate_id": 396658,
"_links": {
"evaluations": {
"href": "/evaluation?assessment_section_candidate_id=396658&panel_id=22287"
}
}
},
{
"id": 111139,
"panel_id": 22285,
"assessment_candidate_id": 346973,
"created_at": "2018-04-19 09:29:34+00",
"isEvaluated": false,
"updated_at": "2018-04-19 09:30:01+00",
"added_by": 25939,
"assessment_section_candidate_id": 396516,
"_links": {
"evaluations": {
"href": "/evaluation?assessment_section_candidate_id=396516&panel_id=22285"
}
}
},
{
"id": 111138,
"panel_id": 22285,
"assessment_candidate_id": 346970,
"created_at": "2018-04-19 09:29:34+00",
"isEvaluated": false,
"updated_at": "2018-04-19 09:30:01+00",
"added_by": 25939,
"assessment_section_candidate_id": 396510,
"_links": {
"evaluations": {
"href": "/evaluation?assessment_section_candidate_id=396510&panel_id=22285"
}
}
}
]
Returns all the details of panel candidate
HTTP Request
GET https://apiv4.talview.com/models/panel-candidate
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
panel_id | integer | false | Filter based on panel Id of the Panel |
assessment_candidate_id | integer | false | Filter records based on the Id of the Assessment Candidate |
assessment_section_candidate_id | integer | false | Filter records based on the Id of the Assessment Section Candidate |
Retrieve a Panel Candidate
GET /models/panel-candidate/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 111146,
"panel_id": 22287,
"assessment_candidate_id": 347099,
"created_at": "2018-04-19 11:55:21+00",
"isEvaluated": false,
"updated_at": "2018-04-19 11:55:21+00",
"added_by": 275,
"assessment_section_candidate_id": 396658,
"_links": {
"evaluations": {
"href": "/evaluation?assessment_section_candidate_id=396658&panel_id=22287"
}
}
}
Retrieve the details of an existing panel candidate
HTTP Request
GET https://apiv4.talview.com/models/panel-candidate/<id>
URL Parameter
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create Panel Candidate
POST /models/panel-candidate HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 111155,
"panel_id": 22287,
"assessment_candidate_id": 347099,
"created_at": "2018-04-19T15:08:48+00:00",
"isEvaluated": false,
"updated_at": "2018-04-19T15:08:48+00:00",
"added_by": null,
"assessment_section_candidate_id": 396658,
"_links": {
"evaluations": {
"href": "/evaluation?assessment_section_candidate_id=396658&panel_id=22287"
}
}
}
This endpoint creates new Panel candidate.
HTTP Request
POST https://apiv4.talview.com/models/panel-candidate
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
panel_id | integer | true | The Id of the Panel |
assessment_candidate_id | integer | true | The id of the Assessment Candidate |
assessment_section_candidate_id | integer | true | The id of the Assessment Section Candidate |
Update Panel Candidate
PUT /models/panel-candidate/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 111155,
"panel_id": 22287,
"assessment_candidate_id": 347099,
"created_at": "2018-04-19T15:08:48+00:00",
"isEvaluated": false,
"updated_at": "2018-04-19T15:08:48+00:00",
"added_by": null,
"assessment_section_candidate_id": 396658,
"_links": {
"evaluations": {
"href": "/evaluation?assessment_section_candidate_id=396658&panel_id=22287"
}
}
}
This endpoint updates the panel candidate
HTTP Request
PUT https://apiv4.talview.com/models/panel-candidate/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
panel_id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
panel_id | integer | false | The Id of the Panel |
assessment_candidate_id | integer | false | The id of the Assessment Candidate |
assessment_section_candidate_id | integer | false | The id of the Assessment Section Candidate |
Parameter
The Parameter end point gives the details of all the parameter defined.
The Parameter Object
{
"id": 116092,
"external_id": null,
"category_id": null,
"type": 1,
"name": "Communication",
"recommended_value": null,
"max_value": 5
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
external_id | text | The reference id of the object |
category_id | integer | The Id of the Category |
type | integer | The type of the Parameter. Example: Numeric. The types are listed below. |
name | text | The name of the Parameter. |
recommended_value | integer | The recommendation value given by a panel for an Assessment Section Candidate |
max_value | integer | The max value of the Parameter |
List of Parameter Types:
Event Types | Key | Description |
---|---|---|
Numeric | 1 | The numeric type Parameter |
Text | 2 | The text type Parameter |
List all Parameter
GET /models/parameter HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 116092,
"external_id": null,
"category_id": null,
"type": 1,
"name": "Communication",
"recommended_value": null,
"max_value": 5
},
{
"id": 116091,
"external_id": null,
"category_id": null,
"type": 1,
"name": "Testing",
"recommended_value": null,
"max_value": 5
},
{
}
....
]
This endpoint returns all the details of notification template type.
HTTP Request
GET https://apiv4.talview.com/models/parameter
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
type | Integer | false | Filter based on Parameter Type |
external_id | Integer | false | Filter based on External Id |
name | text | false | Filter based on the name of the Parameter |
recommended_value | integer | flase | Filter records based on the recommendation value given by a panel for an Assessment Section Candidate |
category_id | integer | flase | Filter records based on the Id of the Category |
Retrieve a parameter
GET /models/parameter/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 116092,
"external_id": null,
"category_id": null,
"type": 1,
"name": "Communication",
"recommended_value": null,
"max_value": 5
}
This endpoint retrieves specific parameter.
HTTP Request
GET https://apiv4.talview.com/models/parameter/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on id |
Create Parameter
POST /models/parameter HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 116619,
"external_id": null,
"category_id": null,
"type": 1,
"name": "test50",
"recommended_value": null,
"max_value": null
}
This endpoint creates a parameter.
HTTP Request
POST https://apiv4.talview.com/models/parameter
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
name | string | true | Name of the parameter |
external_id | text | false | The reference id of the object |
category_id | integer | false | The Id of the Category |
type | integer | false | The type of the Parameter. Example: Numeric. The types are listed below. |
name | text | false | The name of the Parameter. |
max_value | integer | false | The max value of the Parameter |
Pearson Assessment
The Pearson Assessment endpoint provides the details of Pearson assessment.
The Pearson Assessment Object
{
"id": 1,
"name": "Quickscreener",
"type": "quickscreener-talview"
}
Parameter | Type | Description |
---|---|---|
id | inetger | The unique identifier of an object |
name | text | The name of the Pearson Assessment |
type | text | The type of the Pearson Assessment |
List all Pearson Assessment
This endpoint returns all existing Assessments.
GET /models/pearson-assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 1,
"name": "Quickscreener",
"type": "quickscreener-talview"
},
{
"id": 2,
"name": "Versant English Test",
"type": "vet-talview"
},
...
]
HTTP Request
GET https://apiv4.talview.com/models/assessment
Query Parameters
Query Parameter not supported.
Retrieve an Assessment
This model does not support retrieving an assessment.
Question
The Question endpoint gives the details of all the available Questions which can be added in the Assessment Section.
The Question Object
{
"id": 62028,
"type": "interview",
"title": "MCA Internship",
"content": {
"text/html": "What is a recent technical challenge you experienced and how did you solve it? ",
"text/plain": "What is a recent technical challenge you experienced and how did you solve it? "
},
"contentRaw": "What is a recent technical challenge you experienced and how did you solve it? ",
"hint": null,
"choice_1": null,
"choice_2": null,
"choice_3": null,
"choice_4": null,
"choice_5": null,
"leftList": null,
"rightList": null,
"matchMap": null,
"tagData": null,
"testCase": null,
"updated_at": "2018-04-04 12:15:58+00",
"correct_choice": 0
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
type | integer | The type of the Question. Example: Interview, Essay. The types of the Question are listed below. |
title | text | The title of the Question |
content | text | The content of the Question. The Question body. |
contentRaw | text | The raw content of the Question. Used for escaping HTML content |
hint | text | The hint for the Question |
choice_1 | text | The first answer choice for the Question |
choice_2 | text | The second answer choice for the Question |
choice_3 | text | The third answer choice for the Question |
choice_4 | text | The forth answer choice for the Question |
choice_5 | text | The fifth answer choice for the Question |
testCase | integer | The test cases for Code type Questions |
correct_choice | integer | The correct choice for the Question |
List of Question Types:
Type | Value | Description |
---|---|---|
Interview | interview | The interview type Question |
Essay | interview | The essay type Question |
Code | code | The code type Question |
Typing | typing | The typing type Question |
Interview Objective | interview with multiple choice | The multiple Choice Question |
Interview Essay | interview with essay | Interview with Essay |
Live | live | The Live Session |
MCQ | multiple choice | The MCQ type Question |
Match List | match the following | Match the following type Question |
Fill Blank | fill blanks | Fill in the blanks type Question |
True False | true or false | True or False type Question |
Multiple Choice Multiple Answer | multiple choice multiple answer | Multiple Choice Multiple Answer type Question |
List all Question
GET /models/question HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 62028,
"type": "interview",
"title": "MCA Internship",
"content": {
"text/html": "What is a recent technical challenge you experienced and how did you solve it? ",
"text/plain": "What is a recent technical challenge you experienced and how did you solve it? "
},
"contentRaw": "What is a recent technical challenge you experienced and how did you solve it? ",
"hint": null,
"choice_1": null,
"choice_2": null,
"choice_3": null,
"choice_4": null,
"choice_5": null,
"leftList": null,
"rightList": null,
"testCase": null,
"updated_at": "2018-04-04 12:15:58+00",
"correct_choice": 0
},
{
"id": 62027,
"type": "interview",
"title": "MCA Internship",
"content": {
"text/html": "Which version control systems are you familiar with? ",
"text/plain": "Which version control systems are you familiar with? "
},
"contentRaw": "Which version control systems are you familiar with? ",
"hint": null,
"choice_1": null,
"choice_2": null,
"choice_3": null,
"choice_4": null,
"choice_5": null,
"leftList": null,
"rightList": null,
"testCase": null,
"updated_at": "2018-04-04 12:15:58+00",
"correct_choice": 0
}
]
Returns all the details of questions.
HTTP Request
GET https://apiv4.talview.com/models/question
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
type | integer | false | Filter records based on the type of the Question. |
title | text | false | Filter records based on the title of the Question |
content | text | false | Filter records based on the content of the Question. |
Retrieve a Question
GET /models/question/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 62028,
"type": "interview",
"title": "MCA Internship",
"content": {
"text/html": "What is a recent technical challenge you experienced and how did you solve it? ",
"text/plain": "What is a recent technical challenge you experienced and how did you solve it? "
},
"contentRaw": "What is a recent technical challenge you experienced and how did you solve it? ",
"hint": null,
"choice_1": null,
"choice_2": null,
"choice_3": null,
"choice_4": null,
"choice_5": null,
"leftList": null,
"rightList": null,
"testCase": null,
"updated_at": "2018-04-04 12:15:58+00",
"correct_choice": 0
}
Retrieves the details of an existing question.
HTTP Request
GET https://apiv4.talview.com/models/question/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create a Question
POST /models/question HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 84073,
"type": "multiple choice",
"title": "qazwsxedc",
"content": "<p>qazwsxedc</p>",
"contentRaw": "<p>qazwsxedc</p>",
"plainTxtContent": "qazwsxedc",
"hint": null,
"randomizeChoice": false,
"choice_1": "qazwsx",
"choice_2": "qazwsx",
"choice_3": "apidoc",
"choice_4": "qazwsx",
"choice_5": null,
"leftList": null,
"rightList": null,
"testCase": null,
"created_at": "2019-02-15 13:47:45+05:30",
"updated_at": "2019-02-19T13:46:16+00:00",
"created_by": 30876,
"updated_by": 30876,
"correct_choice": 4,
"_links": {
"files": {
"href": "/models/file?question_id=84073&file_type_id%5B0%5D=14&file_type_id%5B1%5D=26"
},
"skills": {
"href": "/models/skill?question_id=84073"
},
"categories": {
"href": "/models/category?question_id=84073"
}
}
This endpoint creates a new question..
HTTP Request
POST https://apiv4.talview.com/models/question
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
type | text | true | The type of the question |
content | string | true | The content of the question |
title | text | false | The title of the Question |
hint | text | false | The hint for the Question |
choice_1 | text | false | The first answer choice for the Question |
choice_2 | text | false | The second answer choice for the Question |
choice_3 | text | false | The third answer choice for the Question |
choice_4 | text | false | The forth answer choice for the Question |
choice_5 | text | false | The fifth answer choice for the Question |
correct_choice | integer | false | The correct choice for the Question |
Update an Question
PUT /models/question/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON PUT request
{
"choice_3": "apidoc",
"correct_choice": 3
}
The above request returns JSON structured like this:
{
"id": 84073,
"type": "multiple choice",
"title": "qazwsxedc",
"content": "<p>qazwsxedc</p>",
"contentRaw": "<p>qazwsxedc</p>",
"plainTxtContent": "qazwsxedc",
"hint": null,
"randomizeChoice": false,
"choice_1": "qazwsx",
"choice_2": "qazwsx",
"choice_3": "apidoc",
"choice_4": "qazwsx",
"choice_5": null,
"leftList": null,
"rightList": null,
"testCase": null,
"created_at": "2019-02-15 13:47:45+05:30",
"updated_at": "2019-02-19T13:46:16+00:00",
"created_by": 30876,
"updated_by": 30876,
"correct_choice": 3,
"_links": {
"files": {
"href": "/models/file?question_id=84073&file_type_id%5B0%5D=14&file_type_id%5B1%5D=26"
},
"skills": {
"href": "/models/skill?question_id=84073"
},
"categories": {
"href": "/models/category?question_id=84073"
}
}
}
Updates the question with the requested parameter.
HTTP Request
PUT https://apiv4.talview.com/models/question/<id>
Url Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
type | text | false | The type of the question |
content | string | false | The content of the question |
title | text | false | The title of the Question |
hint | text | false | The hint for the Question |
choice_1 | text | false | The first answer choice for the Question |
choice_2 | text | false | The second answer choice for the Question |
choice_3 | text | false | The third answer choice for the Question |
choice_4 | text | false | The forth answer choice for the Question |
choice_5 | text | false | The fifth answer choice for the Question |
correct_choice | integer | false | The correct choice for the Question |
Delete a Question
DELETE /models/question/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This endpoint allows you to delete an existing question.
HTTP Request
DELETE https://apiv4.talview.com/models/question/<id>
Question Skill
The Question Skill end point gives the mapping of Question and Skill.
The Question Skill Object
{
"id": 53499,
"question_id": 71943,
"skill_id": 7467,
"created_by": 30876,
"updated_by": 30876,
"created_at": "2018-07-11 14:04:21+05:30",
"updated_at": "2018-07-11 14:04:21+05:30"
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
question_id | integer | The Id of the Question |
skill_id | integer | The Id of the Skill |
created_by | integer | The id of the User who created the question skill |
updated_by | integer | The id of the User who updated the question skill |
List all Question Skill
GET /models/question-skill HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 53499,
"question_id": 71943,
"skill_id": 7467,
"created_by": 30876,
"updated_by": 30876,
"created_at": "2018-07-11 14:04:21+05:30",
"updated_at": "2018-07-11 14:04:21+05:30"
},
{
"id": 63210,
"question_id": 82411,
"skill_id": 7799,
"created_by": 30876,
"updated_by": 30876,
"created_at": "2018-12-13 13:40:42+05:30",
"updated_at": "2018-12-13 13:40:42+05:30"
},
{
}
....
]
This endpoint returns all the details of the Question Skill
HTTP Request
GET https://apiv4.talview.com/models/question-skill
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
question_id | integer | false | Filter records based on the Id of the Question |
skill_id | integer | false | Filter records based on the Id of the Skill |
Retrieve a Question Skill
GET /models/question-skill/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 53499,
"question_id": 71943,
"skill_id": 7467,
"created_by": 30876,
"updated_by": 30876,
"created_at": "2018-07-11 14:04:21+05:30",
"updated_at": "2018-07-11 14:04:21+05:30"
}
This endpoint retrieves specific question skill.
HTTP Request
GET https://apiv4.talview.com/models/question-skill/<id>
URL Parameters
PParameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on Id |
Create a Question Skill
POST /models/question-skill HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 64692,
"question_id": 71943,
"skill_id": 7800,
"created_by": 30876,
"updated_by": 30876,
"created_at": "2019-01-17T13:09:46+00:00",
"updated_at": "2019-01-17T13:09:46+00:00"
}
This endpoint retrieves specific question skill.
HTTP Request
POST https://apiv4.talview.com/models/question-skill
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
question_id | integer | true | The Id of the Question |
skill_id | integer | true | The Id of the Skill |
Update an Question Skill
PUT /models/question-skill/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 64692,
"question_id": 71943,
"skill_id": 7800,
"created_by": 30876,
"updated_by": 30876,
"created_at": "2019-01-17T13:09:46+00:00",
"updated_at": "2019-01-17T13:09:46+00:00"
}
This endpoint updates specific question skill.
HTTP Request
PUT https://apiv4.talview.com/models/question-skill/<id>
Url Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
question_id | integer | false | The Id of the Question |
skill_id | integer | false | The Id of the Skill |
Delete an Question Skill
DELETE /models/question-skill/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This endpoint deletes a specific question skill.
HTTP Request
DELETE https://apiv4.talview.com/models/question-skill/<id>
Section
The Section end point gives the details of the section. An Assessment consists of one or more Section associated with it. Section combined with the Assessment gives the Assessment Section.
The Section Object
{
"id": 3516932,
"external_id": null,
"title": "Typing Test",
"is_template": false,
"is_default": false,
"evaluation_form_id": null,
"candidateFormUrl": null,
"evaluationFormUrl": null,
"content": null,
"questionsCount": 1,
"type": "Typing Test",
"form_id": null,
"type_id": 10,
"section_type_id": 10,
"external_assessment_id": null,
"created_at": "2019-03-13 05:38:39+00",
"updated_at": "2019-03-13 05:38:39+00",
"_links": {
"sectionParameters": {
"href": "/models/section-parameter?section_id=3516932"
},
"sectionQuestions": {
"href": "/models/section-question?section_id=3516932"
},
"sectionSkills": {
"href": "/models/section-skill?section_id=3516932"
},
"parameters": {
"href": "/models/parameter?section_id=3516932"
},
"questions": {
"href": "/models/question?section_id=3516932"
},
"skills": {
"href": "/models/skills?section_id=3516932"
},
"combined": {
"href": "/models/section-combined?section_id=3516932"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
external_id | text | The external id of the object |
title | text | The title of the Section |
is_template | boolean | Whether the Section is template or not |
is_default | boolean | Whether the section is a default Section |
evaluation_form_id | integer | The id of the evaluation From |
candidateFormUrl | text | The url of the candidate Form |
evaluationFormUrl | The url of the evaluation Form | |
content | text | The content of the Section |
questionsCount | integer | The number of questions in the Section |
type | text | The type of the Section. Example: Typing, Objective. The type of the Section are listed below. |
form_id | integer | The id of the From |
type_id | integer | The id of the Form type |
section_type_id | integer | The id of the Section type |
external_assessment_id | integer | The id of the external assessment |
List of Section Types:
Type | Key | Description |
---|---|---|
Default | 1 | The default Section |
Landing | 2 | The landing Section |
Form | 3 | The Form Section |
Essay | 4 | The Essay Section |
Interview | 5 | The Interview Section |
Objective | 6 | The objective Section |
Live | 7 | The Live Section |
Post | 8 | The Post Section |
Promo | 9 | The Promo Section |
Typing | 10 | The Typing Section |
Instruction | 11 | The Instruction Section |
List all Section
GET /models/section HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 3516932,
"external_id": null,
"title": "Typing Test",
"is_template": false,
"is_default": false,
"evaluation_form_id": null,
"candidateFormUrl": null,
"evaluationFormUrl": null,
"content": null,
"questionsCount": 1,
"type": "Typing Test",
"form_id": null,
"type_id": 10,
"section_type_id": 10,
"external_assessment_id": null,
"created_at": "2019-03-13 05:38:39+00",
"updated_at": "2019-03-13 05:38:39+00",
"_links": {
"sectionParameters": {
"href": "/models/section-parameter?section_id=3516932"
},
"sectionQuestions": {
"href": "/models/section-question?section_id=3516932"
},
"sectionSkills": {
"href": "/models/section-skill?section_id=3516932"
},
"parameters": {
"href": "/models/parameter?section_id=3516932"
},
"questions": {
"href": "/models/question?section_id=3516932"
},
"skills": {
"href": "/models/skills?section_id=3516932"
},
"combined": {
"href": "/models/section-combined?section_id=3516932"
}
}
}
]
This endpoint returns all the details of the Section
HTTP Request
GET https://apiv4.talview.com/models/section
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
title | text | false | Filter records based on the title of the Section |
is_template | boolean | false | Filter records based on Whether the Section is template or not |
is_default | boolean | false | Filter records based on Whether the section is a default Section |
evaluation_form_id | integer | false | Filter records based on the Id of the evaluation From |
section_type_id | integer | false | Filter records based on the id of the Section type |
Retrieve a Section
GET /models/section/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 3516932,
"external_id": null,
"title": "Typing Test",
"is_template": false,
"is_default": false,
"evaluation_form_id": null,
"candidateFormUrl": null,
"evaluationFormUrl": null,
"content": null,
"questionsCount": 1,
"type": "Typing Test",
"form_id": null,
"type_id": 10,
"section_type_id": 10,
"external_assessment_id": null,
"created_at": "2019-03-13 05:38:39+00",
"updated_at": "2019-03-13 05:38:39+00",
"_links": {
"sectionParameters": {
"href": "/models/section-parameter?section_id=3516932"
},
"sectionQuestions": {
"href": "/models/section-question?section_id=3516932"
},
"sectionSkills": {
"href": "/models/section-skill?section_id=3516932"
},
"parameters": {
"href": "/models/parameter?section_id=3516932"
},
"questions": {
"href": "/models/question?section_id=3516932"
},
"skills": {
"href": "/models/skills?section_id=3516932"
},
"combined": {
"href": "/models/section-combined?section_id=3516932"
}
}
}
This endpoint fetch section by id.
HTTP Request
GET https://apiv4.talview.com/models/section/<id>
URL Parameters|
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Section Combined
The Section Combined Model gives the details of the Question associated with the Section.
Question can be mapped in two ways
1) By mapping individual Question form Question model
2) By providing the Skill Id and the Question Count(q_count)
It will generate random number of question form Question Skill during the time of Assessment. The number of question to be generated depends on the Question count provided. If Question count is 3, 3 random question will be generated.
The Section Combined Object
{
"id": 15712,
"question_id": 89071,
"q_count": 1,
"skill_id": null,
"section_id": 3550947,
"sort_order": 5,
"created_by": 30876,
"updated_by": 30876
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
question_id | integer | The Id of the Question] |
q_count | integer | The total count of Questions in the Section |
skill_id | integer | The id of the Skill |
section_id | integer | The id of the Assessment Section |
sort_order | integer | The sequence order of the Question |
created_by | integer | The id of the User who created the section |
updated_by | integer | The id of the User who updated the section |
GET /models/section-combined HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
List all Section Combine
The above request returns JSON structured like this:
[
{
"id": 14,
"question_id": 83199,
"q_count": 1,
"skill_id": null,
"section_id": 2856943,
"sort_order": 0,
"created_by": null,
"updated_by": null
},
{
"id": 15,
"question_id": 83027,
"q_count": 1,
"skill_id": null,
"section_id": 2856943,
"sort_order": 1,
"created_by": null,
"updated_by": null
},
{
}
]
This endpoint returns all the details of section combined.
HTTP Request
GET https://apiv4.talview.com/models/section-combined
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
skill_id | integer | false | Filter records based on the id of the Skill |
section_id | integer | false | Filter records based on the id of the Assessment Section |
Retrieve a Section Combined
GET /models/section-combined/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 14,
"question_id": 83199,
"q_count": 1,
"skill_id": null,
"section_id": 2856943,
"sort_order": 0,
"created_by": null,
"updated_by": null
}
This endpoint retrieves a specific section combined.
HTTP Request
GET https://apiv4.talview.com/models/section-combined/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create a Section Combined
POST /models/section-combined HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST request
{
"section_id": 2856943,
"sort_order": 0
}
The above request returns JSON structured like this:
{
"id": 127,
"question_id": null,
"q_count": null,
"skill_id": null,
"section_id": 2856943,
"sort_order": 0,
"created_by": 30876,
"updated_by": 30876
}
This endpoint creates a section combined.
HTTP Request
POST https://apiv4.talview.com/models/section-combined
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
section_id | integer | true | The id of the Assessment Section |
sort_order | integer | true | The sequence order of the Question |
question_id | integer | false | The Id of the Question] |
q_count | integer | false | The total count of Questions in the Section |
skill_id | integer | false | The id of the Skill |
Section Parameter
The Section Parameter endpoint gives the details of the Parameter associated with the Section.
The Section Parameter Object
{
"id": 5241115,
"parameter_id": 116091,
"section_id": 1294348,
"sort_order": null,
"recommended_value": null,
"is_mandatory": false,
"created_at": "2018-07-11 08:35:03+00",
"updated_at": "2018-07-11 08:35:03+00"
}
Parameters | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
parameter_id | integer | The Id of the Parameter |
section_id | integer | The id of the Section |
sort_order | integer | The sort order value |
recommended_value | integer | The recommended value for the particular Section |
is_mandatory | boolean | Whether the parameter for the section is mandatory |
List all Section Parameter
GET /models/section-parameter HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 5241219,
"parameter_id": 116091,
"section_id": 1294430,
"sort_order": null,
"recommended_value": null,
"is_mandatory": false
},
{
"id": 4987234,
"parameter_id": 116091,
"section_id": 884638,
"sort_order": null,
"recommended_value": null,
"is_mandatory": false
},
{
}
....
]
This endpoint retrieves all section parameters.
HTTP Request
GET https://apiv4.talview.com/models/section-parameter
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
parameter_id | integer | false | Filter records based on the Id of the Parameter |
section_id | integer | false | Filter records based on the Id of the Section |
Retrieve a Section Parameter
GET /models/section-parameter/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 5241219,
"parameter_id": 116091,
"section_id": 1294430,
"sort_order": null,
"recommended_value": null,
"is_mandatory": false
}
This endpoint retrieves a specific section parameter.
HTTP Request
GET https://apiv4.talview.com/models/section-parameter/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create a Section Parameter
POST /models/section-parameter HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST request
{
"parameter_id": 116716,
"section_id": 2192778
}
The above request returns JSON structured like this:
{
"id": 6689029,
"parameter_id": 116716,
"section_id": 2192778,
"sort_order": null,
"recommended_value": null,
"is_mandatory": null
}
This endpoint creates a section parameter.
HTTP Request
POST https://apiv4.talview.com/models/section-parameter
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
parameter_id | integer | true | The Id of the Parameter |
section_id | integer | true | The id of the Section |
sort_order | integer | false | The sort order value for the parameter |
recommended_value | integer | false | The recommended value for the particular Section |
Update a Section Parameter
PUT /models/section-parameter/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 6091257,
"parameter_id": 79588,
"section_id": 1950033,
"sort_order": null,
"recommended_value": null,
"is_mandatory": false
}
This end point updates a section parameter.
HTTP Request
PUT https://apiv4.talview.com/models/section-parameter/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
sort_order | integer | false | The sort order value for the parameter |
Delete a Section Parameter
DELETE /models/section-parameter/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code: 204 No Content
This endpoint deletes an existing section parameter
HTTP Request
DELETE https://apiv4.talview.com/models/section-parameter/<id>
Section Question
The Section Question endpoint gives the mapping of Section and Question.
The Section Question Object
{
"id": 1876735,
"section_id": 3539089,
"question_id": 56657,
"question_order": 1,
"created_at": "2019-03-18 14:05:03+00",
"created_by": 67656,
"updated_at": "2019-03-18 14:05:03+00",
"updated_by": 67656
}
Parameters | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
section_id | integer | The id of the Section |
question_id | integer | The id of the Question |
question_order | integer | The sequence order of the question |
List all Section Question
GET /models/section-question HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 1570065,
"section_id": 2923542,
"question_id": 56652,
"question_order": 1,
"created_at": "2019-01-16 10:56:22+00",
"created_by": 30876,
"updated_at": "2019-01-16 10:56:22+00",
"updated_by": 30876
},
{
"id": 1570064,
"section_id": 2923541,
"question_id": 56652,
"question_order": 1,
"created_at": "2019-01-16 10:53:31+00",
"created_by": 30876,
"updated_at": "2019-01-16 10:53:31+00",
"updated_by": 30876
},
{
}
]
This endpoint retrieves all section questions.
HTTP Request
GET https://apiv4.talview.com/models/section-question
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
section_id | integer | false | Filter records based on the id of the Section |
question_id | integer | false | Filter records based on the id of the Question |
Retrieve a Section Question
GET /models/section-question/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 1570065,
"section_id": 2923542,
"question_id": 56652,
"question_order": 1,
"created_at": "2019-01-16 10:56:22+00",
"created_by": 30876,
"updated_at": "2019-01-16 10:56:22+00",
"updated_by": 30876
}
This endpoint retrieves a specific section question.
HTTP Request
GET https://apiv4.talview.com/models/section-question/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on id |
Create a Section Question
POST /models/section-question HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST request
{
"section_id": 2923542,
"question_id": 83948,
"question_order": 1
}
The above request returns JSON structured like this:
{
"id": 6689029,
"parameter_id": 116716,
"section_id": 2192778,
"sort_order": null,
"recommended_value": null,
"is_mandatory": null
}
This endpoint creates a section question.
HTTP Request
POST https://apiv4.talview.com/models/section-question
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
section_id | integer | true | The id of the Section |
question_id | integer | true | The id of the Question |
question_order | integer | true | The sequence order of the question |
Section Skill
The Section Skill endpoint gives he mapping of Section and Skill.
The Section Skill Object
{
"id": 821373,
"is_mandatory": true,
"section_id": 3328043,
"skill_id": 7800,
"q_count": 2,
"q_order": 1,
"created_at": "2019-02-27 06:20:52+00",
"updated_at": "2019-02-27 06:20:52+00"
}
Parameters | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
is_mandatory | boolean | If true, the Section is mandatory |
section_id | integer | The id of the Section |
skill_id | integer | The id of the Skill |
q_count | integer | The total count of the Question in the particular Section |
q_order | integer | The Sequence of the Question |
List all Section Skill
GET /models/section-skill HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 455837,
"is_mandatory": false,
"section_id": 880531,
"skill_id": 4932,
"q_count": 2,
"q_order": 1
},
{
"id": 455838,
"is_mandatory": false,
"section_id": 880531,
"skill_id": 5410,
"q_count": 2,
"q_order": 2
}
...
]
This endpoint lists all section skill.
HTTP Request
GET https://apiv4.talview.com/models/section-skill
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
section_id | integer | false | Filter records based on the Id of the Section |
skill_id | integer | false | Filter records based on the Id The id of the Skill |
Create a Section Skill
POST /models/section-skill HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST request
{
"section_id":2959918,
"skill_id":7459,
"q_count":2,
"q_order":1
}
The above request returns JSON structured like this:
{
"id": 745715,
"is_mandatory": null,
"section_id": 2959918,
"skill_id": 7459,
"q_count": 2,
"q_order": 1,
"created_at": "2019-02-20T09:53:35+00:00",
"updated_at": "2019-02-20T09:53:35+00:00"
}
...
This endpoint creates a new section skill.
HTTP Request
POST https://apiv4.talview.com/models/section-skill
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
section_id | integer | true | The id of the Section |
skill_id | integer | true | The id of the Skill |
q_count | integer | true | The total count of the Question in the particular Section |
q_order | integer | true | The Sequence of the Question |
is_mandatory | boolean | false | If true, the Section is mandatory |
Update a Section Skill
PUT /models/section-skill/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 745715,
"is_mandatory": null,
"section_id": 2959918,
"skill_id": 7459,
"q_count": 4,
"q_order": 2,
"created_at": "2019-02-20T09:53:35+00:00",
"updated_at": "2019-02-20T09:53:35+00:00"
}
...
This endpoint updates an existing section skill.
HTTP Request
PUT https://apiv4.talview.com/models/section-skill/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
section_id | integer | false | The id of the Section |
skill_id | integer | false | The id of the Skill |
q_count | integer | false | The total count of the Question in the particular Section |
q_order | integer | false | The Sequence of the Question |
is_mandatory | boolean | false | If true, the Section is mandatory |
Delete a Section Skill
DELETE /models/section-skill/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code: 204 No Content
This endpoint deletes an existing section skill.
HTTP Request
DELETE https://apiv4.talview.com/models/section-skill/<id>
Select Field Data
The Select Field Data end point give all the details of the dropdown value. All the dropdown value is associated with the Select Field Type.
The Select Field Data Object
{
"id": 5972549,
"key": "Four Years College",
"value": "Four Years College",
"parent_value": null,
"parent_id": null,
"select_field_type_id": 58,
"sort_order": null,
"updated_by": 31670,
"updated_at": "2018-04-19 14:10:10+00",
"created_at": "2018-04-19 14:10:10+00",
"created_by": 31670
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
key | text | The Key value of the Select Field Data |
value | text | The value of the Select Field Data |
parent_value | text | The parent value of the Select Field Data. Child select field data is associated with parent select field data. If we select the parent value then the child Select Field Data will be triggered |
parent_id | integer | The Id of the parent Select Field Data |
select_field_type_id | integer | The id of the Select Field Type |
sort_order | integer | The sequence order of the Select Field Data |
List all Select Field Data
GET /models/select-field-data HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 5972549,
"key": "Four Years College",
"value": "Four Years College",
"parent_value": null,
"parent_id": null,
"select_field_type_id": 58,
"sort_order": null,
"updated_by": 31670,
"updated_at": "2018-04-19 14:10:10+00",
"created_at": "2018-04-19 14:10:10+00",
"created_by": 31670
},
{
...
},
{
...
}
]
This endpoint lists all select field datas.
HTTP Request
GET https://apiv4.talview.com/models/select-field-data
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
key | text | false | Filter records based on the Key value of the Select Field Data |
value | text | false | Filter records based on the value of the Select Field Data |
parent_value | text | false | Filter records based on the parent value of the Select Field Data. |
parent_id | integer | false | Filter records based on the Id of the parent Select Field Data |
select_field_type_id | integer | false | Filter records based on the id of the Select Field Type |
Create a new Select Field Data
POST /models/select-field-data HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST request
{
"select_field_type_id": 90,
"key": "12345",
"value": "Solution Manager"
}
The above request returns JSON structured like this:
{
"id": 7091483,
"key": "12345",
"value": "Solution Manager",
"parent_value": null,
"parent_id": null,
"select_field_type_id": 90,
"sort_order": null,
"updated_by": 30876,
"updated_at": "2019-02-20T11:43:27+00:00",
"created_at": "2019-02-20T11:43:27+00:00",
"created_by": 30876
}
This endpoint creates a new select field datas.
HTTP Request
POST https://apiv4.talview.com/models/select-field-data
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
select_field_type_id | integer | true | The id of the Select Field Type |
key | text | true | The Key value of the Select Field Data |
value | text | true | The value of the Select Field Data |
parent_value | text | false | The parent value of the Select Field Data |
parent_id | integer | false | The Id of the parent Select Field Data |
Delete a Select Field Data
DELETE /models/select-field-data/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code: 204 No Content
This endpoint deletes an existing select field data.
HTTP Request
DELETE https://apiv4.talview.com/models/select-field-data/<id>
Select Field Type
The Select Field Type endpoint gives the details of the types of Select Field Data.
The Select Field Type Object
{
"id": 90,
"name": "Test Name Select Field Type",
"isPublic": false,
"updated_by": 30876,
"updated_at": "2018-04-20T10:53:22+00:00",
"created_at": "2018-04-20T10:53:22+00:00",
"created_by": 30876
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
name | text | The name of the Select Field Type |
is_public | boolean | Whether the select field type is public or private |
List all Select Field Type
GET /models/select-field-type HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The Response
[
{
"id": 90,
"name": "Test Name Select Field Type",
"isPublic": false,
"updated_by": 30876,
"updated_at": "2018-04-20 10:53:22+00",
"created_at": "2018-04-20 10:53:22+00",
"created_by": 30876
},
{
"id": 91,
"name": "Test Name Select Field Type",
"isPublic": false,
"updated_by": 30876,
"updated_at": "2018-04-20 10:59:24+00",
"created_at": "2018-04-20 10:59:24+00",
"created_by": 30876
}
]
This endpoint returns all the details of select field types.
HTTP Request
GET https://apiv4.talview.com/models/select-field-type
Query Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
name | text | false | Filter records based on the Name of the Select Field Type |
Create a Select Field Type
POST /models/select-field-type HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
Request Body
{
"name": "Test Name Select Field Type"
}
The Response
{
"id": 90,
"name": "Test Name Select Field Type",
"isPublic": false,
"updated_by": 30876,
"updated_at": "2018-04-20T10:53:22+00:00",
"created_at": "2018-04-20T10:53:22+00:00",
"created_by": 30876
}
This endpoint add select field types.
HTTP Request
POST https://apiv4.talview.com/models/select-field-type
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
name | text | truw | The name of the Select Field Type |
Delete an Select Field Type
DELETE /models/select-field-type/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns the status code: 204 No Content
This endpoint deletes select field types.
HTTP Request
DELETE https://apiv4.talview.com/models/select-field-type/<id>
Settings Allowed Value
The Settings Allowed Value Object
{
"id": 95,
"settings_id": 2,
"item_value": "3",
"caption": "Clients",
"created_at": "2015-04-27 07:15:45+00",
"updated_at": null
}
Parameters | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
settings_id | integer | The id of Setting |
item_value | integer | The item value of Setting Allowed Value |
caption | text | The caption for the Setting Allowed Value |
List all Settings Allowed Value
GET /models/settings-allowed-value HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 95,
"settings_id": 2,
"item_value": "3",
"caption": "Clients",
"created_at": "2015-04-27 07:15:45+00",
"updated_at": null
},
{
"id": 96,
"settings_id": 2,
"item_value": "4",
"caption": "Users",
"created_at": "2015-04-27 07:15:45+00",
"updated_at": null
},
{
}
....
]
This endpoint retrieves all settings allowed value.
HTTP Request
GET https://apiv4.talview.com/models/settings-allowed-value
Query Parameters
Parameter | Type | mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
settings_id | integer | false | Filter records based on the id of Setting |
item_value | integer | false | Filter records based on the item value of Setting Allowed Value |
caption | text | false | Filter records based on the caption of the Setting Allowed Value |
Retrieve a Settings Allowed Value
GET /models/settings-allowed-value/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 97,
"settings_id": 2,
"item_value": "5",
"caption": "Organization",
"created_at": "2015-04-27 07:15:45+00",
"updated_at": null
}
This endpoint retrieves a specific settings allowed value.
HTTP Request
GET https://apiv4.talview.com/models/settings-allowed-value/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Skill
The Skill endpoint gives the details of all the Skills. Example: Coding Skill, Communication Skill etc. Skill is associated with the Section.
The Skill Object
{
"id": 9760,
"external_id": null,
"description": null,
"created_at": "2019-03-13 10:57:55+00",
"updated_at": "2019-03-13 10:59:01+00",
"created_by": 30876,
"updated_by": 30876,
"name": "API DOC TEST updated again "
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
external_id | string | The external id of the object |
name | string | The name of the skill |
description | string | The description of the Skill |
created_by | integer | The id of the User who created the skill |
updated_by | integer | The id of the User who updated the skill |
List all Skill
GET /models/skill HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 9760,
"external_id": null,
"description": null,
"created_at": "2019-03-13 10:57:55+00",
"updated_at": "2019-03-13 10:59:01+00",
"created_by": 30876,
"updated_by": 30876,
"name": "API DOC TEST updated again "
},
{
"id": 9574,
"external_id": null,
"description": "jj",
"created_at": "2019-03-05 11:37:31+00",
"updated_at": "2019-03-05 11:37:31+00",
"created_by": 45239,
"updated_by": 45239,
"name": "j"
}
]
This endpoint returns all the details of Skill
HTTP Request
GET https://apiv4.talview.com/models/skill
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
name | string | false | Filter records based on the name of the Skill |
description | string | false | Filter records based on the description of the Skill |
Retrieve a Skill
GET /models/skill/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above command returns JSON structured like this:
{
"id": 5661,
"external_id": null,
"name": "Communication test"
}
This endpoint fetch skill based on specific skill id.
HTTP Request
GET https://apiv4.talview.com/models/skill/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create new Skill
POST /models/skill HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 5661,
"external_id": null,
"name": "Communication test"
}
This endpoint create a new skill.
HTTP Request
POST https://apiv4.talview.com/models/skill
Body parameter
Parameter | Type | Mandatory | Description |
---|---|---|---|
name | text | true | Name of the new skill |
Update a Skill
PUT /models/skill HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON body to do PUT request
{
"external_id": "985474",
"name": "test1088"
}
The above request returns JSON structured like this:
{
"id": 9419,
"external_id": "985474",
"description": null,
"created_at": "2019-01-09 07:53:20+00",
"updated_at": "2019-01-15T08:24:50+00:00",
"created_by": 30876,
"updated_by": 30876,
"name": "test1088"
}
This endpoint update the fields of a skill.
HTTP Request
PUT https://apiv4.talview.com/models/skill/<id>
URL parameter
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Status Reason
The Status Reason endpoint provides the reasons for changing the status of an Assessment Candidate. Example: REJECTED because of incomplete background verification.
The Status Reason Object
{
"id": 462,
"external_id": null,
"status_id": 1,
"type": null,
"key": "Test Key",
"label": "Test Label",
"created_at": "2018-04-20 11:49:53+00",
"updated_at": "2018-04-20 11:49:53+00",
"created_by": 30876,
"updated_by": 30876
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
external_id | text | The external id of the object |
status_id | integer | The Id of the Status |
type | text | The type of the Status. Example: Reject, Hold etc. |
key | text | The key of the Status |
label | text | The label of the Status. Example: Reject Status |
List all Status Reason
GET /models/status-reason HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 462,
"external_id": null,
"status_id": 1,
"type": null,
"key": "Test Key",
"label": "Test Label",
"created_at": "2018-04-20 11:49:53+00",
"updated_at": "2018-04-20 11:49:53+00",
"created_by": 30876,
"updated_by": 30876
},
{
"id": 463,
"external_id": null,
"status_id": 1,
"type": null,
"key": "Test Key",
"label": "Test Label",
"created_at": "2018-04-20 11:52:37+00",
"updated_at": "2018-04-20 11:52:37+00",
"created_by": 30876,
"updated_by": 30876
},
{
}
....
]
This endpoint returns all the details of the status reason
HTTP Request
GET https://apiv4.talview.com/models/status-reason
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
external_id | text | false | Filter records based on the id of the object |
status_id | integer | false | Filter records based on the Id of the Status |
key | text | false | Filter records based on the key of the Status |
label | text | false | Filter records based on the label of the Status |
Retrieve a Status Reason
GET /models/status-reason/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 462,
"external_id": null,
"status_id": 1,
"type": null,
"key": "Test Key",
"label": "Test Label",
"created_at": "2018-04-20 11:49:53+00",
"updated_at": "2018-04-20 11:49:53+00",
"created_by": 30876,
"updated_by": 30876
}
This endpoint retrieves a specific status reason.
HTTP Request
GET https://apiv4.talview.com/models/status-reason/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | Filter records based on Id |
Create a Status Reason
POST /models/status-reason HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST request
{
"status_id": 3,
"key": "anonymous",
"label": "Test Label"
}
The above request returns JSON structured like this:
{
"id": 469,
"external_id": null,
"status_id": 3,
"type": null,
"key": "anonymous",
"label": "Test Label",
"created_at": "2019-01-17T14:43:19+00:00",
"updated_at": "2019-01-17T14:43:19+00:00",
"created_by": 30876,
"updated_by": 30876
}
This endpoint creates a status reason.
HTTP Request
POST https://apiv4.talview.com/models/section-parameter
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
key | text | true | The key of the Status |
status_id | integer | false | The Id of the Status |
label | text | false | The label of the Status |
User
The User endpoint gives the details of the Users of the Organization.
The User Object
{
"id": 41,
"external_id": "",
"username": "user+tumgoucxkw2@talview.com",
"name": "sahana",
"location": "",
"is_common_login": true,
"email": "user+tumgoucxkw2@talview.com",
"roles": [
"Account Manager",
"Content Manager",
"Evaluator",
"Hiring Manager",
"Master Hiring Manager",
"Master Recruiter",
"Recruiter",
"Sourcer"
],
"organization_id": 2,
"phone": "8253497844",
"current_role": "Account Manager",
"status": 10,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2019-12-21T06:19:15+00:00",
"created_by": 1,
"updated_by": 1,
"logged_in_at": "2019-12-19 06:40:30+00",
"logged_in_from": "172.19.0.1",
"gravatarLink": "https://www.gravatar.com/avatar/30284b632a71f240d086e3d877b3e70d?s=100&d=mm&r=g",
"appState": null,
"profilePicUrl": null,
"defaultLocale": "en-US",
"countryCode": "+1",
"_links": {
"self": {
"href": "/user/me"
},
"permissions": {
"href": "/user/me/permission?type=2"
},
"settings_data": {
"href": "/user/me/settings_data"
},
"index": {
"href": "/user"
},
"metrics": {
"href": "/user/metrics/41"
},
"categories": {
"href": "/models/user-category?user_id=41"
},
"busyCalendar": {
"href": "/models/user-calendar?type=3&user_id=41&startsAt=1576909155"
},
"liveCalendar": {
"href": "/models/user-calendar?type=1&user_id=41&startsAt=1576909155"
},
"availability": {
"href": "/models/user-calendar?type=2&user_id=41&startsAt=1576909155"
},
"userProfilePic": {
"href": "/user/display-picture"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
external_id | text | The reference Id for the object |
username | text | The username of the User. The email address of an user is the Username to the user |
name | text | The name of the User |
location | text | The location of the user. Example: Bangalore |
is_common_login | boolean | Is common login enabled for a particular user |
text | Email address of the User | |
roles | array of texts | The role assigned to an User. Example: Recruiter, Evaluator. The roles are listed in the table below |
organization_id | integer | The Id of the organization to which the User belongs |
phone | integer | The phone number of the User |
current_role | text | The role of the User currently working as |
status | integer | The status of an user. Example: Active, Inactive, Suspended. The Status are listed in the table below |
logged_in_at | timestamptz | The time when the User logged in |
logged_in_from | inet | Network address of the User's system |
gravatarLink | text | The URL to an user's gravatar account |
appState | jsonb | The information of the application. Example: "web": {"releaseShown": ["v4.0.0"]} |
profilePicUrl | string | The URL of the user's profile picture |
defaultLocale | string | The default language of the user |
countryCode | String | The country code of the user |
List of Event Roles:
Value | Role |
---|---|
SuperAdmin | Super Admin |
Developer | Developer |
Recruiter | Recruiter |
Evaluator | Evaluator |
Account_Manager | Account Manager |
Content_Manager | Content Manager |
Support_Engineer | Support Engineer |
Master_Recruiter | Master Recruiter |
Walk_In_Admin | Walkin Admin |
Admin | Admin |
API | API User |
Candidate | Candidate |
Consultant | Consultant |
Student | Student |
Sourcer | Sourcer |
Onboarding | Onboarding |
Offer | Offer |
Candidate_Verification | Candidate Verification |
Background_Check | Background Check |
Hiring_Manager | Hiring Manager |
Master_Hiring_Manager | Master Hiring Manager |
External_Assessment_Admin | External Assessment Admin |
List of User Status:
Status | Key | Description |
---|---|---|
New | 10 | The User is new |
Active | 20 | The User is Active |
Inactive | 30 | The User is Inactive |
Suspended | 40 | The User id Suspended |
Allowed Country Codes
["+93","+355","+213","+376","+244","+672","+54","+374","+297", "+61","+43","+994","+973","+880","+375","+32","+501","+229", "+975","+591","+387","+267","+55","+673","+359","+226","+257", "+855","+237","+236","+235","+56","+86","+53","+61","+57","+269", "243","+242","+682","+506","+225","+385","+53","+357","+420","+45", "+253","+670","+593","+20","+503","+240","+291","+372","+251", "+500","+298","+679","+358","+33","+594","+689","+241","+220", "+995","+49","+233","+350","+30","+299","+590","+502","+224", "+245","+592","+509","+504","+852","+36","+354","+91","+62","+98", "+964","+353","+972","+39","+81","+962","+7","+254","+686","+850", "+82","+965","+996","+856","+371","+961","+266","+231","+218","+423", "+370","+352","+853","+389","+261","+265","+60","+960","+223","+356", "+692","+596","+222","+230","+269","+52","+691","+373","+377","+976", "+212","+258","+95","+264","+674","+977","+31","+599","+687","+64", "+505","+227","+234","+683","+672","+47","+968","+92","+680","+970", "+507","+675","+595","+51","+63","+48","+351","+974","+262","+40","+7", "+250","+290","+508","+685","+378","+239","+966","+221","+248","+232", "+65","+421","+386","+677","+252","+27","+34","+94","+249","+597","+268", "+46","+41","+963","+886","+992","+255","+66","+690","+676","+216","+90", "+993","+688","+256","+380","+971","+44","+998","+678","+418","+58","+84", "+681","+967","+260","+263","+1684","+1264","+1268","+1242","+1246","+1441", "+1345","+1809","+1829","+1473","+1671","+1876","+1664","+1670","+1787", "+1939","+1869","+1758","+1784","+1868","+1649","+1284","+1340","+381","+500", "+47","+228","+246","+212","+38","+243","+1"]
List all Users
GET /user HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 41,
"external_id": "",
"username": "user+tumgoucxkw2@talview.com",
"name": "sahana",
"location": "",
"is_common_login": true,
"email": "user+tumgoucxkw2@talview.com",
"roles": [
"Account Manager",
"Content Manager",
"Evaluator",
"Hiring Manager",
"Master Hiring Manager",
"Master Recruiter",
"Recruiter",
"Sourcer"
],
"organization_id": 2,
"phone": "8253497844",
"current_role": "Account Manager",
"status": 10,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2019-12-21T06:19:15+00:00",
"created_by": 1,
"updated_by": 1,
"logged_in_at": "2019-12-19 06:40:30+00",
"logged_in_from": "172.19.0.1",
"gravatarLink": "https://www.gravatar.com/avatar/30284b632a71f240d086e3d877b3e70d?s=100&d=mm&r=g",
"appState": null,
"profilePicUrl": null,
"defaultLocale": "en-US",
"countryCode": "+1",
"_links": {
"self": {
"href": "/user/me"
},
"permissions": {
"href": "/user/me/permission?type=2"
},
"settings_data": {
"href": "/user/me/settings_data"
},
"index": {
"href": "/user"
},
"metrics": {
"href": "/user/metrics/41"
},
"categories": {
"href": "/models/user-category?user_id=41"
},
"busyCalendar": {
"href": "/models/user-calendar?type=3&user_id=41&startsAt=1576909155"
},
"liveCalendar": {
"href": "/models/user-calendar?type=1&user_id=41&startsAt=1576909155"
},
"availability": {
"href": "/models/user-calendar?type=2&user_id=41&startsAt=1576909155"
},
"userProfilePic": {
"href": "/user/display-picture"
}
}
}
]
This endpoint returns all users of an organization.
HTTP Request
GET https://apiv4.talview.com/user
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
external_id | text | false | Filter records based on the reference Id |
username | text | false | Filter records based on User Name |
name | text | false | Filter records based on the name of the User |
false | Filter records based on email of the User | ||
roles | array of texts | false | Filter records based on the roles of the User |
Retrieve an User
GET /user/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 41,
"external_id": "",
"username": "user+tumgoucxkw2@talview.com",
"name": "sahana",
"location": "",
"is_common_login": true,
"email": "user+tumgoucxkw2@talview.com",
"roles": [
"Account Manager",
"Content Manager",
"Evaluator",
"Hiring Manager",
"Master Hiring Manager",
"Master Recruiter",
"Recruiter",
"Sourcer"
],
"organization_id": 2,
"phone": "8253497844",
"current_role": "Account Manager",
"status": 10,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2019-12-21T06:19:15+00:00",
"created_by": 1,
"updated_by": 1,
"logged_in_at": "2019-12-19 06:40:30+00",
"logged_in_from": "172.19.0.1",
"gravatarLink": "https://www.gravatar.com/avatar/30284b632a71f240d086e3d877b3e70d?s=100&d=mm&r=g",
"appState": null,
"profilePicUrl": null,
"defaultLocale": "en-US",
"countryCode": "+1",
"_links": {
"self": {
"href": "/user/me"
},
"permissions": {
"href": "/user/me/permission?type=2"
},
"settings_data": {
"href": "/user/me/settings_data"
},
"index": {
"href": "/user"
},
"metrics": {
"href": "/user/metrics/41"
},
"categories": {
"href": "/models/user-category?user_id=41"
},
"busyCalendar": {
"href": "/models/user-calendar?type=3&user_id=41&startsAt=1576909155"
},
"liveCalendar": {
"href": "/models/user-calendar?type=1&user_id=41&startsAt=1576909155"
},
"availability": {
"href": "/models/user-calendar?type=2&user_id=41&startsAt=1576909155"
},
"userProfilePic": {
"href": "/user/display-picture"
}
}
}
This endpoint retrieves a single user based on user id.
HTTP Request
GET https://apiv4.talview.com/user/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on id |
Create an user
POST /models/user HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
{
"id": 41,
"external_id": "",
"username": "user+tumgoucxkw2@talview.com",
"name": "sahana",
"location": "",
"is_common_login": true,
"email": "user+tumgoucxkw2@talview.com",
"roles": [
"Account Manager",
"Content Manager",
"Evaluator",
"Hiring Manager",
"Master Hiring Manager",
"Master Recruiter",
"Recruiter",
"Sourcer"
],
"organization_id": 2,
"phone": "8253497844",
"current_role": "Account Manager",
"status": 10,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2019-12-21T06:19:15+00:00",
"created_by": 1,
"updated_by": 1,
"logged_in_at": "2019-12-19 06:40:30+00",
"logged_in_from": "172.19.0.1",
"gravatarLink": "https://www.gravatar.com/avatar/30284b632a71f240d086e3d877b3e70d?s=100&d=mm&r=g",
"appState": null,
"profilePicUrl": null,
"defaultLocale": "en-US",
"countryCode": "+1",
"_links": {
"self": {
"href": "/user/me"
},
"permissions": {
"href": "/user/me/permission?type=2"
},
"settings_data": {
"href": "/user/me/settings_data"
},
"index": {
"href": "/user"
},
"metrics": {
"href": "/user/metrics/41"
},
"categories": {
"href": "/models/user-category?user_id=41"
},
"busyCalendar": {
"href": "/models/user-calendar?type=3&user_id=41&startsAt=1576909155"
},
"liveCalendar": {
"href": "/models/user-calendar?type=1&user_id=41&startsAt=1576909155"
},
"availability": {
"href": "/models/user-calendar?type=2&user_id=41&startsAt=1576909155"
},
"userProfilePic": {
"href": "/user/display-picture"
}
}
}
HTTP Request
POST https://apiv4.talview.com/models/user
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
text | true | Email address of the User | |
username | text | true | The username of the User. The email address of an user is the Username to the user |
name | text | false | The name of the User |
location | text | false | The location of the user. Example: Bangalore |
external_id | text | false | The reference Id for the object |
roles | array of texts | false | The role assigned to an User. Example: Recruiter, Evaluator |
status | integer | false | The status of an user. Example: Active, Inactive, Suspended |
phone | integer | false | The phone number of the user |
countryCode | string | false | The country code of the user |
Update an user
PUT /models/user/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
{
"id": 41,
"external_id": "",
"username": "user+tumgoucxkw2@talview.com",
"name": "sahana",
"location": "",
"is_common_login": true,
"email": "user+tumgoucxkw2@talview.com",
"roles": [
"Account Manager",
"Content Manager",
"Evaluator",
"Hiring Manager",
"Master Hiring Manager",
"Master Recruiter",
"Recruiter",
"Sourcer"
],
"organization_id": 2,
"phone": "8253497844",
"current_role": "Account Manager",
"status": 10,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2019-12-21T06:19:15+00:00",
"created_by": 1,
"updated_by": 1,
"logged_in_at": "2019-12-19 06:40:30+00",
"logged_in_from": "172.19.0.1",
"gravatarLink": "https://www.gravatar.com/avatar/30284b632a71f240d086e3d877b3e70d?s=100&d=mm&r=g",
"appState": null,
"profilePicUrl": null,
"defaultLocale": "en-US",
"countryCode": "+1",
"_links": {
"self": {
"href": "/user/me"
},
"permissions": {
"href": "/user/me/permission?type=2"
},
"settings_data": {
"href": "/user/me/settings_data"
},
"index": {
"href": "/user"
},
"metrics": {
"href": "/user/metrics/41"
},
"categories": {
"href": "/models/user-category?user_id=41"
},
"busyCalendar": {
"href": "/models/user-calendar?type=3&user_id=41&startsAt=1576909155"
},
"liveCalendar": {
"href": "/models/user-calendar?type=1&user_id=41&startsAt=1576909155"
},
"availability": {
"href": "/models/user-calendar?type=2&user_id=41&startsAt=1576909155"
},
"userProfilePic": {
"href": "/user/display-picture"
}
}
}
HTTP Request
PUT https://apiv4.talview.com/models/user/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
text | false | Email address of the User | |
username | text | false | The username of the User. The email address of an user is the Username to the user |
name | text | true | The name of the User |
location | text | false | The location of the user. Example: Bangalore |
external_id | text | false | The reference Id for the object |
roles | array of texts | false | The role assigned to an User. Example: Recruiter, Evaluator |
status | integer | false | The status of an user. Example: Active, Inactive, Suspended |
phone | integer | false | The phone number of the User |
current_role | text | false | The role of the User currently working as |
appState | jsonb | false | The information of the application. Example: "web": {"releaseShown": ["v4.0.0"]} |
countryCode | string | false | The countryCode of the user |
Delete a User
DELETE models/user/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns the status code : 204 No Content
This endpoint deletes a user whose organization_id is same as that of the logged in user.
HTTP Request
DELETE https://apiv4.talview.com/models/user/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The id of the user to be deleted |
Get Organization details of an user
GET /user/organization/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"id": 488,
"name": "Talview Dev Team Testing",
"external_id": "80909",
"domain": null,
"address1": "117/Q/184, Indrapuri",
"address2": "Sharda Nagar",
"city": "Bangalore",
"state": "Karnataka",
"country": "India",
"pincode": null,
"phone": "9933323319",
"isInterview": true,
"isLive": true,
"isObjective": true,
"isEssay": true,
"isCode": true,
"isEvaluation": true,
"promo": null,
"isTyping": true,
"isSimulation": true,
"logo": "https://assets.talview.com/clients/488/1513147530_logo.png",
"advancedProctorV3Enabled": true,
"isUploadPreForm": false,
"defaultFont": "Roboto, sans-serif",
"isPearsonEnabled": false,
"created_by": 1,
"updated_by": 1,
"senderEmailEnabled": false,
"mailDateTimeFormat": "full",
"isPublicAttachment": true,
"updated_at": "2019-02-14 06:32:45+00",
"theme": "default",
"hideCandidateInfoPanel": false,
"termsLink": "https://pages.talview.com/terms/",
"hasAssessmentCreate": true,
"logoutUrl": "/login",
"timeZone": "Etc/GMT+10",
"isAdhocLive": false,
"isIconEvaluation": false,
"created_at": "2017-11-03 15:42:05+00",
"live_bulk_schedule_enabled":true,
"bulk_schedule_url" : "https://recruit.talview.com/test",
"isBpmEnabled": false,
"isBfiEnabled": false
}
This endpoint retrieves details of the organization of current user
HTTP Request
GET https://apiv4.talview.com/user/organization/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
ID | integer | true | The Id of the Organization |
Reset Password
POST user/reset-password/change HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"new_password": "test-password",
"new_password_repeat": "test-password",
"token": "0YlbnJqtLgoDhAE97yk8U4kIdTy2CB645GJFfFdI5gY6t9PLS6qXG2vH2bbT7_DD29mtuwkVoW1nIB_6PsUBPbLVcayg1FDD5MAcMrDbe5tKwal42q7_totv"
}
The above request returns JSON structured like this:
{
"id": "9133f756-0d12-11ea-b672-ace04def3892"
}
The endpoint is used to reset a password.
HTTP Request
POST https://apiv4.talview.com/user/reset-password/change
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
new_password | text | true | password to be set |
new_password_repeat | text | true | same password as new password |
token | text | true | token received in the email when requested for a password reset |
Verify Password Reset Link
GET user/reset-password/verify-link?c=0YlbnJqtLgoDhAE97yk8U4kIdTy2CB645GJFfFdI5gY6t9PLS6qXG2vH2bbT7_DD29mtuwkVoW1nIB_6PsUBPbLVcayg1FDD5MAcMrDbe5tKwal42q7_totv HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
{
"message": "valid link",
"status": 200
}
The endpoint is used to verify the password reset link that is received through an email to the user when requested for a password reset.
HTTP Request
GET https://apiv4.talview.com/user/reset-password/verify-link?c=<password-reset-token>
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
c | text | true | the token received when requested for a password reset |
User Calendar
User calendar provides the schedule of an user. You can check if an user has any scheduled event for a particular period of time. This helps you to check the availability of an user to schedule a live session.
The User Calendar object
{
"id": 3042793,
"from": "2019-03-13 05:10:00+00",
"to": "2019-03-13 07:10:00+00",
"eventType": "live-schedule",
"isAllDay": false,
"created_at": "2019-03-13 04:59:20+00",
"updated_at": "2019-03-13 04:59:20+00",
"event": "Live Session with X for Essay Parameters Checking ",
"type": 3,
"user_id": 45331,
"_links": {
"user": {
"href": "/models/user?id=45331"
},
"self": {
"href": "/models/user-calendar?id=3042793"
}
}
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
from | timestamptz | The time from when an event is scheduled |
to | timestamptz | The time when an event ends |
eventType | text | The type of the event. Example: Available, Busy. The event types are described in the enumerator list below |
isAllDay | boolean | Whether the event is of whole day |
event | text | The name of the event |
type | integer | The key to an event type. The keys to the event types are mentioned in the enumerator list below |
user_id | integer | The Id of the user associated to an user calendar object |
List of Event Types:
Event Types | Key | Description |
---|---|---|
Live-Schedule | 1 | A live session event has been scheduled |
Available | 2 | The user is available for an event |
Busy | 3 | The user is busy in an event |
Tentative | 4 | The schedule is not certain |
List all User Calendars
GET models/user-calendar HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The above request returns JSON structured like this:
[
{
"id": 3007230,
"from": "2019-01-14 13:02:06+00",
"to": "2019-01-14 14:02:06+00",
"eventType": "live-schedule",
"isAllDay": false,
"event": "Live Session with X for Essay Parameters Checking",
"type": 3,
"user_id": 30876,
"_links": {
"user": {
"href": "/models/user?id=30876"
},
"self": {
"href": "/models/user-calendar?id=3007230"
}
}
}
{
...
}
]
This endpoint returns all existing user calendars.
HTTP Request
GET https://apiv4.talview.com/models/user-calendar
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | false | Filter records based on the Id of the object |
ids | array of integer | false | Filter records based on the Ids of the objects |
from | timestamptz | false | The time from when an event is scheduled |
to | timestamptz | false | The time when an event ends |
type | integer | false | The key to an event type. The keys are mentioned in the enumerator list above |
user_id | integer | false | The Id of the user associated to an user calendar object |
Create an User Calendar
This endpoint creates a new user calendar. An user must have the role of evaluator to create an user calendar.
POST /user/me/calendar HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"from": "2019-04-06T13:00:00.000Z",
"to": "2019-04-06T14:00:00.000Z",
"event": "Live Disussion with Mr. Smith",
"type" : 1
}
The above request returns JSON structured like this:
{
"id": 3010010,
"from": "2019-04-06T13:00:00.000Z",
"to": "2019-04-06T14:00:00.000Z",
"eventType": null,
"isAllDay": false,
"created_at": "2019-04-05T10:10:14+00:00",
"updated_at": "2019-04-05T10:10:14+00:00",
"event": "Live Disussion with Mr. Smith",
"type": 1,
"user_id": 35432,
"_links": {
"user": {
"href": "/models/user?id=35432"
},
"self": {
"href": "/models/user-calendar?id=3010010"
}
}
}
HTTP Request
POST https://apiv4.talview.com/user/me/calendar
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
from | timestamptz | true | The time from when an event is scheduled |
to | timestamptz | true | The time when an event ends |
event | text | true | The name of the event |
type | integer | true | The key to an event type. The keys to the event types are mentioned in the enumerator list |
Update an User Calendar
The endpoint updates an user calendar.
PUT /user/me/calendar/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
{
"from": "2019-04-07T13:00:00.000Z",
"to": "2019-04-07T14:00:00.000Z",
"event": "Live Disussion with Mr. Smith",
"type" : 1,
"date": "2019-04-07T13:00:00.000Z"
}
The above command returns JSON structured like this:
{
"id": 3010010,
"from": "2019-04-07T13:00:00.000Z",
"to": "2019-04-07T14:00:00.000Z",
"eventType": null,
"isAllDay": false,
"created_at": "2019-04-05 15:40:14+05:30",
"updated_at": "2019-04-05T10:35:16+00:00",
"event": "Live Disussion with Mr. Smith",
"type": 1,
"user_id": 35432,
"_links": {
"user": {
"href": "/models/user?id=35432"
},
"self": {
"href": "/models/user-calendar?id=3010010"
}
}
}
HTTP Request
PUT https://apiv4.talview.com/user/me/calendar/<id>
URL Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The unique identifier of the object |
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
from | timestamptz | false | The time from when an event is scheduled |
to | timestamptz | false | The time when an event ends |
type | integer | false | The key to an event type. The keys are mentioned in the enumerator list above |
event | text | false | The name of the event |
Permission
The Permission endpoint gives the details of the permissions/access given to a user/organization.
The Permission Object
{
"created_at": 1556236219,
"id": 435367,
"item_name": "event.create",
"organization_id": 488,
"type": 2,
"updated_at": 1556236219,
"user_id": null
}
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
item_name | text | The name of the auth_item |
organization_id | boolean | The id of the organization |
type | integer | The type of permission given |
user_id | integer | The id of the user |
created_at | timestamp | The timestamp when the permission was created |
updated_at | timestamp | The timestamp when the permission was updated |
Id | Type |
---|---|
1 | TYPE_ROLE |
2 | TYPE_PERMISSION |
List all permissions
GET /user/me/permission HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The Response
[
{
"created_at": 1556236219,
"id": 435367,
"item_name": "event.create",
"organization_id": 488,
"type": 2,
"updated_at": 1556236219,
"user_id": null
}
]
This endpoint returns all the permissions given to a user or organization. The user will by default get all the permissions given to the organization but not vice versa.
HTTP Request
GET https://apiv4.talview.com/user/me/permission
Query Parameters
Parameter | Type | is optional | Description |
---|---|---|---|
type | integer | false | Filter records based on the type of the object |
View a Permission
GET /user/me/permission/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
The Response
{
"created_at": 1556236219,
"id": 435367,
"item_name": "event.create",
"organization_id": 488,
"type": 2,
"updated_at": 1556236219,
"user_id": null
}
This endpoint return the permission based on the auth_assignment id.
HTTP Request
GET https://apiv4.talview.com/user/me/permssion/<id>
Query Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
id | integer | true | The Id of the auth assignment model |
Actions
Actions are designed to perform multiple operations in single api request.
The Action Object
All the listed actions inherit this base Action object. All Action API endpoints have the following common response parameters.
Parameter | Type | Description |
---|---|---|
id | inetger | The unique identifier of an object |
external_id | text | The external id of an action |
name | text | The name of the action |
comment | text | Comments are reference notes for an user when performing an action |
status | integer | The status of the action |
type | integer | The type of the action |
skipCallback | boolean | If FALSE, skipcallback will do callback to specified url |
total_count | integer | The total number of task an action does while doing bulk operation |
error_count | integer | The number of times an action failed to complete a task while doing bulk operation |
success_count | integer | The number of times an action compleye a task successfully while doing bulk operation |
completed_at | timestamptz | The time when the action was completed |
requested_at | timestamptz | The time when the action was requested |
started_at | timestamptz | The time when the action was started |
last_attempt_at | timestamptz | The time when the last attempt was made |
requested_by | integer | The id of the user who requested the panel |
isNotify | boolean | If TRUE, a notification will be sent |
created_at | timestamptz | The time when the action was created |
updated_at | timestamptz | The time when the action was updated |
response | text | Response includes the error messages. Example: Unable to process your request |
List of Status:
Status | Key | Description |
---|---|---|
STATUS_DELETED | 0 | When ac action is deleted |
STATUS_REQUESTED | 1 | The status when requesting for an action |
STATUS_PROCESSING | 2 | When an action is in progress |
STATUS_COMPLETED | 3 | When an action is completed successfully |
Add Panel
The action Add Panel is used to add a new panel to an Assessment Section Candidate. While making a POST request, the endpoint checks for an old Panel Candidate, if found, it replaces the old Panel with a new one and creates a new User Calendar.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/add-panel HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"endDate": "2019-04-15T10:17:14.+0530",
"assessmentSections": {
"id": 6983414
},
"isNotify": true,
"panel": [
{
"id": 30953
}
],
"assessmentCandidates": [
{
"id": 2201713
}
]
}
The above request returns JSON structured like this:
{
"id": 3341121,
"external_id": "6a3d2ab2-57a2-11e9-823c-000d3a249d3a",
"name": null,
"comment": null,
"status": 1,
"type": 7,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-05T12:57:49+00:00",
"updated_at": "2019-04-05T12:57:49+00:00",
"response": null,
"assessmentCandidates": [
{
"id": 2201713
}
],
"assessmentSections": {
"id": 6983414
},
"endDate": "2019-04-15T10:17:14.+0530",
"panel": [
{
"id": 30953
}
],
"oldPanel": []
HTTP Request
POST https://apiv4.talview.com/actions/add-panel
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
endDate | timestamptz | true | End Date for an action |
assessmentSections | object | true | Assessment Section object |
isNotify | boolean | false | If true, notification will be sent to the panel |
panel | Array [{ id: Integer }] |
true | Array of object with fields as id of the panel |
assessmentCandidates | Array [{ id: Integer }] |
false | Array of object with fields as id of Assessment Candidate |
Change Status
The action Change Status is used to change the status of an Assessment Candidate. Example: From Invited to Selected. The provision of changing status is available only for Master Recruiter and Recruiter. The action also provides an option to send notification to the candidate for SELECT and REJECT status.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/change-status HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"comment": null,
"status": null,
"type": null,
"total_count": 0,
"error_count": 0,
"success_count": 0,
"isNotify": false,
"message": null,
"reasonText": null,
"statusSortKey": 70,
"assessmentCandidates": [
2206831
],
"requested_by": null
}
The above request returns JSON structured like this:
{
"id": 3345129,
"external_id": "784706da-59d4-11e9-8cea-000d3a249d3a",
"name": null,
"comment": null,
"status": 1,
"type": null,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": false,
"message": null,
"created_at": "2019-04-08T08:01:09+00:00",
"updated_at": "2019-04-08T08:01:09+00:00",
"response": null,
"assessmentCandidates": [
2206831
],
"statusSortKey": 70,
"reasonText": null,
"reasonKey": null
}
HTTP Request
POST https://apiv4.talview.com/actions/change-status
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
statusSortKey | integer | True | The key value for sort status |
assessmentCandidates | Array [ id: Integer ] |
True | Array of object with fields as id of the Assessment Candidate |
isNotify | boolean | True | If true, the candidate will be notified |
List of Status Sort Key:
Status | Status Sort Key | Description |
---|---|---|
SELECT | 70 | To select a candidate |
HOLD | 80 | To keep a candidate on hold |
REJECT | 90 | To reject a candidate |
SUSPEND | 100 | To suspend a candidate |
ARCHIVE | 120 | To archive a candidate |
Create Assessment
The action Create Assessment is used to create an assessment.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/create-assessment HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"title": "Assessment",
"start_date": "2019-03-23T13:20:11.121Z",
"end_date": "2019-04-20T13:10:11.125Z",
"assessmentStatus": 3,
"description": "Assessment Description"
"sections": [
{
"order": 1,
"title": "Objective Test",
"section_type_id": 6,
"positive_mark": 1,
"negative_mark": 0,
"isPlaylist": true,
"is_review": false,
"is_proctor": true,
"isSkipAllowed": true,
"is_screenshare": false,
"max_section_time": 60,
"combined": [
{
"question_id": 84108,
"sort_order": 1
}
]
}
]
}
The above request returns JSON structured like this:
{
"id": 3345276,
"external_id": null,
"name": null,
"comment": null,
"status": 3,
"type": 14,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": "2019-04-08T09:30:59+00:00",
"requested_at": null,
"started_at": "2019-04-08T09:30:59+00:00",
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": null,
"message": null,
"created_at": "2019-04-08T09:30:59+00:00",
"updated_at": "2019-04-08T09:30:59+00:00",
"response": null,
"title": "Assessment",
"externalID": null,
"templateID": null,
"description": "Assessment Description",
"start_date": "2019-03-23T13:20:11.121Z",
"assessmentStatus": 3,
"end_date": "2019-04-20T13:10:11.125Z",
"cf": null,
"owned_by": null,
"theme": null,
"pre_page": null,
"form_url": null,
"post_url": null,
"promo": null,
"is_practice": null,
"sections": [
{
"order": 1,
"title": "Objective Test",
"section_type_id": 6,
"positive_mark": 1,
"negative_mark": 0,
"isPlaylist": true,
"is_review": false,
"is_proctor": true,
"isSkipAllowed": true,
"is_screenshare": false,
"max_section_time": 60,
"combined": [
{
"question_id": 84108,
"sort_order": 1
}
]
}
],
"_links": {
"assessment": {
"href": "/models/assessment/884947"
}
}
}
HTTP Request
POST https://apiv4.talview.com/actions/create-assessment
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
title | text | true | Title of the assessment |
start_date | timestamptz | false | The time from when the assessment is active |
end_date | timestamptz | false | The time when the assessment expires |
assessmentStatus | integer | false | The status of an assessment Example: Open, Draft |
description | text | false | Description of the assessment |
sections | Array of object | true | Section Object Section object contains Section Combined object as provided in the sample request |
List of Assessment Status:
Status | Key | Description |
---|---|---|
Draft | 1 | When no candidate is assigned to an assessment |
Closed | 2 | The assessment is closed |
Open | 3 | The assessment is open for candidate |
Filled | 4 | The requirement of positions is filled |
Evaluate
The action Evaluate is used to evaluate an Assessment Section Candidate.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
- Evaluator
POST /actions/evaluate HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"assessmentSectionCandidateID": 2364848,
"recommendation": "select",
"evaluationComment": "Good communication skill",
"rating": [
{
"parameter_id": 127775,
"rating": 3
},
{
"parameter_id": 127765,
"rating": 16
}
]
}
The above request returns JSON structured like this:
{
"id": 3345458,
"external_id": null,
"name": null,
"comment": null,
"status": 3,
"type": 19,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": 1,
"completed_at": "2019-04-08T10:58:02+00:00",
"requested_at": null,
"started_at": "2019-04-08T10:58:02+00:00",
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": null,
"message": null,
"created_at": "2019-04-08T10:58:02+00:00",
"updated_at": "2019-04-08T10:58:02+00:00",
"response": null,
"assessmentSectionCandidateID": 2364848,
"rating": [
{
"parameter_id": 127775,
"rating": 3
},
{
"parameter_id": 127765,
"rating": 16
}
],
"evaluationComment": "Good communication skill",
"formData": null,
"formInstanceID": null,
"files": [
null
],
"recommendation": "select",
"_links": {
"evaluation": {
"href": "/models/evaluation/12846637"
}
}
}
HTTP Request
POST https://apiv4.talview.com/actions/evaluate
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessmentSectionCandidateID | Integer | true | The unique identifier of the Assessment Section Candidate object |
recommendation | text | true | The evaluation result. Example: Select , Reject, Hold |
evaluationComment | text | false | Comment given by the evaluator |
rating | Array of object | false | Rating given in different Parameter as provided in the sample request |
Event Candidate Invite
The action Event Candidate Invite is designed to invite a candidate to an Event.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/event-candidate-invite HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"isNotify": true,
"candidates": {
"_content": {
"email": "tujit.bora+1@talview.com",
"first_name": "tujit",
"middle_name": null,
"last_name": "bora",
"phone": "1234567890",
"external_id": null
}
},
"event_id": "660"
}
The above request returns JSON structured like this:
{
"id": 4063641,
"external_id": "22c190e8-9734-11e9-b039-000d3a249d3a",
"name": null,
"comment": null,
"status": 3,
"type": 21,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": "2019-06-25T10:29:39+00:00",
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-06-25T10:29:38+00:00",
"updated_at": "2019-06-25T10:29:38+00:00",
"response": null,
"candidates": {
"_content": {
"email": "tujit.bora+1@talview.com",
"first_name": "tujit",
"middle_name": null,
"last_name": "bora",
"phone": "1234567890",
"external_id": null
}
},
"event_id": "660",
"has_select_assessment": null,
"assessment_section_id": null,
"registration_end_date": null
}
HTTP Request
POST https://apiv4.talview.com/actions/event-candidate-invite
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
isNotify | boolean | false | If true, the candidate will be notified when he/she is invited |
candidates | object | true | The candidate object to be invited to an event |
event_id | integer | true | The id of the Event |
Event Candidate Notify
The action Event Candidate Notify is designed to remind the candidates invited/registered to an event.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/event-candidate-notify HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"attend_reminder": false,
"register_reminder": true,
"event_id": "660"
}
The above request returns JSON structured like this:
{
"id": 4062971,
"external_id": null,
"name": null,
"comment": null,
"status": 1,
"type": 22,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": null,
"message": null,
"created_at": "2019-06-25T09:56:22+00:00",
"updated_at": "2019-06-25T09:56:22+00:00",
"response": null,
"event_id": "660",
"eventCandidates": [],
"register_reminder": true,
"attend_reminder": null
}
HTTP Request
POST https://apiv4.talview.com/actions/event-candidate-notify
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
event_id | timestamptz | true | The id of the event |
register_reminder | boolean | false | If true, the registered candidates will be notified to attend the event |
attend_reminder | boolean | false | If true, the invited candidates who has not registered will be notified to register |
Event Panel Notify
The action Event Panel Notify is used to send reminder email to the panel members about the assessment they need to evaluate in the event they were invited in.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
{
"event_id": "633",
"eventUsers": [
{
"id": "1135"
}
],
"attend_reminder": false,
"register_reminder": false
}
HTTP Request
POST https://apiv4.talview.com/actions/event-panel-notify
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
event_id | integer | true | The unique identifier of an Event |
eventUsers | array/integer | false | The id/ids of Event User who has to recieve the reminder email |
The above request returns JSON structured like this:
[
{
"id": 382,
"external_id": null,
"name": null,
"comment": null,
"status": 3,
"type": 24,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": "2020-05-27T04:56:15+00:00",
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 41,
"isNotify": null,
"message": null,
"created_at": "2020-05-27T04:56:15+00:00",
"updated_at": "2020-05-27T04:56:15+00:00",
"response": null,
"event_id": "30",
"eventUsers": [
{
"id": "1200"
}
],
"register_reminder": false,
"attend_reminder": false,
"_links": []
}
]
Extend Date
The action Extend Date is used to extend the last date of an Assessment Candidate. It extends the last date for attending an assessment for a single candidate as well as multiple candidates. It also provides an option to send notification to the candidate notifying the updated date.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/extend-date HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"isNotify": true,
"endDate": "2019-04-18T11:46:23.466Z",
"assessmentCandidates": [
{
"id": 2210485
}
]
}
The above request returns JSON structured like this:
{
"id": 3346213,
"external_id": "5af7291a-59f8-11e9-b8af-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 5,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-08T12:18:02+00:00",
"updated_at": "2019-04-08T12:18:02+00:00",
"response": null,
"assessmentCandidates": [
{
"id": 2210485
}
],
"endDate": "2019-04-18T11:46:23.466Z"
}
HTTP Request
POST https://apiv4.talview.com/actions/extend-date
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
isNotify | boolean | true | If true, a notification will be sent to the candidate |
endDate | timestamptz | true | The updated last date to attend the assessment |
assessmentCandidates | Array of object [ id: Integer ] |
True | Array of object with fields as id of the Assessment Candidate |
Invite
The action Invite is used to invite a candidate to an assessment.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/invite HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"assessmentSectionID": 7125672,
"isNotify": true,
"endDate": "2019-04-18T13:06:04.155Z",
"candidates": [
{
"id": 6828106
}
]
}
The above request returns JSON structured like this:
{
"id": 3346631,
"external_id": "fb46fe66-59ff-11e9-9f77-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 1,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-08T13:12:37+00:00",
"updated_at": "2019-04-08T13:12:37+00:00",
"response": null,
"callbackUrl": null,
"candidates": [
{
"id": 6828106
}
],
"assessmentID": null,
"externalAssessmentID": null,
"assessmentSectionID": 7125672,
"endDate": "2019-04-18T13:06:04.155Z"
}
HTTP Request
POST https://apiv4.talview.com/actions/invite
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessmentSectionID | integer | true | The unique identifier of the Assessment Section object |
assessmentID | integer | false | The unique identifier of the Assessment object |
isNotify | boolean | false | If true, a notification will be sent to the candidate |
endDate | timestamptz | true | The date when the assessment will expire |
candidates | Array of objects | true | The id of the Candidate/Candidates to be invited to an assessment |
Live Cancel
The action Live Cancel is used to cancel any of the Live Sessions scheduled for a Candidate. It also provides an option to send notification to the candidate notifying about the cancelation of live schedule.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/live-cancel HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"liveSessionID":368594383,
"isNotify": true
}
The above request returns JSON structured like this:
{
"id": 3349254,
"external_id": "30530730-5aaf-11e9-a1a1-000d3a249d3a",
"name": null,
"comment": null,
"status": 1,
"type": 9,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-09T10:06:48+00:00",
"updated_at": "2019-04-09T10:06:48+00:00",
"response": null,
"liveSessionID": 368594383
}
HTTP Request
POST https://apiv4.talview.com/actions/live-cancel
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
liveSessionID | Integer | true | The Id of Live Sessions |
is_notify | boolean | false | If true, the candidate will be notified regarding the cancel of the live session |
Live Change Panel
The action Live Change Panel is used to change the Panel associated to a Live Session. While making a POST request on the Live Change Panel endpoint, the panel already assigned to a live session is replaced with a new panel member.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/live-change-panel HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"liveSessionID": 104910,
"panel": [
{
"id": 67656
},
{
"id": 30876
},
{
"id": 45285
}
],
"isNotify": true
}
The above request returns JSON structured like this:
{
"id": 3349720,
"external_id": "2f644cae-5abd-11e9-9535-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 11,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-09T11:47:00+00:00",
"updated_at": "2019-04-09T11:47:00+00:00",
"response": null,
"liveSessionID": 104910
}
HTTP Request
POST https://apiv4.talview.com/actions/live-change-panel
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
liveSessionID | Integer | true | Id of Live Session |
panel | Array [{id: Integer}] |
true | Array of object with fields as id of the new Panel |
isNotify | boolean | false | If true, notification will be sent to the newly added panel |
Live Reminder
The action Live Reminder is used to send reminder mails to the candidate, the Panel members and the recruiter regarding the Live Session scheduled by the organizer.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/live-reminder HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"isNotify": false,
"liveSessionID": [
{
"id": 104886
}
]
}
The above request returns JSON structured like this:
{
"id": 3349694,
"external_id": "aaf745e8-5abc-11e9-8912-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 12,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": null,
"message": null,
"created_at": "2019-04-09T11:43:18+00:00",
"updated_at": "2019-04-09T11:43:18+00:00",
"response": null,
"liveSessionID": [
{
"id": 104886
}
],
"liveAttendees": []
}
HTTP Request
POST https://apiv4.talview.com/actions/live-reminder
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
liveSessionID | Integer | true | Id of Live Session |
isNotify | boolean | false | If true, notification will be sent to all Live Attendees |
Live Schedule
The action Live Schedule is used to schedule a Live Session for a Candidate.It also provides an option to send notification to the Live Attendee regarding the scheduled live session.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/live-schedule HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"startDate": "2019-04-09T14:12:36.760Z",
"endDate": "2019-04-09T15:12:36.760Z",
"panel": [
{
"id": 67656
},
{
"id": 30876
},
{
"id": 45285
}
],
"assessmentCandidateID": 2206746,
"isNotify": true
}
The above request returns JSON structured like this:
{
"id": 3349734,
"external_id": "af234ec2-5abd-11e9-b13f-000d3a2717d9",
"name": null,
"comment": null,
"status": 99,
"type": 8,
"skipCallback": null,
"total_count": 0,
"error_count": 0,
"success_count": 0,
"completed_at": null,
"requested_at": "2019-04-09 11:50:35+00",
"started_at": "2019-04-09 11:50:34+00",
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-09 11:50:34+00",
"updated_at": "2019-04-09 11:50:34+00",
"response": "\"Invalid configuration\"",
"assessmentCandidateID": 2206746,
"panelMessage": null,
"endDate": "2019-04-09T15:12:36.760Z",
"startDate": "2019-04-09T14:12:36.760Z",
"panel": [
{
"id": 67656
},
{
"id": 30876
},
{
"id": 45285
}
],
"candidateMessage": null,
"askCandidate": false,
"askPanel": false,
"location": null,
"isOffline": null,
"assessmentSectionID": null,
"timezone": null,
"candidateID": null,
"currentTimezone": null,
"currentOffset": null,
"_links": []
}
HTTP Request
POST https://apiv4.talview.com/actions/live-schedule
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
endDate | Timestamp | true | The date and time when the live session expires |
startDate | Timestamp | true | The date and time from when the live session is active |
panel | Array [{ id: Integer }] | true | Array of object with fields as id of the Panel for the live session |
assessmentCandidateID | integer | false | The id of Assessment Candidate |
isNotify | boolean | false | If true, notification will be sent to all Live Attendees about the live schedule |
Live Reschedule
The action Live Reschedule is used to prepone or postpone the Live Session scheduled by an organizer for a candidate. While making a POST request on the Live Reschedule action, the start_date or end_date of the Live Session is replaced with a new date.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/live-reschedule HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"startDate": "Tue, 09 Apr 2019",
"timezone": "Asia/Kolkata",
"endDate": "Tue, 09 Apr 2019",
"liveSessionID": 97286,
"isNotify": true,
}
The above returns returns JSON structured like this:
{
"id": 3349819,
"external_id": "94f1c144-5abf-11e9-8654-000d3a249d3a",
"name": null,
"comment": null,
"status": 1,
"type": 10,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-09T12:04:09+00:00",
"updated_at": "2019-04-09T12:04:09+00:00",
"response": null,
"liveSessionID": 97286,
"startDate": "Tue, 09 Apr 2019",
"timezone": "Asia/Kolkata",
"endDate": "Tue, 09 Apr 2019",
"currentOffset": null,
"currentTimezone": null
}
HTTP Request
POST https://apiv4.talview.com/actions/live-reschedule
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
endDate | Timestamp | true | The updated date and time when the live session expires |
startDate | Timestamp | true | The updated date and time from when the live session is active |
liveSessionID | integer | false | Id of the Live Session |
timezone | String | true | Timezone of the Recruiter |
isNotify | boolean | false | If true, notification will be sent to all Live Attendees about the updated schedule. |
Re Invite
The action Reinvite is used to re invite single or multiple candidates to an Assessment Section. If the assessment_section_id value is set then this action will re invite candidates for that particular assessment section else candidates will be re invited for whole Assessment.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/re-invite HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"isNotify": true,
"assessmentCandidates": [
2206746
],
"assessmentSectionID": 128203
}
The above request returns JSON structured like this:
{
"id": 3350056,
"external_id": "bf913bb8-5ac5-11e9-aa5d-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 13,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": null,
"message": null,
"created_at": "2019-04-09T12:48:18+00:00",
"updated_at": "2019-04-09T12:48:18+00:00",
"response": null,
"assessmentSectionID": null,
"assessmentCandidates": [
2206746
],
"endDate": null,
"assessmentSectionCandidateID": null
}
HTTP Request
POST https://apiv4.talview.com/actions/re-invite
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessment_section_id | integer | false | The Id of the Assessment Section |
assessmentCandidates | Array [{ id: Integer }] |
true | Array of object with fields as id of Assessment Candidate |
isNotify | boolean | true | If true, notification will be sent to the candidate notifying re invite to the assessment |
Remind Candidate
The action Remind Candidate is used to send a reminder notification mail to the Candidate regarding the Assessment scheduled by the recruiter.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/remind-candidate HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request: (When reminder has to be sent to selected assessment candidates)
{
"assessmentCandidates": [
{
"id": 2215547
}
],
"isNotify": true,
"endDate": "2019-04-20T06:33:40.977Z"
}
The above request returns JSON structured like this:
{
"id": 3352538,
"external_id": "e270c9ba-5b5a-11e9-93e9-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 4,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-10T06:35:51+00:00",
"updated_at": "2019-04-10T06:35:51+00:00",
"response": null,
"assessmentCandidates": [
{
"id": 2215547
}
],
"endDate": "2019-04-20T06:33:40.977Z"
}
JSON POST Request: (When reminder has to be sent to all the assessment candidates in the section, who are in invited or in-progress status)
{
"assessmentSections": [
{
"id": 381499
}
],
"isNotify": true,
"endDate": "2019-04-20T06:33:40.977Z"
}
The above request returns JSON structured like this:
{
"id": 3352538,
"external_id": "e270c9ba-5b5a-11e9-93e9-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 4,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-10T06:35:51+00:00",
"updated_at": "2019-04-10T06:35:51+00:00",
"response": null,
"assessmentSections": [
{
"id": 381499
}
],
"endDate": "2019-04-20T06:33:40.977Z"
}
HTTP Request
POST https://apiv4.talview.com/actions/remind-candidate
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessmentCandidates | Array [{ id: Integer }] |
false | Array of object with fields as id of Assessment Candidate |
isNotify | boolean | true | If true, notification will be sent to the candidate notifying re binvite to the Assessment |
endDate | Timestamp | false | The date and time when the assessment expires |
assessmentSections | Array [{ id: Integer }] |
false | Array of object with assessment section ids |
Remind Panel
The action Remind Panel is used to send an email reminder to the assigned Panel members about Assessment Section they need to evaluate.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/remind-panel HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"isNotify": true,
"endDate": "2019-04-20T07:54:32.288Z",
"assessmentCandidates": [
{
"id": 2215547
},
{
"id": 2215440
}
]
}
The above request returns JSON structured like this:
{
"id": 3354398,
"external_id": "a826183a-5b66-11e9-bca5-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 3,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-10T08:00:07+00:00",
"updated_at": "2019-04-10T08:00:07+00:00",
"response": null,
"assessmentCandidates": [
{
"id": 2215547
},
{
"id": 2215440
}
],
"endDate": "2019-04-20T07:54:32.288Z",
"panel": null
}
HTTP Request
POST https://apiv4.talview.com/actions/remind-panel
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessmentCandidates | Array [{ id: Integer }] |
true | Array of object with fields as id of Assessment Candidate |
isNotify | boolean | true | If true, notification will be sent to the Panel notifying about the Assessment Candidate they are assigned to by the recruiter |
endDate | Timestamp | false | The date and time when the assessment expires |
Section Workflow
The action Section Workflow provides the facility to add or delete multiple sections to an existing assessment creating multiple Assessment Section.
Endpoint can be accessed by the following roles :
- Master Recruiter
- Recruiter
- API user
POST /actions/section-workflow HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"parent": 7194900,
"child": 7194899,
"assessmentID": 892847,
"type": "insert",
"section": {
"title": "Video Interview",
"section_type_id": "5",
"evaluation_form_id": null,
"evaluation_form_url": null,
"parameters": [],
"combined": [
{
"question_id": "89075",
"sort_order": 1
}
],
"isRetake": false,
"is_review": false,
"is_proctor": false,
"isPlaylist": false,
"isSkipAllowed": true,
"questionDisplayTime": 10,
"max_question_time": 180
},
"sync": true
}
The above request returns JSON structured like this:
{
"id": 3490619,
"external_id": null,
"name": null,
"comment": null,
"status": 3,
"type": "insert",
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": null,
"message": null,
"created_at": "2019-04-19T12:01:44+00:00",
"updated_at": "2019-04-19T12:01:44+00:00",
"response": null,
"parent": 7194900,
"child": 7194899,
"assessmentSectionID": null,
"assessmentID": 892847
}
HTTP Request
POST https://apiv4.talview.com/actions/section-workflow
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
parent | integer | true | The parent id of the assessment section order |
child | integer | true | The child id of the assessment section order |
assessmentID | integer | true | The id of the Assessment |
type | text | true | The type of section workflow. Example: insert, delete |
section | jsonb | true | The details of Section |
List of Types:
Event Types | Value | Description |
---|---|---|
DELETE | delete | To delete an existing section |
INSERT | insert | To add a new section |
Simulation Cancel
The action Simulation Cancel is used to cancel a Simulation Session invited by the recruiter. It also provides the provision for sending the notification mail to the Candidate as well as the Panel associated with the session.
POST /actions/simulation-cancel HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"assessmentSectionCandidateID": 2382572,
"isNotify": true
}
The above request returns JSON structured like this:
{
"id": 3356304,
"external_id": "597377c2-5b7b-11e9-8286-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 16,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-10T10:28:15+00:00",
"updated_at": "2019-04-10T10:28:15+00:00",
"response": null,
"assessmentSectionCandidateID": 2382572
}
HTTP Request
POST https://apiv4.talview.com/actions/simulation-cancel
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessmentSectionCandidateID | Integer | true | The unique identifier of the Assessment Section Candidate object |
isNotify | boolean | true | If true, notification will be sent to the Panel as well as Candidate notifying about the cancel of Simulation Session |
Simulation Reminder
The action Simulation Reminder is used to send reminder notification email to the Candidate as well as the Panel regarding the Simulation Session invited by the recruiter.
POST /actions/simulation-reminder HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"assessmentSectionCandidateID": 2382572,
"isNotify": true
}
The above request returns JSON structured like this:
{
"id": 3356304,
"external_id": "597377c2-5b7b-11e9-8286-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 16,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": true,
"message": null,
"created_at": "2019-04-10T10:28:15+00:00",
"updated_at": "2019-04-10T10:28:15+00:00",
"response": null,
"assessmentSectionCandidateID": 2382572
}
HTTP Request
POST https://apiv4.talview.com/actions/simulation-reminder
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessmentSectionCandidateID | Integer | true | The unique identifier of the Assessment Section Candidate object. |
isNotify | boolean | true | If true, a reminder notification mail will be sent to the Panel as well as the Candidate |
Simulation Reschedule
The action Simulation Reschedule is used to prepone or postpone the Simulation Session scheduled by the recruiter. While making a POST request, the start date and the end date of the session is replaced with the updated date. It also provides the provision for sending the notification mail to the candidate and the panel notifying the details of the updated schedule.
POST /actions/simulation-reschedule HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON POST Request:
{
"isNotify": false,
"endDate": "2019-04-12T09:25:00.000Z",
"startDate": "2019-04-12T08:55:00.000Z",
"assessmentSectionCandidateID": 2382572,
"timezone": "Asia/Kolkata"
}
The above request returns JSON structured like this:
{
"id": 3357111,
"external_id": "0d910b46-5b83-11e9-9887-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 17,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": false,
"message": null,
"created_at": "2019-04-10T11:23:23+00:00",
"updated_at": "2019-04-10T11:23:23+00:00",
"response": null,
"assessmentSectionCandidateID": 2382572,
"startDate": "2019-04-12T08:55:00.000Z",
"timezone": "Asia/Kolkata",
"endDate": "2019-04-12T09:25:00.000Z",
"currentOffset": null,
"currentTimezone": null
}
HTTP Request
POST https://apiv4.talview.com/actions/simulation-reschedule
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessmentSectionCandidateID | Integer | true | The unique identifier of the Assessment Section Candidate object |
isNotify | boolean | true | If true, notification mail will be sent to the Panel as well as Candidate notifying about the updated schedule of Simulation Session |
endDate | timestamptz | true | The updated date and time when the Simulation session expires |
startDate | timestamptz | true | The updated date and time from when the Simulation session is active |
timezone | string | false | Time zone of the recruiter |
Simulation Schedule
The action Simulation Reschedule is used to schedule the Simulation Session for the Candidate.It also provides the provision for sending the notification mail to the candidate as well as the panel notifying the details of the scheduled session.
POST /actions/simulation-schedule HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
{
"startDate": "2019-04-10T17:25:00.000Z",
"endDate": "2019-04-10T18:25:00.000Z",
"candidateID": 6833196,
"email": "abc@xyz.com",
"assessmentCandidateID": 2219008,
"assessmentSectionID": 842438,
"isNotify": true,
"timezone": "Asia/Kolkata",
"panel": [
{
"id": 30876
}
]
}
The above request returns JSON structured like this:
{
"id": 3357111,
"external_id": "0d910b46-5b83-11e9-9887-000d3a2717d9",
"name": null,
"comment": null,
"status": 1,
"type": 17,
"skipCallback": null,
"total_count": null,
"error_count": null,
"success_count": null,
"completed_at": null,
"requested_at": null,
"started_at": null,
"last_attempt_at": null,
"requested_by": 30876,
"isNotify": false,
"message": null,
"created_at": "2019-04-10T11:23:23+00:00",
"updated_at": "2019-04-10T11:23:23+00:00",
"response": null,
"assessmentSectionCandidateID": 2382572,
"startDate": "2019-04-12T08:55:00.000Z",
"timezone": "Asia/Kolkata",
"endDate": "2019-04-12T09:25:00.000Z",
"currentOffset": null,
"currentTimezone": null
}
HTTP Request
POST https://apiv4.talview.com/actions/simulation-schedule
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
assessmentCandidateID | Integer | true | The unique identifier of the Assessment Candidate object |
assessmentSectionCandidateID | Integer | true | The unique identifier of the Assessment Section Candidate object |
isNotify | boolean | true | If true, notification mail will be sent to the Panel as well as Candidate notifying about the schedule of Simulation Session |
endDate | timestamptz | true | The date and time when the Simulation session expires |
startDate | timestamptz | true | The date and time from when the Simulation session is active |
timezone | string | false | Time zone of the recruiter |
candidateID | Integer | false | The unique identifier of the Candidate object |
panel | Array [{ id: Integer }] |
false | Array of object with fields as id of the panel |
text | false | The email address of the Candidate |
Mobile
A Bearer Token must be added in the header in order to execute the mobile APIs.
Header Object
{
"Authorization" : "Bearer <Enter the token here>"
}
Answer Recording (Mobile)
Create an Answer Recording (Mobile)
POST /mobile/answer-recording HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Request:
{
"answer_id": "flash",
"file": "<file>",
"sequence": 1,
"type": "<type>"
}
JSON Response:
{
"id": 3576987,
"answer_id": 2664282,
"sequence": null,
"file_id": 8947570,
"file": {
"id": 8947570,
"name": "1528966508389_81481",
"external_id": null,
"description": "recording",
"file_type_id": 2,
"size": null,
"resourceUrl": "https://cdn3.talview.com/data/1528966508389_81481.flv?Expires=1529607936&Signature=JJq9Qvfq9c9hwOaqXDb7CdY9SKaBC2a8nAED1aQiWc9KGz9X8qN1M-y2qPrlIyhKcGTEYOXMNBI~PwBG~fYMv0MwpAP-E0e82H2AUs~x14F5S4Ur0NbKluLkS3J48SiexNe6RDxgIM7nddknNLKoswW5hR7Yqj6N-rOiyDkNqNs_&Key-Pair-Id=APKAIA7P6AHKG2K37MXA",
"extension": "flv",
"originalName": "1528966508389_81481.flv",
"_links": {
"fileType": {
"href": "/models/file-type/2"
}
}
}
}
Creates a new answer recording
HTTP Request
POST https://apiv4.talview.com/mobile/answer-recording
Body Parameters
Parameter | Type | Description | required |
---|---|---|---|
type | text | The type of the recording. It can be one value from the list ['webrtc','flash'] | true |
sequence | integer | Sequence of the answer recording | true |
answer_id | integer | The answer id | true |
file | binary | The file to be uploaded | true |
Answer (Mobile)
List all Answer (Mobile)
GET /mobile/answer HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
[
{
"id": 2687836,
"question": {
"id": 56659,
"type": "essay",
"title": "TEST",
"content": {
"text/html": "<p>Write a note on the last project which you worked on . </p>",
"text/plain": "Write a note on the last project which you worked on . "
},
"contentRaw": "<p>Write a note on the last project which you worked on . </p>",
"hint": null,
"choice_1": null,
"choice_2": null,
"choice_3": null,
"choice_4": null,
"choice_5": null,
"leftList": null,
"rightList": null,
"matchMap": null,
"tagData": null,
"testCase": null,
"updated_at": "2017-11-03 15:52:17+00"
},
"status": 3000,
"answered_at": "2018-06-20 05:58:16+00",
"choice": null,
"text": null,
"question_id": 56659,
"max_sequence": 0,
"submitted_files": [],
"_links": {
"question": {
"href": "/attend/question?id=56659"
}
}
}
]
HTTP Request
GET https://apiv4.talview.com/mobile/answer
Retrieve an Answer (Mobile)
GET /mobile/answer/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 2687836,
"question": {
"id": 56659,
"type": "essay",
"title": "TEST",
"content": {
"text/html": "<p>Write a note on the last project which you worked on . </p>",
"text/plain": "Write a note on the last project which you worked on . "
},
"contentRaw": "<p>Write a note on the last project which you worked on . </p>",
"hint": null,
"choice_1": null,
"choice_2": null,
"choice_3": null,
"choice_4": null,
"choice_5": null,
"leftList": null,
"rightList": null,
"matchMap": null,
"tagData": null,
"testCase": null,
"updated_at": "2017-11-03 15:52:17+00"
},
"status": 3000,
"answered_at": "2018-06-20 05:58:16+00",
"choice": null,
"text": null,
"question_id": 56659,
"max_sequence": 0,
"submitted_files": [],
"_links": {
"question": {
"href": "/attend/question?id=56659"
}
}
}
Retrieves the details of answer
HTTP Request
GET https://apiv4.talview.com/mobile/answer/<id>
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Create an Answer (Mobile)
POST /mobile/answer HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Request:
{
"question_id":56652,
"choice":1,
"text":"",
"status":1000,
"question":null
}
JSON Response:
{
"id": 2701497,
"question": {
"id": 56652,
"type": "multiple choice",
"title": "TEST",
"content": {
"text/html": "<p>What is 1+1 ?</p>",
"text/plain": "What is 1+1 ?"
},
"contentRaw": "<p>What is 1+1 ?</p>",
"hint": null,
"choice_1": "<p>1</p>",
"choice_2": "<p>2</p>",
"choice_3": "<p>3</p>",
"choice_4": "<p>4</p>",
"choice_5": null,
"leftList": null,
"rightList": null,
"matchMap": null,
"tagData": null,
"testCase": null,
"updated_at": "2017-11-03 15:47:20+00"
},
"status": 3000,
"answered_at": null,
"choice": 1,
"text": "",
"question_id": 56652,
"max_sequence": 0,
"submitted_files": [],
"_links": {
"question": {
"href": "/attend/question?id=56652"
}
}
}
Create a new answer
HTTP Request
POST https://apiv4.talview.com/mobile/answer
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Body Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
question_id | integer | The question id for which the answer is created | false | |
choice | integer | The choice from the MCQ options | false | |
text | text | The text for the answer in case of subjective questions | false | |
status | integer | The status. (Default value is 1000) | false | |
question | text | The question | false |
Update an Answer (Mobile)
PUT /mobile/answer/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Request for the fields you want to update:
{
"text": "updated text"
}
JSON Response:
{
"id": 2664338,
"question": {
"id": 56659,
"type": "essay",
"title": "TEST",
"content": {
"text/html": "<p>Write a note on the last project which you worked on . </p>",
"text/plain": "Write a note on the last project which you worked on . "
},
"contentRaw": "<p>Write a note on the last project which you worked on . </p>",
"hint": null,
"choice_1": null,
"choice_2": null,
"choice_3": null,
"choice_4": null,
"choice_5": null,
"leftList": null,
"rightList": null,
"matchMap": null,
"tagData": null,
"testCase": null,
"updated_at": "2017-11-03 15:52:17+00"
},
"status": 3000,
"answered_at": "2018-06-14 08:57:12+00",
"choice": null,
"text": "updated text",
"question_id": 56659,
"max_sequence": 0,
"submitted_files": [],
"_links": {
"question": {
"href": "/attend/question?id=56659"
}
}
}
Retrieves the updated details of answer
HTTP Request
PUT https://apiv4.talview.com/mobile/answer/<id>
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Answer File(Mobile)
The answer-file API is used to save the files attached to each question in an essay section. The file can be sent as a multi-part form data.
Create an Answer File (Mobile)
POST /mobile/answer-file HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Request body:
{
"answer_id":56652,
"file":"<file>",
"sequence":1
}
JSON Response:
{
"id": 4817,
"answer_id": 3233,
"sequence": 1,
"file_id": 18086,
"file": {
"id": 18086,
"name": "158504840468767",
"external_id": null,
"description": "",
"pdfUrl": null,
"file_type_id": 25,
"size": null,
"created_at": "2020-03-24 11:13:24+00",
"updated_at": "2020-03-24 11:13:24+00",
"resourceUrl": "https://object.talview.com/test/mani/candidate/essay/158504840468767.csv?X-Amz-Content-Sha256=UNSIGNED-PAYLOAD&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAJ5GZJUFNA7J2GFZQ%2F20200324%2Fap-southeast-1%2Fs3%2Faws4_request&X-Amz-Date=20200324T111327Z&X-Amz-SignedHeaders=host&X-Amz-Expires=518400&X-Amz-Signature=689290da6e39ecc4b7b472017511f155dd4e7d2e7d189c0bb8f91a1293c45d45",
"extension": "csv",
"originalName": "SalesJan2009.csv",
"_links": {
"fileType": {
"href": "/models/file-type/25"
}
}
},
"created_at": "2020-03-24 11:13:27+00",
"updated_at": "2020-03-24 11:13:27+00"
}
Create a new answer file.
HTTP Request
POST https://apiv4.talview.com/mobile/answer-file
Body Parameters
Parameter | Type | Description | is optional |
---|---|---|---|
answer_id | integer | The answer id for which the attachment is created | false |
sequence | integer | The sequence of the answer file | false |
file | binary | The file to be uploaded | false |
Assessment Section (Mobile)
List all Assessment Section (Mobile)
GET /mobile/assessment-section HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 128219,
"section": {
"id": 42389,
"external_id": null,
"title": "Essay Question",
"is_template": false,
"is_default": false,
"evaluation_form_id": null,
"candidateFormUrl": null,
"evaluationFormUrl": null,
"content": null,
"questionsCount": 1,
"type": "Essay",
"form_id": null,
"type_id": 4,
"section_type_id": 4,
"_links": []
},
"is_public": false,
"is_proctor": true,
"is_review": false,
"isSkipAllowed": true,
"is_visible": true,
"is_retake": false,
"start_date": null,
"duration": "36 - 44 minutes",
"end_date": null,
"hasLargeForm": false,
"assessment_id": 21318,
"max_section_time": 600,
"max_question_time": null,
"questionDisplayTime": 3,
"intro": null,
"conclusion": null,
"assessment": {
"id": 21318,
"external_id": null,
"title": "Dummy Assessment",
"description": " \nLorem ipsum dolor sit amet, consectetur adipiscing elit. Quisque blandit eros sit amet lorem varius venenatis. Cras scelerisque dapibus lorem. Ut malesuada orci posuere sollicitudin mollis. Cras nec efficitur quam. Integer neque nisi, rutrum ac lacus at, porta finibus orci. Duis pretium imperdiet nisi, ac luctus nisi ornare quis. Ut interdum commodo ipsum id condimentum. Etiam iaculis suscipit lacus vulputate laoreet. Ut ante risus, malesuada sed eleifend vitae, malesuada ac ex. Aliquam vitae nunc at sem faucibus placerat.\n ",
"promo": null,
"position": {
"id": 16403,
"external_id": null,
"name": "Dummy Assessment",
"designation": null,
"job_requirements": null,
"job_responsibilities": null
},
"organization": {
"id": 488,
"name": "Talview Dev Team Testing",
"logo": "https://assets.talview.com/clients/488/1513147530_logo.png",
"theme": "default",
"timeZone": "Etc/GMT+10",
"promo": null,
"logoutUrl": "/login",
"termsLink": "https://pages.talview.com/terms/"
},
"start_date": "2017-11-03 05:15:00+00",
"status_id": 3,
"customFields": [],
"end_date": "2018-05-10 18:29:00+00",
"theme": "default",
"proviewUrl": "https://cdn.proview.io/init.js",
"proviewToken": "U23A5311",
"created_at": "2017-11-03 15:46:17+00",
"updated_at": "2018-03-06 13:44:45+00",
"formUrl": "https://forms.talview.com/candidate/default/index.html",
"preTemplate": null,
"skin": null
},
"_links": {
"parameters": {
"href": "/models/parameter?section_id=42389"
},
"assessment": {
"href": "/models/assessment/21318"
}
}
}
HTTP Request
GET https://apiv4.talview.com/mobile/assessment-section
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
id | integer | Filter records based on status id | true | |
fields | text | Filter to get specific key from response | true |
Assessment Section Candidate (Mobile)
List all Assessment Section Candidate (Mobile)
GET /mobile/assessment-section-candidate HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 425863,
"external_id": null,
"assessment_candidate_id": 368710,
"timeElapsed": 0,
"isComplete": true,
"section_id": 42389,
"assessment_id": 21318,
"assessment_section_id": 128219,
"c": "2at3HeMPWitZebku94qRIouHfh9VDFvswO~lwj2d7VQxNTI5NDkzNzM4NDI1ODYz",
"preTemplate": "",
"verification_code": "75498121",
"start_date": null,
"externalComplete": false,
"end_date": "2018-06-30 05:55:49+00",
"isRegistered": true,
"intro": null,
"conclusion": null,
"hasBooked": false,
"duration": "10 - 15 minutes",
"started_at": null,
"postAssessmentUrl": null,
"status_id": 13,
"completed_at": "2018-06-20 05:59:27+00",
"status_reason_id": null,
"status": {
"id": 13,
"sort_key": 50,
"label": "Completed",
"type": 20
},
"simulationUrl": "https://gc.talview.com/",
"liveUrl": null,
"questions": [
{
"id": 56659,
"type": "essay",
"title": "TEST",
"content": {
"text/html": "<p>Write a note on the last project which you worked on . </p>",
"text/plain": "Write a note on the last project which you worked on . "
},
"contentRaw": "<p>Write a note on the last project which you worked on . </p>",
"hint": null,
"choice_1": null,
"choice_2": null,
"choice_3": null,
"choice_4": null,
"choice_5": null,
"leftList": null,
"rightList": null,
"matchMap": null,
"tagData": null,
"testCase": null,
"updated_at": "2017-11-03 15:52:17+00"
}
],
"_links": {
"self": {
"href": "/models/assessment-section-candidate?id=425863"
},
"answers": {
"href": "/models/answer?assessment_section_candidate_id=425863"
},
"formInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=425863"
},
"preFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=425863&type%5B0%5D=3&type%5B1%5D=17&type%5B2%5D=18"
},
"evaluationFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=425863&type=2"
},
"evaluations": {
"href": "/evaluation?assessment_section_candidate_id=425863"
},
"panelCandidates": {
"href": "/models/panel-candidate?assessment_section_candidate_id=425863"
},
"status": {
"href": "/status?id=13"
},
"index": {
"href": "/models/assessment-section-candidate"
},
"reports": {
"href": "/models/file?assessment_section_candidate_id=425863&file_type_id%5B0%5D=31&file_type_id%5B1%5D=40"
},
"liveSessions": {
"href": "/models/live-session?assessment_section_candidate_id=425863"
},
"liveSessionsWithRecording": {
"href": "/models/live-session?assessment_section_candidate_id=425863&expand=recordings"
}
}
}
HTTP Request
GET https://apiv4.talview.com/mobile/assessment-section-candidate
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
id | integer | Filter records based on status id | true | |
fields | text | Filter to get specific key from response | true |
Retrieve a Assessment Section Candidate (Mobile)
GET /mobile/assessment-section-candidate/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 425863,
"external_id": null,
"assessment_candidate_id": 368710,
"timeElapsed": 0,
"isComplete": true,
"section_id": 42389,
"assessment_id": 21318,
"assessment_section_id": 128219,
"c": "4I-Iq-GSaIPB1wz2OJQcr3Rtz3tnvsxml1uM8WwgjLIxNTI5NDk0MDE0NDI1ODYz",
"preTemplate": "",
"verification_code": "75498121",
"start_date": null,
"externalComplete": false,
"end_date": "2018-06-30 05:55:49+00",
"isRegistered": true,
"intro": null,
"conclusion": null,
"hasBooked": false,
"duration": "10 - 15 minutes",
"started_at": null,
"postAssessmentUrl": null,
"status_id": 13,
"completed_at": "2018-06-20 05:59:27+00",
"status_reason_id": null,
"status": {
"id": 13,
"sort_key": 50,
"label": "Completed",
"type": 20
},
"simulationUrl": "https://gc.talview.com/",
"liveUrl": null,
"questions": [
{
"id": 56659,
"type": "essay",
"title": "TEST",
"content": {
"text/html": "<p>Write a note on the last project which you worked on . </p>",
"text/plain": "Write a note on the last project which you worked on . "
},
"contentRaw": "<p>Write a note on the last project which you worked on . </p>",
"hint": null,
"choice_1": null,
"choice_2": null,
"choice_3": null,
"choice_4": null,
"choice_5": null,
"leftList": null,
"rightList": null,
"matchMap": null,
"tagData": null,
"testCase": null,
"updated_at": "2017-11-03 15:52:17+00"
}
],
"_links": {
"self": {
"href": "/models/assessment-section-candidate?id=425863"
},
"answers": {
"href": "/models/answer?assessment_section_candidate_id=425863"
},
"formInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=425863"
},
"preFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=425863&type%5B0%5D=3&type%5B1%5D=17&type%5B2%5D=18"
},
"evaluationFormInstances": {
"href": "/models/form-instance?assessment_section_candidate_id=425863&type=2"
},
"evaluations": {
"href": "/evaluation?assessment_section_candidate_id=425863"
},
"panelCandidates": {
"href": "/models/panel-candidate?assessment_section_candidate_id=425863"
},
"status": {
"href": "/status?id=13"
},
"index": {
"href": "/models/assessment-section-candidate"
},
"reports": {
"href": "/models/file?assessment_section_candidate_id=425863&file_type_id%5B0%5D=31&file_type_id%5B1%5D=40"
},
"liveSessions": {
"href": "/models/live-session?assessment_section_candidate_id=425863"
},
"liveSessionsWithRecording": {
"href": "/models/live-session?assessment_section_candidate_id=425863&expand=recordings"
}
}
}
Retrieves the details of assessment section candidate
HTTP Request
GET https://apiv4.talview.com/mobile/assessment-section-candidate/<id>
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Form Submission (Mobile)
List all Form Submission (Mobile)
GET /mobile/form-submission HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
[
{
"id": 263842,
"status": 500,
"form_id": 6600,
"data": [
{
"key": "sharan",
"name": "FirstName",
"label": "First Name",
"value": "sharan"
},
{
"key": "joseph",
"name": "LastName",
"label": "Last Name",
"value": "joseph"
},
{
"key": "sharan.joseph+12@talview.com",
"name": "Email",
"label": "Email",
"value": "sharan.joseph+12@talview.com"
},
{
"key": "652652",
"name": "Phone",
"label": "Phone",
"value": "652652"
}
],
"assessment_section_id": 128219,
"assessment_candidate_id": 368710,
"assessment_section_candidate_id": 425863
}
]
HTTP Request
GET https://apiv4.talview.com/mobile/form-submission
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
id | integer | Filter records based on status id | true | |
fields | text | Filter to get specific key from response | true |
Retrieve a Form Submission (Mobile)
GET /mobile/form-submission/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 263842,
"status": 500,
"form_id": 6600,
"data": [
{
"key": "sharan",
"name": "FirstName",
"label": "First Name",
"value": "sharan"
},
{
"key": "joseph",
"name": "LastName",
"label": "Last Name",
"value": "joseph"
},
{
"key": "sharan.joseph+12@talview.com",
"name": "Email",
"label": "Email",
"value": "sharan.joseph+12@talview.com"
},
{
"key": "652652",
"name": "Phone",
"label": "Phone",
"value": "652652"
}
],
"assessment_section_id": 128219,
"assessment_candidate_id": 368710,
"assessment_section_candidate_id": 425863
}
Retrieves the details of form-submission
HTTP Request
GET https://apiv4.talview.com/mobile/form-submission/<id>
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Update a Form Submission (Mobile)
POST /mobile/form-submission HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Request:
{
"data": [
{
"key": "rtfyghj",
"name": "FirstName",
"label": "First Name",
"value": "sharan"
},
{
"key": "rtfghyjk",
"name": "LastName",
"label": "Last Name",
"value": "rtfghyjk"
},
{
"key": "sharan.joseph+1@talview.com",
"name": "Email",
"label": "Email",
"value": "sharan.joseph+1@talview.com"
},
{
"key": "8945",
"name": "Phone",
"label": "Phone",
"value": "8945"
}
],
"form_id": 6179
}
JSON Response:
{
"id": 264252,
"status": 500,
"form_id": 6179,
"data": [
{
"key": "rtfyghj",
"name": "FirstName",
"label": "First Name",
"value": "sharan"
},
{
"key": "rtfghyjk",
"name": "LastName",
"label": "Last Name",
"value": "rtfghyjk"
},
{
"key": "sharan.joseph+1@talview.com",
"name": "Email",
"label": "Email",
"value": "sharan.joseph+1@talview.com"
},
{
"key": "8945",
"name": "Phone",
"label": "Phone",
"value": "8945"
}
],
"assessment_section_id": 128234,
"assessment_candidate_id": 369490,
"assessment_section_candidate_id": 427264
}
Updates the details of form-submission
HTTP Request
POST https://apiv4.talview.com/mobile/form-submission
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Body Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
data | Array of Object | The array of objects for which the values are to be changed | true | |
form_id | Integer | The form id | true |
Delete a Form Submission (Mobile)
DELETE /mobile/form-submission/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
Deletes the details of form-submission
HTTP Request
DELETE https://apiv4.talview.com/mobile/form-submission/<id>
File Upload
The File Upload endpoint is used for uploading the file in the server.
The File Upload Object
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
file_type_id | integer | The Id of the File Type |
is_public | boolean | If true, the file can be retrieved |
organization_id | integer | The id of the Organization |
status | integer | The status of the file upload.Example: Uploaded, Queued. The Status of the File Upload are described in the list below |
server_id | integer | The Id of the server where the file will be uploaded |
name | text | The name of the File |
extension | text | The extension of the File |
path | string | The path where the File will be uploaded |
modified_by | integer | The id of the User who uploaded the file |
List of File Upload Status:
Status | Key | Description |
---|---|---|
Available | 100 | The file is available in the server |
UnAvailable | 999 | The file is not available in the server |
Queued | 101 | The file is queued for the process |
Processing | 102 | The file is under process |
Complete | 103 | The file upload is complete |
Error | 104 | Error while uploading the file |
Upload a File
POST /mobile/file-upload HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
This end point is used for uploading a file in the server.
HTTP Request
POST https://apiv4.talview.com/mobile/file-upload
Body Parameters
Parameter | Type | Mandatory | Description |
---|---|---|---|
data | text | true | The File (base64 string) |
name | text | false | The name of the File |
file_type_id | integer | false | The file_type_id of the file |
- data attribute is the base64 of the file uploaded.
Form (Mobile)
List all Form (Mobile)
GET /mobile/form HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
[
{
"id": 6600,
"name": "21318",
"structure": [],
"url": null,
"type": 3,
"status": 0,
"start_date": null,
"end_date": null,
"is_public": false,
"article_id": null,
"custom_url": null,
"position_id": null,
"organization_id": 488,
"is_editable": false
}
]
HTTP Request
GET https://apiv4.talview.com/mobile/form
Retrieve a Form (Mobile)
GET /mobile/form/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 6600,
"name": "21318",
"structure": [],
"url": null,
"type": 3,
"status": 0,
"start_date": null,
"end_date": null,
"is_public": false,
"article_id": null,
"custom_url": null,
"position_id": null,
"organization_id": 488,
"is_editable": false
}
Retrieves the details of form
HTTP Request
GET https://apiv4.talview.com/mobile/form/<id>
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Live Attendee (Mobile)
List all Live Attendee (Mobile)
GET /mobile/live-attendee HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 109883,
"live_session_id": 3102,
"email": "asiya.s+live@talview.com",
"phone": null,
"name": "asiya.s+live@talview.com",
"status": null,
"role": 1,
"candidate_id": 21471,
"organization": {
"id": 2,
"name": "company 1 -Public Ltd.",
"logo": "https://assets.talview.com/clients/2/1599735830_logo.png",
"termsLink": "https://www.talview.com/terms",
"privacyLink": "https://www.talview.com/privacy",
"chatbot_enabled": true
},
"postAssessmentUrl": "https://careers.talview.com/",
"c": "DRxW9VRHwD3Kv05B4QEzhGU2NjBmMGI2YWNiZDIwOTFjMWIxMmEwM2FhNGFiZGMxYTg5MTBlNGI0ZjhmNTA0YTdkMTc0MGI0MTg3OTZhZGQPf8K-Y6yG3hhNPAYKQRMb7Orz1tKNr6fSw6E-6mmtOhlDD4vSCG-566DZ1BcKsMs_",
"fullName": "asiya.s+live@talview.com",
"joined_at": "2020-12-17 07:07:27+00",
"user_id": null,
"left_at": "2020-12-17 07:07:27+00",
"passcode": null,
"joinUrl": "https://attend.talview.dev/live-session/invite?c=f7ixWEACiO2IwHXhwv78ADBkZmI3Y2I1ZTVhMmJkYWE4Y2ViZmZiNGJiOTAyNGI5NThiZmU4OGVmMmNmMTk1ODYwZTY3ODA5ZWJmZjEwNDUZYQ3gG9aliEYMUmoUqXSRAok2FKHLVcytzHh8fhdXvF4eNFh66jh9kX1WXX0keT4_",
"created_by": 41,
"updated_by": 41,
"created_at": "2020-12-17 07:07:27+00",
"theme": "default",
"skin": null,
"updated_at": "2020-12-17 07:07:27+00",
"token": "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJjb250ZXh0Ijp7InVzZXIiOnsibmFtZSI6ImFzaXlhLnMrbGl2ZUB0YWx2aWV3LmNvbSIsImVtYWlsIjoiYXNpeWEucytsaXZlQHRhbHZpZXcuY29tIn19LCJhdWQiOiJ0YWx2aWV3LW1lZXQiLCJpc3MiOiJtZWV0NS50YWx2aWV3LmNvbSIsInN1YiI6Im1lZXQtc3RhZ2luZy50YWx2aWV3LmNvbSIsInJvb20iOiIyNzMyMDQ3NTEwIiwiZXhwIjoxNjA4MTk4MDAwfQ.Kzzx1Y-bUx3YVGpq0KCnumNT-Omng4W_40Q0epuPNaw",
"flashJoinUrl": null,
"_links": []
}
HTTP Request
GET https://apiv4.talview.com/mobile/live-attendee
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Retrieve a Live Attendee (Mobile)
GET /mobile/live-attendee/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 12524,
"live_session_id": 3947,
"email": "shivesh.nigam@talview.com",
"phone": null,
"status": 3,
"role": 1,
"candidate_id": 933054,
"organization": {
"id": 488,
"name": "Talview Dev Team Testing",
"logo": "https://assets.talview.com/clients/488/1513147530_logo.png",
"termsLink": "https://pages.talview.com/terms/"
},
"postAssessmentUrl": null,
"c": "lotdxZZo~qJ9OzBT0g~TKzk0YTMwNjZkZGZjNzQxOWI1Mjk3NGE4MTdlN2MwZDUyNTg3YTVlMmU3NmU4NGEzOWNmNTMwYTQ0NTg4YjU4YjeoY5gKzaiCzwl8wEolGUz9jSURmcGY3s8C3MnxN27XLA__",
"fullName": "shivesh.nigam@talview.com",
"joined_at": "2018-06-22 09:14:20+00",
"user_id": null,
"left_at": "2018-06-22 09:14:20+00",
"passcode": null,
"joinUrl": "https://attend.talview.com/live-session/invite?c=je5IlIhdkyqR09rV3MRt6mQxYmRjN2E4Zjk0MjQxZTFhZWQ1YWJmNGVmYzE5M2VkNWExMjUyOWVjMzcwMmRmOTU1MzY5MWI0NWQwNjk0MmFDcfmcqYq-2oefL7enoUFtnZM5494ubguXgvwBH-hzPA__",
"created_by": null,
"updated_by": 1,
"created_at": "2018-06-22 09:14:19+00",
"theme": "default",
"skin": null,
"updated_at": "2018-06-22 09:18:19+00",
"flashJoinUrl": "https://live101.talview.com/bigbluebutton/api/join?meetingID=207061&fullName=shivesh.nigam%40talview.com&password=M13759326&checksum=6ace7765e77c4991a220e3951a2031dc3c099562",
"_links": []
}
Retrieves the details of live attendee
HTTP Request
GET https://apiv4.talview.com/mobile/live-attendee/<id>
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Live Session (Mobile)
The Live Session Object
{
"id": 1665,
"name": "612791",
"is_record": false,
"description": null,
"status": 1,
"starts_at": "2018-03-21 20:30:00+00",
"ends_at": "2018-03-21 21:30:00+00",
"duration": "01:00:00",
"timezone": "Asia/Kolkata",
"assessment_section_candidate_id": null,
"recording_status": null,
"hosted_by": 36209,
"version": 2,
"domain": "meet.talview.com",
"created_at": "2018-03-21 14:38:28+00",
"updated_at": "2018-03-21 14:38:28+00",
"created_by": 36209,
"updated_by": 36209,
"_links": []
Parameter | Type | Description |
---|---|---|
id | integer | The unique identifier of the object |
name | string | The name of the Live Session |
is_record | boolean | Flag to check whether live session recording start/stop feature is enabled for a live session |
description | string | The description of the Live Session |
status | integer | The status of Live Session. Example: Scheduled, In Progress. |
location | string | The location where the live interview is scheduled. Used in offline Live Interview |
ends_at | timestamptz | The time when the Live Session ends |
duration | timestamptz | The duration of the Live Session |
timezone | timestamptz | The timezone of Live Attendee |
assessment_section_candidate_id | integer | The id of the Assessment Section Candidate |
recording_status | integer | The status of the Live Session Recording. Example: Disabled, Processing. |
hosted_by | integer | The id of the Organizer who hosted the Live Session |
domain | string | The URl generated for Live Attendee to redirect to Live Session window |
created_by | integer | The id of the User who created the Live Session |
updated_by | integer | The id of the User who updated the Live Session |
List all Live session (Mobile)
GET /mobile/live-session HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 3947,
"name": "207061",
"is_record": false,
"description": null,
"status": 1,
"starts_at": "2018-06-21 23:30:00+00",
"ends_at": "2018-06-22 00:30:00+00",
"duration": "01:00:00",
"timezone": "Asia/Kolkata",
"assessment_section_candidate_id": 422473,
"recording_status": null,
"hosted_by": 30876,
"version": 2,
"domain": "meet.talview.com",
"created_at": "2018-06-22 09:14:19+00",
"updated_at": "2018-06-22 09:14:19+00",
"created_by": 30876,
"updated_by": 30876,
"_links": []
}
HTTP Request
GET https://apiv4.talview.com/mobile/live-session
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Retrieve a Live Session (Mobile)
GET /mobile/live-session/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 3947,
"name": "207061",
"is_record": false,
"description": null,
"status": 1,
"starts_at": "2018-06-21 23:30:00+00",
"ends_at": "2018-06-22 00:30:00+00",
"duration": "01:00:00",
"timezone": "Asia/Kolkata",
"assessment_section_candidate_id": 422473,
"recording_status": null,
"hosted_by": 30876,
"version": 2,
"domain": "meet.talview.com",
"created_at": "2018-06-22 09:14:19+00",
"updated_at": "2018-06-22 09:14:19+00",
"created_by": 30876,
"updated_by": 30876,
"_links": []
}
Retrieves the details of live session
HTTP Request
GET https://apiv4.talview.com/mobile/live-session/<id>
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Public Assessment Section (Mobile)
Retrieve a Public Assessment Section (Mobile)
GET /mobile/public-assessment-section/<id> HTTP/1.1
Host: https://apiv4.talview.com
JSON Response:
{
"id": 43,
"section": {
"id": 29,
"external_id": null,
"title": "Essay",
"is_template": false,
"is_default": false,
"evaluation_form_id": null,
"candidateFormUrl": null,
"isIframe": false,
"evaluationFormUrl": null,
"content": null,
"isDynamic": false,
"questionsCount": 1,
"type": "Essay",
"form_id": null,
"type_id": 4,
"section_type_id": 4,
"external_assessment_id": null,
"created_at": "2019-10-09 14:51:18+00",
"updated_at": null,
"_links": []
},
"is_public": true,
"is_proctor": true,
"is_review": false,
"isSkipAllowed": true,
"isPlaylist": true,
"hasNegative": true,
"is_visible": true,
"is_retake": false,
"isRetake": false,
"allowedCompiler": null,
"publicLinkUrl": "https://talview.page.link/g2x5",
"start_date": null,
"duration": "0 - 0 minutes",
"end_date": null,
"hasLargeForm": false,
"assessment_id": 8,
"max_section_time": null,
"max_question_time": null,
"questionDisplayTime": 10,
"intro": null,
"conclusion": null,
"positive_mark": null,
"negative_mark": null,
"assessment": {
"id": 8,
"external_id": null,
"title": "Only Essay",
"description": "",
"descriptionHTML": null,
"descriptionText": "",
"promo": null,
"hasLive": false,
"hasLargeForm": false,
"position": null,
"organization": {
"id": 2,
"name": "company 1 -Public Ltd.",
"logo": "https://assets.talview.com/images/talview_grey.png",
"theme": "default",
"timeZone": "Asia/Kolkata",
"promo": null,
"logoutUrl": "/login",
"cssSkin": null,
"candidateIOSBundleID": "com.talview.candidate",
"candidateDroidPackageName": "com.talview.candidate",
"candidateIOSDownloadUrl": "https://itunes.apple.com/in/app/talview-candidate-app/id1015320525?mt=8",
"created_at": "2019-10-09 14:51:18+00",
"isMobileAssessment": true,
"chatbot_enabled": true,
"watermark_enabled": false,
"termsLink": "https://www.talview.com/terms",
"privacyLink": "https://www.talview.com/privacy"
},
"start_date": "2020-12-15 18:30:00+00",
"first_assessment_section_id": 43,
"publicLinkUrl": "https://talview.page.link/g2x5",
"status_id": null,
"end_date": "2020-12-25 18:30:00+00",
"hasMobile": true,
"theme": "default",
"location": null,
"type": null,
"experience": null,
"proviewUrl": "https://cdn.proview.io/init.js",
"proviewToken": "U23A5311",
"created_at": "2019-10-09 14:51:18+00",
"updated_at": "2019-10-09 14:51:18+00",
"formUrl": "https://forms.talview.com/candidate/default/index.html",
"reportUrl": "https://apiv4.talview.com/reports/excel/assessment/8.xls",
"preTemplate": false,
"skin": null
},
"_links": {
"unassigned": {
"href": "/models/assessment-section-candidate?assessment_section_id=43&unassigned=1"
},
"panel": {
"href": "/models/panel?assessment_section_id=43"
},
"parameters": {
"href": "/models/parameter?section_id=29"
},
"assessment": {
"href": "/models/assessment/8"
}
}
}
HTTP Request
GET https://apiv4.talview.com/mobile/public-assessment-section/<id>
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Question (Mobile)
List all Question (Mobile)
GET /mobile/question HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 56659,
"type": "essay",
"title": "TEST",
"content": {
"text/html": "<p>Write a note on the last project which you worked on . </p>",
"text/plain": "Write a note on the last project which you worked on . "
},
"contentRaw": "<p>Write a note on the last project which you worked on . </p>",
"hint": null,
"choice_1": null,
"choice_2": null,
"choice_3": null,
"choice_4": null,
"choice_5": null,
"leftList": null,
"rightList": null,
"matchMap": null,
"tagData": null,
"testCase": null,
"updated_at": "2017-11-03 15:52:17+00"
}
HTTP Request
GET https://apiv4.talview.com/mobile/question
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
id | integer | Filter records based on status id | true | |
fields | text | Filter to get specific key from response | true |
Retrieve a Question (Mobile)
GET /mobile/question/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 56659,
"type": "essay",
"title": "TEST",
"content": {
"text/html": "<p>Write a note on the last project which you worked on . </p>",
"text/plain": "Write a note on the last project which you worked on . "
},
"contentRaw": "<p>Write a note on the last project which you worked on . </p>",
"hint": null,
"choice_1": null,
"choice_2": null,
"choice_3": null,
"choice_4": null,
"choice_5": null,
"leftList": null,
"rightList": null,
"matchMap": null,
"tagData": null,
"testCase": null,
"updated_at": "2017-11-03 15:52:17+00"
}
Retrieves the details of question
HTTP Request
GET https://apiv4.talview.com/mobile/question/<id>
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Register (Mobile)
Create a Register (Mobile)
POST /mobile/register HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Request:
{
"assessment_section_id": 2412800
}
JSON Response:
{
"id": 427731,
"c": "OLRYVL6uWYxSPxjIKaU426lAckDcbsLXIaErC4ralh0xNTI5NjU1MzYwNDI3NzMx"
}
Creates a new answer recording
HTTP Request
POST https://apiv4.talview.com/mobile/register/<id>
Body Parameters
Parameter | Type | Description | required | commnet |
---|---|---|---|---|
assessment_section_id | integer | The public assessment section id | true |
Select Field Data (Mobile)
List all Select Field Data (Mobile)
GET /mobile/select-field-data HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
[
{
"id": 6475518,
"key": "ITSVK|IINS88888888|XXXX88BC8888|XXXX88BC2888|000000000000|000000000000",
"value": "Not Applicable",
"parent_value": "ITSVK|IINS88888888|XXXX88BC8888|XXXX88BC2888|000000000000",
"parent_id": null,
"select_field_type_id": 35,
"sort_order": null,
"updated_by": 31670,
"updated_at": "2018-05-29 14:19:35+00",
"created_at": "2018-05-29 14:19:35+00",
"created_by": 31670
},
{
...
},
{
...
}
]
HTTP Request
GET https://apiv4.talview.com/mobile/select-field-data
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Answer (Mobile)
List all Answer (Mobile)
GET /attend/answer HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 8,
"status": 3000,
"answered_at": "2020-06-08 10:20:36+00",
"choice": null,
"text": "",
"question_id": 8,
"assessment_section_id": 63,
"assessment_candidate_id": 49,
"assessment_section_candidate_id": 100008,
"max_sequence": 0,
"created_at": "2020-06-08 10:20:36+00",
"updated_at": null,
"_links": {
"question": {
"href": "/attend/question?id=8"
}
}
}
HTTP Request
GET https://apiv4.talview.com/attend/answer
Retrieve an Answer (Mobile)
GET /attend/answer/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Response:
{
"id": 8,
"status": 3000,
"answered_at": "2020-06-08 10:20:36+00",
"choice": null,
"text": "",
"question_id": 8,
"assessment_section_id": 63,
"assessment_candidate_id": 49,
"assessment_section_candidate_id": 100008,
"max_sequence": 0,
"created_at": "2020-06-08 10:20:36+00",
"updated_at": null,
"_links": {
"question": {
"href": "/attend/question?id=8"
}
}
}
Retrieves the details of an answer
HTTP Request
GET https://apiv4.talview.com/attend/answer/<id>
Query Parameters
Parameter | Type | Description | is optional | commnet |
---|---|---|---|---|
fields | text | Filter to get specific key from response | true |
Create an Answer (Mobile)
POST /attend/answer HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Request:
{
"question_id":56652,
"choice":1,
"text":"",
"status":1000,
"question":null
}
JSON Response:
{
"id": 8,
"status": 3000,
"answered_at": "2020-06-08 10:20:36+00",
"choice": null,
"text": "",
"question_id": 8,
"assessment_section_id": 63,
"assessment_candidate_id": 49,
"assessment_section_candidate_id": 100008,
"max_sequence": 0,
"created_at": "2020-06-08 10:20:36+00",
"updated_at": null,
"_links": {
"question": {
"href": "/attend/question?id=8"
}
}
}
Create a new answer
HTTP Request
POST https://apiv4.talview.com/attend/answer
Body Parameters
Parameter | Type | Description | is optional |
---|---|---|---|
question_id | integer | The question id for which the answer is created | false |
choice | integer | The choice from the MCQ options | false |
text | text | The text for the answer in case of subjective questions | false |
status | integer | The status. (Default value is 1000) | false |
question | text | The question | false |
Update an Answer (Mobile)
PUT /attend/answer/<id> HTTP/1.1
Host: https://apiv4.talview.com
Authorization: Bearer token
JSON Request for the fields you want to update:
{
"text": "updated text"
}
JSON Response:
{
"id": 8,
"status": 3000,
"answered_at": "2020-06-08 10:20:36+00",
"choice": null,
"text": "",
"question_id": 8,
"assessment_section_id": 63,
"assessment_candidate_id": 49,
"assessment_section_candidate_id": 100008,
"max_sequence": 0,
"created_at": "2020-06-08 10:20:36+00",
"updated_at": null,
"_links": {
"question": {
"href": "/attend/question?id=8"
}
}
}
Retrieves the updated details of an answer
HTTP Request
PUT https://apiv4.talview.com/attend/answer/<id>
Query Parameters
Parameter | Type | Description | is optional |
---|---|---|---|
fields | text | Filter to get specific key from response | true |